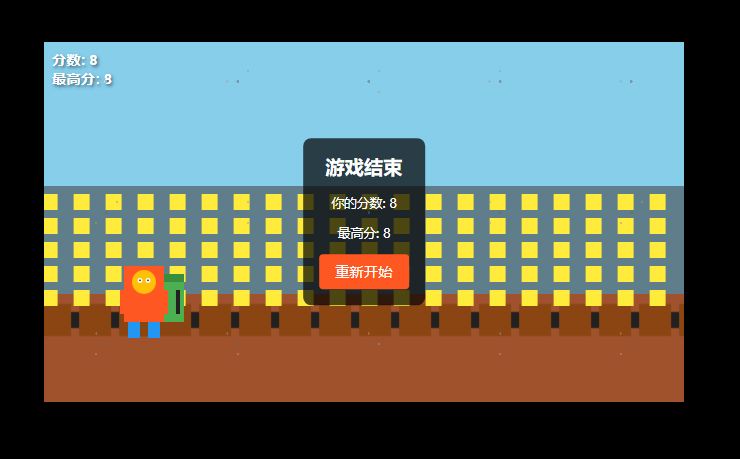
以下是一个简化版的"地铁快跑"小游戏H5页面实现。这个游戏包含基本的角色跳跃、障碍物生成和计分系统,使用Canvas绘图技术实现。
完整源码
登录后复制
plain
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=no">
<title>地铁快跑</title>
<style>
body {
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #000;
overflow: hidden;
}
canvas {
display: block;
background-color: #f0f0f0;
}
.game-container {
position: relative;
}
.game-info {
position: absolute;
top: 10px;
left: 10px;
color: white;
font-family: Arial, sans-serif;
font-size: 18px;
font-weight: bold;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.5);
z-index: 10;
}
.game-over {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: rgba(0, 0, 0, 0.7);
color: white;
padding: 20px;
text-align: center;
border-radius: 10px;
display: none;
z-index: 20;
}
.game-over h2 {
margin-top: 0;
}
.start-screen {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.8);
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
color: white;
font-family: Arial, sans-serif;
z-index: 30;
}
.start-screen h1 {
font-size: 36px;
margin-bottom: 20px;
}
.start-button {
background-color: #ff5722;
color: white;
border: none;
padding: 10px 20px;
font-size: 18px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s;
}
.start-button:hover {
background-color: #f4511e;
}
.restart-button {
background-color: #ff5722;
color: white;
border: none;
padding: 10px 20px;
font-size: 18px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s;
}
.restart-button:hover {
background-color: #f4511e;
}
</style>
</head>
<body>
<div class="game-container">
<canvas id="gameCanvas"></canvas>
<div class="game-info">
<div id="scoreDisplay">分数: 0</div>
<div id="highScoreDisplay">最高分: 0</div>
</div>
<div class="game-over" id="gameOverScreen">
<h2>游戏结束</h2>
<p>你的分数: <span id="finalScore">0</span></p>
<p>最高分: <span id="finalHighScore">0</span></p>
<button class="restart-button" id="restartButton">重新开始</button>
</div>
<div class="start-screen" id="startScreen">
<h1>地铁快跑</h1>
<p>点击或按空格键跳跃</p>
<button class="start-button" id="startButton">开始游戏</button>
</div>
</div>
<script>
// 游戏常量
const GAME_WIDTH = 800;
const GAME_HEIGHT = 450;
const GRAVITY = 0.8;
const JUMP_FORCE = -15;
const PLAYER_WIDTH = 50;
const PLAYER_HEIGHT = 70;
const OBSTACLE_WIDTH = 30;
const OBSTACLE_HEIGHT = 60;
const BACKGROUND_SPEED = 3;
const INITIAL_OBSTACLE_SPEED = 5;
const MAX_OBSTACLE_SPEED = 12;
const OBSTACLE_SPEED_INCREMENT = 0.001;
const OBSTACLE_MIN_DISTANCE = 200;
const OBSTACLE_MAX_DISTANCE = 500;
// 游戏状态
let gameState = {
running: false,
score: 0,
highScore: localStorage.getItem('subwaySurfersHighScore') || 0,
player: {
x: 100,
y: GAME_HEIGHT - PLAYER_HEIGHT - 100,
width: PLAYER_WIDTH,
height: PLAYER_HEIGHT,
velocityY: 0,
isJumping: false
},
obstacles: [],
background: {
x: 0,
speed: BACKGROUND_SPEED
},
obstacleSpeed: INITIAL_OBSTACLE_SPEED,
lastObstaclePosition: 0
};
// 获取DOM元素
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const scoreDisplay = document.getElementById('scoreDisplay');
const highScoreDisplay = document.getElementById('highScoreDisplay');
const gameOverScreen = document.getElementById('gameOverScreen');
const finalScore = document.getElementById('finalScore');
const finalHighScore = document.getElementById('finalHighScore');
const restartButton = document.getElementById('restartButton');
const startScreen = document.getElementById('startScreen');
const startButton = document.getElementById('startButton');
// 设置Canvas尺寸
canvas.width = GAME_WIDTH;
canvas.height = GAME_HEIGHT;
// 更新分数显示
function updateScoreDisplay() {
scoreDisplay.textContent = `分数: ${Math.floor(gameState.score)}`;
highScoreDisplay.textContent = `最高分: ${gameState.highScore}`;
}
// 绘制玩家
function drawPlayer() {
const { x, y, width, height } = gameState.player;
// 绘制玩家主体
ctx.fillStyle = '#ff5722';
ctx.fillRect(x, y, width, height);
// 绘制头部
ctx.fillStyle = '#ffc107';
ctx.beginPath();
ctx.arc(x + width / 2, y + 20, 15, 0, Math.PI * 2);
ctx.fill();
// 绘制眼睛
ctx.fillStyle = 'white';
ctx.beginPath();
ctx.arc(x + width / 2 - 5, y + 18, 3, 0, Math.PI * 2);
ctx.arc(x + width / 2 + 5, y + 18, 3, 0, Math.PI * 2);
ctx.fill();
// 绘制瞳孔
ctx.fillStyle = 'black';
ctx.beginPath();
ctx.arc(x + width / 2 - 5, y + 18, 1, 0, Math.PI * 2);
ctx.arc(x + width / 2 + 5, y + 18, 1, 0, Math.PI * 2);
ctx.fill();
// 绘制手臂
ctx.fillStyle = '#ff5722';
ctx.fillRect(x - 5, y + 30, 10, 30);
ctx.fillRect(x + width - 5, y + 30, 10, 30);
// 绘制腿部
ctx.fillStyle = '#2196f3';
ctx.fillRect(x + 5, y + height, 15, 20);
ctx.fillRect(x + width - 20, y + height, 15, 20);
}
// 绘制障碍物
function drawObstacles() {
gameState.obstacles.forEach(obstacle => {
// 绘制障碍物主体
ctx.fillStyle = '#4caf50';
ctx.fillRect(obstacle.x, obstacle.y, obstacle.width, obstacle.height);
// 绘制障碍物顶部
ctx.fillStyle = '#388e3c';
ctx.fillRect(obstacle.x, obstacle.y, obstacle.width, 10);
// 绘制障碍物细节
ctx.fillStyle = '#212121';
ctx.fillRect(obstacle.x + 5, obstacle.y + 20, 5, 30);
ctx.fillRect(obstacle.x + 20, obstacle.y + 20, 5, 30);
});
}
// 绘制背景
function drawBackground() {
// 绘制天空
ctx.fillStyle = '#87ceeb';
ctx.fillRect(0, 0, GAME_WIDTH, GAME_HEIGHT * 0.7);
// 绘制地面
ctx.fillStyle = '#a0522d';
ctx.fillRect(0, GAME_HEIGHT * 0.7, GAME_WIDTH, GAME_HEIGHT * 0.3);
// 绘制轨道
ctx.fillStyle = '#212121';
ctx.fillRect(0, GAME_HEIGHT * 0.75, GAME_WIDTH, 20);
// 绘制轨道上的枕木
ctx.fillStyle = '#8b4513';
for (let i = Math.floor(-gameState.background.x / 50); i < GAME_WIDTH / 50 + 1; i++) {
ctx.fillRect((i * 50 + gameState.background.x) % GAME_WIDTH, GAME_HEIGHT * 0.75 - 10, 40, 40);
}
// 绘制远处的建筑物
ctx.fillStyle = '#607d8b';
ctx.fillRect(0, GAME_HEIGHT * 0.4, GAME_WIDTH, GAME_HEIGHT * 0.3);
// 绘制建筑物窗户
ctx.fillStyle = '#ffeb3b';
for (let i = 0; i < 20; i++) {
for (let j = 0; j < 5; j++) {
ctx.fillRect(
(i * 40 + gameState.background.x * 0.5) % GAME_WIDTH,
GAME_HEIGHT * 0.4 + 10 + j * 30,
20,
20
);
}
}
}
// 更新玩家位置
function updatePlayer(deltaTime) {
const { player } = gameState;
// 应用重力
player.velocityY += GRAVITY;
player.y += player.velocityY;
// 限制玩家在地面上
if (player.y > GAME_HEIGHT - player.height - 100) {
player.y = GAME_HEIGHT - player.height - 100;
player.velocityY = 0;
player.isJumping = false;
}
}
// 生成障碍物
function generateObstacles() {
if (gameState.obstacles.length === 0) {
// 游戏开始时生成第一个障碍物
gameState.obstacles.push({
x: GAME_WIDTH,
y: GAME_HEIGHT - OBSTACLE_HEIGHT - 100,
width: OBSTACLE_WIDTH,
height: OBSTACLE_HEIGHT
});
gameState.lastObstaclePosition = GAME_WIDTH;
} else {
// 计算最后一个障碍物的位置
const lastObstacle = gameState.obstacles[gameState.obstacles.length - 1];
// 随机决定是否生成新障碍物
if (GAME_WIDTH - lastObstacle.x > gameState.lastObstaclePosition +
Math.random() * (OBSTACLE_MAX_DISTANCE - OBSTACLE_MIN_DISTANCE) +
OBSTACLE_MIN_DISTANCE) {
gameState.obstacles.push({
x: GAME_WIDTH,
y: GAME_HEIGHT - OBSTACLE_HEIGHT - 100,
width: OBSTACLE_WIDTH,
height: OBSTACLE_HEIGHT
});
gameState.lastObstaclePosition = GAME_WIDTH - lastObstacle.x;
}
}
}
// 更新障碍物位置
function updateObstacles(deltaTime) {
// 增加障碍物速度
gameState.obstacleSpeed = Math.min(
gameState.obstacleSpeed + OBSTACLE_SPEED_INCREMENT * deltaTime,
MAX_OBSTACLE_SPEED
);
// 移动所有障碍物
gameState.obstacles.forEach(obstacle => {
obstacle.x -= gameState.obstacleSpeed;
});
// 移除离开屏幕的障碍物
gameState.obstacles = gameState.obstacles.filter(obstacle => {
return obstacle.x + obstacle.width > 0;
});
}
// 更新背景
function updateBackground(deltaTime) {
gameState.background.x -= gameState.background.speed;
if (gameState.background.x < -50) {
gameState.background.x = 0;
}
}
// 检测碰撞
function checkCollision() {
const { player } = gameState;
for (let i = 0; i < gameState.obstacles.length; i++) {
const obstacle = gameState.obstacles[i];
// 简单的矩形碰撞检测
if (player.x < obstacle.x + obstacle.width &&
player.x + player.width > obstacle.x &&
player.y < obstacle.y + obstacle.height &&
player.y + player.height > obstacle.y) {
return true; // 碰撞发生
}
}
return false; // 没有碰撞
}
// 游戏结束
function gameOver() {
gameState.running = false;
// 更新最高分
if (Math.floor(gameState.score) > gameState.highScore) {
gameState.highScore = Math.floor(gameState.score);
localStorage.setItem('subwaySurfersHighScore', gameState.highScore);
}
// 显示游戏结束屏幕
finalScore.textContent = Math.floor(gameState.score);
finalHighScore.textContent = gameState.highScore;
gameOverScreen.style.display = 'block';
}
// 重置游戏
function resetGame() {
gameState = {
running: true,
score: 0,
highScore: gameState.highScore,
player: {
x: 100,
y: GAME_HEIGHT - PLAYER_HEIGHT - 100,
width: PLAYER_WIDTH,
height: PLAYER_HEIGHT,
velocityY: 0,
isJumping: false
},
obstacles: [],
background: {
x: 0,
speed: BACKGROUND_SPEED
},
obstacleSpeed: INITIAL_OBSTACLE_SPEED,
lastObstaclePosition: 0
};
updateScoreDisplay();
gameOverScreen.style.display = 'none';
startScreen.style.display = 'none';
// 开始游戏循环
lastTime = performance.now();
requestAnimationFrame(gameLoop);
}
// 玩家跳跃
function jump() {
if (!gameState.player.isJumping && gameState.running) {
gameState.player.velocityY = JUMP_FORCE;
gameState.player.isJumping = true;
}
}
// 键盘事件处理
document.addEventListener('keydown', function(event) {
if (event.code === 'Space') {
if (!gameState.running && gameOverScreen.style.display === 'block') {
resetGame();
} else if (!gameState.running && startScreen.style.display === 'block') {
resetGame();
} else {
jump();
}
}
});
// 触摸事件处理
canvas.addEventListener('touchstart', function(event) {
event.preventDefault();
if (!gameState.running && gameOverScreen.style.display === 'block') {
resetGame();
} else if (!gameState.running && startScreen.style.display === 'block') {
resetGame();
} else {
jump();
}
});
// 按钮点击事件
startButton.addEventListener('click', resetGame);
restartButton.addEventListener('click', resetGame);
// 游戏循环变量
let lastTime = 0;
// 游戏主循环
function gameLoop(timestamp) {
if (!gameState.running) return;
const deltaTime = timestamp - lastTime;
lastTime = timestamp;
// 清空画布
ctx.clearRect(0, 0, GAME_WIDTH, GAME_HEIGHT);
// 更新游戏状态
updateBackground(deltaTime);
updatePlayer(deltaTime);
generateObstacles();
updateObstacles(deltaTime);
gameState.score += deltaTime * 0.01;
// 绘制游戏元素
drawBackground();
drawObstacles();
drawPlayer();
// 更新分数显示
updateScoreDisplay();
// 检测碰撞
if (checkCollision()) {
gameOver();
}
// 继续游戏循环
requestAnimationFrame(gameLoop);
}
// 初始化游戏
updateScoreDisplay();
</script>
</body>
</html>
这个简化版的"地铁快跑"小游戏包含以下特点:
- 基本游戏机制:玩家可以跳跃躲避障碍物
- 视觉元素:包括背景、玩家角色和障碍物
- 计分系统:根据游戏时间计算分数,并保存最高分
- 难度递增:随着游戏进行,障碍物移动速度会逐渐增加
- 响应式控制:支持键盘空格键和触摸屏点击跳跃
- 游戏状态:开始界面、游戏界面和结束界面
游戏操作很简单:点击"开始游戏"按钮或按空格键开始,然后通过点击或按空格键让角色跳跃,避开障碍物。当角色碰到障碍物时,游戏结束,可以点击"重新开始"按钮再次游戏。
这个实现是简化版的,没有包含原版游戏的所有功能,但已经具备了核心的游戏体验。你可以直接运行代码开始游戏。