Spring 事件机制
允许在应用程序中不同的组件之间进行松耦合的通信。下面是一个简单的例子,展示如何在 Spring 中使用事件机制。
java
import org.springframework.context.ApplicationEvent;
public class CustomEvent extends ApplicationEvent {
private String message;
public CustomEvent(Object source, String message) {
super(source);
this.message = message;
}
public String getMessage() {
return message;
}
}

java
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Component;
@Component
public class CustomEventListener implements ApplicationListener<CustomEvent> {
@Override
public void onApplicationEvent(CustomEvent event) {
System.out.println("Received custom event - " + event.getMessage());
}
}

java
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.context.ApplicationEventPublisherAware;
import org.springframework.stereotype.Component;
@Component
public class CustomEventPublisher implements ApplicationEventPublisherAware {
private ApplicationEventPublisher eventPublisher;
@Override
public void setApplicationEventPublisher(ApplicationEventPublisher
applicationEventPublisher) {
this.eventPublisher = applicationEventPublisher;
}
public void publishEvent(String message) {
CustomEvent customEvent = new CustomEvent(this, message);
eventPublisher.publishEvent(customEvent);
}
}

java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class EventDemo {
private final CustomEventPublisher customEventPublisher;
@Autowired
public EventDemo(CustomEventPublisher customEventPublisher) {
this.customEventPublisher = customEventPublisher;
}
public void run() {
customEventPublisher.publishEvent("Hello, Spring Event!");
}
}
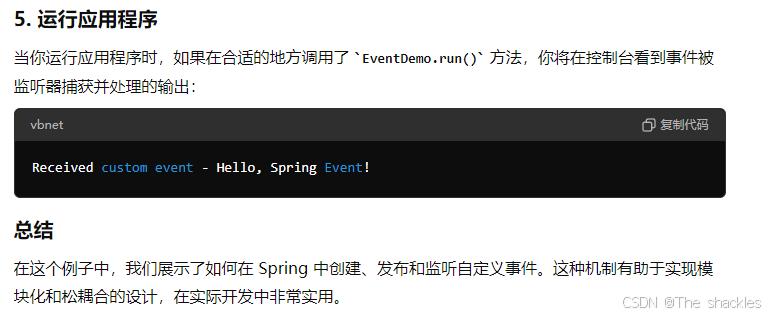