0 - Package version
python
Package Version
----------------------------- --------------
imageio 2.33.1
keras 2.6.0
Keras-Preprocessing 1.1.2
matplotlib 3.7.2
numpy 1.21.6
tensorflow 2.6.0
1 - Import packages
python
import os
import imageio
import matplotlib.pyplot as plt
import numpy as np
import tensorflow as tf
from keras import backend as K
from keras.models import load_model
from yad2k.models.keras_yolo import yolo_boxes_to_corners, yolo_head
from yolo_utils import (
draw_boxes,
generate_colors,
preprocess_image,
read_anchors,
read_classes,
scale_boxes,
)
2 - Filtering with a threshold on class scores
python
# GRADED FUNCTION: yolo_filter_boxes
def yolo_filter_boxes(box_confidence, boxes, box_class_probs, threshold=0.6):
"""Filters YOLO boxes by thresholding on object and class confidence.
Arguments:
box_confidence -- tensor of shape (19, 19, 5, 1)
boxes -- tensor of shape (19, 19, 5, 4)
box_class_probs -- tensor of shape (19, 19, 5, 80)
threshold -- real value, if [ highest class probability score < threshold], then get rid of the corresponding box
Returns:
scores -- tensor of shape (None,), containing the class probability score for selected boxes
boxes -- tensor of shape (None, 4), containing (b_x, b_y, b_h, b_w) coordinates of selected boxes
classes -- tensor of shape (None,), containing the index of the class detected by the selected boxes
Note: "None" is here because you don't know the exact number of selected boxes, as it depends on the threshold.
For example, the actual output size of scores would be (10,) if there are 10 boxes.
"""
# Step 1: Compute box scores
### START CODE HERE ### (≈ 1 line)
box_scores = box_confidence * box_class_probs
### END CODE HERE ###
# Step 2: Find the box_classes thanks to the max box_scores, keep track of the corresponding score
### START CODE HERE ### (≈ 2 lines)
box_classes = K.argmax(box_scores, axis=-1)
box_class_scores = K.max(box_scores, axis=-1, keepdims=False)
### END CODE HERE ###
# Step 3: Create a filtering mask based on "box_class_scores" by using "threshold". The mask should have the
# same dimension as box_class_scores, and be True for the boxes you want to keep (with probability >= threshold)
### START CODE HERE ### (≈ 1 line)
filtering_mask = box_class_scores > threshold
### END CODE HERE ###
# Step 4: Apply the mask to scores, boxes and classes
### START CODE HERE ### (≈ 3 lines)
scores = tf.boolean_mask(box_class_scores, filtering_mask)
boxes = tf.boolean_mask(boxes, filtering_mask)
classes = tf.boolean_mask(box_classes, filtering_mask)
### END CODE HERE ###
return scores, boxes, classes
python
box_confidence = tf.random.normal([19, 19, 5, 1], mean=1, stddev=4, seed=1)
boxes = tf.random.normal([19, 19, 5, 4], mean=1, stddev=4, seed=1)
box_class_probs = tf.random.normal([19, 19, 5, 80], mean=1, stddev=4, seed=1)
scores, boxes, classes = yolo_filter_boxes(
box_confidence, boxes, box_class_probs, threshold=0.5
)
print("scores[2] = " + str(scores[2].numpy()))
print("boxes[2] = " + str(boxes[2].numpy()))
print("classes[2] = " + str(classes[2].numpy()))
print("scores.shape = " + str(scores.shape))
print("boxes.shape = " + str(boxes.shape))
print("classes.shape = " + str(classes.shape))
'''
Output 输出
scores[2] = 16.064978
boxes[2] = [ 1.0273384 -2.1178942 4.8872733 -4.0143332]
classes[2] = 78
scores.shape = (1786,)
boxes.shape = (1786, 4)
classes.shape = (1786,)
'''
3 - Non-max suppression
python
# GRADED FUNCTION: iou
def iou(box1, box2):
"""Implement the intersection over union (IoU) between box1 and box2
Arguments:
box1 -- first box, list object with coordinates (x1, y1, x2, y2)
box2 -- second box, list object with coordinates (x1, y1, x2, y2)
"""
# Calculate the (y1, x1, y2, x2) coordinates of the intersection of box1 and box2. Calculate its Area.
### START CODE HERE ### (≈ 5 lines)
xi1 = max(box1[0], box2[0])
yi1 = max(box1[1], box2[1])
xi2 = min(box1[2], box2[2])
yi2 = min(box1[3], box2[3])
inter_area = (xi2 - xi1) * (yi2 - yi1)
### END CODE HERE ###
# Calculate the Union area by using Formula: Union(A,B) = A + B - Inter(A,B)
### START CODE HERE ### (≈ 3 lines)
box1_area = (box1[2] - box1[0]) * (box1[3] - box1[1])
box2_area = (box2[2] - box2[0]) * (box2[3] - box2[1])
union_area = box1_area + box2_area - inter_area
### END CODE HERE ###
# compute the IoU
### START CODE HERE ### (≈ 1 line)
iou = inter_area / union_area
### END CODE HERE ###
return iou
python
box1 = (2, 1, 4, 3)
box2 = (1, 2, 3, 4)
print("iou = " + str(iou(box1, box2)))
'''
Output 输出
iou = 0.14285714285714285
'''
python
# GRADED FUNCTION: yolo_non_max_suppression
def yolo_non_max_suppression(scores, boxes, classes, max_boxes=10, iou_threshold=0.5):
"""
Applies Non-max suppression (NMS) to set of boxes
Arguments:
scores -- tensor of shape (None,), output of yolo_filter_boxes()
boxes -- tensor of shape (None, 4), output of yolo_filter_boxes() that have been scaled to the image size (see later)
classes -- tensor of shape (None,), output of yolo_filter_boxes()
max_boxes -- integer, maximum number of predicted boxes you'd like
iou_threshold -- real value, "intersection over union" threshold used for NMS filtering
Returns:
scores -- tensor of shape (, None), predicted score for each box
boxes -- tensor of shape (4, None), predicted box coordinates
classes -- tensor of shape (, None), predicted class for each box
Note: The "None" dimension of the output tensors has obviously to be less than max_boxes. Note also that this
function will transpose the shapes of scores, boxes, classes. This is made for convenience.
"""
max_boxes_tensor = tf.Variable(
max_boxes, dtype="int32", name="max_boxes_tensor"
) # initialize variable max_boxes_tensor
# Use tf.image.non_max_suppression() to get the list of indices corresponding to boxes you keep
### START CODE HERE ### (≈ 1 line)
nms_indices = tf.image.non_max_suppression(
boxes, scores, max_boxes, iou_threshold, name=None
)
### END CODE HERE ###
# Use K.gather() to select only nms_indices from scores, boxes and classes
### START CODE HERE ### (≈ 3 lines)
scores = K.gather(scores, nms_indices)
boxes = K.gather(boxes, nms_indices)
classes = K.gather(classes, nms_indices)
### END CODE HERE ###
return scores, boxes, classes
python
scores = tf.random.normal(
[
54,
],
mean=1,
stddev=4,
seed=1,
)
boxes = tf.random.normal([54, 4], mean=1, stddev=4, seed=1)
classes = tf.random.normal(
[
54,
],
mean=1,
stddev=4,
seed=1,
)
scores, boxes, classes = yolo_non_max_suppression(scores, boxes, classes)
print("scores[2] = " + str(scores[2].numpy()))
print("boxes[2] = " + str(boxes[2].numpy()))
print("classes[2] = " + str(classes[2].numpy()))
print("scores.shape = " + str(scores.shape))
print("boxes.shape = " + str(boxes.shape))
print("classes.shape = " + str(classes.shape))
'''
Output 输出
scores[2] = 7.830904
boxes[2] = [ 2.2589586 0.29471445 -2.2524776 0.87786186]
classes[2] = -0.8636918
scores.shape = (10,)
boxes.shape = (10, 4)
classes.shape = (10,)
'''
4 - Wrapping up the filtering
python
# GRADED FUNCTION: yolo_eval
def yolo_eval(
yolo_outputs,
image_shape=(720.0, 1280.0),
max_boxes=10,
score_threshold=0.6,
iou_threshold=0.5,
):
"""
Converts the output of YOLO encoding (a lot of boxes) to your predicted boxes along with their scores, box coordinates and classes.
Arguments:
yolo_outputs -- output of the encoding model (for image_shape of (608, 608, 3)), contains 4 tensors:
box_confidence: tensor of shape (None, 19, 19, 5, 1)
box_xy: tensor of shape (None, 19, 19, 5, 2)
box_wh: tensor of shape (None, 19, 19, 5, 2)
box_class_probs: tensor of shape (None, 19, 19, 5, 80)
image_shape -- tensor of shape (2,) containing the input shape, in this notebook we use (608., 608.) (has to be float32 dtype)
max_boxes -- integer, maximum number of predicted boxes you'd like
score_threshold -- real value, if [ highest class probability score < threshold], then get rid of the corresponding box
iou_threshold -- real value, "intersection over union" threshold used for NMS filtering
Returns:
scores -- tensor of shape (None, ), predicted score for each box
boxes -- tensor of shape (None, 4), predicted box coordinates
classes -- tensor of shape (None,), predicted class for each box
"""
### START CODE HERE ###
# Retrieve outputs of the YOLO model (≈1 line)
box_xy, box_wh, box_confidence, box_class_probs = yolo_outputs
# Convert boxes to be ready for filtering functions
boxes = yolo_boxes_to_corners(box_xy, box_wh)
# Use one of the functions you've implemented to perform Score-filtering with a threshold of score_threshold (≈1 line)
scores, boxes, classes = yolo_filter_boxes(
box_confidence, boxes, box_class_probs, score_threshold
)
# Scale boxes back to original image shape.
boxes = scale_boxes(boxes, image_shape)
# Use one of the functions you've implemented to perform Non-max suppression with a threshold of iou_threshold (≈1 line)
scores, boxes, classes = yolo_non_max_suppression(
scores, boxes, classes, max_boxes, iou_threshold
)
### END CODE HERE ###
return scores, boxes, classes
python
yolo_outputs = (
tf.random.normal([19, 19, 5, 2], mean=1, stddev=4, seed=1),
tf.random.normal([19, 19, 5, 2], mean=1, stddev=4, seed=1),
tf.random.normal([19, 19, 5, 1], mean=1, stddev=4, seed=1),
tf.random.normal([19, 19, 5, 80], mean=1, stddev=4, seed=1),
)
scores, boxes, classes = yolo_eval(yolo_outputs)
print("scores[2] = " + str(scores[2].numpy()))
print("boxes[2] = " + str(boxes[2].numpy()))
print("classes[2] = " + str(classes[2].numpy()))
print("scores.shape = " + str(scores.shape))
print("boxes.shape = " + str(boxes.shape))
print("classes.shape = " + str(classes.shape))
'''
Output 输出
scores[2] = 131.62581
boxes[2] = [5489.0874 7872.257 4824.162 8717.479 ]
classes[2] = 29
scores.shape = (10,)
boxes.shape = (10, 4)
classes.shape = (10,)
'''
5 - Defining classes, anchors and image shape.
python
class_names = read_classes("model_data/coco_classes.txt")
anchors = read_anchors("model_data/yolo_anchors.txt")
image_shape = (720.0, 1280.0)
6 - Loading a pretrained model
python
yolo_model = load_model("model_data/yolo.h5")
bash
yolo_model.summary()
下载的文件里没有h5模型的,需要重新生产模型,步骤:
6.1 - 下载预训练的权重:
Linux
bash
wget http://pjreddie.com/media/files/yolo.weights
Window
http://pjreddie.com/media/files/yolo.weights
点击上面链接之后自动下载
6.2 - 下载配置文件:
https://github.com/pjreddie/darknet/tree/master/cfg
找到yolov2.cfg下载下来,并改名成yolo.cfg(调用该文件时,使用的名字是这个)。
6.3 - 下载需要脚本
https://github.com/allanzelener/YAD2K
点击下载zip。
当然你使用git命令下载下来也可以。
6.4 - 准备工作
复制或剪切yolo.weights和yolo.cfg以及yad2k.py三个文件,以及一个文件夹yad2k到同一文件夹中。
注意文件夹吴恩达提供的作业里已经有yad2k文件夹,所有windows会提示你是否替换原有文件,点击替换。
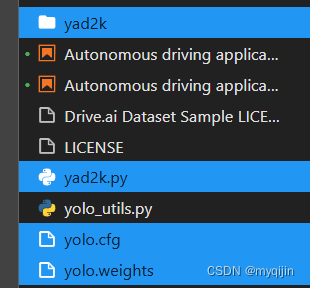
6.5 - 打开Anaconda Prompt
在prompt中运行command
bash
(tensorflow) C:\Users\>python yad2k.py yolo.cfg yolo.weights model_data/yolo.h5
7 - Run the graph on an image
bash
def predict(image_file):
"""
Runs the graph stored in "sess" to predict boxes for "image_file". Prints and plots the preditions.
Arguments:
sess -- your tensorflow/Keras session containing the YOLO graph
image_file -- name of an image stored in the "images" folder.
Returns:
out_scores -- tensor of shape (None, ), scores of the predicted boxes
out_boxes -- tensor of shape (None, 4), coordinates of the predicted boxes
out_classes -- tensor of shape (None, ), class index of the predicted boxes
Note: "None" actually represents the number of predicted boxes, it varies between 0 and max_boxes.
"""
# Preprocess your image
image, image_data = preprocess_image(
"images/" + image_file, model_image_size=(608, 608)
)
# Run the session with the correct tensors and choose the correct placeholders in the feed_dict.
# You'll need to use feed_dict={yolo_model.input: ... , K.learning_phase(): 0})
### START CODE HERE ### (≈ 1 line)
out_scores = yolo_model(image_data, training=False)
yolo_outputs = yolo_head(out_scores, anchors, len(class_names))
out_scores, out_boxes, out_classes = yolo_eval(yolo_outputs, image_shape)
### END CODE HERE ###
# Print predictions info
print("Found {} boxes for {}".format(len(out_boxes), image_file))
# Generate colors for drawing bounding boxes.
colors = generate_colors(class_names)
# Draw bounding boxes on the image file
draw_boxes(image, out_scores, out_boxes, out_classes, class_names, colors)
# Save the predicted bounding box on the image
image.save(os.path.join("out", image_file), quality=90)
# Display the results in the notebook
output_image = imageio.imread(os.path.join("out", "test.jpg"))
plt.imshow(output_image)
return out_scores, out_boxes, out_classes
python
predict("test.jpg")
predict function中使用到了yolo_head,在yad2k/models/keras_yolo.py代码中 line 32 需要修改:
python
#return tf.space_to_depth(x, block_size=2) #TF1
return tf.nn.space_to_depth(x, block_size=2) #TF2
predict function中使用到了yolo_units.draw_boxes(),yolo_units.py代码中由于Pillow版本的问题,ImageDraw.textsize() 已弃用,对应需要使用ImageDraw.textbbox()
python
def draw_boxes(image, out_scores, out_boxes, out_classes, class_names, colors):
font = ImageFont.truetype(font='font/FiraMono-Medium.otf',size=np.floor(3e-2 * image.size[1] + 0.5).astype('int32'))
thickness = (image.size[0] + image.size[1]) // 300
for i, c in reversed(list(enumerate(out_classes))):
predicted_class = class_names[c]
box = out_boxes[i]
score = out_scores[i]
label = '{} {:.2f}'.format(predicted_class, score)
draw = ImageDraw.Draw(image)
#label_size = draw.textlength(label, font) #Deprecated
box_size = draw.textbbox((0,0), label, font)
label_size = [box_size[2] - box_size[0], box_size[3] - box_size[1]]
top, left, bottom, right = box
top = max(0, np.floor(top + 0.5).astype('int32'))
left = max(0, np.floor(left + 0.5).astype('int32'))
bottom = min(image.size[1], np.floor(bottom + 0.5).astype('int32'))
right = min(image.size[0], np.floor(right + 0.5).astype('int32'))
print(label, (left, top), (right, bottom))
if top - label_size[1] >= 0:
text_origin = np.array([left, top - label_size[1]])
else:
text_origin = np.array([left, top + 1])
# My kingdom for a good redistributable image drawing library.
for i in range(thickness):
draw.rectangle([left + i, top + i, right - i, bottom - i], outline=colors[c])
draw.rectangle([tuple(text_origin), tuple(text_origin + label_size)], fill=colors[c])
draw.text(text_origin, label, fill=(0, 0, 0), font=font)
del draw