目录
492、pandas.DataFrame.describe方法

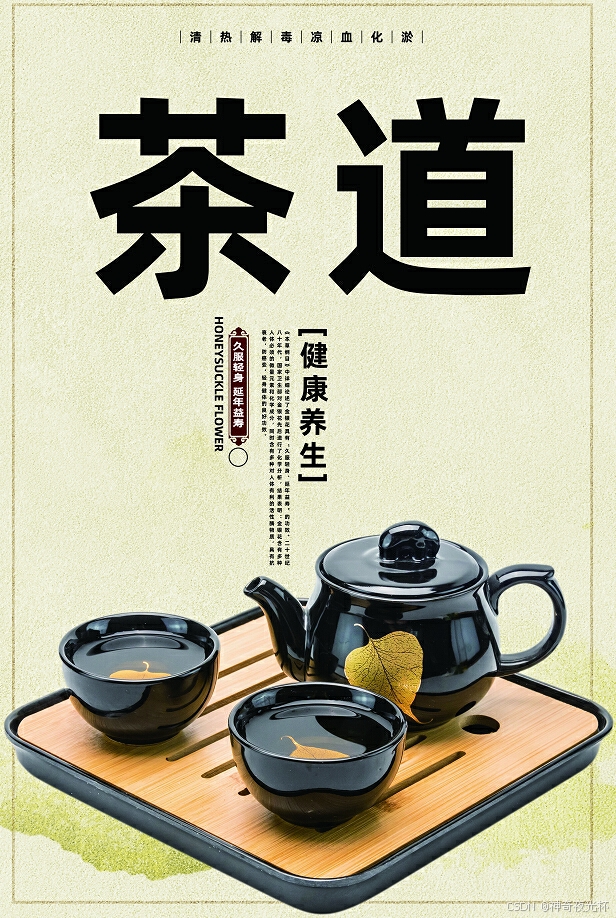

一、用法精讲
491、pandas.DataFrame.cumsum方法
491-1、语法
python
# 491、pandas.DataFrame.cumsum方法
pandas.DataFrame.cumsum(axis=None, skipna=True, *args, **kwargs)
Return cumulative sum over a DataFrame or Series axis.
Returns a DataFrame or Series of the same size containing the cumulative sum.
Parameters:
axis
{0 or 'index', 1 or 'columns'}, default 0
The index or the name of the axis. 0 is equivalent to None or 'index'. For Series this parameter is unused and defaults to 0.
skipna
bool, default True
Exclude NA/null values. If an entire row/column is NA, the result will be NA.
*args, **kwargs
Additional keywords have no effect but might be accepted for compatibility with NumPy.
Returns:
Series or DataFrame
Return cumulative sum of Series or DataFrame.
491-2、参数
491-2-1、axis**(可选,默认值为None)****:**{0 or 'index', 1 or 'columns'},指定沿哪个轴计算累积和,0表示按列计算,1表示按行计算。
491-2-2、skipna**(可选,默认值为True)****:**布尔值,是否忽略缺失值(NaN),如果为True,缺失值将被忽略;如果为False,结果中可能会出现NaN。
491-2-3、*args**(可选)****:**其他的额外位置参数,通常在使用自定义函数时会用到。
491-2-4、**kwargs**(可选)****:**其他的额外关键字参数,通常在使用自定义函数时会用到。
491-3、功能
用于计算DataFrame中每列或每行的累积和,它返回一个与原DataFrame形状相同的新DataFrame,其中每个值是其前面所有值的和。
491-4、返回值
返回一个与原DataFrame形状相同的新DataFrame,其中每个元素表示其前面所有元素的和。
491-5、说明
491-5-1、缺失值处理:如果skipna为True,所有NaN值将被忽略;如果为False,结果中的任何NaN都会导致后续的结果也为NaN。
491-5-2、适用于时间序列:cumsum方法也常用于处理时间序列数据,以帮助识别随时间变化的总和趋势。
491-5-3、效率:计算累积和的效率很高,适用于处理大规模数据集。
491-6、用法
491-6-1、数据准备
python
无
491-6-2、代码示例
python
# 491、pandas.DataFrame.cumsum方法
import pandas as pd
# 创建一个示例DataFrame
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
}
df = pd.DataFrame(data)
# 计算累积和
cumsum_df = df.cumsum()
print(cumsum_df)
491-6-3、结果输出
python
# 491、pandas.DataFrame.cumsum方法
# A B C
# 0 1 4 7
# 1 3 9 15
# 2 6 15 24
492、pandas.DataFrame.describe方法
492-1、语法
python
# 492、pandas.DataFrame.describe方法
pandas.DataFrame.describe(percentiles=None, include=None, exclude=None)
Generate descriptive statistics.
Descriptive statistics include those that summarize the central tendency, dispersion and shape of a dataset's distribution, excluding NaN values.
Analyzes both numeric and object series, as well as DataFrame column sets of mixed data types. The output will vary depending on what is provided. Refer to the notes below for more detail.
Parameters:
percentiles
list-like of numbers, optional
The percentiles to include in the output. All should fall between 0 and 1. The default is [.25, .5, .75], which returns the 25th, 50th, and 75th percentiles.
include
'all', list-like of dtypes or None (default), optional
A white list of data types to include in the result. Ignored for Series. Here are the options:
'all' : All columns of the input will be included in the output.
A list-like of dtypes : Limits the results to the provided data types. To limit the result to numeric types submit numpy.number. To limit it instead to object columns submit the numpy.object data type. Strings can also be used in the style of select_dtypes (e.g. df.describe(include=['O'])). To select pandas categorical columns, use 'category'
None (default) : The result will include all numeric columns.
exclude
list-like of dtypes or None (default), optional,
A black list of data types to omit from the result. Ignored for Series. Here are the options:
A list-like of dtypes : Excludes the provided data types from the result. To exclude numeric types submit numpy.number. To exclude object columns submit the data type numpy.object. Strings can also be used in the style of select_dtypes (e.g. df.describe(exclude=['O'])). To exclude pandas categorical columns, use 'category'
None (default) : The result will exclude nothing.
Returns:
Series or DataFrame
Summary statistics of the Series or Dataframe provided.
492-2、参数
492-2-1、percentiles**(可选,默认值为None)****:**列表,指定要计算的百分位数的列表,所有值应该在[0, 1]范围内。
492-2-2、include**(可选,默认值为None)****:**指定要包含的数据类型,可以是数据类型的字符串(如 'number', 'object', 'bool'),或者是数据类型的列表,如果该参数为None,将包含所有数据类型。
492-2-3、exclude**(可选,默认值为None)****:**指定要排除的数据类型,使用方法与include相同。
492-3、功能
用于生成DataFrame各列的描述性统计信息,它提供了数据的汇总,包括计数、均值、标准差、最小值、四分位数和最大值等,该方法对于快速了解数据分布非常有用。
492-4、返回值
返回一个DataFrame,其中包含描述性统计信息,对于数值型列,输出包括以下值:
- **count:**非空值的计数
- **mean:**平均值
- **std:**标准差
- **min:**最小值
- **25%:**第 25 百分位数
- **50%:**中位数
- **75%:**第 75 百分位数
- **max:**最大值
对于分类(object)类型的列,输出包括:
- **count:**非空值的计数
- **unique:**唯一值的数量
- **top:**出现频率最高的值
- freq: 最高频值的频率
492-5、说明
492-5-1、缺失值:该方法会自动忽略缺失值(NaN)进行统计。
492-5-2、数据类型:默认情况下,describe会根据数据类型自动选择要计算的列。
492-5-3、自定义百分位数:可以根据需求自定义百分位数,以便更好地理解数据分布。
492-6、用法
492-6-1、数据准备
python
无
492-6-2、代码示例
python
# 492、pandas.DataFrame.describe方法
import pandas as pd
# 创建一个示例DataFrame
data = {
'A': [1, 2, 3, 4, 5],
'B': [10, 20, 30, 40, 50],
'C': ['a', 'b', 'a', 'b', 'c']
}
df = pd.DataFrame(data)
# 计算描述性统计
description = df.describe()
print(description, end='\n\n')
# 包含特定百分位数
description_percentiles = df.describe(percentiles=[0.1, 0.5, 0.9])
print(description_percentiles, end='\n\n')
# 仅对数字列进行描述
description_numeric = df.describe(include=['number'])
print(description_numeric, end='\n\n')
# 仅对象列进行描述
description_object = df.describe(include=['object'])
print(description_object)
492-6-3、结果输出
python
# 492、pandas.DataFrame.describe方法
# A B
# count 5.000000 5.000000
# mean 3.000000 30.000000
# std 1.581139 15.811388
# min 1.000000 10.000000
# 25% 2.000000 20.000000
# 50% 3.000000 30.000000
# 75% 4.000000 40.000000
# max 5.000000 50.000000
#
# A B
# count 5.000000 5.000000
# mean 3.000000 30.000000
# std 1.581139 15.811388
# min 1.000000 10.000000
# 10% 1.400000 14.000000
# 50% 3.000000 30.000000
# 90% 4.600000 46.000000
# max 5.000000 50.000000
#
# A B
# count 5.000000 5.000000
# mean 3.000000 30.000000
# std 1.581139 15.811388
# min 1.000000 10.000000
# 25% 2.000000 20.000000
# 50% 3.000000 30.000000
# 75% 4.000000 40.000000
# max 5.000000 50.000000
#
# C
# count 5
# unique 3
# top a
# freq 2
493、pandas.DataFrame.diff方法
493-1、语法
python
# 493、pandas.DataFrame.diff方法
pandas.DataFrame.diff(periods=1, axis=0)
First discrete difference of element.
Calculates the difference of a DataFrame element compared with another element in the DataFrame (default is element in previous row).
Parameters:
periods
int, default 1
Periods to shift for calculating difference, accepts negative values.
axis
{0 or 'index', 1 or 'columns'}, default 0
Take difference over rows (0) or columns (1).
Returns:
DataFrame
First differences of the Series.
493-2、参数
493-2-1、periods**(可选,默认值为1)****:**整数,指定计算差异时的步长,默认是计算当前行与前一行的差异,可以设置为其他整数值,例如2,则计算当前行与前两行的差异。
493-2-2、axis**(可选,默认值为0)****:**{0或'index', 1或'columns'},指定沿着哪个方向计算差异,axis=0表示按行计算(纵向),axis=1表示按列计算(横向)。
493-3、功能
用于计算DataFrame中某一列值与前一个(或指定间隔的)值之间的差异,这对于时间序列数据分析非常有用,尤其是在需要了解数据如何变化时。
493-4、返回值
返回一个与原DataFrame同大小的DataFrame,包含每个元素的差异,其中第一个元素将会是NaN,因为没有前一个值可供计算。
493-5、说明
493-5-1、NaN 值:由于存在没有前一个值的情况,计算出的第一行/列会包含NaN值。
493-5-2、适用数据:该方法主要适用于数值型数据,对于其他类型的数据(如字符串)可能未按预期工作。
493-5-3、时间序列数据:在处理时间序列数据时,使用diff可以帮助了解数据的变化趋势。
493-6、用法
493-6-1、数据准备
python
无
493-6-2、代码示例
python
# 493、pandas.DataFrame.diff方法
import pandas as pd
# 创建一个示例DataFrame
data = {
'A': [10, 20, 30, 25, 15],
'B': [100, 200, 300, 250, 150],
}
df = pd.DataFrame(data)
# 计算差异(默认是前一行)
diff_default = df.diff()
print("默认差异:\n", diff_default)
# 计算与前两行的差异
diff_two_periods = df.diff(periods=2)
print("\n与前两行的差异:\n", diff_two_periods)
# 沿列方向计算差异
diff_axis1 = df.diff(axis=1)
print("\n沿列方向的差异:\n", diff_axis1)
493-6-3、结果输出
python
# 493、pandas.DataFrame.diff方法
# 默认差异:
# A B
# 0 NaN NaN
# 1 10.0 100.0
# 2 10.0 100.0
# 3 -5.0 -50.0
# 4 -10.0 -100.0
#
# 与前两行的差异:
# A B
# 0 NaN NaN
# 1 NaN NaN
# 2 20.0 200.0
# 3 5.0 50.0
# 4 -15.0 -150.0
#
# 沿列方向的差异:
# A B
# 0 NaN 90
# 1 NaN 180
# 2 NaN 270
# 3 NaN 225
# 4 NaN 135
494、pandas.DataFrame.eval方法
494-1、语法
python
# 494、pandas.DataFrame.eval方法
pandas.DataFrame.eval(expr, *, inplace=False, **kwargs)
Evaluate a string describing operations on DataFrame columns.
Operates on columns only, not specific rows or elements. This allows eval to run arbitrary code, which can make you vulnerable to code injection if you pass user input to this function.
Parameters:
expr
str
The expression string to evaluate.
inplace
bool, default False
If the expression contains an assignment, whether to perform the operation inplace and mutate the existing DataFrame. Otherwise, a new DataFrame is returned.
**kwargs
See the documentation for eval() for complete details on the keyword arguments accepted by query().
Returns:
ndarray, scalar, pandas object, or None
The result of the evaluation or None if inplace=True.
494-2、参数
494-2-1、expr**(必须)****:**字符串,要评估的表达式,该表达式可以使用DataFrame的列名,支持各种运算符,如加法、减法、乘法和除法。
494-2-2、inplace**(可选,默认值为False)****:**布尔值,如果为True,则在原DataFrame上进行修改,而不是返回一个新的DataFrame。
494-2-3、**kwargs**(可选)****:**额外的关键字参数,可以用于传递其他变量和参数。
494-3、功能
用于在DataFrame上评估一个表达式,并返回一个新的DataFrame或对象,该方法能够利用numexpr(如果可用的话)来加速计算,适合处理大型数据集的情况。
494-4、返回值
返回一个新的DataFrame(如果inplace=False)或原DataFrame(如果inplace=True),表达式计算的结果。
494-5、说明
494-5-1、表达式限制:在表达式中只允许使用DataFrame的列名,表达式不能使用Python语言中的特殊功能。
494-5-2、运算符支持:支持基本的算术运算符和一些常见的数学函数。
494-5-3、效率 :eval方法在处理大型DataFrame时通常比逐列计算要快,尤其是在numexpr
可用的情况下。
494-6、用法
494-6-1、数据准备
python
无
494-6-2、代码示例
python
# 494、pandas.DataFrame.eval方法
import pandas as pd
# 创建示例DataFrame
data = {
'A': [1, 2, 3],
'B': [4, 5, 6],
}
df = pd.DataFrame(data)
# 使用eval计算新列C = A + B
df['C'] = df.eval('A + B')
print("新列C:\n", df)
# 或者在原地修改
df.eval('D = A * B', inplace=True)
print("\n新增列D,原地修改后:\n", df)
# 使用额外的变量
factor = 10
df['E'] = df.eval('A + B + @factor')
print("\n使用额外变量后的列E:\n", df)
494-6-3、结果输出
python
# 494、pandas.DataFrame.eval方法
# 新列C:
# A B C
# 0 1 4 5
# 1 2 5 7
# 2 3 6 9
#
# 新增列D,原地修改后:
# A B C D
# 0 1 4 5 4
# 1 2 5 7 10
# 2 3 6 9 18
#
# 使用额外变量后的列E:
# A B C D E
# 0 1 4 5 4 15
# 1 2 5 7 10 17
# 2 3 6 9 18 19
495、pandas.DataFrame.kurt方法
495-1、语法
python
# 495、pandas.DataFrame.kurt方法
pandas.DataFrame.kurt(axis=0, skipna=True, numeric_only=False, **kwargs)
Return unbiased kurtosis over requested axis.
Kurtosis obtained using Fisher's definition of kurtosis (kurtosis of normal == 0.0). Normalized by N-1.
Parameters:
axis{index (0), columns (1)}
Axis for the function to be applied on. For Series this parameter is unused and defaults to 0.
For DataFrames, specifying axis=None will apply the aggregation across both axes.
New in version 2.0.0.
skipnabool, default True
Exclude NA/null values when computing the result.
numeric_onlybool, default False
Include only float, int, boolean columns. Not implemented for Series.
**kwargs
Additional keyword arguments to be passed to the function.
Returns:
Series or scalar.
495-2、参数
495-2-1、axis**(可选,默认值为0)****:**{0或'index', 1或'columns'},计算峭度的轴,0或'index'表示按列计算峭度,1或'columns'表示按行计算峭度。
495-2-2、skipna**(可选,默认值为True)****:**布尔值,是否忽略缺失值(NaNs),如果设置为False,将会计算缺失值。
495-2-3、numeric_only**(可选,默认值为False)****:**布尔值,如果设置为True,只计算数值列的峭度,其他类型的列将会被排除。
495-2-4、**kwargs**(可选)****:**额外的关键字参数,供底层函数使用。
495-3、功能
用于计算DataFrame中每一列的峭度(Kurtosis),峭度是描述概率分布形状的一种统计量,主要用于评估分布的尾部厚度。
495-4、返回值
返回一个Series或DataFrame,包含每一列或每一行的峭度值。
495-5、说明
495-5-1、数值类型:峭度计算仅适用于数值类型的数据,非数值类型的数据将被忽略,或者如numeric_only=True,将会被排除。
495-5-2、缺失值:如果数据中存在缺失值,根据skipna参数的设置,峭度可能会受到影响。
495-5-3、统计性质:峭度值可以用于判断数据的分布特征:较高的峭度值表示分布的尾部较重,较低的峭度值表示分布的尾部较轻。
495-6、用法
495-6-1、数据准备
python
无
495-6-2、代码示例
python
# 495、pandas.DataFrame.kurt方法
import pandas as pd
# 创建示例DataFrame
data = {
'A': [4, 5, 8, 9],
'B': [1, 2, None, 4],
'C': [1, 1, 1, 1]
}
df = pd.DataFrame(data)
# 计算每列的峭度
kurtosis = df.kurt()
print("每列的峭度:\n", kurtosis)
# 计算每行的峭度
kurtosis_axis1 = df.kurt(axis=1)
print("每行的峭度:\n", kurtosis_axis1)
495-6-3、结果输出
python
# 495、pandas.DataFrame.kurt方法
# 每列的峭度:
# A -4.3391
# B NaN
# C 0.0000
# dtype: float64
# 每行的峭度:
# 0 NaN
# 1 NaN
# 2 NaN
# 3 NaN
# dtype: float64