思维导图
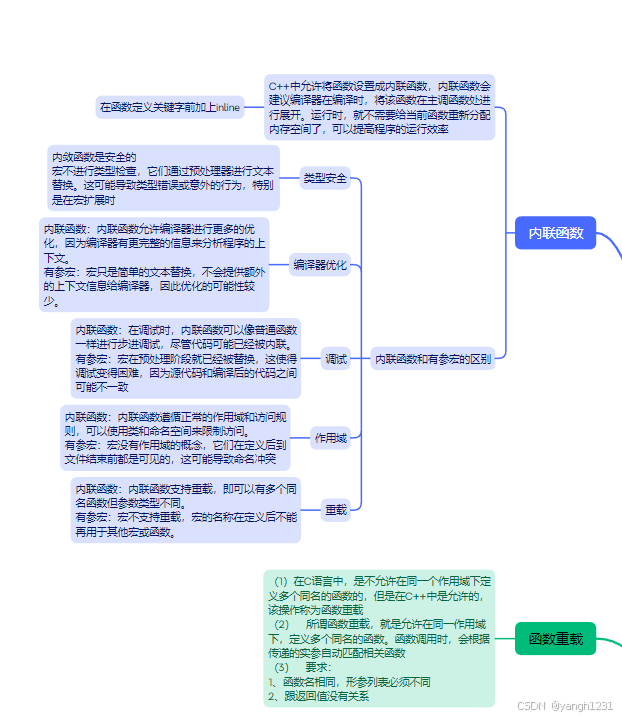
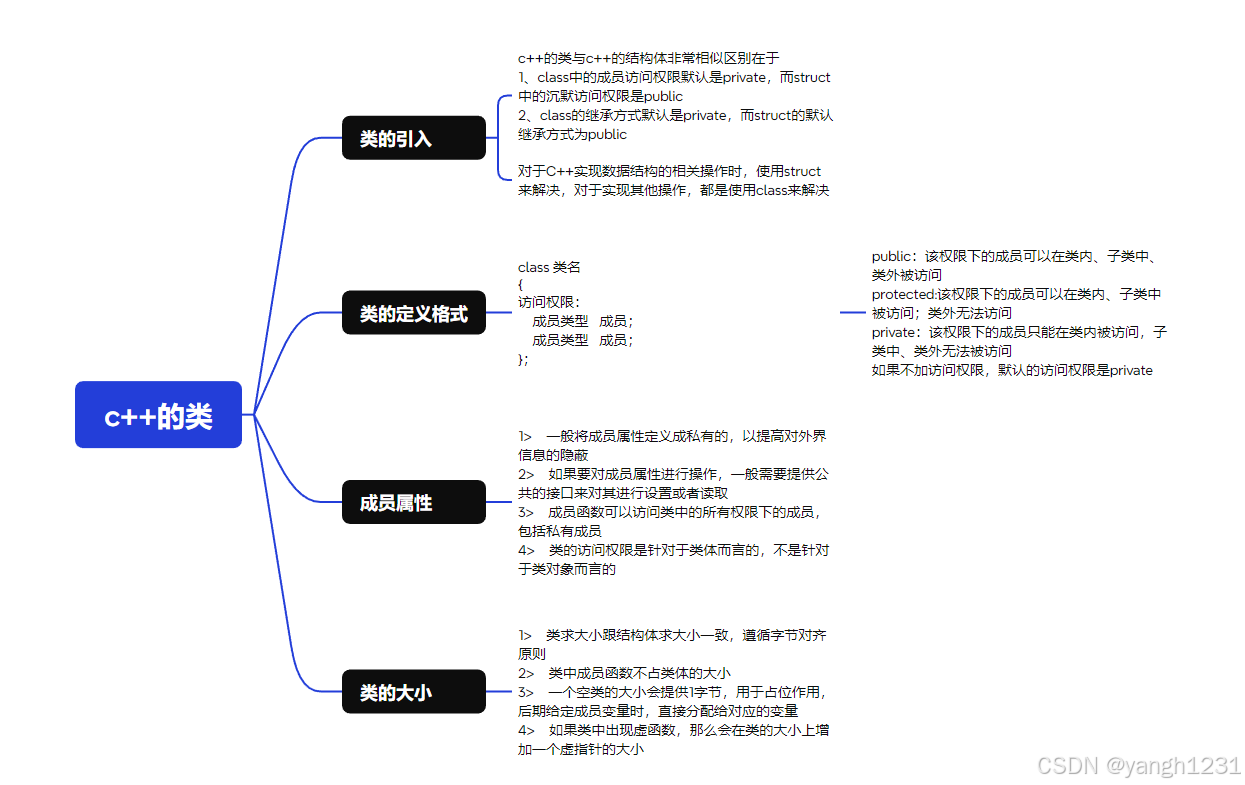
头文件
cpp
#ifndef SEQLIST_H
#define SEQLIST_H
using datatype = int;
class seqlist
{
private:
datatype *ptr; // 动态数组指针
int size; // 顺序表最大容量
int len = 0; // 当前长度
public:
void init(int n); // 初始化顺序表
bool empty(); // 判断是否为空
bool full(); // 判断是否已满
void push_back(datatype e); // 在顺序表末尾添加元素
void show(); // 显示顺序表中的元素
void insert(int n, datatype e); // 在指定位置插入元素
void erase(int n); // 删除指定位置的元素
void pop_back(); // 删除末尾元素
void getany(int n); // 获取任意位置的元素
void sort(int n); // 排序,输入1为升序,输入0为降序
};
#endif
源文件
cpp
#include "seqlist.h"
#include <iostream>
using namespace std;
// 初始化顺序表
void seqlist::init(int n)
{
this->ptr = new datatype[n];
this->len = 0;
this->size = n;
}
// 判断是否为空
bool seqlist::empty()
{
return this->len == 0;
}
// 判断是否已满
bool seqlist::full()
{
return this->len == this->size;
}
// 在顺序表末尾添加元素
void seqlist::push_back(datatype e)
{
if (this->full())
{
return;
}
else
{
this->ptr[this->len++] = e;
}
}
// 显示顺序表中的元素
void seqlist::show()
{
cout << "当前顺序表的元素是: ";
for (int i = 0; i < this->len; i++)
{
cout << this->ptr[i] << " ";
}
cout << endl;
}
// 在指定位置插入元素
void seqlist::insert(int n, datatype e)
{
if (this->full())
{
return;
}
else
{
for (int i = this->len; i >= n; i--)
{
this->ptr[i] = this->ptr[i - 1];
}
this->ptr[n - 1] = e;
this->len++;
}
}
// 删除指定位置的元素
void seqlist::erase(int n)
{
if (this->empty())
{
return;
}
else
{
for (int i = n - 1; i < this->len - 1; i++)
{
this->ptr[i] = this->ptr[i + 1];
}
this->len--;
}
}
// 删除末尾元素
void seqlist::pop_back()
{
if (this->empty())
{
return;
}
else
{
this->ptr[this->len--] = 0;
}
}
// 获取任意位置的元素
void seqlist::getany(int n)
{
if (n > 0 && n <= this->len)
{
cout << "位置 " << n << " 的元素是: " << this->ptr[n - 1] << endl;
}
else
{
cout << "位置无效" << endl;
}
}
// 排序,输入1为升序,输入0为降序
void seqlist::sort(int n)
{
if (n == 1) // 升序排序
{
for (int i = 0; i < this->len - 1; i++)
{
for (int j = 0; j < this->len - i - 1; j++)
{
if (this->ptr[j] > this->ptr[j + 1])
{
swap(this->ptr[j], this->ptr[j + 1]);
}
}
}
}
else if (n == 0) // 降序排序
{
for (int i = 0; i < this->len - 1; i++)
{
for (int j = 0; j < this->len - i - 1; j++)
{
if (this->ptr[j] < this->ptr[j + 1])
{
swap(this->ptr[j], this->ptr[j + 1]);
}
}
}
}
}
主函数
cpp
#include "seqlist.h"
#include <iostream>
using namespace std;
int main()
{
seqlist s1;
s1.init(8); // 初始化顺序表,容量为5
s1.push_back(1);
s1.push_back(2);
s1.push_back(3);
s1.push_back(5);
s1.push_back(6);
s1.push_back(7);
s1.show(); // 显示顺序表
s1.insert(2, 4); // 在第2个位置插入元素4
s1.show(); // 显示顺序表
s1.erase(2); // 删除第2个位置的元素
s1.show(); // 显示顺序表
s1.pop_back(); // 删除末尾元素
s1.show(); // 显示顺序表
s1.getany(2); // 获取第2个位置的元素
s1.sort(1); // 升序排序
s1.show(); // 显示顺序表
s1.sort(0); // 降序排序
s1.show(); // 显示顺序表
return 0;
}