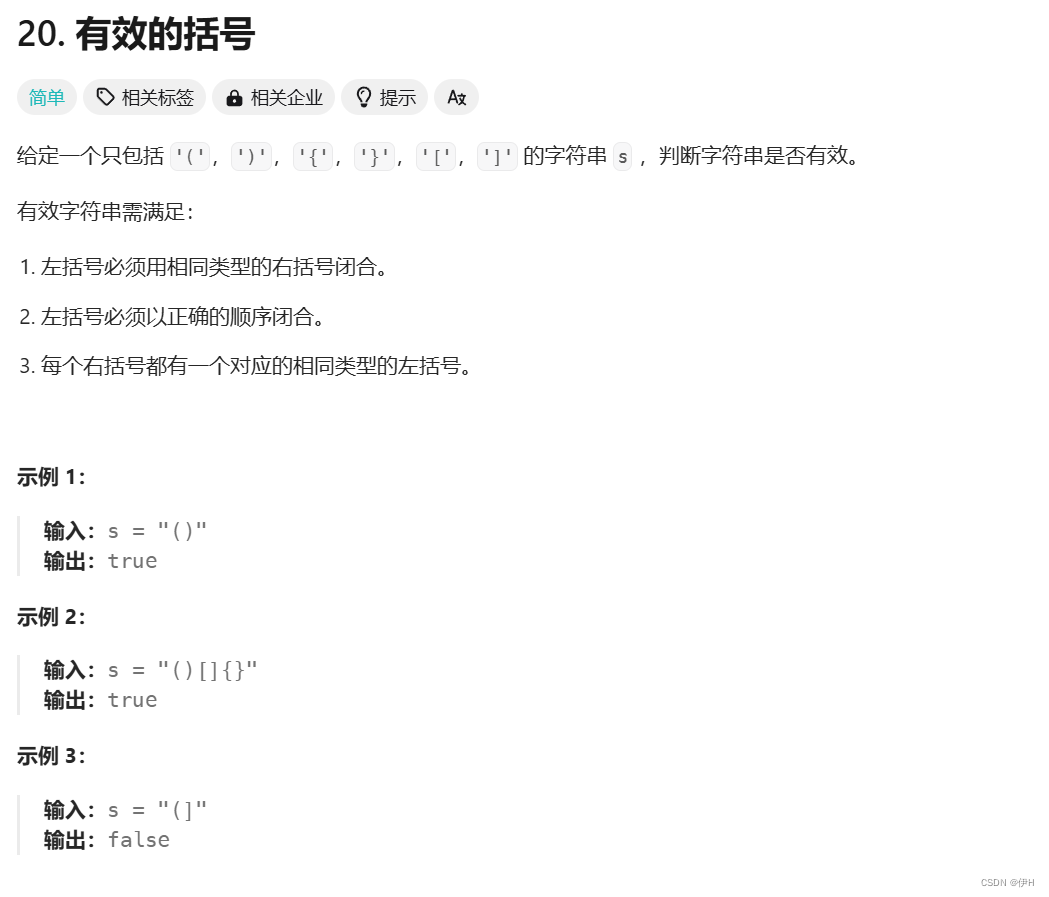
错误记录
cpp
#include<stddef.h>
#include<stdlib.h>
#include<assert.h>
#include<stdbool.h>
typedef char STDataType;
typedef struct Stack
{
STDataType* a;
int capacity;
int top;
} Stack;
void STInit(Stack* st);
void STDestroy(Stack* st);
void STPush(Stack* st, STDataType x);
STDataType STPop(Stack* st);
STDataType STTop(Stack* st);
int STSize(Stack* st);
bool STEmpty(Stack* st);
void STInit(Stack* st)
{
assert(st);
st->a = NULL;
st->capacity = st->top = 0;
}
void STDestroy(Stack* st)
{
assert(st);
free(st->a);
st->a = NULL;
st->capacity = st->top = 0;
}
void STPush(Stack* st, STDataType x)
{
assert(st);
//checkcapacity
if (st->capacity == st->top)
{
int newcapacity = st->capacity == 0 ? 4 : st->capacity * 2;
STDataType* temp = (STDataType*)realloc(st->a, sizeof(STDataType) * newcapacity);
if (NULL == temp)
{
perror("realloc failed");
exit(-1);
}
st->a = temp;
st->capacity = newcapacity;
}
st->a[st->top] = x;
++st->top;
}
STDataType STPop(Stack* st)
{
assert(st);
assert(st->top);
STDataType ret = STTop(st);
--st->top;
return ret;
}
STDataType STTop(Stack* st)
{
assert(st);
assert(st->top);
return st->a[st->top - 1];
}
int STSize(Stack* st)
{
return st->top;
}
bool STEmpty(Stack* st)
{
return st->top == 0;
}
bool isValid(char* s)
{
Stack st;
STInit(&st);
int i = 0;
while(*s)
{
if(*s == '(' || *s == '[' || *s == '{')
STPush(&st, *s);
else
{
if((STPop(&st) == '(' && *s != ')') ||
(STPop(&st) == '[' && *s != ']') ||
(STPop(&st) == '{' && *s != '}'))
{
STDestroy(&st);
return false;
}
}
++s;
}
STDestroy(&st);
return true;
}
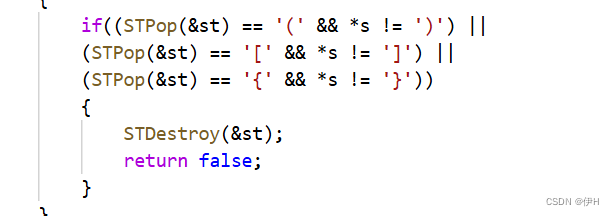
这里比较三次直接出了3次栈,应该只出一次比较三次。
修改成下面这样:
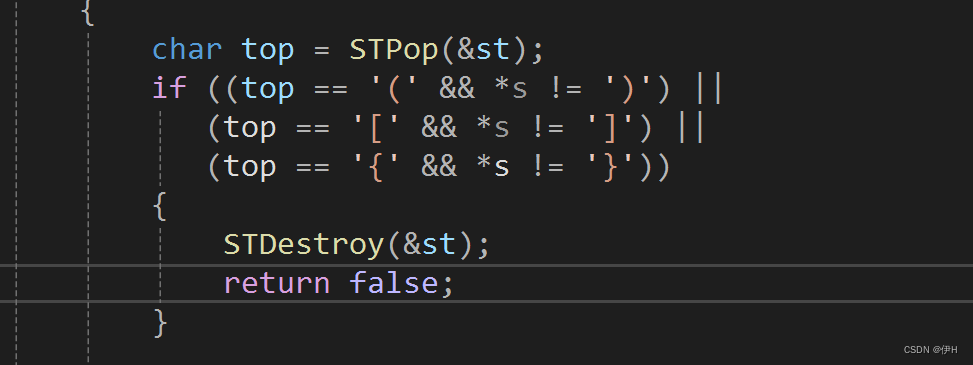
之后在" ( "和" ) "又分别翻车了,加了下面两句判断。
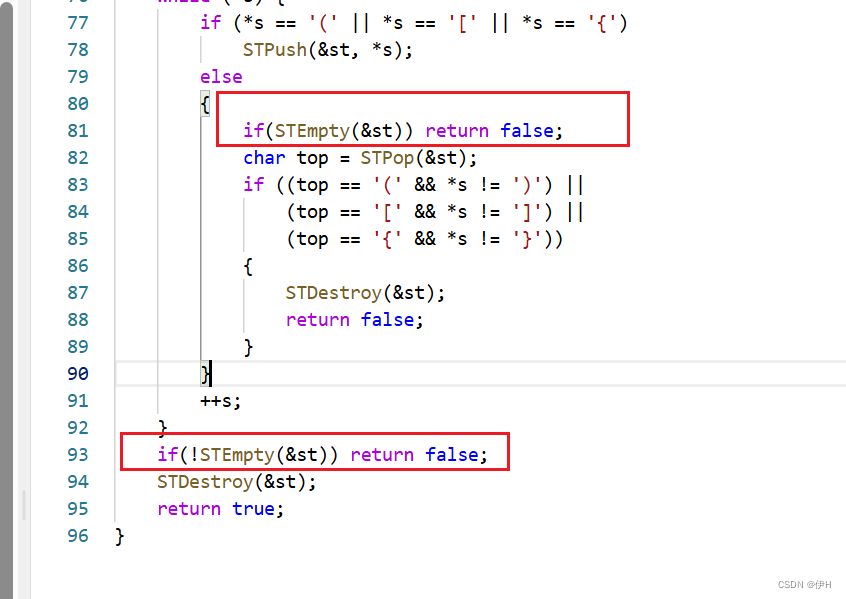
最终提交
cpp
#include <assert.h>
#include <stdbool.h>
#include <stddef.h>
#include <stdlib.h>
typedef char STDataType;
typedef struct Stack {
STDataType* a;
int capacity;
int top;
} Stack;
void STInit(Stack* st);
void STDestroy(Stack* st);
void STPush(Stack* st, STDataType x);
STDataType STPop(Stack* st);
STDataType STTop(Stack* st);
int STSize(Stack* st);
bool STEmpty(Stack* st);
void STInit(Stack* st) {
assert(st);
st->a = NULL;
st->capacity = st->top = 0;
}
void STDestroy(Stack* st) {
assert(st);
free(st->a);
st->a = NULL;
st->capacity = st->top = 0;
}
void STPush(Stack* st, STDataType x) {
assert(st);
// checkcapacity
if (st->capacity == st->top) {
int newcapacity = st->capacity == 0 ? 4 : st->capacity * 2;
STDataType* temp =
(STDataType*)realloc(st->a, sizeof(STDataType) * newcapacity);
if (NULL == temp) {
perror("realloc failed");
exit(-1);
}
st->a = temp;
st->capacity = newcapacity;
}
st->a[st->top] = x;
++st->top;
}
STDataType STPop(Stack* st) {
assert(st);
assert(st->top);
STDataType ret = STTop(st);
--st->top;
return ret;
}
STDataType STTop(Stack* st) {
assert(st);
assert(st->top);
return st->a[st->top - 1];
}
int STSize(Stack* st) { return st->top; }
bool STEmpty(Stack* st) { return st->top == 0; }
bool isValid(char* s) {
Stack st;
STInit(&st);
int i = 0;
while (*s) {
if (*s == '(' || *s == '[' || *s == '{')
STPush(&st, *s);
else
{
if(STEmpty(&st))
{
STDestroy(&st);
return false;
}
char topval = STPop(&st);
if ((topval == '(' && *s != ')') ||
(topval == '[' && *s != ']') ||
(topval == '{' && *s != '}'))
{
STDestroy(&st);
return false;
}
}
++s;
}
if(!STEmpty(&st)) return false;
STDestroy(&st);
return true;
}
修改日志
2024/6/12
一处return的时候忘记调用STDestroy,有内存泄漏风险,补上了。
把变量top名称改成了topval,避免与Stack的成员top混杂,增强代码可读性。