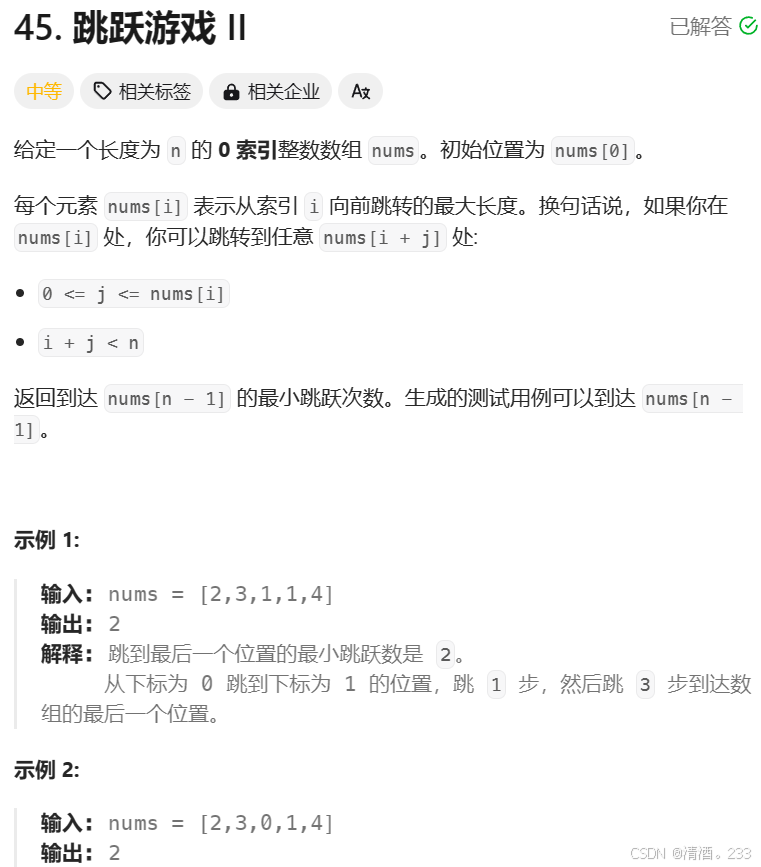
cpp
class Solution {
public:
int jump(vector<int>& nums)
{
if (nums.size() == 1) return 0; // 如果数组长度为1,已经在终点,不需要跳跃
int cur = 0; // 当前跳跃能到达的最远位置
int flag = 0; // 记录跳跃次数
int next = 0; // 下一次跳跃能到达的最远位置
for (int i = 0; i < nums.size(); i++)
{
next = max(i + nums[i], next); // 更新下一次跳跃能到达的最远位置
if (i == cur) // 当遍历到当前跳跃的最远位置时
{
flag++; // 增加跳跃次数
cur = next; // 更新当前跳跃的最远位置
if (next >= nums.size() - 1) break; // 如果下一次跳跃能到达终点,结束循环
}
}
return flag; // 返回跳跃次数
}
};
核心思想
该算法使用贪心策略,每次选择当前能跳跃的最远位置,并更新下一次能跳跃的最远位置。遍历过程中,每当到达当前能跳跃的最远位置时,增加跳跃次数,并更新当前跳跃能到达的最远位置。如果在更新过程中,发现能到达或超过终点,跳出循环。
示例
考虑 nums = [2, 3, 1, 1, 4]
:
- 初始
cur = 0
,flag = 0
,next = 0
。 - 第一次循环:
i = 0
:next = max(0 + 2, 0) = 2
i == cur
,跳跃次数增加:flag = 1
,更新cur = next = 2
- 第二次循环:
i = 1
:next = max(1 + 3, 2) = 4
i == cur
,跳跃次数增加:flag = 2
,更新cur = next = 4
- 终点可达,跳出循环
最终返回 flag = 2
,表示最少需要跳跃两次。