一、Protobuf
Protobuf是接口规范的描述语言,可以通过工具生成代码,将结构化数据序列化。
二、grpc
gRPC 是 Google 公司基于 Protobuf 开发的跨语言的开源 RPC 框架。
三、使用教程
3.1 student.proto
syntax = "proto3";
import "google/api/annotations.proto";
package main;
option go_package = "/main;student";
message Student {
string name = 1;
int32 age = 2;
repeated int32 scores = 3;
}
service StudentService {
rpc GetStudent(Student) returns (Student){
option (google.api.http) = {
post: "/v1/student/get"
body: "*"
};
};
}
3.2 根据proto文件生成接口和结构定义
third_party 存放annotations.proto
bash
protoc --proto_path=./third_party --proto_path=. --go_out=. --go-grpc_out=. student.proto
编译生成目标文件
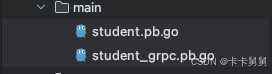
3.3 grpc provider 实现
Go
// 定义提供者
type StudentService struct {
student.UnimplementedStudentServiceServer `wire:"-"`
}
// 实现提供者方法
func (s *StudentService) GetStudent(context.Context, *student.Student) (*student.Student, error) {
return &student.Student{
Age: 18,
Name: "vicyor",
Scores: []int32{1, 2, 3, 4, 5},
}, nil
}
3.4 grpc server 实现
Go
func main_grpc() {
serverPort := 50051
// 创造grpc服务端
server := grpc.NewServer()
// 创建listener
lis, _ := net.Listen("tcp", fmt.Sprintf(":%d", serverPort))
// 注册grpc服务
student.RegisterStudentServiceServer(server, &StudentService{})
// grpc 启动
server.Serve(lis)
}
3.5 grpc client 实现
Go
func main_grpc() {
<-time.NewTimer(time.Second * 2).C
// 这里启动一个消费者
conn, _ := grpc.NewClient("127.0.0.1:50051",
grpc.WithTransportCredentials(insecure.NewCredentials()))
defer conn.Close()
cli := student.NewStudentServiceClient(conn)
// 3秒读超时
ctx, _ := context.WithTimeout(context.Background(), time.Second*3)
res, _ := cli.GetStudent(ctx, &student.Student{})
fmt.Println(res)
}