适配器模式(Adapter)
适配器模式是一种结构型设计模式, 它能使接口不兼容的对象能够相互合作。
当系统的数据和行为都正确,但是接口不符合的时候,应该考虑使用适配器,目的是使控制范围之外的一个原有对象与某个接口匹配。适配器模式主要应用于希望复用一些现存的类,但是接口又与复用环境要求不一致的情况。
适配器模式包含两种类型:类适配器模式和对象适配器
类适配器模式通过多重继承对一个接口与另一个接口进行匹配。注意C#、Java等语言不支持多重继承。
对象适配器 适配器实现了其中一个对象的接口, 并对另一个对象进行封装。 所有流行的编程语言都可以实现适配器。
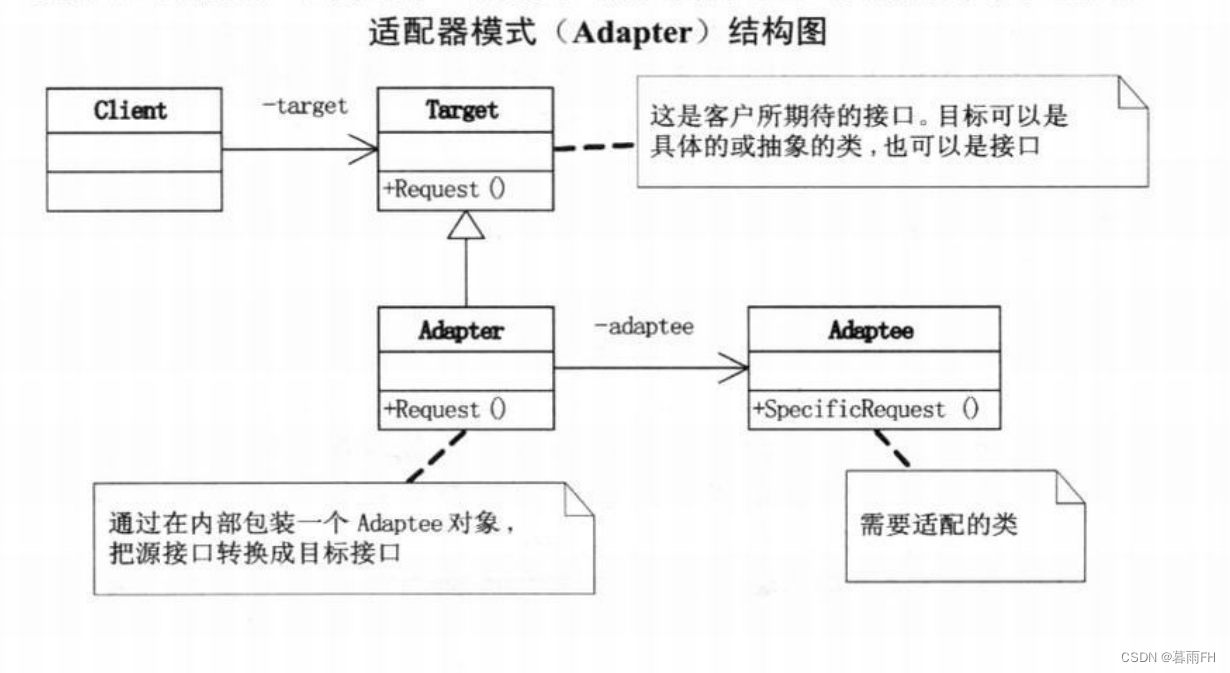
类适配器 适配器同时继承两个对象的接口。 请注意, 这种方式仅能在支持多重继承的编程语言中实现, 例如 C++。
何时使用适配器模式?
当双方都不太容易修改的时候,就要考虑使用适配器模式进行适配。但是在设计的时候应该尽可能保证接口相同,治未病
c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
// 目标接口
class Target
{
public:
virtual ~Target() = default;
virtual string Request() const
{
return "Target: The default target's behavior.";
}
};
// 需要适配的类
class Adaptee
{
public:
string SpecificRequest() const
{
return ".eetpadA eht fo roivaheb laicepS";
}
};
// 适配器
class Adapter : public Target
{
private:
Adaptee *adaptee_;
public:
Adapter(Adaptee *adaptee) : adaptee_(adaptee) {}
string Request() const override
{
string to_reverse = this->adaptee_->SpecificRequest();
reverse(to_reverse.begin(), to_reverse.end());
return "Adapter: (TRANSLATED) " + to_reverse;
}
};
// 客户端代码,使用的是target接口
void ClientCode(const Target *target)
{
cout << target->Request() << endl;
}
int main()
{
cout << "I can work just fine with the Target object:" << endl;
Target *target = new Target;
ClientCode(target);
cout << "\n\n";
Adaptee *adaptee = new Adaptee;
cout << "Client: The Adaptee class has a weird interface. See, I don't understand it : \n";
cout << "Adaptee: " << adaptee->SpecificRequest();
cout << "\n\n";
cout << "Client: But I can work with it via the Adapter:\n";
Adapter *adapter = new Adapter(adaptee);
ClientCode(adapter);
cout << "\n";
delete target;
delete adaptee;
delete adapter;
return 0;
}
输出为
I can work just fine with the Target object:
Target: The default target's behavior.
Client: The Adaptee class has a weird interface. See, I don't understand it :
Adaptee: .eetpadA eht fo roivaheb laicepS
Client: But I can work with it via the Adapter:
Adapter: (TRANSLATED) Special behavior of the Adaptee.