消息类型介绍
Spring AI中提供了四种消息角色:
- System Role(系统角色):指导AI的行为和响应风格,设定AI解释和回复输入的参数或规则。这类似于在开始对话之前向AI提供指示。
- User Role(用户角色):表示用户输入的内容,用于描述对AI的提问、命令或陈述信息。该角色构成了AI回应的基础。
- Assistant Role(助理角色):AI对用户输入的响应。它不仅仅是一个答案或反应,它对于维持对话流是至关重要的。通过跟踪AI之前的响应,系统确保了连贯性和上下文相关的互动性。该消息也可能包含函数工具调用请求信息,当需要执行特定功能(如计算、获取数据或其他超出单纯对话的任务)时使用。
- Tool(Function)Role(工具角色): 该侧重于返回响应函数调用的信息。
源码中对消息角色的定义:
java
public enum MessageType {
USER("user"),
ASSISTANT("assistant"),
SYSTEM("system"),
TOOL("tool");
}
通过ChatModel对象发送消息
ChatModel对象通常可以通过调用call()方法发送消息,ChatModel接口中对应的call()方法主要包括:
java
public interface ChatModel extends Model<Prompt, ChatResponse>, StreamingChatModel {
default String call(String message) {
Prompt prompt = new Prompt(new UserMessage(message));
Generation generation = this.call(prompt).getResult();
return generation != null ? generation.getOutput().getText() : "";
}
default String call(Message... messages) {
Prompt prompt = new Prompt(Arrays.asList(messages));
Generation generation = this.call(prompt).getResult();
return generation != null ? generation.getOutput().getText() : "";
}
ChatResponse call(Prompt prompt);
......
}
通过源码可以看到,调用call()方法,内部都会将消息封装为Prompt对象(笔者将Prompt称为提示词对象),其接口内容如下:
java
public class Prompt implements ModelRequest<List<Message>> {
private final List<Message> messages;
@Nullable
private ChatOptions chatOptions;
public Prompt(String contents) {
this((Message)(new UserMessage(contents)));
}
public Prompt(Message message) {
this(Collections.singletonList(message));
}
public Prompt(List<Message> messages) {
this((List)messages, (ChatOptions)null);
}
public Prompt(Message... messages) {
this((List)Arrays.asList(messages), (ChatOptions)null);
}
public Prompt(String contents, @Nullable ChatOptions chatOptions) {
this((Message)(new UserMessage(contents)), chatOptions);
}
public Prompt(Message message, @Nullable ChatOptions chatOptions) {
this(Collections.singletonList(message), chatOptions);
}
public Prompt(List<Message> messages, @Nullable ChatOptions chatOptions) {
Assert.notNull(messages, "messages cannot be null");
Assert.noNullElements(messages, "messages cannot contain null elements");
this.messages = messages;
this.chatOptions = chatOptions;
}
......
}
通过源码可以到,Prompt对象中包含属性为List<Message>(聊天消息的列表)和ChatOptions(大模型属性信息的对象)。
相关接口关系如下:
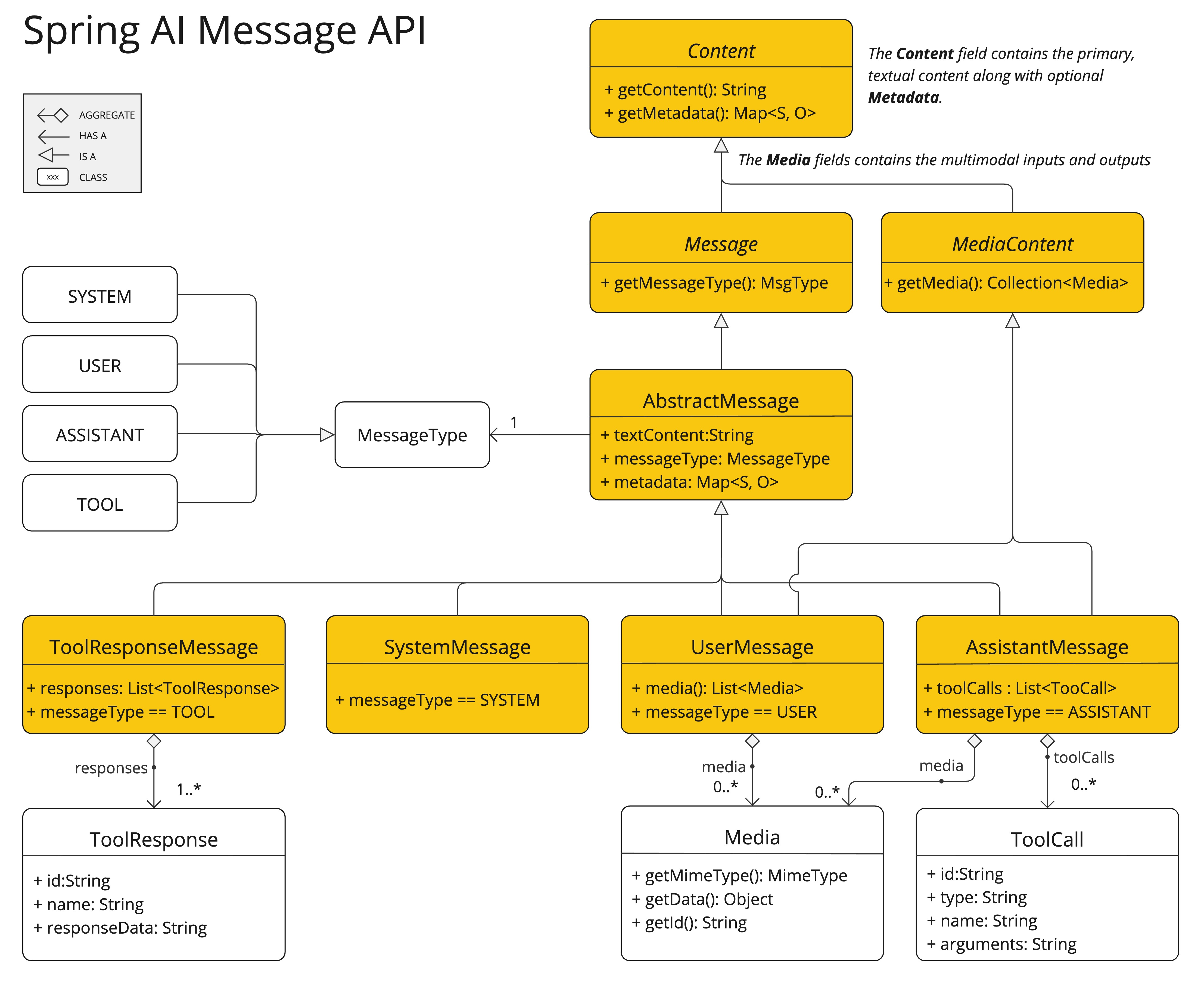
call()参数使用String
java
@GetMapping("/chat")
public String chat(String message) {
String answer = this.chatModel.call(message);
System.out.println(answer);
return "success";
}
call()参数使用Message
java
@GetMapping("/chat")
public String chat(String message) {
// 创建用户消息对象
Message userMessage = new UserMessage(message);
System.out.println(userMessage);
String answer = this.chatModel.call(userMessage);
System.out.println(answer);
return "success";
}
call()参数使用Prompt
java
@GetMapping("/chat")
public String chat(String message) {
Message userMessage = new UserMessage(message);
// 创建提示词对象
Prompt prompt = new Prompt(userMessage);
// 如果参数是Prompt对象,返回值类型为ChatResponse
ChatResponse response = this.chatModel.call(prompt);
// getOutput()方法返回AssistantMessage对象
System.out.println(response.getResult().getOutput().getText());
return "success";
}
提示词模版PromptTemplate
提示词模板主要用于结构化提示的创建,然后将这些提示发送到 AI 模型进行处理。
java
@GetMapping("/chat")
public String chat(String message) {
// PromptTemplate是针对用户信息的模版,对类似的还有SystemPromptTemplate,AssistantPromptTemplate
// {question}相当于占位符
PromptTemplate promptTemplate = new PromptTemplate("针对用户提出的问题:{question},尽量回答的言简意赅");
// 设置占位符对应的内容
Message userMessage = promptTemplate.createMessage(Map.of("question", message));
System.out.println(userMessage);
String answer = this.chatModel.call(userMessage);
System.out.println(answer);
return "success";
}
PromptTemplate是针对用户信息的模版,类似的还有:SystemPromptTemplate,AssistantPromptTemplate。
{question}可以理解为占位符,{}中的占位符名称可以随便定义。在创建消息时,需要对占位符设置替换的内容。
输出UserMessage对象内容如下:

通过ChatClient对象发送消息
ChatClient对象通过prompt()方法设置消息内容,其方法参数如下:
java
public interface ChatClient {
ChatClientRequestSpec prompt();
ChatClientRequestSpec prompt(String content);
ChatClientRequestSpec prompt(Prompt prompt);
......
}
如果使用不带参数的propmt()方法,需要调用额外调用user()、system()方法设置需要发送的信息。
prompt()使用String参数
java
@GetMapping("/chat")
public String chat(String message) {
String answer = this.client.prompt(message).call().content();
System.out.println(answer);
return "success";
}
prompt()使用Prompt参数
java
@GetMapping("/chat")
public String chat(String message) {
Prompt prompt = new Prompt(message);
String answer = this.client.prompt(prompt).call().content();
System.out.println(answer);
return "success";
}
不带参数的prompt()方法
java
@GetMapping("/chat")
public String chat(String message) {
String answer = this.client.prompt().user(message).call().content();
System.out.println(answer);
return "success";
}
使用提示词模版
可以通过PromptTemplate设置,也可以通过user()或者system()方法设置。
java
@GetMapping("/chat")
public String chat(String message) {
String answer = this.client.prompt()
.user(item -> item.text("针对用户的问题:{question},尽量回答的简略")
.param("question", message))
.call().content();
System.out.println(answer);
return "success";
}