目录
- 题目
- [1- 思路](#1- 思路)
- [2- 实现](#2- 实现)
-
- [⭐415. 字符串相加------题解思路](#⭐415. 字符串相加——题解思路)
- [3- ACM 实现](#3- ACM 实现)
题目
- 原题连接:415. 字符串相加
1- 思路
- 模式识别:字符串相加
逆向遍历过程模拟
- 数据结构 ① String res :记录res 、② carry 记录进位值
- ① 定义两个整数遍历
nums1
和nums2
------>index1 = nums1.length
,index2 - nums2.length
- ② 利用 while循环遍历
while( index1>=0 || index2>=0 || carry != 0)
- ③ 定义
x = index1>=0 ? nums1.charAt(i) - '0' : 0;
- 同理
y = index2>=0 ? nums1.charAt(i) - '0' : 0;
- 计算
int res = x+y+carry;
之后通过 StringBuffer ans = new StringBuffer(); sb.append(res%10) carry = res/10
,之后index1--;
index2--;
- ④ 最终答案 revers
2- 实现
⭐415. 字符串相加------题解思路
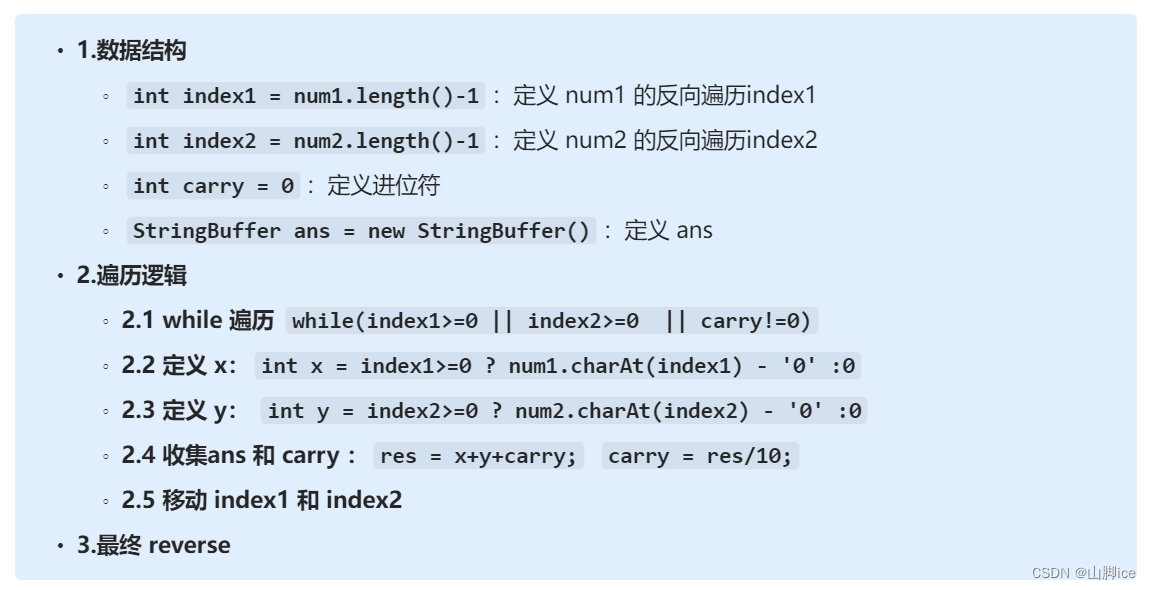
java
class Solution {
public String addStrings(String num1, String num2) {
int index1 = num1.length()-1;
int index2 = num2.length()-1;
int carry = 0;
StringBuffer ans = new StringBuffer();
// 逆向遍历
while(index1>=0 || index2>=0 || carry!=0){
int x = index1>=0 ? num1.charAt(index1) - '0' :0;
int y = index2>=0 ? num2.charAt(index2) - '0' :0;
int res = x+y+carry;
ans.append(res%10);
carry = res/10;
index1--;
index2--;
}
ans.reverse();
return ans.toString();
}
}
3- ACM 实现
java
public class addString {
public static String addTwo(String num1,String num2){
int index1 = num1.length()-1;
int index2 = num2.length()-1;
int carry = 0;
StringBuffer ans = new StringBuffer();
// 遍历
while( index1 >=0 || index2>= 0 || carry!=0){
int x = index1>=0 ? num1.charAt(index1)-'0' : 0;
int y = index2>=0 ? num2.charAt(index2)-'0' : 0;
int res = x+y+carry;
ans.append(res%10);
carry = res/10;
index1--;
index2--;
}
ans.reverse();
return ans.toString();
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("输入str1");
String input1 = sc.next();
System.out.println("输入str2");
String input2 = sc.next();
System.out.println("相加的结果是"+addTwo(input1,input2));
}
}