Netty在Java网络编程中的应用:实现高性能的异步通信
在当今的分布式系统中,高效、稳定的网络通信是保障系统运行的关键。Java作为一门广泛使用的编程语言,提供了多种网络编程方式,但传统的Socket编程在面对高并发场景时往往显得力不从心。这时,Netty框架以其高性能、高效的异步通信机制脱颖而出。本文将深入探讨Netty在Java网络编程中的应用,通过详细代码示例,展示如何利用Netty实现高性能的异步通信。
一、Netty简介
Netty是一个高性能的网络编程框架,它提供了丰富的API来支持网络应用的开发。与传统Socket相比,Netty具有以下优势:
- 异步非阻塞:Netty采用了NIO (New Input/Output) 的异步非阻塞模式,极大地提高了网络I/O的性能。
- 事件驱动:Netty基于事件驱动模型,允许开发者通过事件回调机制来处理各种网络事件,如连接建立、数据读取、连接断开等,极大地简化了网络编程的复杂性。
- 高度可扩展性:Netty采用责任链模式设计,通过一系列ChannelHandler来处理网络事件,使得开发者可以轻松地扩展和定制功能。
- 丰富的功能组件:Netty提供了各种编解码器、协议栈、线程模型等组件,使得开发高性能、高可靠的网络应用变得更加简单。
二、Netty核心组件
在使用Netty之前,我们需要了解其核心组件,这些组件是构建高性能网络应用的基础。
(一)Channel
Channel是Netty中表示网络连接的抽象类。它封装了网络操作,如读取、写入和连接等。Netty提供了多种类型的Channel,如SocketChannel
用于TCP连接,ServerSocketChannel
用于监听TCP请求等。每个Channel都有一个对应的ChannelPipeline,用于处理网络事件。
(二)EventLoop
EventLoop是Netty中处理I/O事件的线程。它负责监听和处理来自Channel的事件,如数据读取、写入、连接建立等。Netty提供了两种类型的EventLoop:EventLoopGroup
用于管理一组EventLoop线程,通常用于处理客户端事件;BossGroup
用于监听服务端端口,负责接收新连接并将新连接注册到WorkerGroup
的EventLoop中。
(三)ChannelPipeline
ChannelPipeline是处理网络事件的责任链,它由一系列的ChannelHandler组成。当一个网络事件发生时,Netty会将事件逐个传递给Pipeline中的ChannelHandler进行处理。开发者可以通过添加、删除或替换Pipeline中的Handler来定制网络事件的处理逻辑。
(四)ChannelHandler
ChannelHandler是Netty中处理网络事件的核心接口。它定义了一系列方法来处理各种网络事件,如channelRead()
用于处理读取事件,channelActive()
用于处理连接激活事件等。Netty提供了多种类型的ChannelHandler,如ChannelInboundHandler
用于处理入站事件,ChannelOutboundHandler
用于处理出站事件等。
三、Netty实现高性能异步通信
接下来,我们将通过一个简单的聊天服务器和客户端示例来展示如何使用Netty实现高性能的异步通信。
(一)创建Netty聊天服务器
1. 引入依赖
在开始编码之前,我们需要在pom.xml
中添加Netty的依赖:
xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.94.Final</version>
</dependency>
2. 编写服务器启动类
java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class ChatServer {
private int port;
public ChatServer(int port) {
this.port = port;
}
public void run() throws Exception {
// 创建两个线程组,一个用于监听端口,一个用于处理连接
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new ChatServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
// 绑定端口并启动服务器
ChannelFuture f = b.bind(port).sync();
// 等待服务器关闭
f.channel().closeFuture().sync();
} finally {
// 释放线程组资源
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8080;
new ChatServer(port).run();
}
}
3. 编写服务器处理器
java
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.group.ChannelGroup;
import io.netty.channel.group.DefaultChannelGroup;
import io.netty.util.concurrent.GlobalEventExecutor;
public class ChatServerHandler extends SimpleChannelInboundHandler<String> {
// 使用ChannelGroup管理所有客户端连接
private static final ChannelGroup channels = new DefaultChannelGroup(GlobalEventExecutor.INSTANCE);
@Override
public void handlerAdded(ChannelHandlerContext ctx) {
Channel incoming = ctx.channel();
channels.writeAndFlush("[服务器] - " + incoming.remoteAddress() + " 加入聊天室\n");
channels.add(ctx.channel());
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
System.out.println(ctx.channel().remoteAddress() + " 上线了");
}
@Override
public void channelInactive(ChannelHandlerContext ctx) {
System.out.println(ctx.channel().remoteAddress() + " 离线了");
channels.writeAndFlush("[服务器] - " + ctx.channel().remoteAddress() + " 离开了聊天室\n");
}
@Override
public void channelRead0(ChannelHandlerContext ctx, String msg) {
Channel incoming = ctx.channel();
for (Channel channel : channels) {
if (channel != incoming) {
channel.writeAndFlush(incoming.remoteAddress() + " 说:" + msg + "\n");
} else {
channel.writeAndFlush("[自己] " + msg + "\n");
}
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
(二)创建Netty聊天客户端
1. 编写客户端启动类
java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
public class ChatClient {
private String host;
private int port;
public ChatClient(String host, int port) {
this.host = host;
this.port = port;
}
public void run() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChatClientHandler());
// 连接到服务器
ChannelFuture f = b.connect(host, port).sync();
// 等待客户端关闭
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
String host = "127.0.0.1";
int port = 8080;
new ChatClient(host, port).run();
}
}
2. 编写客户端处理器
java
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.socket.SocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
public class ChatClientHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) {
System.out.println(msg);
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
System.out.println("连接到服务器:" + ctx.channel().remoteAddress());
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
// 在客户端启动类中添加编码解码器
public class ChatClientInitializer extends ChannelInitializer<SocketChannel> {
@Override
protected void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new StringDecoder());
ch.pipeline().addLast(new StringEncoder());
ch.pipeline().addLast(new ChatClientHandler());
}
}
四、Netty性能优化技巧
Netty在Java网络编程中的应用:实现高性能的异步通信
在当今的分布式系统中,高效、稳定的网络通信是保障系统运行的关键。Java作为一门广泛使用的编程语言,提供了多种网络编程方式,但传统的Socket编程在面对高并发场景时往往显得力不从心。这时,Netty框架以其高性能、高效的异步通信机制脱颖而出。本文将深入探讨Netty在Java网络编程中的应用,通过详细代码示例,展示如何利用Netty实现高性能的异步通信。
一、Netty简介
Netty是一个高性能的网络编程框架,它提供了丰富的API来支持网络应用的开发。与传统Socket相比,Netty具有以下优势:
- 异步非阻塞:Netty采用了NIO (New Input/Output) 的异步非阻塞模式,极大地提高了网络I/O的性能。
- 事件驱动:Netty基于事件驱动模型,允许开发者通过事件回调机制来处理各种网络事件,如连接建立、数据读取、连接断开等,极大地简化了网络编程的复杂性。
- 高度可扩展性:Netty采用责任链模式设计,通过一系列ChannelHandler来处理网络事件,使得开发者可以轻松地扩展和定制功能。
- 丰富的功能组件:Netty提供了各种编解码器、协议栈、线程模型等组件,使得开发高性能、高可靠的网络应用变得更加简单。
二、Netty核心组件
在使用Netty之前,我们需要了解其核心组件,这些组件是构建高性能网络应用的基础。
(一)Channel
Channel是Netty中表示网络连接的抽象类。它封装了网络操作,如读取、写入和连接等。Netty提供了多种类型的Channel,如SocketChannel
用于TCP连接,ServerSocketChannel
用于监听TCP请求等。每个Channel都有一个对应的ChannelPipeline,用于处理网络事件。
(二)EventLoop
EventLoop是Netty中处理I/O事件的线程。它负责监听和处理来自Channel的事件,如数据读取、写入、连接建立等。Netty提供了两种类型的EventLoop:EventLoopGroup
用于管理一组EventLoop线程,通常用于处理客户端事件;BossGroup
用于监听服务端端口,负责接收新连接并将新连接注册到WorkerGroup
的EventLoop中。
(三)ChannelPipeline
ChannelPipeline是处理网络事件的责任链,它由一系列的ChannelHandler组成。当一个网络事件发生时,Netty会将事件逐个传递给Pipeline中的ChannelHandler进行处理。开发者可以通过添加、删除或替换Pipeline中的Handler来定制网络事件的处理逻辑。
(四)ChannelHandler
ChannelHandler是Netty中处理网络事件的核心接口。它定义了一系列方法来处理各种网络事件,如channelRead()
用于处理读取事件,channelActive()
用于处理连接激活事件等。Netty提供了多种类型的ChannelHandler,如ChannelInboundHandler
用于处理入站事件,ChannelOutboundHandler
用于处理出站事件等。
三、Netty实现高性能异步通信
接下来,我们将通过一个简单的聊天服务器和客户端示例来展示如何使用Netty实现高性能的异步通信。
(一)创建Netty聊天服务器
1. 引入依赖
在开始编码之前,我们需要在pom.xml
中添加Netty的依赖:
xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.94.Final</version>
</dependency>
2. 编写服务器启动类
java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class ChatServer {
private int port;
public ChatServer(int port) {
this.port = port;
}
public void run() throws Exception {
// 创建两个线程组,一个用于监听端口,一个用于处理连接
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new ChatServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
// 绑定端口并启动服务器
ChannelFuture f = b.bind(port).sync();
// 等待服务器关闭
f.channel().closeFuture().sync();
} finally {
// 释放线程组资源
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8080;
new ChatServer(port).run();
}
}
3. 编写服务器处理器
java
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.group.ChannelGroup;
import io.netty.channel.group.DefaultChannelGroup;
import io.netty.util.concurrent.GlobalEventExecutor;
public class ChatServerHandler extends SimpleChannelInboundHandler<String> {
// 使用ChannelGroup管理所有客户端连接
private static final ChannelGroup channels = new DefaultChannelGroup(GlobalEventExecutor.INSTANCE);
@Override
public void handlerAdded(ChannelHandlerContext ctx) {
Channel incoming = ctx.channel();
channels.writeAndFlush("[服务器] - " + incoming.remoteAddress() + " 加入聊天室\n");
channels.add(ctx.channel());
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
System.out.println(ctx.channel().remoteAddress() + " 上线了");
}
@Override
public void channelInactive(ChannelHandlerContext ctx) {
System.out.println(ctx.channel().remoteAddress() + " 离线了");
channels.writeAndFlush("[服务器] - " + ctx.channel().remoteAddress() + " 离开了聊天室\n");
}
@Override
public void channelRead0(ChannelHandlerContext ctx, String msg) {
Channel incoming = ctx.channel();
for (Channel channel : channels) {
if (channel != incoming) {
channel.writeAndFlush(incoming.remoteAddress() + " 说:" + msg + "\n");
} else {
channel.writeAndFlush("[自己] " + msg + "\n");
}
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
(二)创建Netty聊天客户端
1. 编写客户端启动类
java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
public class ChatClient {
private String host;
private int port;
public ChatClient(String host, int port) {
this.host = host;
this.port = port;
}
public void run() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChatClientInitializer());
// 连接到服务器
ChannelFuture f = b.connect(host, port).sync();
// 等待客户端关闭
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
String host = "127.0.0.1";
int port = 8080;
new ChatClient(host, port).run();
}
}
2. 编写客户端初始化类
java
import io.netty.channel.ChannelInitializer;
import io.netty.channel.socket.SocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
public class ChatClientInitializer extends ChannelInitializer<SocketChannel> {
@Override
protected void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new StringDecoder());
ch.pipeline().addLast(new StringEncoder());
ch.pipeline().addLast(new ChatClientHandler());
}
}
3. 编写客户端处理器
java
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
public class ChatClientHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) {
System.out.println(msg);
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
System.out.println("连接到服务器:" + ctx.channel().remoteAddress());
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
四、Netty性能优化技巧
在实际应用中,为了充分发挥Netty的性能优势,我们可以通过以下几种方式进行优化:
(一)线程模型优化
Netty默认的线程模型是基于EventLoopGroup
的多线程模型。对于高并发场景,我们可以根据实际需求调整线程数量。例如,可以将BossGroup和WorkerGroup的线程数量设置为CPU核心数的1.5倍到2倍,以充分利用多核CPU的性能。
java
int cpuCores = Runtime.getRuntime().availableProcessors();
EventLoopGroup bossGroup = new NioEventLoopGroup(cpuCores);
EventLoopGroup workerGroup = new NioEventLoopGroup(cpuCores * 2);
(二)内存管理优化
Netty使用ByteBuf
作为其缓冲区实现,它提供了比传统ByteBuffer
更高效的内存管理和操作。我们可以通过复用ByteBuf
,减少内存分配和垃圾回收的开销。
java
ByteBuf buffer = ByteBufAllocator.DEFAULT.buffer();
try {
// 写入数据
buffer.writeBytes(data);
// 使用数据
channel.writeAndFlush(buffer);
} finally {
buffer.release(); // 手动释放缓冲区
}
(三)零拷贝技术
Netty支持零拷贝技术,通过CompositeByteBuf可以将多个ByteBuf合并成一个逻辑上的ByteBuf,而无需物理上拷贝数据。这在处理大文件传输或消息拼接时非常有用。
java
CompositeByteBuf compositeBuffer = ByteBufAllocator.DEFAULT.compositeBuffer();
compositeBuffer.addComponent(true, headerBuffer);
compositeBuffer.addComponent(true, bodyBuffer);
channel.writeAndFlush(compositeBuffer);
(四)异步操作优化
Netty的异步操作是其高性能的关键。我们可以通过合理使用异步操作,避免同步等待,从而提高系统的吞吐量。例如,可以使用ChannelFuture
的监听器来处理异步操作的结果。
java
ChannelFuture future = channel.writeAndFlush(message);
future.addListener(new ChannelFutureListener() {
@Override
public void operationComplete(ChannelFuture future) {
if (future.isSuccess()) {
System.out.println("消息发送成功");
} else {
System.err.println("消息发送失败:" + future.cause());
}
}
});
五、Netty在实际项目中的应用
Netty因其高性能和易用性,被广泛应用于各种实际项目中。以下是一些常见的应用场景:
(一)即时通讯系统
Netty可以用于构建高性能的即时通讯系统,如企业级的IM系统。通过Netty的异步通信模型,可以轻松处理大量并发连接,并实现消息的快速传递。
(二)游戏服务器
在游戏开发中,Netty常用于构建游戏服务器,处理玩家的连接和数据交互。其高性能和低延迟特性,使得游戏体验更加流畅。
(三)微服务通信
在微服务架构中,Netty可以作为底层通信框架,支持服务之间的高效通信。例如,gRPC就是基于Netty实现的高性能RPC框架。
(四)大数据处理
Netty在大数据处理领域也有应用,如数据采集和传输。其高效的数据处理能力和网络通信能力,使得数据传输更加高效。
六、总结
Netty作为一款高性能的网络编程框架,为Java开发者提供了一种高效、易用的网络编程解决方案。通过本文的介绍和代码示例,我们可以看到Netty在实现高性能异步通信方面的优势。在实际项目中,我们可以根据需求合理使用Netty的各种功能组件和优化技巧,构建出高性能、高可靠的网络应用。
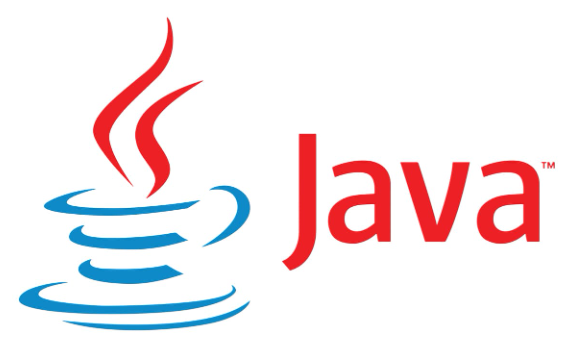