- 操作系统:ubuntu22.04
- OpenCV版本:OpenCV4.9
- IDE:Visual Studio Code
- 编程语言:C++11
算法描述
绘制一个从第一个点指向第二个点的箭头线段。
cv::arrowedLine 函数在图像中绘制一个从 pt1 到 pt2 的箭头。另见 line 函数。
函数原型
cpp
void cv::arrowedLine
(
InputOutputArray img,
Point pt1,
Point pt2,
const Scalar & color,
int thickness = 1,
int line_type = 8,
int shift = 0,
double tipLength = 0.1
)
参数
- 参数img 图像.
- 参数pt1 箭头起始的点。
- 参数pt2 箭头指向的点。
- 参数color 线条的颜色。
- 参数thickness 线条的粗细。
- 参数line_type 线条的类型。参见 LineTypes。
- 参数shift 点坐标中的小数位数。
- 参数tipLength 相对于箭头长度的箭头尖端长度。
代码示例
cpp
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
int main(int argc, char** argv)
{
// 创建一个空白的图像
cv::Mat image = cv::Mat::zeros(512, 512, CV_8UC3);
// 定义箭头的起点和终点
cv::Point pt1(100, 100); // 起始点
cv::Point pt2(400, 400); // 结束点
// 设置箭头颜色为红色
cv::Scalar color(0, 0, 255);
// 设置箭头的宽度
int thickness = 2;
// 设置箭头线条类型
int line_type = 8; // 可以是 8 或者 CV_AA (抗锯齿)
// 设置箭头尖端的长度
double tipLength = 0.15;
// 绘制箭头
cv::arrowedLine(image, pt1, pt2, color, thickness, line_type, 0, tipLength);
// 显示图像
cv::imshow("Arrowed Line Example", image);
cv::waitKey(0);
return 0;
}
运行结果
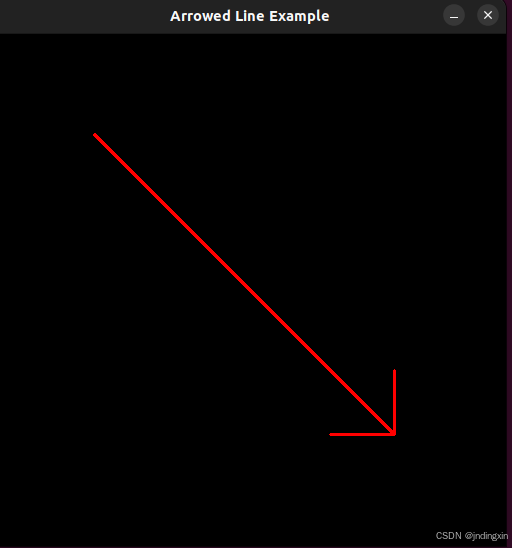