目录
- [1- 思路](#1- 思路)
- [2- 实现](#2- 实现)
-
- [⭐23. 合并 K 个升序链表------题解思路](#⭐23. 合并 K 个升序链表——题解思路)
- [3- ACM 实现](#3- ACM 实现)
- 原题连接:23. 合并 K 个升序链表
1- 思路
优先队列
- 1- 提供的数据结构:
ListNode[] lists
- 2- 由于提供的数据结构已经是有序的,不能通过指针实现是因为不知道一共要定义多少个指针来遍历链表
- 3- 所以引出优先队列解决,将链表的数据加入 优先队列,实现排序
实现思路
java
PriorityQueue<ListNode> pq = new PriorityQueue<>(len,Comparator.comparingInt(a -> a.val));
- 上述操作实际上实现了一个最小堆,每次弹出的是一个堆顶元素。
- 如果定义的是升序优先队列,则其弹出
poll
的时候,始终是最小的元素先弹出``
2- 实现
⭐23. 合并 K 个升序链表------题解思路
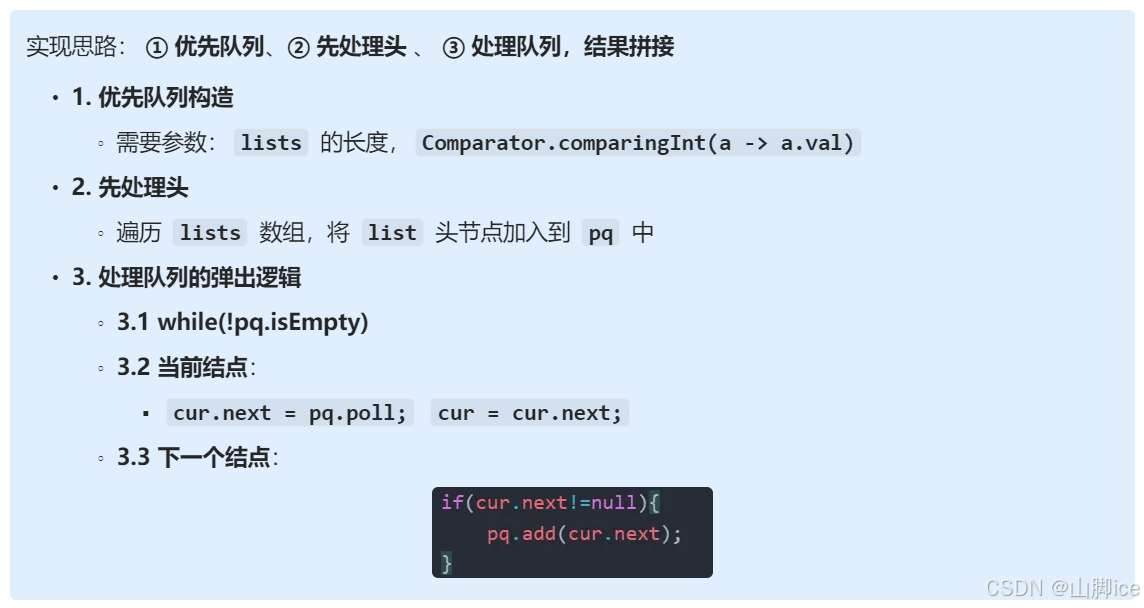
java
class Solution {
public ListNode mergeKLists(ListNode[] lists) {
ListNode dummyHead = new ListNode(-1);
ListNode cur = dummyHead;
// 定义长度
int len = lists.length;
if(len==0){
return null;
}
// 1.优先队列
PriorityQueue<ListNode> pq = new PriorityQueue<>(len,Comparator.comparingInt(a -> a.val));
// 2.先加入头
for(ListNode list:lists){
if(list!=null){
pq.add(list);
}
}
// 3. 处理弹出逻辑
while(!pq.isEmpty()){
cur.next = pq.poll();
cur = cur.next;
if(cur.next!=null){
pq.add(cur.next);
}
}
return dummyHead.next;
}
}
3- ACM 实现
java
public class mergeKLists {
public static class ListNode {
int val;
ListNode next;
ListNode(int x) {
val = x;
next = null;
}
}
public static ListNode mergKLists(ListNode[] lists){
// 1. 求长度
int len = lists.length;
if(len==0){
return null;
}
// 1. 定义 pq
PriorityQueue<ListNode> pq = new PriorityQueue<>(len, Comparator.comparingInt(a -> a.val));
// 2.处理头
for(ListNode list:lists){
if(list!=null){
pq.add(list);
}
}
ListNode dummyHead = new ListNode(-1);
ListNode cur = dummyHead;
// 3. 处理pq
while(!pq.isEmpty()){
cur.next = pq.poll();
cur = cur.next;
if(cur.next!=null){
pq.add(cur.next);
}
}
return dummyHead.next;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("数组长度");
int k = scanner.nextInt();
ListNode[] lists = new ListNode[k];
for (int i = 0; i < k; i++) {
System.out.println("输入链表长度");
int n = scanner.nextInt();
if (n == 0) {
lists[i] = null;
} else {
lists[i] = new ListNode(scanner.nextInt());
ListNode current = lists[i];
for (int j = 1; j < n; j++) {
current.next = new ListNode(scanner.nextInt());
current = current.next;
}
}
}
ListNode forRes = mergKLists(lists);
while(forRes!=null){
System.out.print(forRes.val+" ");
forRes = forRes.next;
}
}
}