RestClient操作索引库
示例:
一.分析数据结构,写索引库
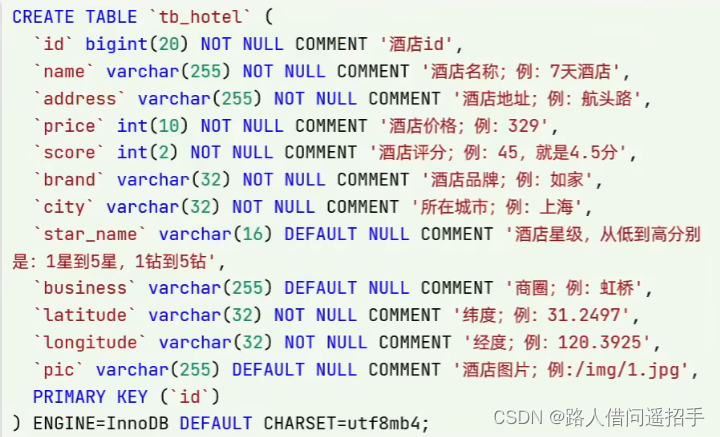
properties
#酒店的mapper
PUT /hotel
{
"mappings": {
"properties": {
"id":{
"type": "keyword"
},
"name":{
"type": "text",
"analyzer": "ik_max_word",
"copy_to": "all"
},
"address":{
"type": "keyword",
#不参与搜索
"index": false
},
"price":{
"type":"integer"
},
"score":{
"type":"integer"
},
"brand":{
"type":"keyword",
"copy_to": "all"
},
"city":{
"type":"keyword"
},
"star_name":{
"type":"keyword"
},
"business":{
"type":"keyword",
"copy_to": "all"
},
"location":{
#地址坐标数据类型
"type":"geo_point"
},
"pic":{
"type":"keyword",
"index": false
},
#字段拷贝
"all":{
"type": "text",
"analyzer": "ik_max_word"
}
}
}
}
小知识
(一)地址坐标数据类型
-
geo_point
:由纬度(latitude
)和经度(longitude
)确定的一个点。例如:"32.8752345,120.2981576' -
geo_shape
"有多个geo_point
组成的复杂几何图形。例如一条直线,"LINESTRING(-77.03653 38.897676,77.009051 38.889939)"
(二)数据拷贝
使用copy_to
属性将当前字段拷贝到指定字段
二.初始化JavaRestClient
(一)引入es的RestHighLevelClient依赖
java
<!--elasticsearch-->
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
</dependency>
(二)因为SpringBoot默认的ES版本是7.6.2,所以我们需要覆盖默认的ES版本:
java
<properties>
<elasticsearch.version>7.17.21</elasticsearch.version>
</properties>
(三)新建一个测试类,初始化JavaRestClient
java
public class HotelIndexTest {
private RestHighLevelClient client;
@Test
void testInit(){
System.out.println(client);
}
/**
* 在每个测试方法执行前设置测试环境。
* 该方法初始化一个指向本地Elasticsearch实例的RestHighLevelClient。
* 这样做是为了确保每个测试都在一个干净的环境中运行,不会相互干扰。
* 使用@BeforeEach注解确保这个方法在每个测试方法执行前被调用。
*/
@BeforeEach
void setUp() {
// 创建一个新的RestHighLevelClient实例,配置为连接到本地Elasticsearch实例
client = new RestHighLevelClient(RestClient.builder(
HttpHost.create("http://192.168.174.129:9200")
));
}
/**
* 在每个测试方法执行后关闭HTTP客户端。
* <p>
* 此方法使用了JUnit的@AfterEach注解,确保在每个测试方法执行完毕后调用。
* 它的主要目的是关闭HttpClient实例,以释放资源并避免潜在的资源泄露。
* 由于HttpClient在多个测试方法中被共享,确保其在每个测试之后正确关闭是很重要的。
* <p>
* 方法抛出的IOException被声明为被抛出,但在这个上下文中被忽略。
* 这是因为关闭客户端可能引发IO异常,但在这个阶段,我们更关心的是确保客户端关闭,
* 而不是处理可能的异常。如果需要处理这些异常,可以在方法中添加相应的异常处理逻辑。
*
* @throws IOException 如果关闭客户端时发生IO异常
*/
@AfterEach
void tearDown() throws IOException {
client.close();
}
}
运行后,出现如下图结果:
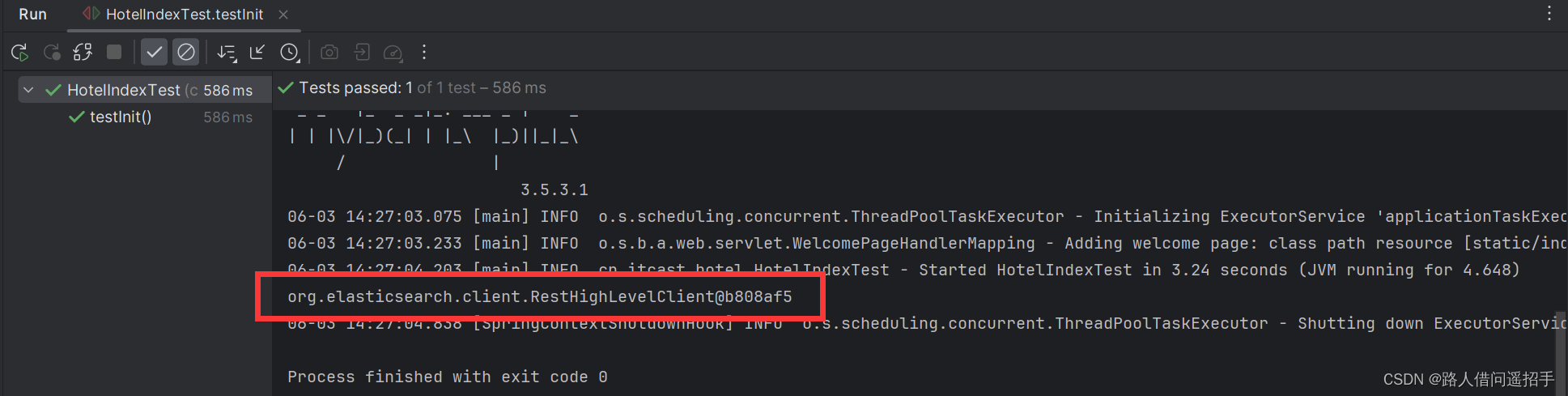
三.使用代码创建索引库
映射模板:MAPPING_TEMPLATE中的内容就是步骤一写的索引库中的内容
java
public class HotelIndexConstants {
public static final String MAPPING_TEMPLATE = "{\n" +
" \"mappings\": {\n" +
" \"properties\": {\n" +
" \"id\": {\n" +
" \"type\": \"keyword\"\n" +
" },\n" +
" \"name\": {\n" +
" \"type\": \"text\",\n" +
" \"analyzer\": \"ik_max_word\",\n" +
" \"copy_to\": \"all\"\n" +
" },\n" +
" \"address\": {\n" +
" \"type\": \"keyword\",\n" +
" \"index\": false\n" +
" },\n" +
" \"price\": {\n" +
" \"type\": \"integer\"\n" +
" },\n" +
" \"score\": {\n" +
" \"type\": \"integer\"\n" +
" },\n" +
" \"brand\": {\n" +
" \"type\": \"keyword\",\n" +
" \"copy_to\": \"all\"\n" +
" },\n" +
" \"city\": {\n" +
" \"type\": \"keyword\"\n" +
" },\n" +
" \"starName\": {\n" +
" \"type\": \"keyword\"\n" +
" },\n" +
" \"business\": {\n" +
" \"type\": \"keyword\",\n" +
" \"copy_to\": \"all\"\n" +
" },\n" +
" \"pic\": {\n" +
" \"type\": \"keyword\",\n" +
" \"index\": false\n" +
" },\n" +
" \"location\": {\n" +
" \"type\": \"geo_point\"\n" +
" },\n" +
" \"all\": {\n" +
" \"type\": \"text\",\n" +
" \"analyzer\": \"ik_max_word\"\n" +
" }\n" +
" }\n" +
" }\n" +
"}";
}
测试类
Java
@Test
void testCreateIndex() throws IOException {
// 1.准备Request PUT /hotel
CreateIndexRequest request = new CreateIndexRequest("hotel");
// 2.准备请求参数:DSL参数
//MAPPING_TEMPLATE静态导入
request.source(MAPPING_TEMPLATE, XContentType.JSON);
// 3.发送请求
client.indices().create(request, RequestOptions.DEFAULT);
}
运行测试类后:
若出现一下报错
properties
[hotel/3L_3wWeHTvSZqCkpc7Rdpw] ElasticsearchStatusException[Elasticsearch exception [type=resource_already_exists_exception, reason=index [hotel/3L_3wWeHTvSZqCkpc7Rdpw] already exists]
]
是因为名为hotel
的索引库已经存在,更改方法如下:
1.删除索引库
properties
#es可视化平台elastic上删除索引库的命令
DELETE /hotel
2.更改测试类中索引库的名称
java
// 1.准备Request PUT /love
CreateIndexRequest request = new CreateIndexRequest("love");
四.创建索引库操作的结果
运行后

在es可视化平台elastic上,查看创建的索引库
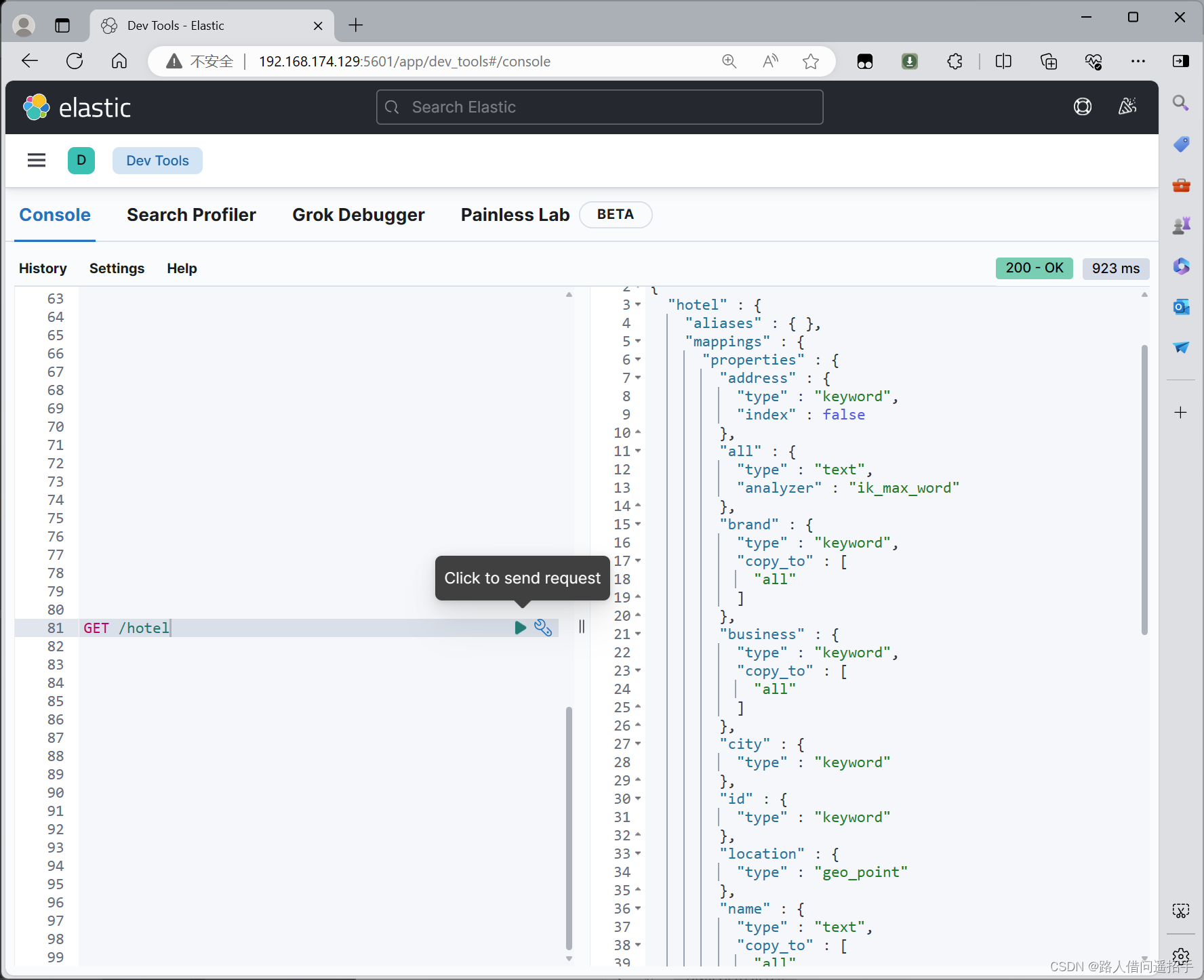
五.删除索引库
java
@Test
void testDeleteIndex() throws IOException {
// 1.准备Request
DeleteIndexRequest request = new DeleteIndexRequest("hotel");
// 3.发送请求
client.indices().delete(request, RequestOptions.DEFAULT);
}
六.判断索引库是否存在
java
@Test
void testExistsIndex() throws IOException {
// 1.准备Request
GetIndexRequest request = new GetIndexRequest("hotel");
// 3.发送请求
boolean isExists = client.indices().exists(request, RequestOptions.DEFAULT);
//输出
System.out.println(isExists ? "存在" : "不存在");
}
先run删除操作,再run判断操作后:
