cs
#define MAXSIZE 255
typedef struct
{
char ch[MAXSIZE + 1];
int length;
}SString;
typedef struct
{
char result[MAXSIZE + 1];
}Detection;
int Index_BF(SString Person, SString temp)
{
int i = 0, j = 0;
while (i<Person.length && j<temp.length)
{
if (Person.ch[i] == temp.ch[j])
{
i++;
j++;
}
else
{
i = i - j + 1;
j = 0;
}
}
if (j >= temp.length)
{
return 1;
}
else {
return 0;
}
}
void Virus_detection(SString S[], SString T[], int num, Detection detection[])
{
SString Person, Virus, temp;
int k = 0, i, j, flag, m;
while (num--)
{
Person = S[k];
Virus = T[k];
flag = 0;
m = Virus.length;
//把病毒DNA扩大两倍
for (i = m,j = 0; j < m; j++)
{
Virus.ch[i++] = Virus.ch[j];
}
//处理病毒与人体dna
Virus.ch[2 * m] = '\0';
for (i = 0; i < m; i++)
{
for (j = 0; j < m; j++)
{
temp.ch[j] = Virus.ch[i + j];
}
temp.ch[m] = '\0';
temp.length = m;
flag = Index_BF(Person, temp);
if (flag == 1)
{
break;
}
}
if (flag == 1)
{
strcpy(detection[k].result, "YES");
}
else {
strcpy(detection[k].result, "NO");
}
k++;
}
}
int main()
{
Detection detection[10];
SString S[10] =
{
{"bbaabbba",8},
{"aaabbbba",8},
{"abceaabb",8},
{"abaabcea",8},
{"cdabbbab",8},
{"cabbbbab",8},
{"bcdedbda",8},
{"bdedbcda",8},
{"cdcdcdec",8},
{"cdccdcce",8}
};
SString T[10] =
{
{"baa" ,3},
{"baa" ,3},
{"aabb" ,4},
{"aabb" ,4},
{"abcd" ,4},
{"abcd" ,4},
{"abcde",5},
{"acc" ,3},
{"cde" ,3},
{"cced" ,4}
};
int num = 10, i;
Virus_detection(S, T, num, detection);
printf("病毒\t人体DNA\t 结果\n");
for (i = 0; i < num; i++)
{
printf("%s \t%s %s\n", T[i].ch, S[i].ch, detection[i].result);
}
return 0;
}
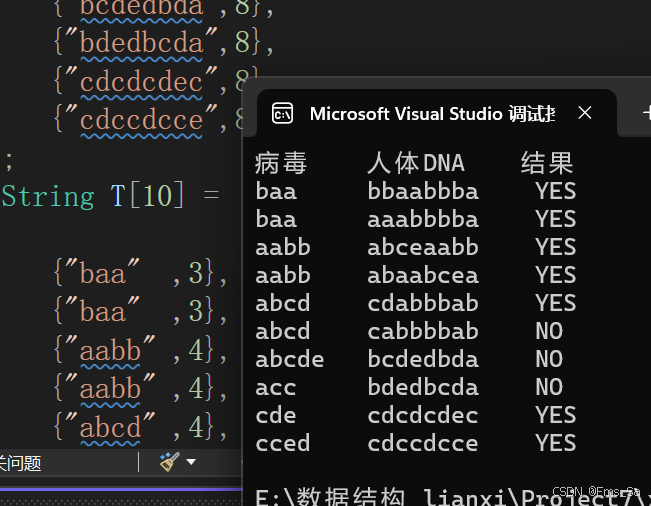
2.下面是可以访问文本文件读取文件内容,然后判断完YES 或 NO后再生成的一个新的结果文本文件代码
cs
#include <stdio.h>
#include <stdlib.h>
typedef struct {
char ch[600];
int len;
} HString;
int Index_BF(HString S, HString T, int pos) {
int i = pos, j = 1;
while (i <= S.len && j <= T.len) {
if (S.ch[i] == T.ch[j]) {
i++;
j++;
} else {
i = i - j + 2;
j = 1;
}
}
if (j > T.len) {
return i - T.len;
} else {
return 0;
}
}
void Virus_detection() {
int num, m, flag, i, j;
char Vir[600];
HString Virus, Person, temp;
FILE *inFile = fopen("病毒感染检测输入数据.txt", "r");
FILE *outFile = fopen("病毒感染检测输出结果.txt", "w");
if (inFile == NULL || outFile == NULL) {
printf("Error opening files\n");
return;
}
fscanf(inFile, "%d", &num);
while (num--) {
fscanf(inFile, "%s", Virus.ch + 1);
fscanf(inFile, "%s", Person.ch + 1);
strcpy(Vir, Virus.ch);
Virus.len = strlen(Virus.ch) - 1;
Person.len = strlen(Person.ch) - 1;
flag = 0;
m = Virus.len;
for (i = m + 1, j = 1; j <= m; j++) {
Virus.ch[i++] = Virus.ch[j];
}
Virus.ch[2 * m + 1] = '\0';
for (i = 0; i < m; i++) {
for (j = 1; j <= m; j++) {
temp.ch[j] = Virus.ch[i + j];
}
temp.ch[m + 1] = '\0';
temp.len = strlen(temp.ch) - 1;
flag = Index_BF(Person, temp, 1);
if (flag) {
break;
}
}
if (flag) {
fprintf(outFile, "%s\t%s\tYES\n", Vir + 1, Person.ch + 1);
} else {
fprintf(outFile, "%s\t%s\tNO\n", Vir + 1, Person.ch + 1);
}
}
fclose(inFile);
fclose(outFile);
}
int main() {
Virus_detection();
return 0;
}
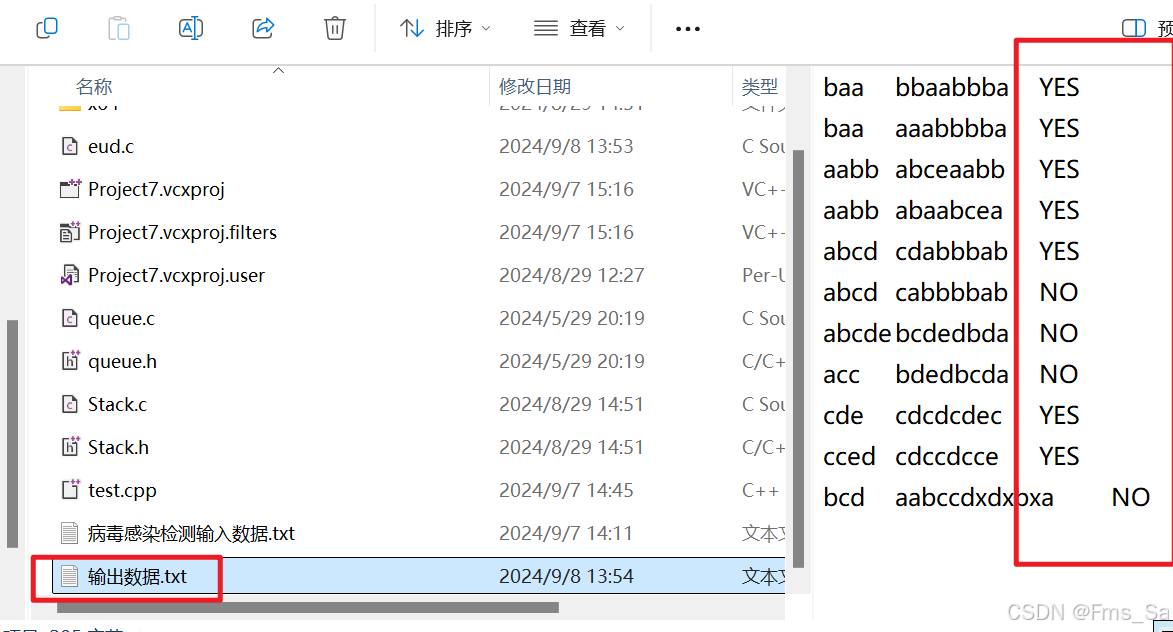
其中病毒感染检测输入数据的内容如下:
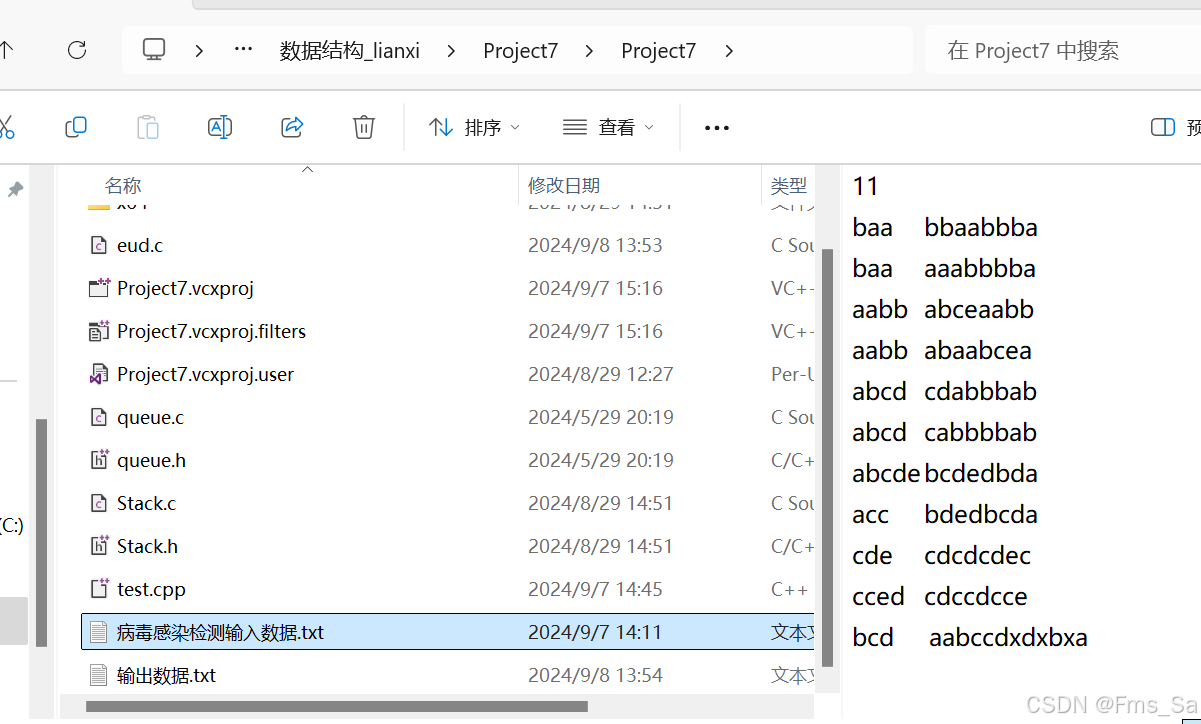
cs
11
baa bbaabbba
baa aaabbbba
aabb abceaabb
aabb abaabcea
abcd cdabbbab
abcd cabbbbab
abcde bcdedbda
acc bdedbcda
cde cdcdcdec
cced cdccdcce
bcd aabccdxdxbxa
读者可以自行创建笔记本,将该笔记本放在自己目标代码的目录下,即可