Django起源:
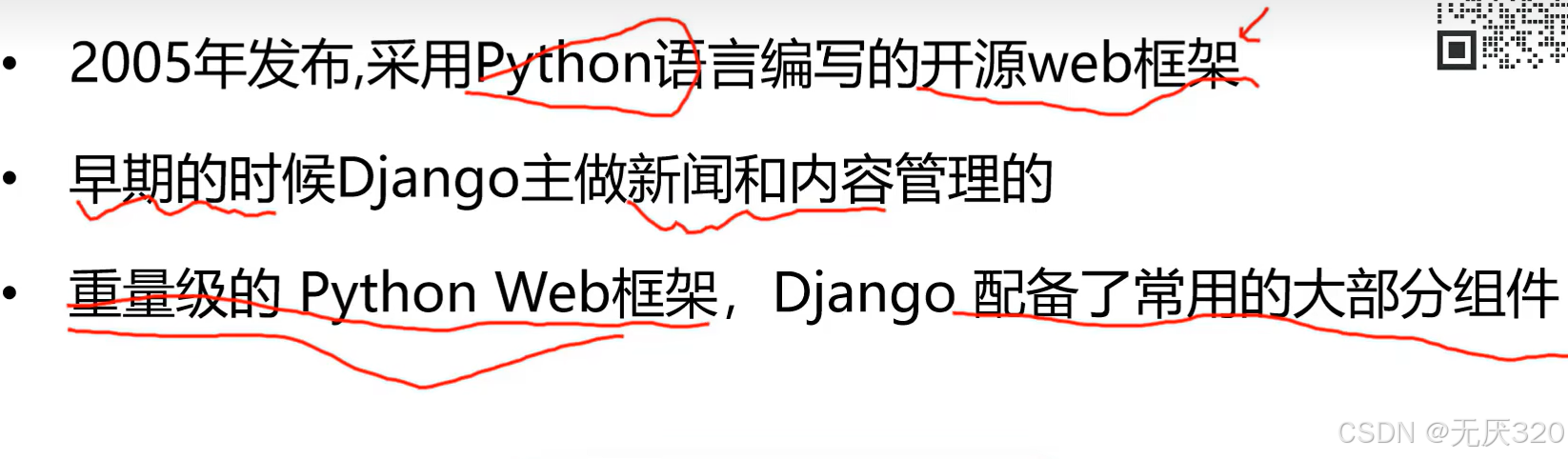
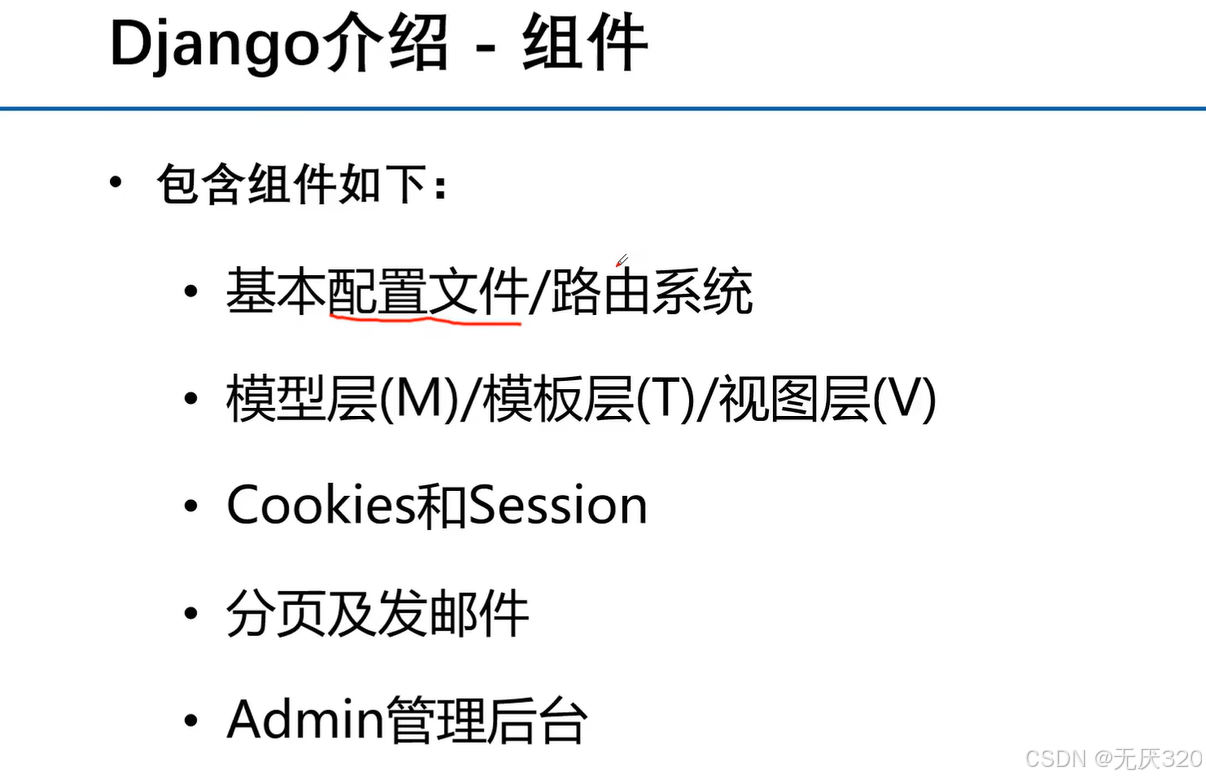
项目创建:
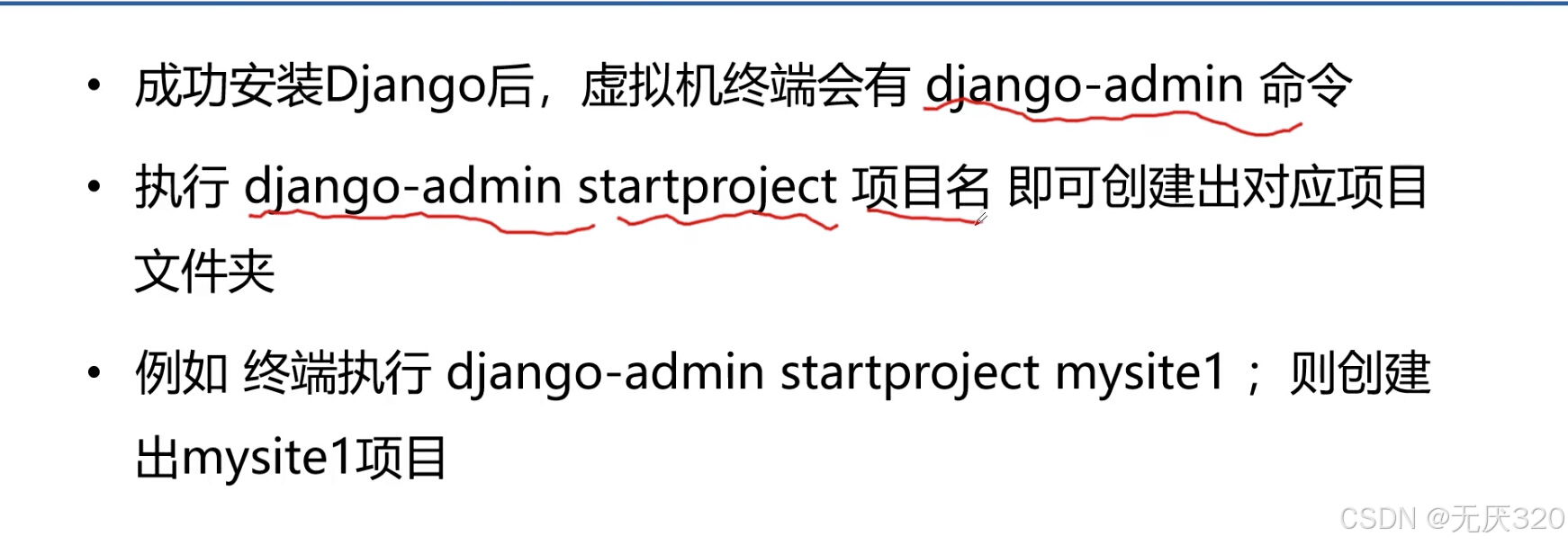
项目执行(测试开发阶段):
项目结束:
结构: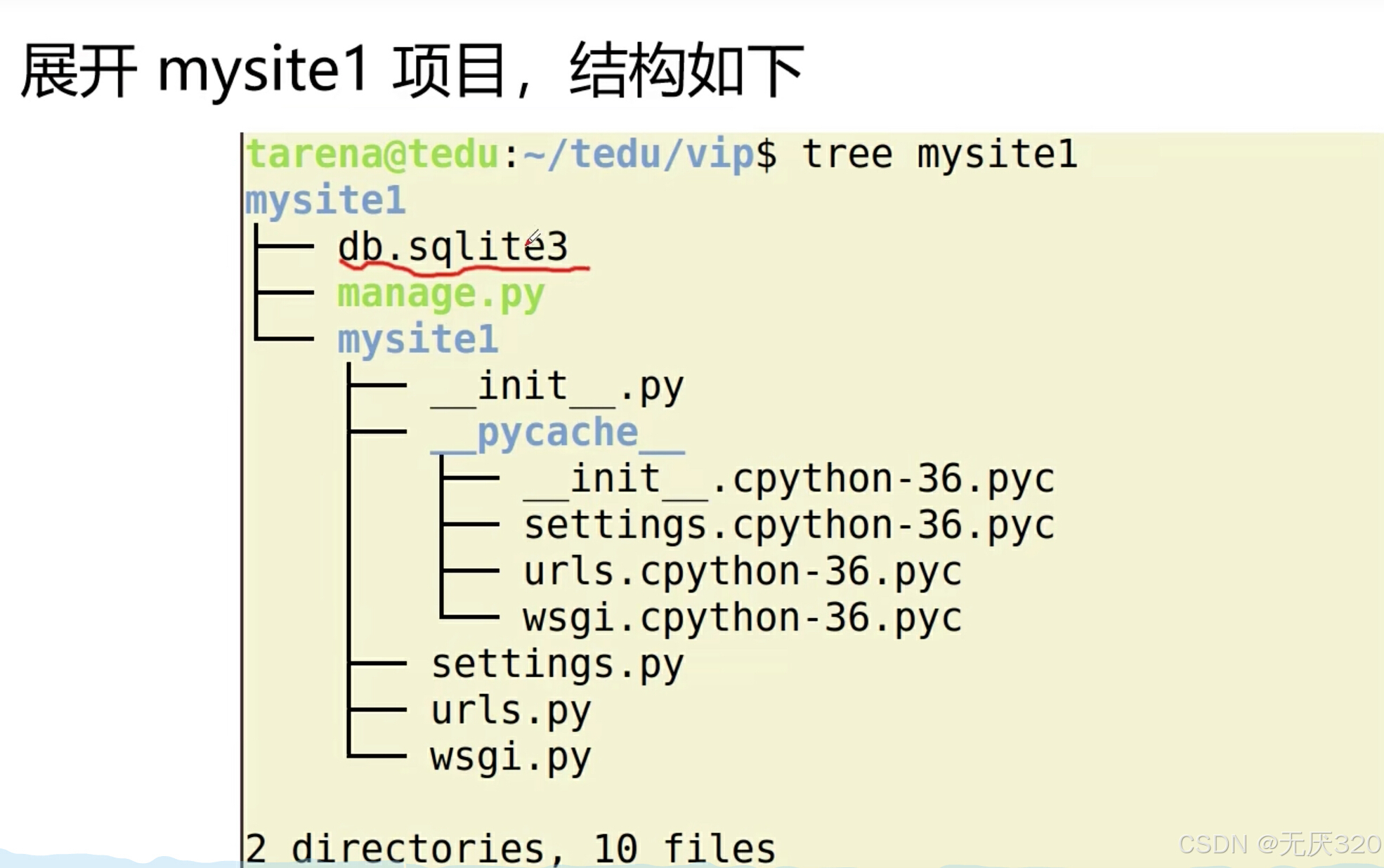
db.sqlite3:数据库,一般不用,会替换成我们自己的数据库
manage.py
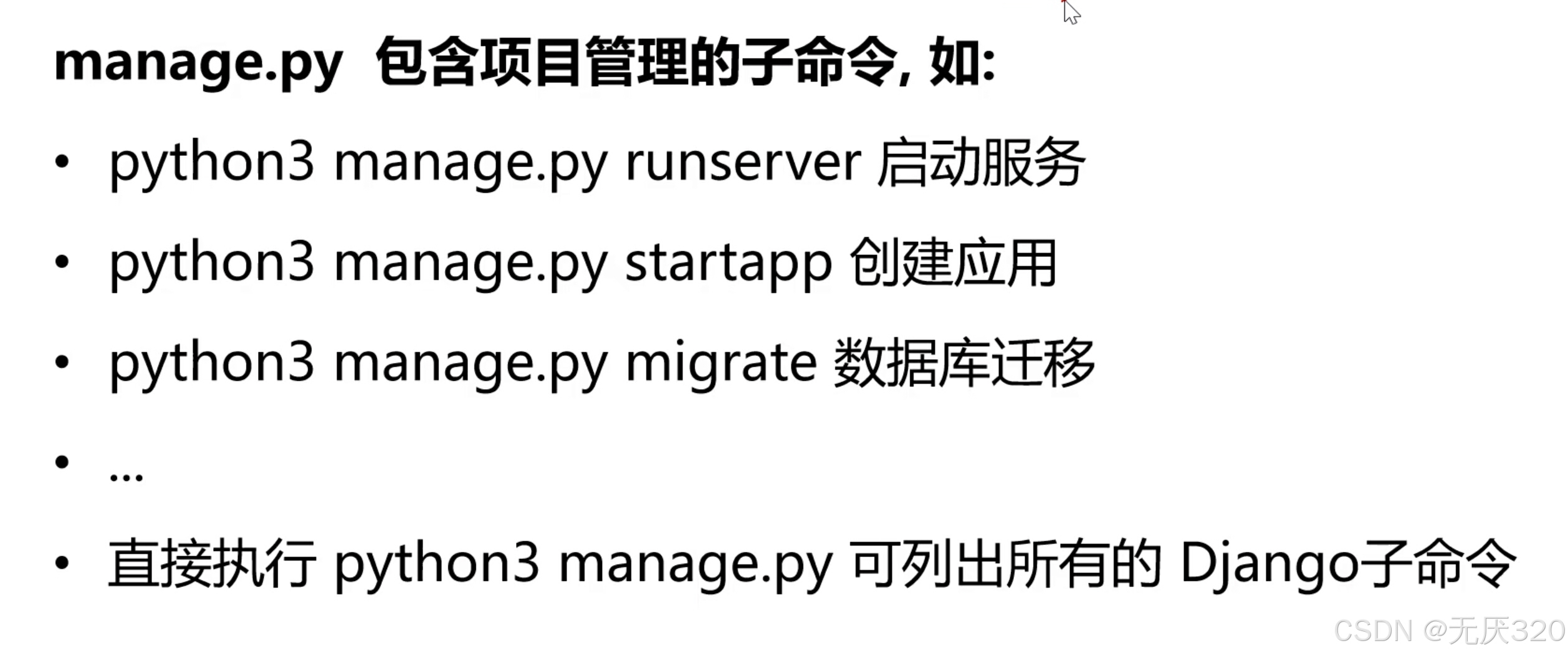
项目同名目录:
settings.py 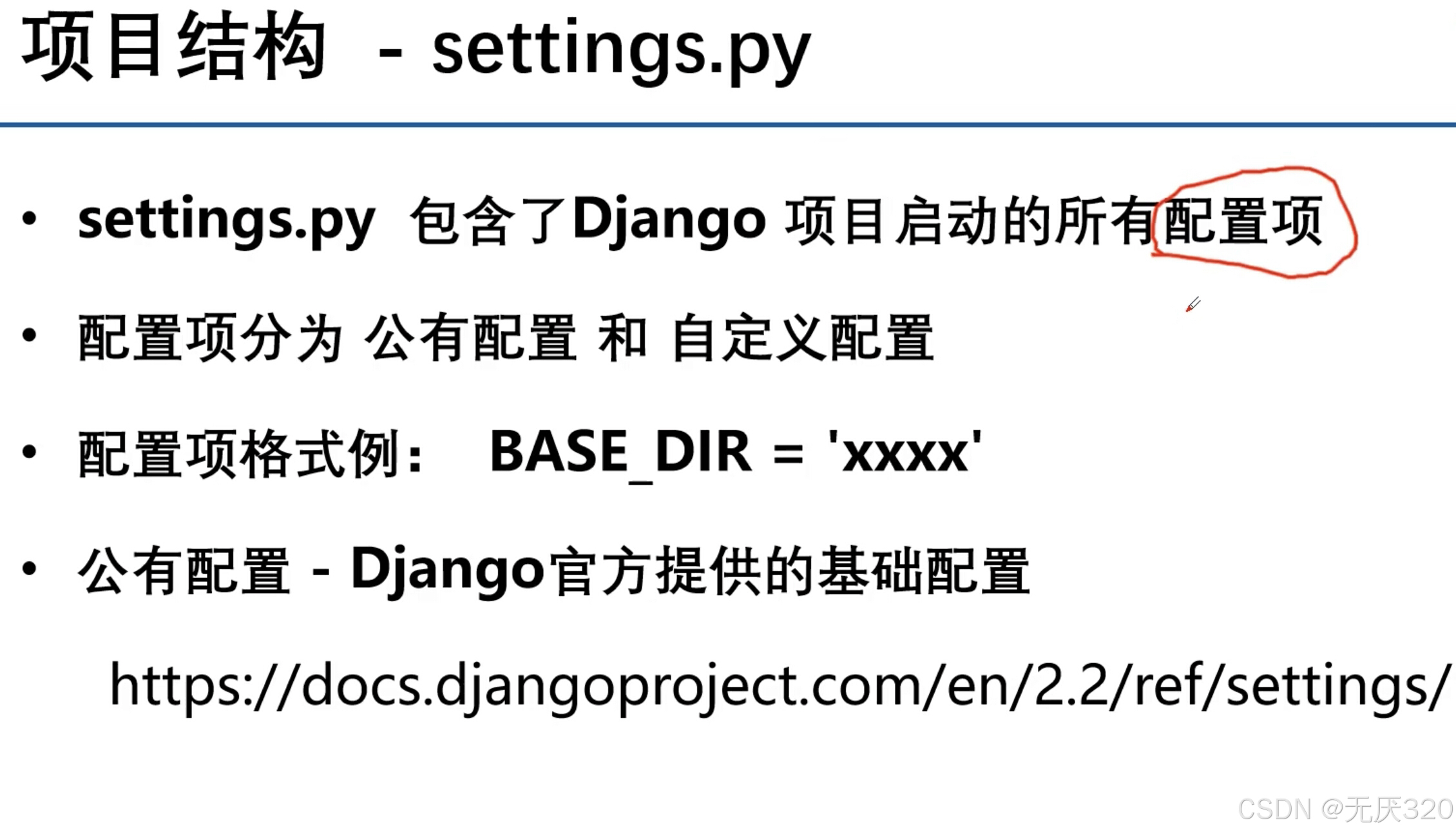
settings中的配置详解:
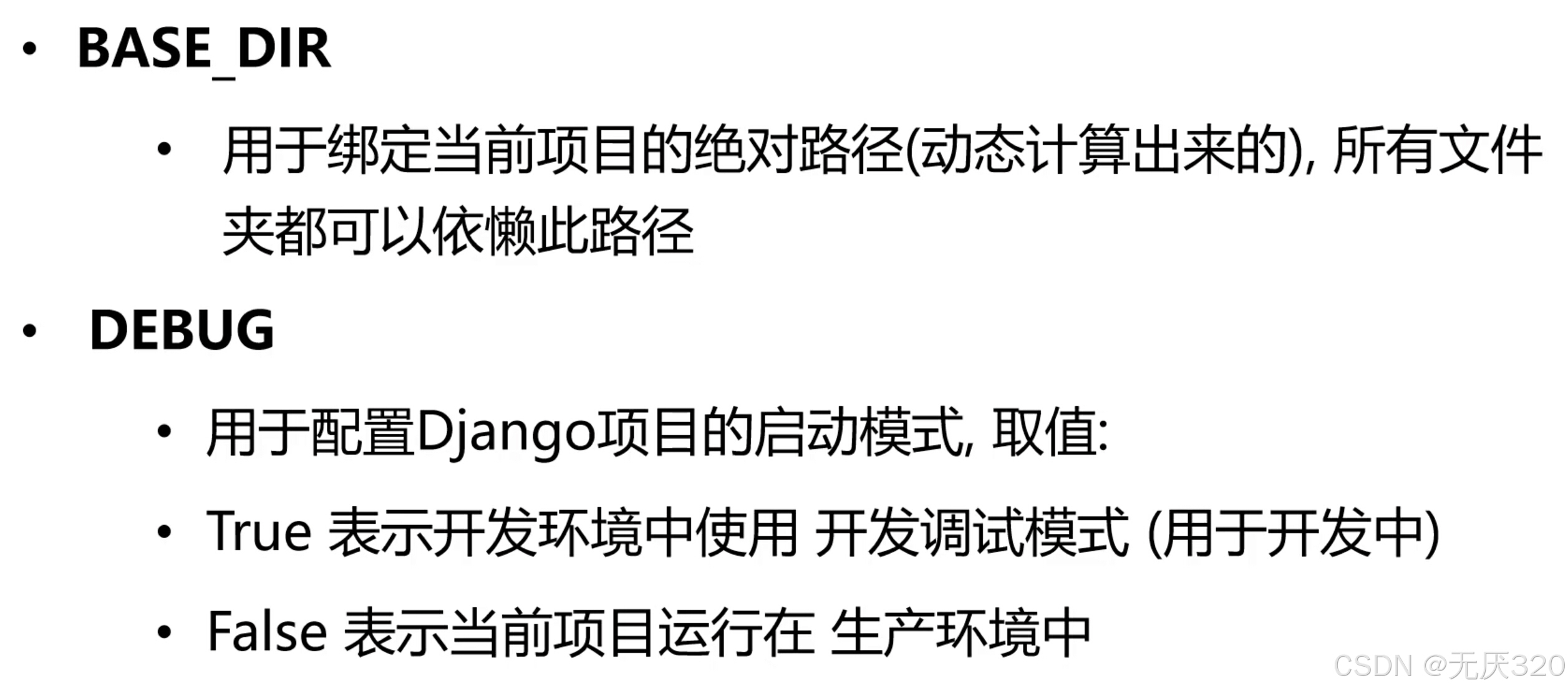
注意:添加自定义配置时名称必须大写
django处理url的过程
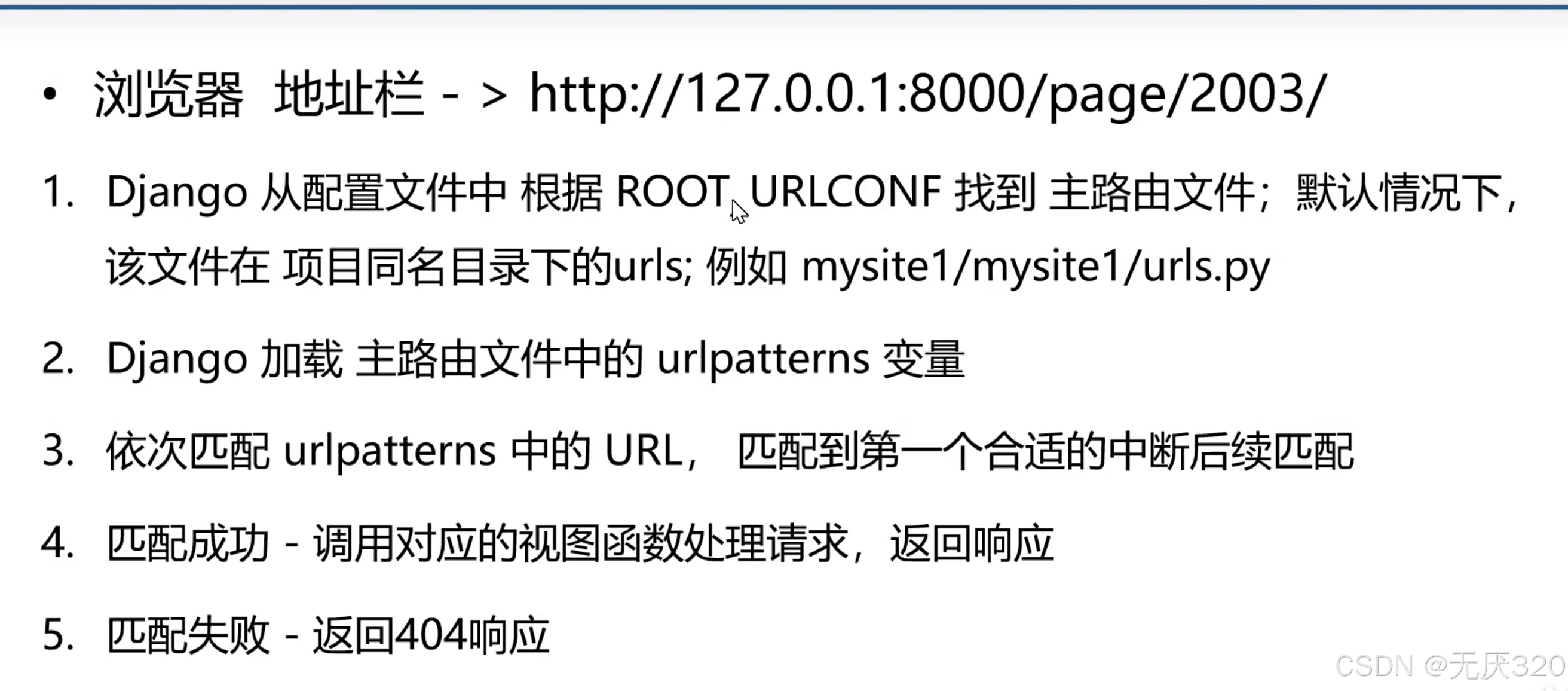
视图函数
路由配置 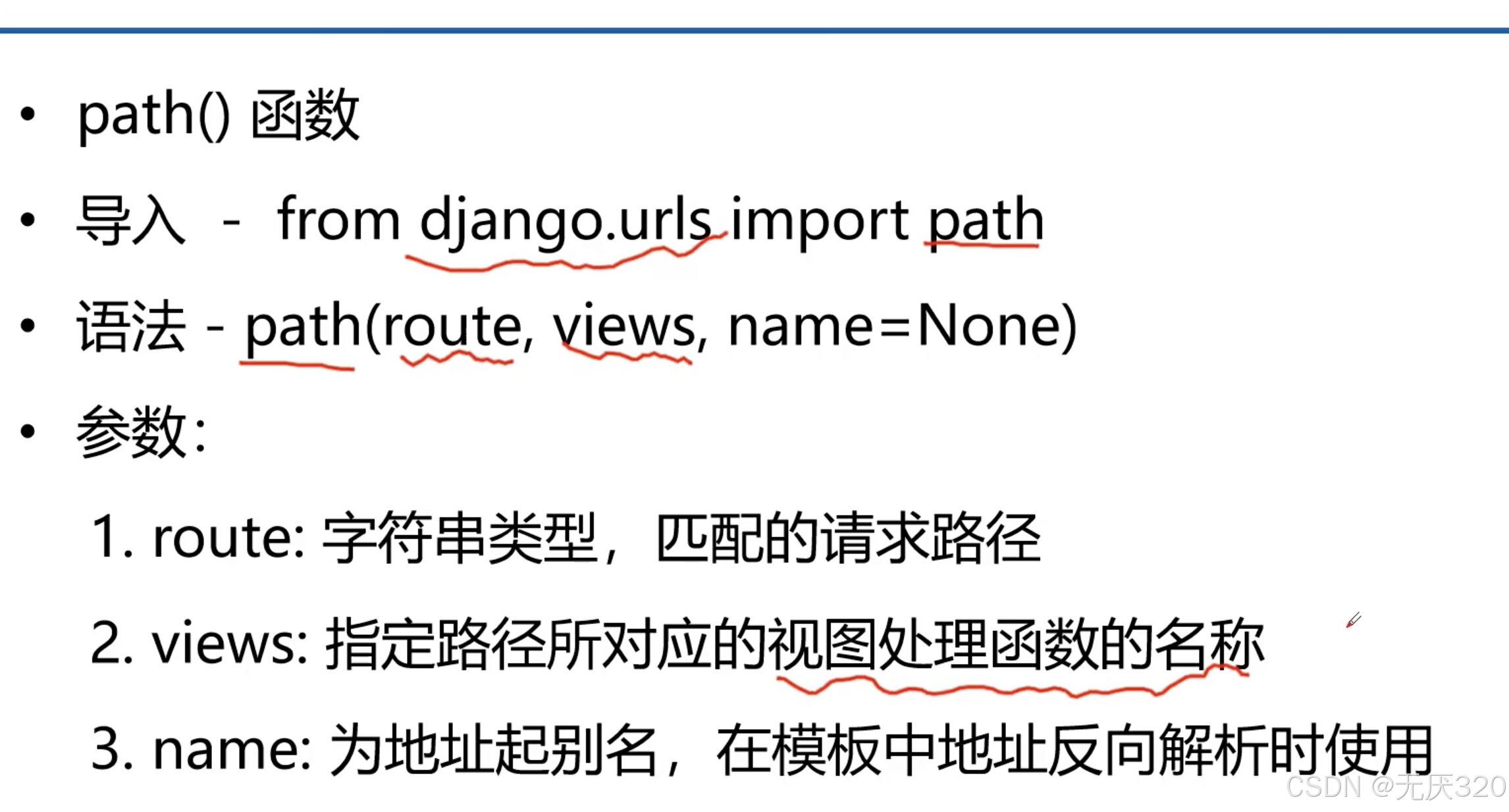
path转换器
re_path
Django中的请求
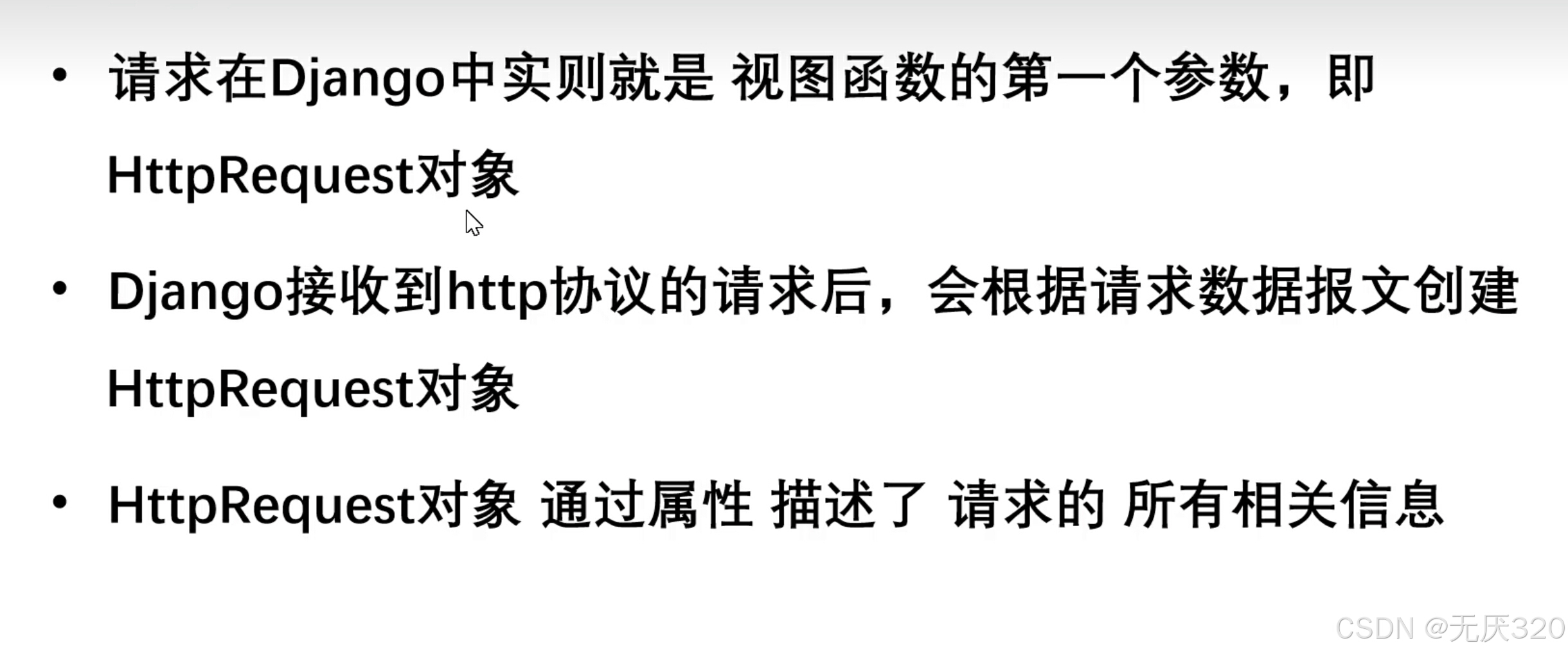
request下的函数
django响应对象:
代码展示:
python
from django.contrib import admin
from django.urls import path
from . import views
from django.urls import re_path
urlpatterns = [
path('admin/', admin.site.urls),
path('page/2004/', views.page_2003_view),
path('', views.index_view),
path('page/1', views.page_1_view),
path('page/2', views.page_2_view),
path('page/<int:pg>', views.page_view),
# 正则表达式匹配
# x为数字(1位和两位),op为字母,y为数字(1位和两位),$表示结束
re_path(r'^(?P<x>\d{1,2})/(?P<op>\w+)/(?P<y>/d{1,2})$', views.cal2_view),
path('<int:n>/<str:op>/<int:m>', views.cal_view),
re_path(r'^birthday/(?P<b>\d{4})/(?P<c>\d{1,2})/(?P<d>\d{1,2})$', views.date_view),
path('aa/', views.test_request)
]
python
from django.http import HttpResponse
def page_2003_view(request):
html = "<h1>这是一个页面</h1>"
return HttpResponse(html)
def index_view(request):
html = "<h1>这是一个首页</h1>"
return HttpResponse(html)
def page_1_view(request):
html = "<h1>这是编号为1的页面</h1>"
return HttpResponse(html)
def page_2_view(request):
html = "<h1>这是编号为2的页面</h1>"
return HttpResponse(html)
def page_view(request, pg):
html = f"<h1>这是编号为{pg}的页面</h1>"
return HttpResponse(html)
def cal_view(request, n, op, m):
if op not in ['add', 'sub', 'mul']:
return HttpResponse('Your op is wrong')
result = 0
if op == 'add':
result = n + m
elif op == 'sub':
result = n - m
elif op == 'mul':
result = n * m
return HttpResponse(f'结果为{result}')
def cal2_view(request, x, op, y):
if op not in ['add', 'sub', 'mul']:
return HttpResponse('Your op is wrong')
result = 0
if op == 'add':
result = x + y
elif op == 'sub':
result = x - y
elif op == 'mul':
result = x * y
return HttpResponse(f'结果shi{result}')
def date_view(request, a, b, c, d):
html = f"<h1>生日为{b}-{c}-{d}</h1>"
return HttpResponse(html)
def test_request(request):
print("url字符集:", request.path_info)
print("method is", request.method)
print("querystring is", request.GET)
print(request.get_full_path())
return HttpResponse("html")
请求过程:
服务起来之后,输入urls.py中配置的连接,就会跳转到链接对应的在views.py文件中编写的视图对象