完善地图,加载出装饰品,检测人员与地面的碰撞,检测子弹与岩壁的碰撞,检测手雷与地面的碰撞。
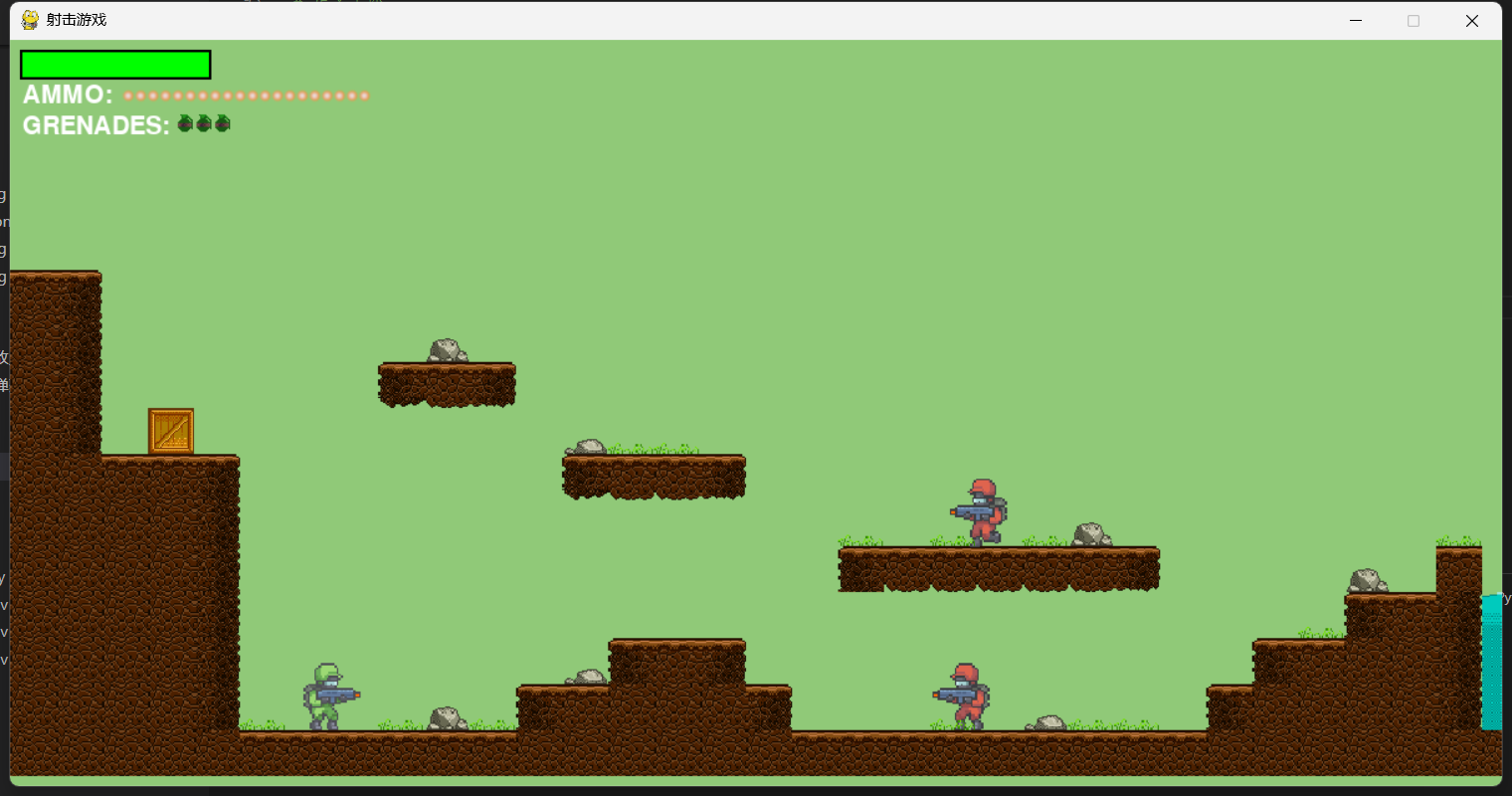
python
import pygame
import sys
import os
import random
import csv
# 初始化Pygame
pygame.init()
# 屏幕宽度
SCREEN_WIDTH = 1200
# 屏幕高度,为屏幕宽度的0.5倍
SCREEN_HEIGHT = int(SCREEN_WIDTH * 0.5)
# 创建一个指定尺寸的显示表面对象
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 设置窗口标题
pygame.display.set_caption('射击游戏')
# 创建一个新的时钟对象来跟踪时间
clock = pygame.time.Clock()
# FPS(每秒帧数):帧率是指一秒内出现的帧数。
# 频率,即连续图像被捕获或显示的频率。
# 设置帧率
FPS = 60
# 定义游戏变量
# 重力值
GRAVITY = 0.75
# 地图的行数
ROWS = 16
# 地图的列数
COLS = 150
# 每个图块的大小,通过屏幕高度除以行数得到
TILE_SIZE = SCREEN_HEIGHT // ROWS
# 图块的类型数量
TILE_TYPES = 21
# 当前关卡数
level = 1
# 定义玩家动作变量
# 是否向左移动
moving_left = False
# 是否向右移动
moving_right = False
# 是否射击
shoot = False
# 是否投掷手雷
grenade = False
# 手雷是否已投掷
grenade_thrown = False
# 将图块存储在列表中
img_list = []
for x in range(TILE_TYPES):
# 加载图块图像
img = pygame.image.load(f'img/tile/{x}.png')
# 缩放图块图像
img = pygame.transform.scale(img, (TILE_SIZE, TILE_SIZE))
img_list.append(img)
# 子弹图像
bullet_img = pygame.image.load('img/icons/bullet.png').convert_alpha()
# 手雷图像
grenade_img = pygame.image.load('img/icons/grenade.png').convert_alpha()
# 生命箱子图像
health_box_img = pygame.image.load('img/icons/health_box.png').convert_alpha()
# 弹药箱子图像
ammo_box_img = pygame.image.load('img/icons/ammo_box.png').convert_alpha()
# 手雷箱子图像
grenade_box_img = pygame.image.load('img/icons/grenade_box.png').convert_alpha()
# 物品箱子字典
item_boxes = {
'Health': health_box_img,
'Ammo': ammo_box_img,
'Grenade': grenade_box_img
}
# 定义颜色
# 背景颜色
BG = (144, 201, 120)
# 红色
RED = (255, 0, 0)
# 白色
WHITE = (255, 255, 255)
# 绿色
GREEN = (0, 255, 0)
# 黑色
BLACK = (0, 0, 0)
# 粉色
PINK = (235, 65, 54)
# 定义字体
# 使用Futura字体,字号为30
font = pygame.font.SysFont('Futura', 30)
def draw_text(text, font, text_col, x, y):
# 创建一个新图像(表面),在上面渲染指定的文本
img = font.render(text, True, text_col)
# 将图像绘制到屏幕上
screen.blit(img, (x, y))
def draw_bg():
# 用背景颜色填充屏幕
screen.fill(BG)
#pygame.draw.line(screen,RED,(0,300),(SCREEN_WIDTH,300))
class Soldier(pygame.sprite.Sprite):
def __init__(self,char_type, x,y,scale,speed,ammo,grenades):
super().__init__()
self.alive = True # 角色是否存活
self.char_type=char_type
self.speed = speed # 移动速度
self.ammo = ammo # 当前弹药量
self.start_ammo = ammo # 初始弹药量
self.shoot_cooldown = 0 # 射击冷却计数器
self.grenades = grenades # 手雷数量
self.health = 100 # 当前生命值
self.max_health = self.health # 最大生命值
self.direction = 1 # 方向(1为右,-1为左)
self.vel_y = 0 # 垂直速度
self.jump = False # 跳跃状态
self.in_air = True # 是否在空中
self.flip = False # 是否翻转图像(左右方向)
self.animation_list = [] # 动画帧列表
self.frame_index = 0 # 当前动画帧索引
self.action = 0 # 当前动作(0:闲置, 1:奔跑, 2:跳跃, 3:死亡)
self.update_time = pygame.time.get_ticks() # 上次更新时间
# 专用变量
self.move_counter = 0 # 移动计数器
self.vision = pygame.Rect(0, 0, 150, 20) # 视野范围
self.idling = False # 是否处于闲置状态
self.idling_counter = 0 # 闲置计时器
# 加载角色所有动画
animation_types = ['Idle', 'Run', 'Jump', 'Death'] # 定义所有动画类型
for animation in animation_types: # 遍历每种动画类型
temp_list = [] # 创建一个临时列表用于存储当前动画类型的帧
# 计算当前动画类型的帧数
num_of_frames = len(os.listdir(f'img/{self.char_type}/{animation}'))
for i in range(num_of_frames): # 遍历每帧
# 加载并缩放图像
img = pygame.image.load(f'img/{self.char_type}/{animation}/{i}.png').convert_alpha()
img = pygame.transform.scale(img, (int(img.get_width() * scale), int(img.get_height() * scale)))
temp_list.append(img) # 将处理后的图像添加到临时列表
self.animation_list.append(temp_list) # 将当前动画类型的全部帧添加到动画列表
# 设置初始图像和碰撞矩形
self.image = self.animation_list[self.action][self.frame_index]
self.rect = self.image.get_rect()
self.rect.center = (x, y)
self.width = self.image.get_width()
self.height = self.image.get_height()
def update(self):
# 更新动画和检查存活状态
self.update_animation()
self.check_alive()
# 更新射击冷却
if self.shoot_cooldown > 0:
self.shoot_cooldown -= 1
def move(self, moving_left, moving_right):
# 初始化移动变量
screen_scroll = 0
dx = 0
dy = 0
# 处理水平移动
if moving_left:
dx = -self.speed
self.flip = True
self.direction = -1
if moving_right:
dx = self.speed
self.flip = False
self.direction = 1
# 处理跳跃
if self.jump and not self.in_air:
self.vel_y = -11
self.jump = False
self.in_air = True
# 应用重力
self.vel_y += GRAVITY
if self.vel_y > 10: # 限制最大下落速度
self.vel_y=10
dy += self.vel_y
# 碰撞检测 - 障碍物
for tile in world.obstacle_list:
# X方向碰撞
if tile[1].colliderect(self.rect.x + dx, self.rect.y, self.width, self.height):
dx = 0
# 如果是敌人碰到墙,转向
if self.char_type == 'enemy':
self.direction *= -1
self.move_counter = 0
# Y方向碰撞
if tile[1].colliderect(self.rect.x, self.rect.y + dy, self.width, self.height):
# 跳跃时碰到天花板
if self.vel_y < 0:
self.vel_y = 0
dy = tile[1].bottom - self.rect.top
# 下落时碰到地面
elif self.vel_y >= 0:
self.vel_y = 0
self.in_air = False
dy = tile[1].top - self.rect.bottom
# 更新位置
self.rect.x += dx
self.rect.y += dy
def shoot(self):
# 射击逻辑:检查冷却和弹药
if self.shoot_cooldown == 0 and self.ammo > 0:
self.shoot_cooldown = 20 # 设置冷却时间
# 创建子弹对象(从角色中心前方发射)
bullet = Bullet(self.rect.centerx + (0.75 * self.rect.size[0] * self.direction),
self.rect.centery, self.direction)
bullet_group.add(bullet)
self.ammo -= 1 # 消耗弹药
def ai(self):
# AI行为逻辑
if self.alive and player.alive:
# 随机进入闲置状态
if not self.idling and random.randint(1, 50) == 1:
self.update_action(0) # 0: 闲置
self.idling = True
self.idling_counter = 50
# 检测是否看到玩家
if self.vision.colliderect(player.rect):
self.update_action(0) # 停止移动,转为闲置
self.shoot() # 向玩家射击
else:
if not self.idling:
# 左右移动逻辑
ai_moving_right = self.direction == 1
ai_moving_left = not ai_moving_right
self.move(ai_moving_left, ai_moving_right)
self.update_action(1) # 1: 奔跑
# 更新视野范围
self.move_counter += 1
self.vision.center = (self.rect.centerx + 75 * self.direction, self.rect.centery)
# 到达边界后转向
if self.move_counter > TILE_SIZE:
self.direction *= -1
self.move_counter = 0
else:
# 闲置计时器递减
self.idling_counter -= 1
if self.idling_counter <= 0:
self.idling = False
def update_animation(self):
# 动画更新逻辑
ANIMATION_COOLDOWN = 100 # 动画帧间隔(毫秒)
# 更新当前帧
self.image = self.animation_list[self.action][self.frame_index]
# 检查是否需要更新帧
if pygame.time.get_ticks() - self.update_time > ANIMATION_COOLDOWN:
self.update_time = pygame.time.get_ticks()
self.frame_index += 1
# 检查是否需要重置动画
if self.frame_index >= len(self.animation_list[self.action]):
if self.action == 3: # 死亡动画停在最后一帧
self.frame_index = len(self.animation_list[self.action]) - 1
else:
self.frame_index = 0
def update_action(self, new_action):
# 切换动作状态
if new_action != self.action:
self.action = new_action
# 重置动画帧
self.frame_index = 0
self.update_time = pygame.time.get_ticks()
def check_alive(self):
# 检查是否存活
if self.health <= 0:
self.health = 0
self.speed = 0
self.alive = False
self.update_action(3) # 3: 死亡动画
def draw(self):
# 绘制角色到屏幕
flipped_image = pygame.transform.flip(self.image, self.flip, False)
screen.blit(flipped_image, self.rect)
class ItemBox(pygame.sprite.Sprite):
def __init__(self, item_type, x, y):
pygame.sprite.Sprite.__init__(self)
self.item_type = item_type
self.image = item_boxes[self.item_type]
self.rect = self.image.get_rect()
self.rect.midtop = (x + TILE_SIZE // 2, y + (TILE_SIZE - self.image.get_height()))
def update(self):
# 检查玩家是否捡起了箱子
if pygame.sprite.collide_rect(self, player):
# 检查是哪种箱子
if self.item_type == 'Health':
player.health += 25
if player.health > player.max_health:
player.health = player.max_health
elif self.item_type == 'Ammo':
player.ammo += 15
elif self.item_type == 'Grenade':
player.grenades += 3
# 删除物品箱
self.kill()
class HealthBar():
def __init__(self, x, y, health, max_health):
self.x = x
self.y = y
self.health = health
self.max_health = max_health
def draw(self, health):
# 更新当前生命值
self.health = health
# 计算生命值比例
ratio = self.health / self.max_health
pygame.draw.rect(screen, BLACK, (self.x - 2, self.y - 2, 154, 24))
pygame.draw.rect(screen, RED, (self.x, self.y, 150, 20))
pygame.draw.rect(screen, GREEN, (self.x, self.y, 150 * ratio, 20))
class Bullet(pygame.sprite.Sprite):
def __init__(self, x, y, direction):
# 初始化子弹精灵
pygame.sprite.Sprite.__init__(self)
self.speed = 10 # 子弹移动速度
self.image = bullet_img # 子弹图像
self.rect = self.image.get_rect() # 获取图像矩形区域
self.rect.center = (x, y) # 设置子弹初始位置
self.direction = direction # 子弹方向(1为右,-1为左)
def update(self):
# 移动子弹
self.rect.x += (self.direction * self.speed)
# 检查子弹是否超出屏幕边界
if self.rect.right < 0 or self.rect.left > SCREEN_WIDTH:
self.kill() # 超出屏幕则销毁子弹
# 检查子弹与地图障碍物的碰撞
for tile in world.obstacle_list:
if tile[1].colliderect(self.rect):
self.kill() # 碰到障碍物则销毁子弹
# 检查子弹与玩家的碰撞
if pygame.sprite.spritecollide(player, bullet_group, False):
if player.alive:
player.health -= 5 # 玩家受伤
self.kill() # 销毁子弹
for enemy in enemy_group:
if pygame.sprite.spritecollide(enemy, bullet_group, False):
if enemy.alive:
enemy.health -= 25 # 敌人受伤
self.kill() # 销毁子弹
class Grenade(pygame.sprite.Sprite):
def __init__(self, x, y, direction):
# 初始化手雷精灵
pygame.sprite.Sprite.__init__(self)
self.timer = 100 # 手雷爆炸计时器
self.vel_y = -11 # 手雷垂直初速度(用于抛射效果)
self.speed = 7 # 手雷水平移动速度
self.image = grenade_img # 手雷图像
self.rect = self.image.get_rect() # 获取图像矩形区域
self.rect.center = (x, y) # 设置手雷初始位置
self.width = self.image.get_width() # 手雷宽度
self.height = self.image.get_height() # 手雷高度
self.direction = direction # 手雷投掷方向(1为右,-1为左)
def update(self):
# 应用重力影响手雷垂直运动
self.vel_y += GRAVITY
dx = self.direction * self.speed # 水平移动距离
dy = self.vel_y # 垂直移动距离
# 检查与地图障碍物的碰撞
for tile in world.obstacle_list:
# 水平方向碰撞检测
if tile[1].colliderect(self.rect.x + dx, self.rect.y, self.width, self.height):
self.direction *= -1 # 碰到墙壁反弹
dx = self.direction * self.speed
# 垂直方向碰撞检测
if tile[1].colliderect(self.rect.x, self.rect.y + dy, self.width, self.height):
self.speed = 0 # 碰撞后停止水平移动
# 向上投掷碰到天花板
if self.vel_y < 0:
self.vel_y = 0
dy = tile[1].bottom - self.rect.top
# 下落时碰到地面
elif self.vel_y >= 0:
self.vel_y = 0
dy = tile[1].top - self.rect.bottom
# 更新手雷位置
self.rect.x += dx
self.rect.y += dy
# 手雷计时器倒计时
self.timer -= 1
if self.timer <= 0:
self.kill() # 销毁手雷
# 创建爆炸效果
explosion = Explosion(self.rect.x, self.rect.y, 0.8)
explosion_group.add(explosion)
# 对手雷一定范围内的角色造成伤害
if abs(self.rect.centerx - player.rect.centerx) < TILE_SIZE * 2 and \
abs(self.rect.centery - player.rect.centery) < TILE_SIZE * 2:
player.health -= 50 # 玩家受伤
# 对敌人造成伤害
for enemy in enemy_group:
if abs(self.rect.centerx - enemy.rect.centerx) < TILE_SIZE * 2 and \
abs(self.rect.centery - enemy.rect.centery) < TILE_SIZE * 2:
enemy.health -= 50 # 敌人受伤
class World():
def __init__(self):
self.obstacle_list = []#障碍列表
def process_data(self, data):
self.level_length = len(data[0])
# 遍历 level_data.csv 文件中的每个值,并创建一个表面矩阵
for y, row in enumerate(data):
for x, tile in enumerate(row):
if tile >= 0:
img = img_list[tile]
img_rect = img.get_rect()
img_rect.x = x * TILE_SIZE
img_rect.y = y * TILE_SIZE
tile_data = (img, img_rect)
if tile >= 0 and tile <= 8:
self.obstacle_list.append(tile_data)
elif tile >= 9 and tile <= 10:
water = Water(img, x * TILE_SIZE, y * TILE_SIZE)
water_group.add(water)
elif tile >= 11 and tile <= 14:
decoration = Decoration(img, x * TILE_SIZE, y * TILE_SIZE)
decoration_group.add(decoration)
elif tile == 15: # 创建玩家
player = Soldier('player', x * TILE_SIZE, y * TILE_SIZE, 1.65, 5, 20, 5)
health_bar = HealthBar(10, 10, player.health, player.health)
elif tile == 16: # 创建敌人
enemy = Soldier('enemy', x * TILE_SIZE, y * TILE_SIZE, 1.65, 2, 20, 0)
enemy_group.add(enemy)
elif tile == 17: # 创建弹药箱
item_box = ItemBox('Ammo', x * TILE_SIZE, y * TILE_SIZE)
item_box_group.add(item_box)
elif tile == 18: # 创建手雷箱
item_box = ItemBox('Grenade', x * TILE_SIZE, y * TILE_SIZE)
item_box_group.add(item_box)
elif tile == 19: # 创建生命箱
item_box = ItemBox('Health', x * TILE_SIZE, y * TILE_SIZE)
item_box_group.add(item_box)
elif tile == 20: # 创建出口
exit = Exit(img, x * TILE_SIZE, y * TILE_SIZE)
exit_group.add(exit)
return player, health_bar
def draw(self):
for tile in self.obstacle_list: # tile = (图像, 矩形) ; rect = (左, 上, 宽, 高)
screen.blit(tile[0], tile[1])
class Decoration(pygame.sprite.Sprite):
def __init__(self, img, x, y):
pygame.sprite.Sprite.__init__(self) # pygame.sprite.Sprite = 可见游戏对象的简单基类
self.image = img
self.rect = self.image.get_rect()
self.rect.midtop = (x + TILE_SIZE // 2, y + (TILE_SIZE - self.image.get_height()))
class Water(pygame.sprite.Sprite):
def __init__(self, img, x, y):
pygame.sprite.Sprite.__init__(self)
self.image = img
self.rect = self.image.get_rect()
self.rect.midtop = (x + TILE_SIZE // 2, y + (TILE_SIZE - self.image.get_height()))
class Exit(pygame.sprite.Sprite):
def __init__(self, img, x, y):
pygame.sprite.Sprite.__init__(self)
self.image = img
self.rect = self.image.get_rect()
self.rect.midtop = (x + TILE_SIZE // 2, y + (TILE_SIZE - self.image.get_height()))
class Explosion(pygame.sprite.Sprite):
def __init__(self, x, y, scale):
pygame.sprite.Sprite.__init__(self)
self.images = []
# 加载爆炸动画的5帧图像
for num in range(1, 6):
img = pygame.image.load(f'img/explosion/exp{num}.png').convert_alpha()
img = pygame.transform.scale(img, (int(img.get_width() * scale), int(img.get_height() * scale)))
self.images.append(img)
self.frame_index = 0 # 当前动画帧索引
self.image = self.images[self.frame_index] # 当前显示的图像
self.rect = self.image.get_rect() # 获取图像的矩形区域
self.rect.center = (x, y) # 设置爆炸位置
self.counter = 0 # 帧计数器
def update(self):
EXPLOSION_SPEED = 4 # 爆炸动画速度(数值越小速度越快)
# 更新爆炸动画
self.counter += 1
if self.counter >= EXPLOSION_SPEED:
self.counter = 0
self.frame_index += 1
# 如果动画播放完毕,则删除爆炸效果
if self.frame_index >= len(self.images):
self.kill()
else:
self.image = self.images[self.frame_index]
# 创建精灵组
enemy_group = pygame.sprite.Group() # 敌人群组
bullet_group = pygame.sprite.Group() # 子弹群组
grenade_group = pygame.sprite.Group() # 手雷群组
explosion_group = pygame.sprite.Group() # 爆炸效果群组
item_box_group = pygame.sprite.Group() # 物品箱子群组
decoration_group = pygame.sprite.Group() # 装饰群组
water_group = pygame.sprite.Group() # 水群组
exit_group = pygame.sprite.Group() # 出口群组
#item_box=ItemBox("Health",100,260)
#item_box_group.add(item_box)
#item_box=ItemBox("Ammo",400,260)
#item_box_group.add(item_box)
#item_box=ItemBox("Grenade",500,260)
#item_box_group.add(item_box)
#player =Soldier("player",200,200,1.65,5,10,5)
#health_bar=HealthBar(10,10,player.health,player.health)
#enemy =Soldier("enemy",400,270,1.65,5,30,0)
#enemy1 =Soldier("enemy",600,270,1.65,5,30,0)
#enemy_group.add(enemy)
#enemy_group.add(enemy1)
# 创建空的地图数据列表
world_data = []
for row in range(ROWS):
r = [-1] * COLS
world_data.append(r)
# 加载关卡数据并创建游戏世界
with open(f'level{level}_data.csv', newline='') as csvfile:
reader = csv.reader(csvfile, delimiter=',')
for x, row in enumerate(reader):
for y, tile in enumerate(row):
world_data[x][y] = int(tile)
world = World()
player, health_bar = world.process_data(world_data)
run = True
while run: # 游戏主循环
clock.tick(FPS) # 设置帧率
draw_bg()
world.draw()
# 显示玩家生命值
health_bar.draw(player.health)
# 显示弹药数量
draw_text('AMMO: ', font, WHITE, 10, 35)
for x in range(player.ammo):
screen.blit(bullet_img, (90 + (x * 10), 40))
# 显示手雷数量
draw_text('GRENADES: ', font, WHITE, 10, 60)
for x in range(player.grenades):
screen.blit(grenade_img, (135 + (x * 15), 60))
player.update()
player.draw()
for enemy in enemy_group:
enemy.ai()
enemy.update() # 更新敌人状态
enemy.draw() # 绘制敌人
# 更新并绘制各个群组
bullet_group.update()
grenade_group.update()
explosion_group.update()
item_box_group.update()
decoration_group.update()
water_group.update()
exit_group.update()
bullet_group.draw(screen)
grenade_group.draw(screen)
explosion_group.draw(screen)
item_box_group.draw(screen)
decoration_group.draw(screen)
water_group.draw(screen)
exit_group.draw(screen)
# 更新玩家动作
if player.alive:
# 射击子弹
if shoot:
player.shoot()
# 投掷手雷
elif grenade and grenade_thrown == False and player.grenades > 0:
grenade = Grenade(player.rect.centerx + (0.5 * player.rect.size[0] * player.direction),
player.rect.top, player.direction)
grenade_group.add(grenade)
# 减少手雷数量
player.grenades -= 1
grenade_thrown = True
if player.in_air:
player.update_action(2) # 2: 跳跃动作
elif moving_left or moving_right:
player.update_action(1) # 1: 奔跑动作
else:
player.update_action(0) # 0: 闲置动作
player.move(moving_left, moving_right) # 移动玩家
for event in pygame.event.get():
# 处理退出游戏事件
if event.type == pygame.QUIT:
run = False
sys.exit()
# 处理键盘按下事件
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_a or event.key == pygame.K_LEFT: # 按下A键或左键 => 向左移动
moving_left = True
if event.key == pygame.K_d or event.key == pygame.K_RIGHT: # 按下D键或右键 => 向右移动
moving_right = True
if event.key == pygame.K_SPACE: # 按下空格键 => 射击
shoot = True
if event.key == pygame.K_q or event.key == pygame.K_g: # 按下Q键或G键 => 投掷手雷
grenade = True
if (event.key == pygame.K_w or event.key == pygame.K_UP) and player.alive: # 按下W键或上键且玩家存活 => 跳跃
player.jump = True
if event.key == pygame.K_ESCAPE: # 按下ESC键 => 关闭窗口
run = False
# 处理键盘释放事件
if event.type == pygame.KEYUP:
if event.key == pygame.K_a or event.key == pygame.K_LEFT:
moving_left = False
if event.key == pygame.K_d or event.key == pygame.K_RIGHT:
moving_right = False
if event.key == pygame.K_SPACE:
shoot = False
if event.key == pygame.K_q or event.key == pygame.K_g:
grenade = False
grenade_thrown = False
pygame.display.update() # 更新屏幕显示