**题意:**使用JavaScript与Azure OpenAI进行连接
问题背景:
I have created my chatbot with javascript and used open ai. I need to change it to azure open ai but can not find the connection details for javascript. This is how i connect with python :
我已经使用JavaScript创建了聊天机器人,并使用了OpenAI。我现在需要将其更改为Azure OpenAI,但找不到用于JavaScript的连接详细信息。以下是我如何使用Python进行连接的方法:
python
import os
import openai
openai.api_type = "azure"
openai.api_base = "https://test-azure-openai-d.openai.azure.com/"
openai.api_version = "2022-12-01"
openai.api_key = os.getenv("OPENAI_API_KEY")
This was the code in js for openai :
这是用于OpenAI的JavaScript代码:
python
import express from 'express';
import * as dotenv from 'dotenv';
import cors from 'cors';
import { Configuration, OpenAIApi } from 'openai';
dotenv.config()
//console.log(process.env.OPENAI_API_KEY)
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
So i would need the connection in javascript
所以,我需要的是JavaScript中的连接(方式/代码)。
问题解决:
I believe that the OpenAI package does not support Azure endpoints yet. You can refer to this open issue on the GitHub repo.
我相信OpenAI包目前还不支持Azure端点。您可以参考GitHub仓库上的这个开放问题。
So for now you have two options: Make raw API calls using fetch
or axios
. You can refer to API reference documentation on Azure website.
所以目前你有两个选择:使用fetch
或axios
进行原始API调用。你可以参考Azure网站上的API参考文档。
python
const basePath = "https://test-azure-openai-d.openai.azure.com/"
const apiVersion = "2022-12-01"
const apiKey = process.env.OPENAI_API_KEY
const url = `${basePath}/openai/deployments/${data.model}/completions?api-version=${apiVersion}`;
const response = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'api-key': `${apiKey}`,
},
body: JSON.stringify(data),
});
return await response.json();
Or use the azure-openai package
或者使用azure-openai
包
python
import { Configuration, OpenAIApi } from "azure-openai";
this.openAiApi = new OpenAIApi(
new Configuration({
apiKey: this.apiKey,
// add azure info into configuration
azure: {
apiKey: {your-azure-openai-resource-key},
endpoint: {your-azure-openai-resource-endpoint},
// deploymentName is optional, if you donot set it, you need to set it in the request parameter
deploymentName: {your-azure-openai-resource-deployment-name},
}
}),
);
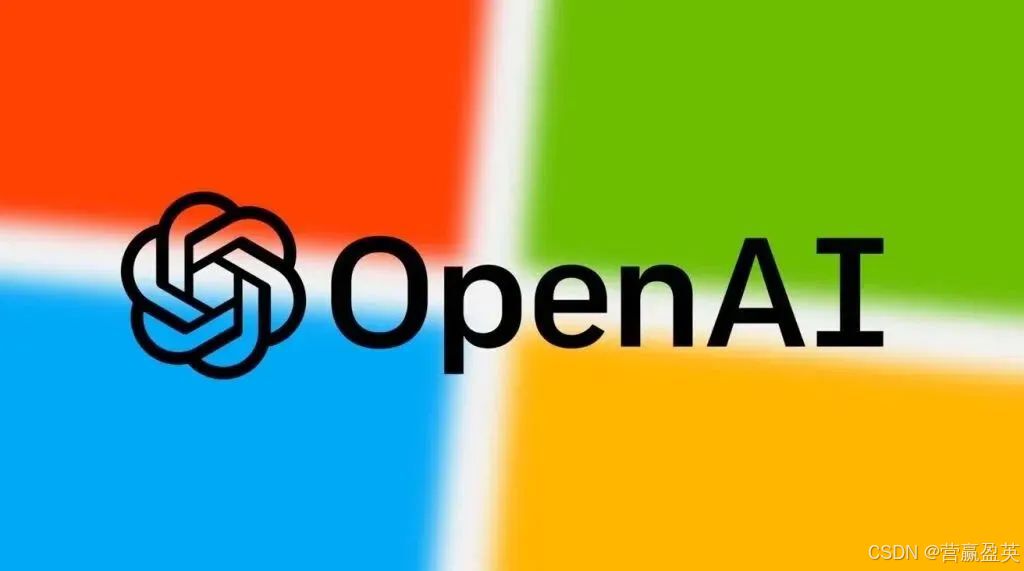