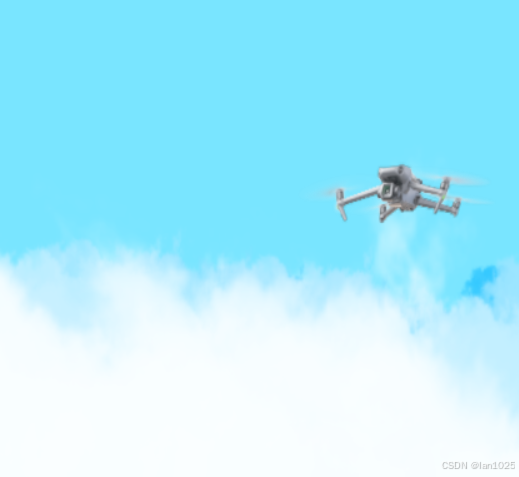
效果图如上
代码:
javascript
<template>
<div class="drones">
<div ref="dronesContainer" class="drones-container"></div>
</div>
</template>
<script setup>
import { ref, onMounted, onUnmounted, render } from 'vue';
import * as THREE from 'three';
// 导入无人机材质
import droneTexture from '../../../assets/images/大疆无人机.png';
const dronesContainer = ref(null);
let scene, camera, renderer, droneMesh, droneGeometry, droneMaterial, animationId;
onMounted(async () => {
try {
await init();
animate();
} catch (error) {
console.error('Initialization error:', error);
}
});
onUnmounted(() => {
cancelAnimationFrame(animationId);
});
async function init() {
const width = window.innerWidth;
const height = window.innerHeight;
// 创建场景
scene = new THREE.Scene();
scene.background = null;
// 创建相机
camera = new THREE.PerspectiveCamera(75, width / height, 0.1, 1000);
camera.position.z = 5;
// 创建渲染器,antialias: true 表示开启抗锯齿
renderer = new THREE.WebGLRenderer({ antialias: true, alpha: true });
// 利用渲染器将场景的透明度设置为0
renderer.setClearAlpha(0);
renderer.setSize(width, height);
dronesContainer.value.appendChild(renderer.domElement);
// 添加光源, AmbientLight 表示环境光,DirectionalLight 表示平行光
const light = new THREE.AmbientLight(0x404040);
scene.add(light);
const directionalLight = new THREE.DirectionalLight(0xffffff, 1);
directionalLight.position.set(-1, 2, 4).normalize();
scene.add(directionalLight);
const texture = new THREE.TextureLoader().load(droneTexture);
// 创建无人机面容器
droneGeometry = new THREE.PlaneGeometry(2, 2);
// 创建无人机材质
droneMaterial = new THREE.MeshBasicMaterial({ map: texture, transparent: true});
// texture.transparent = true;
droneMesh = new THREE.Mesh(droneGeometry, droneMaterial);
droneMesh.position.set(0.5, 1, 0);
scene.add(droneMesh);
renderer.render(scene, camera);
}
const animate = () => {
animationId = requestAnimationFrame(animate);
const positionY = Math.abs(Math.sin(Date.now() * 0.001)) * 1.5;
const rotationX = Math.cos(Date.now() * 0.001) * Math.PI / 3;
droneMesh.rotation.x = rotationX;
droneMesh.position.y = positionY;
renderer.render(scene, camera);
};
window.addEventListener('resize', () => {
const width = window.innerWidth;
const height = window.innerHeight;
renderer.setSize(width, height);
camera.aspect = width / height;
camera.updateProjectionMatrix();
});
</script>
<style scoped>
.drones-container {
width: 100%;
height: 100vh;
position: relative;
overflow: hidden;
z-index: 1000;
}
</style>
说明:
由于材质是二维图片挂载到二维平面,所以无人机的所谓上下翻转运动,是面的绕轴运动和上下运动的复合运动(绕x轴),其次注意png图片作为材质,让透明地方不为黑色,一定要设置MeshBasicMaterial的transparent为true,即透明度使用。