Python进阶之3D图形
在数据可视化中,2D图形通常可以满足大多数需求。然而,对于一些复杂的数据或分析,3D图形可以提供更多的视角和洞察。在Python中,使用 Matplotlib
和 Plotly
等库可以轻松创建各种3D图形。本文将介绍如何使用这些工具绘制3D图形,并展示一些高级用法。
1. 使用Matplotlib绘制3D图形
Matplotlib
提供了一个 mplot3d
模块,用于创建3D图形。以下是一些常见的3D图形示例。
1.1 3D散点图
python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 示例数据
np.random.seed(0)
x = np.random.rand(100)
y = np.random.rand(100)
z = np.random.rand(100)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(x, y, z, c='blue', marker='o')
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
ax.set_zlabel('Z Label')
ax.set_title('3D Scatter Plot')
plt.show()
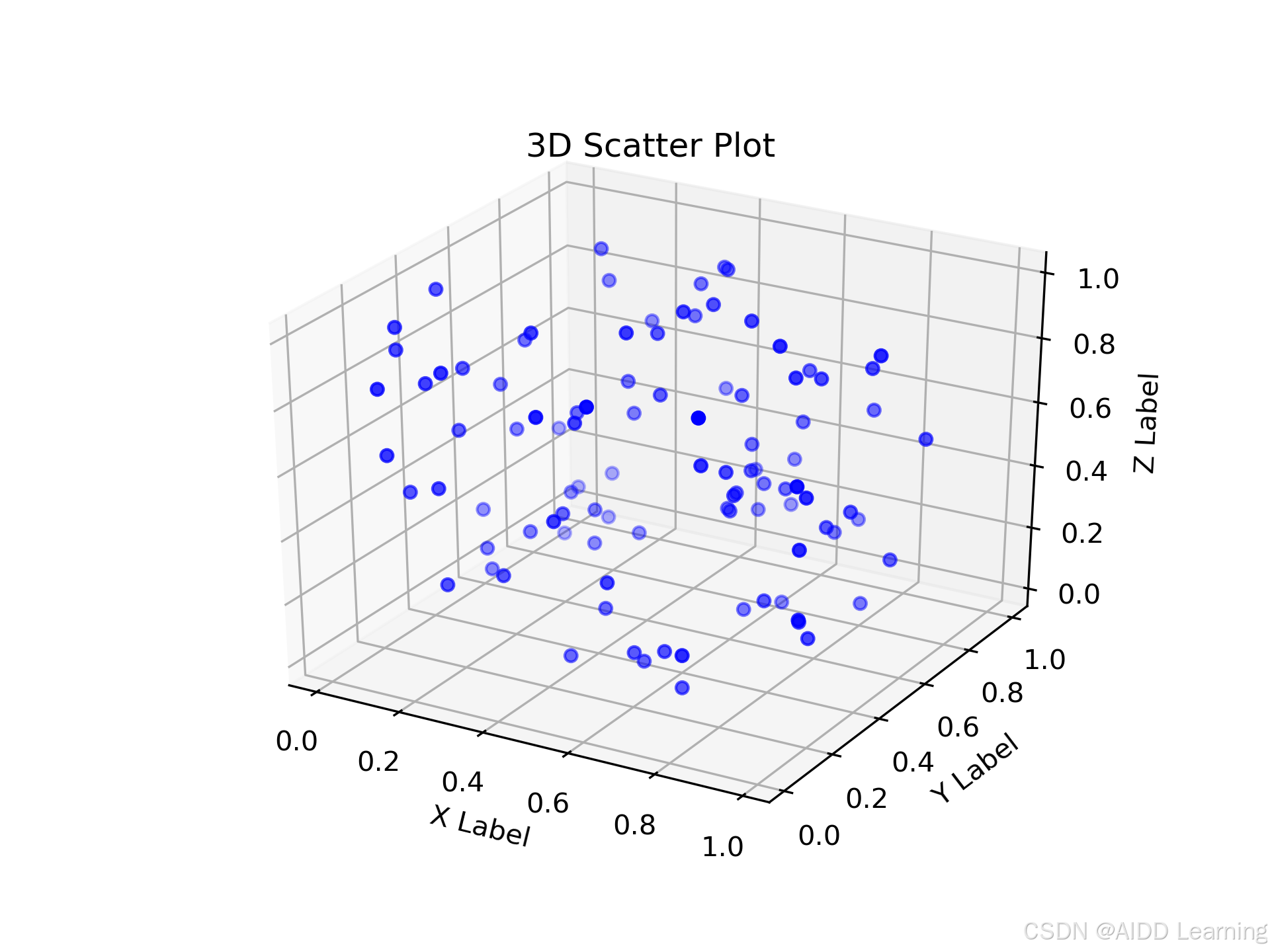
1.2 3D曲面图
python
from mpl_toolkits.mplot3d import Axes3D
# 示例数据
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
x, y = np.meshgrid(x, y)
z = np.sin(np.sqrt(x**2 + y**2))
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(x, y, z, cmap='viridis')
ax.set_xlabel('X Label')
ax.set_ylabel('Y Label')
ax.set_zlabel('Z Label')
ax.set_title('3D Surface Plot')
plt.show()
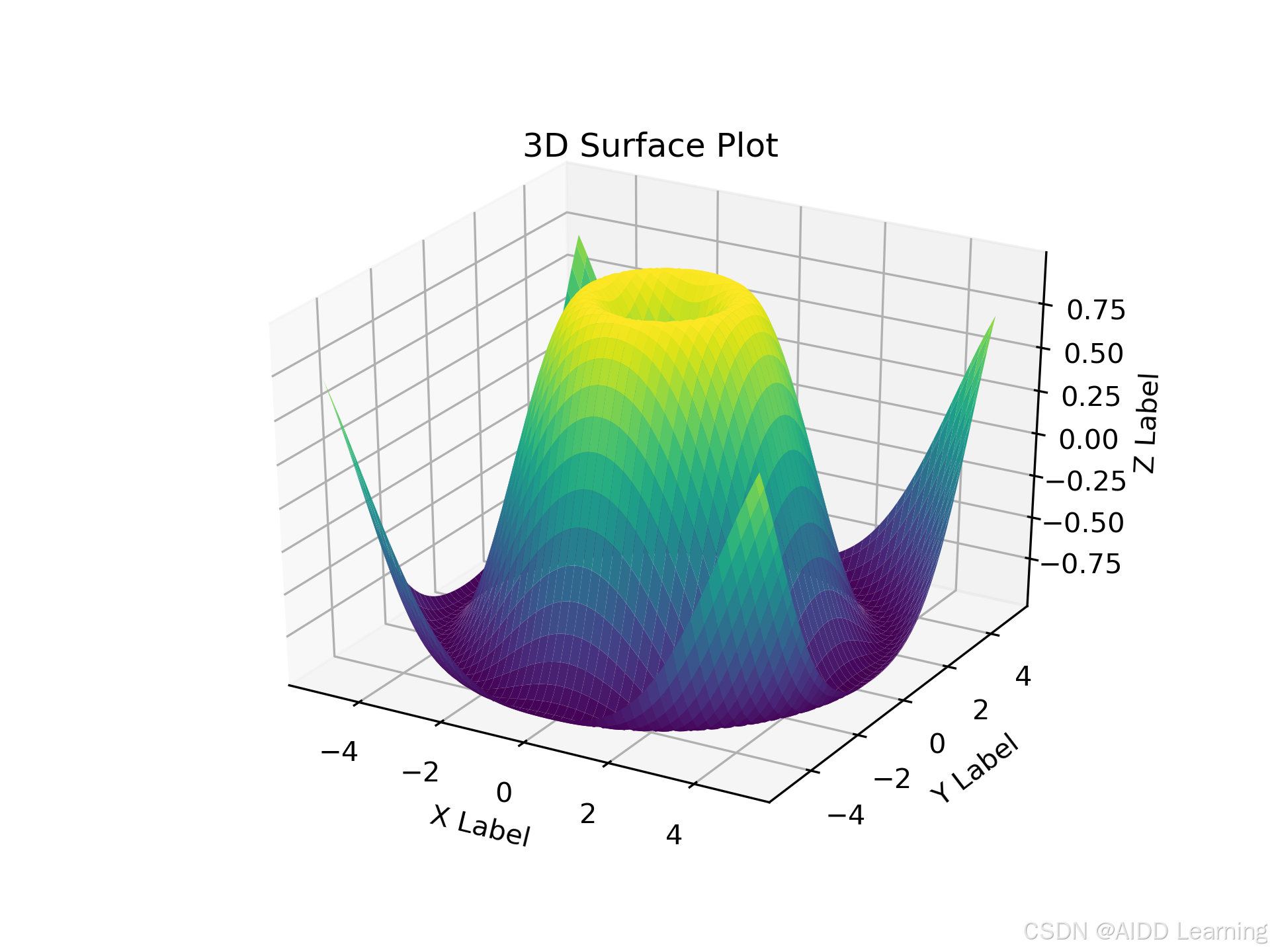
1.3 3D柱状图
python
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(projection='3d')
x, y = np.random.rand(2, 100) * 4
hist, xedges, yedges = np.histogram2d(x, y, bins=4, range=[[0, 4], [0, 4]])
# Construct arrays for the anchor positions of the 16 bars.
xpos, ypos = np.meshgrid(xedges[:-1] + 0.25, yedges[:-1] + 0.25, indexing="ij")
xpos = xpos.ravel()
ypos = ypos.ravel()
zpos = 0
# Construct arrays with the dimensions for the 16 bars.
dx = dy = 0.5 * np.ones_like(zpos)
dz = hist.ravel()
ax.bar3d(xpos, ypos, zpos, dx, dy, dz, zsort='average')
plt.show()
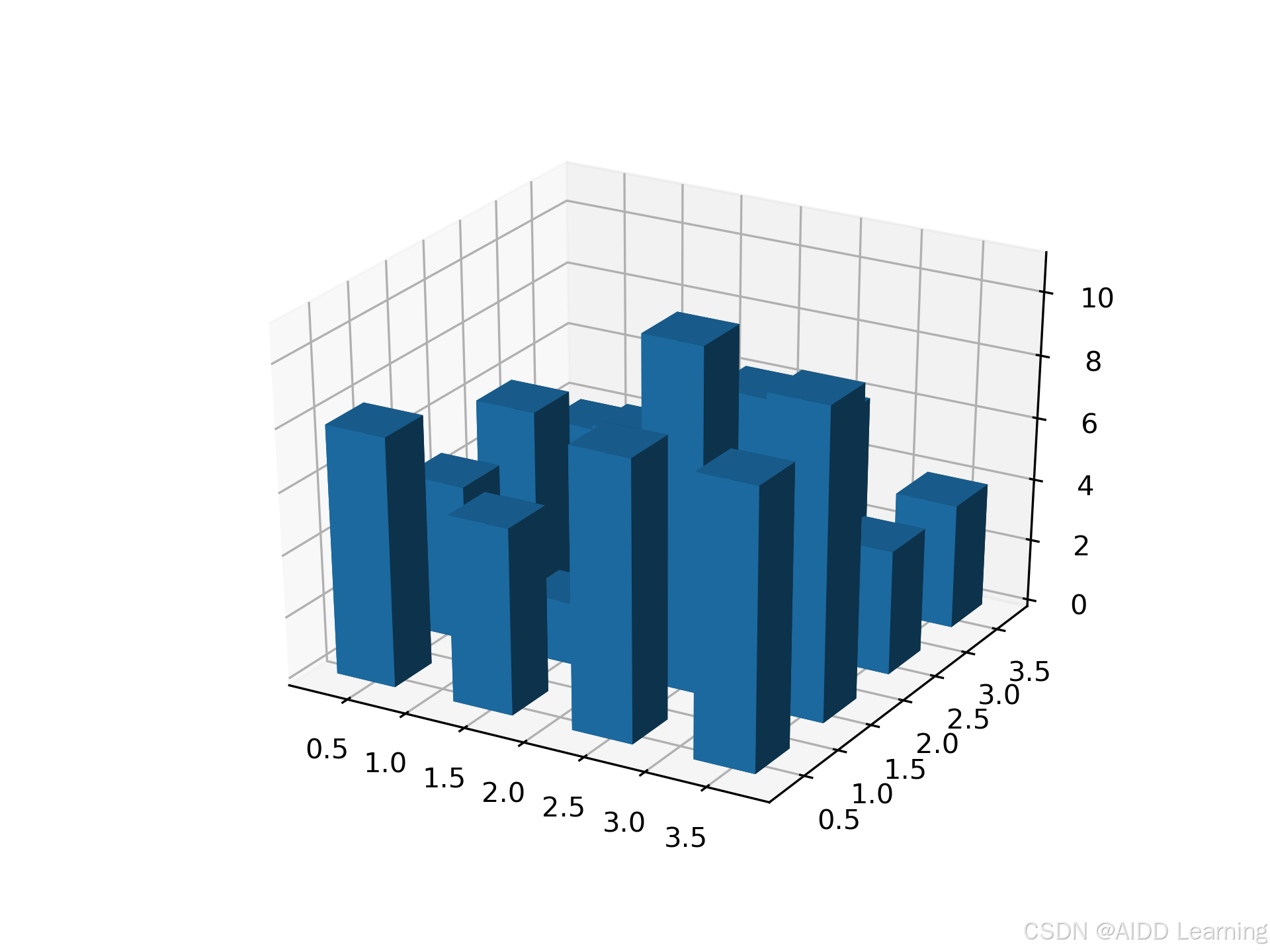
2. 使用Plotly绘制3D图形
Plotly
提供了更为交互的3D图形功能,可以使图表的操作更加灵活和动态。
2.1 3D散点图
python
import plotly.graph_objs as go
import plotly.offline as pyo
# 示例数据
trace = go.Scatter3d(
x=np.random.rand(100),
y=np.random.rand(100),
z=np.random.rand(100),
mode='markers',
marker=dict(size=5, color='blue')
)
layout = go.Layout(
title='3D Scatter Plot',
scene=dict(
xaxis_title='X Label',
yaxis_title='Y Label',
zaxis_title='Z Label'
)
)
fig = go.Figure(data=[trace], layout=layout)
pyo.iplot(fig)
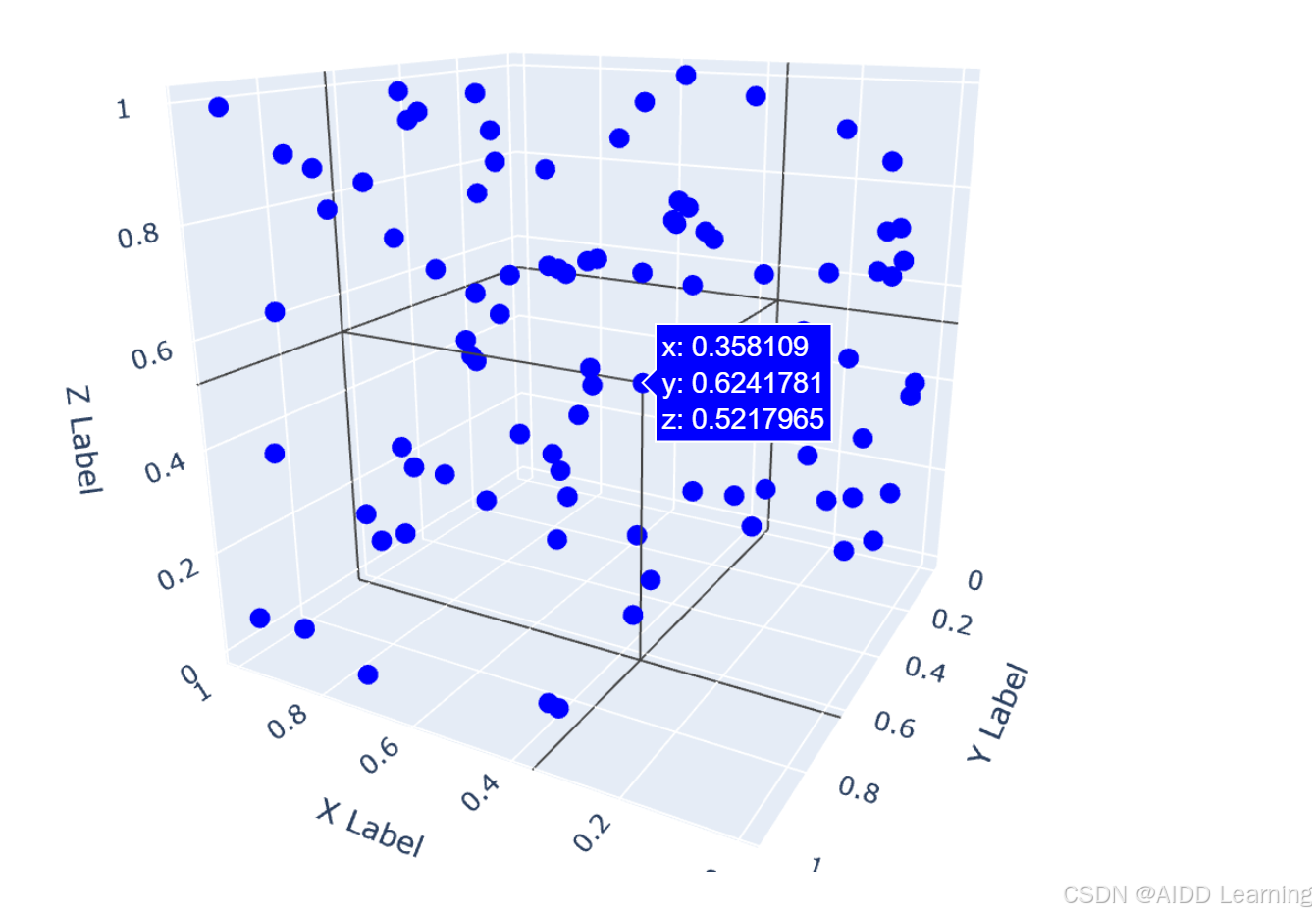
2.2 3D曲面图
python
import plotly.graph_objs as go
import plotly.offline as pyo
# 示例数据
x = np.linspace(-5, 5, 50)
y = np.linspace(-5, 5, 50)
x, y = np.meshgrid(x, y)
z = np.sin(np.sqrt(x**2 + y**2))
trace = go.Surface(
z=z,
x=x,
y=y,
colorscale='Viridis'
)
layout = go.Layout(
title='3D Surface Plot',
scene=dict(
xaxis_title='X Label',
yaxis_title='Y Label',
zaxis_title='Z Label'
)
)
fig = go.Figure(data=[trace], layout=layout)
pyo.iplot(fig)
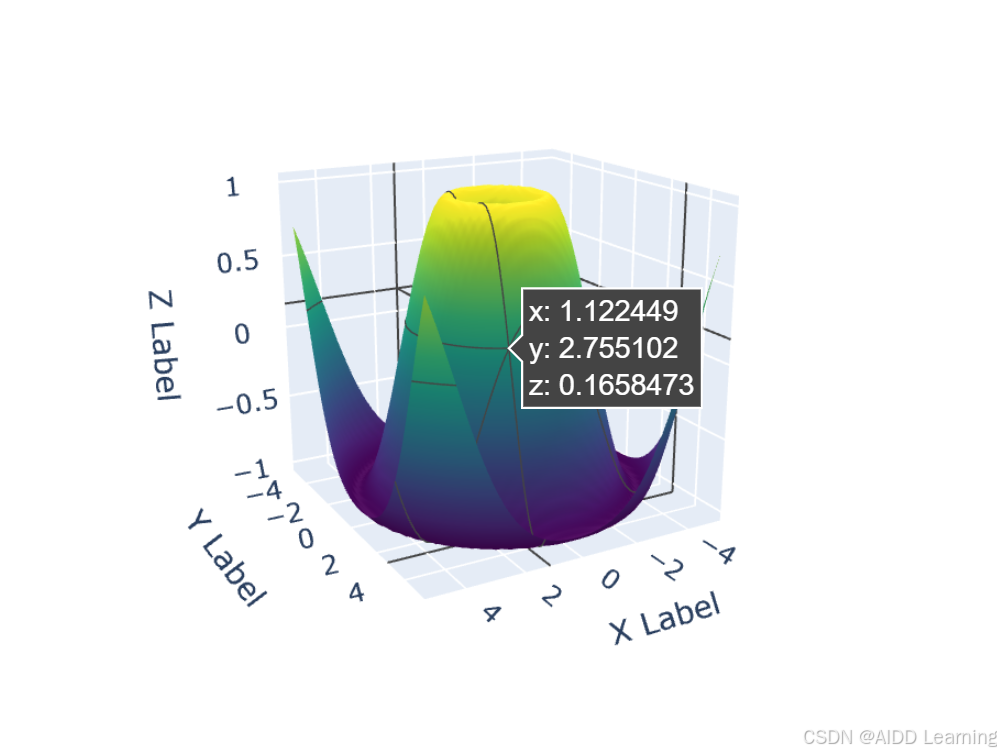
2.3 3D网格图
python
import plotly.graph_objs as go
import plotly.offline as pyo
# 示例数据
x = np.arange(10)
y = np.arange(10)
x, y = np.meshgrid(x, y)
z = np.sin(x) + np.cos(y)
trace = go.Mesh3d(
x=x.flatten(),
y=y.flatten(),
z=z.flatten(),
i=[0, 1, 2],
j=[1, 2, 3],
k=[2, 3, 4],
opacity=0.5
)
layout = go.Layout(
title='3D Mesh Plot',
scene=dict(
xaxis_title='X Label',
yaxis_title='Y Label',
zaxis_title='Z Label'
)
)
fig = go.Figure(data=[trace], layout=layout)
pyo.iplot(fig)
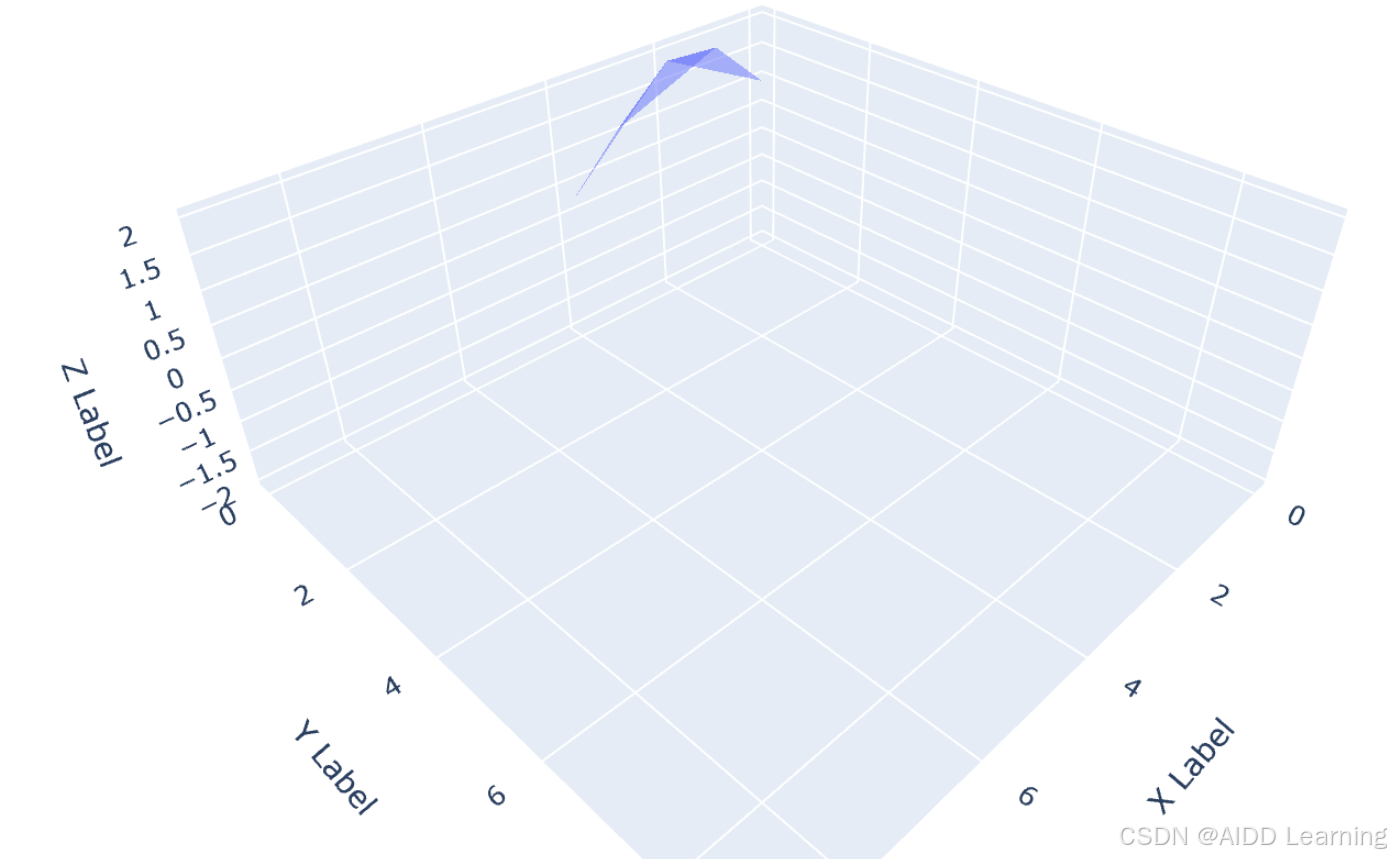
3. 3D图形的应用场景
- 科学数据:如分子结构、地理数据、气象数据等。
- 工程分析:如应力分布、流体力学等。
- 数据探索:通过3D图形发现数据中的模式和异常。
结语
3D图形能够提供额外的维度和视角,帮助更全面地理解数据。在Python中,Matplotlib
和 Plotly
提供了丰富的工具来创建和展示3D图形。希望本文的示例和技巧能够帮助你在数据可视化中更好地利用3D图形。如果你有更多的3D图形需求或问题,欢迎在评论区交流和讨论!
试着使用上述方法创建自己的3D图形,并分享你的成果和心得。你也可以尝试将不同的3D图形结合起来,探索它们在数据分析中的应用。完成后,欢迎在评论区留言,我们可以一起讨论如何进一步优化和改进!
为了方便大家交流和学习,建立了一个绘图交流学习群,欢迎大家的加入。
"Python绘图"专栏已完结,后续会出有关具体的图形的绘制教程,感兴趣的可以关注"AIDD Learning"。