ioLibrary库下载地址:文件下载地址:https://gitee.com/wiznet-hk/STM32F10x_W5500_Examples
源文件下载地址:https://gitee.com/wiznet-hk
没有注册的,只能复制粘贴了。
本文介绍了如何初始化STM32的硬件资源,配置W5500的网络参数,并通过DHCP获取动态IP地址。并使用这个IP地址配置TCP客户端,以及双方交换数据的测试。
前篇文中有wiz_platform.c和wiz_platform.h,用来初始化SPI,RST引脚和TIM2,这里就不用粘贴了。
前篇文中有wiz_interface.h,用来通过DHCP获取动态IP地址,这里也不用粘贴了。
1、W5500_Variable.c和.h文件
#include "W5500_Variable.h"
#include "socket.h" // Just include one header for WIZCHIP
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
#include "string.h" //使能strcpy(),strlen(),memset()
//W5500的网络参数
//本地物理地址:00 08 DC 11 11 11
//本地IP地址:192.168.1.199
//本地子网掩码: 255.255.255.0
//本地网关:192.168.1.1
//DNS服务器IP地址:8.8.8.8
//通过DHCP获取动态IP地址
/* network information */
wiz_NetInfo default_net_info = {
{0x00, 0x08, 0xdc,0x11, 0x11, 0x11},
{192, 168, 1, 199},
{255,255,255,0},
{192, 168, 1, 1},
{8,8,8,8},
NETINFO_DHCP}; //动态IP
wiz_NetInfo net_info;
uint8_t ethernet_buf[ETHERNET_BUF_MAX_SIZE] = {0};
//端口0的网络参数
uint16_t LocalPort0=5000; //端口0的本地端口号(5000)
uint8_t dest_ip[4]={192,168,1,190}; //端口0的远程IP地址:192.168.1.190
uint16_t dest_port=6000; //端口0的远程端口号:6000
#ifndef _W5500_Variable_H
#define _W5500_Variable_H
#include "stm32f10x.h"//使能uint8_t,uint16_t,uint32_t,uint64_t,int8_t,int16_t,int32_t,int64_t
#include "wizchip_conf.h"
extern wiz_NetInfo default_net_info;
extern wiz_NetInfo net_info;
#define ETHERNET_BUF_MAX_SIZE (1024 * 2)
extern uint8_t ethernet_buf[ETHERNET_BUF_MAX_SIZE];
extern uint16_t LocalPort0; //端口0的本地端口号(5000)
extern uint8_t dest_ip[4]; //端口0的远程IP地址:192.168.1.190
extern uint16_t dest_port; //端口0的远程端口号:6000
#endif
2、TestTcpClient.c和.h文件
#include "TestTcpClient.h"
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
#include "w5500.h"
#include "W5500_Variable.h"
#include "socket.h"
/**
* @brief tcp client loopback test
* @param sn: socket number
* @param buf: Data sending and receiving cache
* @param destip: Destination IP address
* @param destport: Destination port
* @return value for SOCK_ERRORs,return 1:no error
*/
int32_t loop_TestTcpClient(uint8_t sn, uint8_t* buf, uint8_t* destip, uint16_t destport)
{
int32_t ret; // return value for SOCK_ERRORs
uint16_t size = 0, sentsize=0;
uint16_t any_port = 50000;//配置本地端口为50000
switch(getSn_SR(sn))//获取W5500端口sn的状态寄存器
{
case SOCK_ESTABLISHED ://W5500端口sn已经连接成功
if(getSn_IR(sn) & Sn_IR_CON)
{//读端口sn的Sn_IR中断标志寄存器的bit0
#ifdef _LOOP_TestTcpClient_DEBUG_
printf("%d:Connected to - %d.%d.%d.%d : %d\r\n",sn, destip[0], destip[1], destip[2], destip[3], destport);
#endif
setSn_IR(sn, Sn_IR_CON); // this interrupt should be write the bit cleared to '1'
//回写端口sn的Sn_IR中断标志寄存器的bit0,清除中断标志
}
if((size = getSn_RX_RSR(sn)) > 0)//读端口sn的Sn_RX_RSR寄存器,获取该端口的接收缓冲区的数据长度
{
if(size > ETHERNET_BUF_MAX_SIZE) size = ETHERNET_BUF_MAX_SIZE; // ETHERNET_BUF_MAX_SIZE means user defined buffer size (array)
ret = recv(sn, buf, size);//读"W5500端口sn"的数据,长度为size个字节,保存到buf
if(ret > 0)
{
buf[ret]='\0'; //添加字符串结束符
printf("recv: %s\n",buf); // print the receive data
}
else return ret; //接收数据错误
size = (uint16_t) ret;
sentsize = 0;
while(size != sentsize)
{
ret = send(sn, buf+sentsize, size-sentsize);
//将(buf+sentsize)为首地址的存储单元的前len个字节通过"W5500端口sn"发送出去
// Data send process (User's buffer -> Destination through H/W Tx socket buffer)
if(ret < 0) // Send Error occurred (sent data length < 0)
{
close(sn); //关闭端口sn的连接, socket close
return ret;
}
else
{
printf("send: %s\n",buf+sentsize); // print the send data
}
sentsize += ret; // Don't care SOCKERR_BUSY, because it is zero.
}
}
//
break;
case SOCK_CLOSE_WAIT ://W5500端口sn处于等待关闭状态
#ifdef _LOOP_TestTcpClient_DEBUG_
printf("%d:CloseWait\r\n",sn);
#endif
if((ret=disconnect(sn)) != SOCK_OK)//关闭端口sn的连接
return ret;
#ifdef _LOOP_TestTcpClient_DEBUG_
printf("%d:Socket Closed\r\n", sn);
#endif
break;
case SOCK_INIT ://W5500端口sn已经初始化
#ifdef _LOOP_TestTcpClient_DEBUG_
printf("%d:Try to connect to the %d.%d.%d.%d : %d\r\n", sn, destip[0], destip[1], destip[2], destip[3], destport);
#endif
if( (ret = connect(sn, destip, destport)) != SOCK_OK)
{//将W5500端口sn连接到远程IP地址destip和远程端口destport上
// Try to TCP connect to the TCP server (destination)
return ret;
}
break;
case SOCK_CLOSED://W5500端口sn处于关闭状态
close(sn);//关闭端口sn的连接, socket close
if((ret=socket(sn, Sn_MR_TCP, any_port++, 0x00)) != sn)
{//修改端口,执行连接服务器
if(any_port == 0xffff) any_port = 50000;
return ret; // TCP socket open with 'any_port' port number
}
#ifdef _LOOP_TestTcpClient_DEBUG_
printf("%d:TCP client loopback start\r\n",sn);
printf("%d:Socket opened\r\n",sn);
#endif
break;
default:
break;
}
return 1;
}
#ifndef _TestTcpClient_H
#define _TestTcpClient_H
#include "stm32f10x.h"//使能uint8_t,uint16_t,uint32_t,uint64_t,int8_t,int16_t,int32_t,int64_t
#define _LOOP_TestTcpClient_DEBUG_ //允许串口跟踪
int32_t loop_TestTcpClient(uint8_t sn, uint8_t* buf, uint8_t* destip, uint16_t destport);
#endif
3、main.c代码如下:
#include "stm32f10x.h"//使能uint8_t,uint16_t,uint32_t,uint64_t,int8_t,int16_t,int32_t,int64_t
#include "stdio.h" //getchar(),putchar(),scanf(),printf(),puts(),gets(),sprintf()
#include "string.h" //使能strcpy(),strlen(),memset()
#include "delay.h"
#include "USART4.h"
#include "LED.h"
//文件下载地址:https://gitee.com/wiznet-hk/STM32F10x_W5500_Examples
//源文件下载地址:https://gitee.com/wiznet-hk
#include "wiz_platform.h"
#include "wizchip_conf.h"
#include "wiz_interface.h"
#include "W5500_Variable.h"
#include "TestTcpClient.h"
const char CPU_Reset_REG[]="\r\nCPU reset!\r\n";
int main(void)
{
// SCB->VTOR = 0x8000000;//中断向量表重定义
// SystemInit();
delay_init();//延时函数初始化
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_4);//设置系统中断优先级分组4
USART4_Serial_Interface_Enable(115200);
printf("%s",CPU_Reset_REG);//调试串口输出"\r\nCPU reset!\r\n"
LED_Init();
LED0_ON();
wiz_timer_init(); //配置TIM2每毫秒中断一次
wiz_spi_init(); //SPI1初始化
wiz_rst_int_init();//初始化W5500的RST引脚和INT引脚
printf("%s network install example\r\n",_WIZCHIP_ID_);
wizchip_initialize();
//1.注册SPI片选函数,单字节读写函数和多字节读写函数
//2.W5500使用RST引脚复位
//3.读取芯片版本号码,并检查是否正确
//4.读PHY配置寄存器的bit[2:0],bit0=1表示W5500连接到局域网
//bit1=1表示当前网速为100M,否则为10M
//bit2=1表示当前以太网采用全双工通讯,否则为半双工通讯
network_init(ethernet_buf, &default_net_info);
//DHCP客户端使用端口0获取本地网络信息
//1.使用"默认网络参数"设置本地网络参数:MAC地址,GW网关,SN子网掩码,本地IP地址,DNS服务器IP地址,DHCP
//2.DHCP获取动态IP地址
//3.使用DHCP获取到的网络参数设置本地网络参数:MAC地址,GW网关,SN子网掩码,本地IP地址,DNS服务器IP地址,DHCP
//4.读本地网络参数:MAC地址,GW网关,SN子网掩码,本地IP地址,DNS服务器IP地址,DHCP,然后从串口输出
setSn_KPALVTR(SOCKET0, 6); // 30s keepalive
//Socket在线时间寄存器,在线验证心跳包传输时间,他只在TCP模式下生效,在其他模式下将会被忽略,单位时间为5秒。
//因此心跳包传输时间为6*5=30秒
while(1)
{
loop_TestTcpClient(SOCKET0, ethernet_buf, dest_ip, dest_port);
LED0=!LED0;
delay_ms(1000);
}
}
4、添加文件如下:
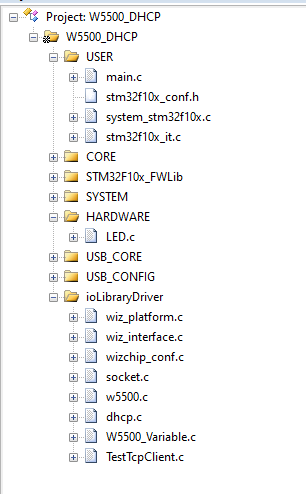
5、 TCP Server配置如下:
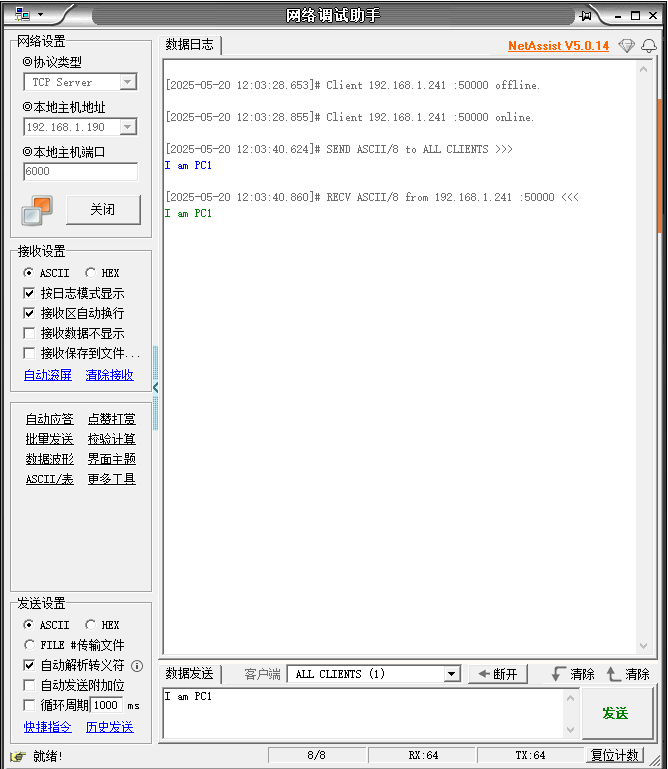
6、串口跟踪如下:
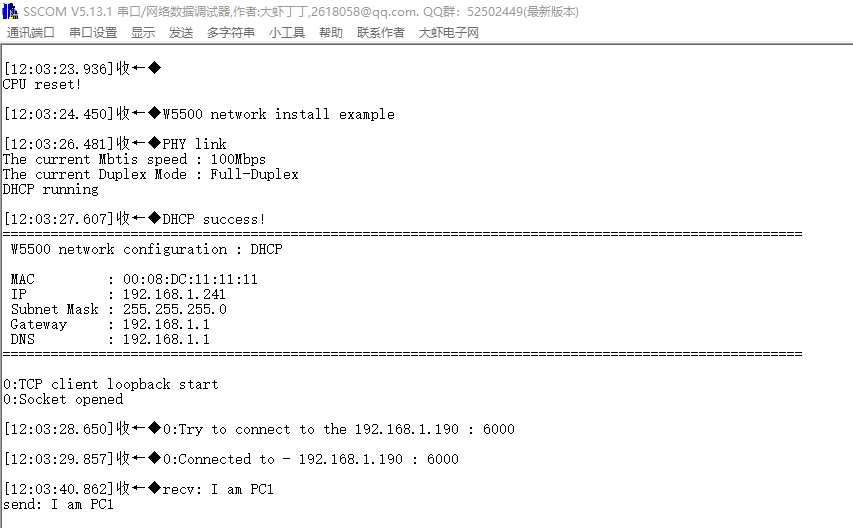