目录
1.加法计算器
(1)需求分析
加法计算器功能, 对两个整数进⾏相加, 需要客⼾端提供参与计算的两个数, 服务端返回这两个整数计算 的结果。
(2)接⼝定义
请求路径: calc/sum
请求⽅式: GET/POST
接⼝描述:计算两个整数相加
(3)请求参数
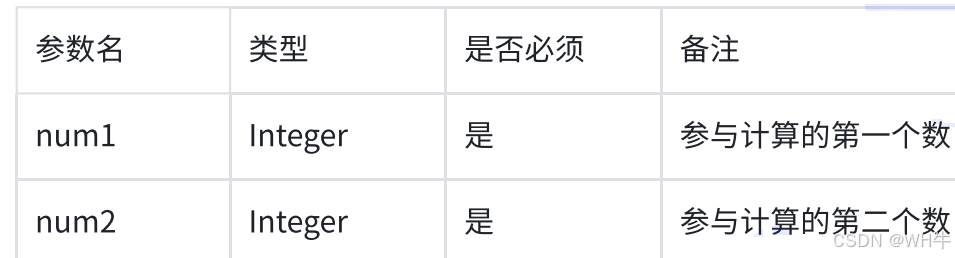
服务器给浏览器返回计算的结果
(4)前端⻚⾯代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="calc/sum" method="post">
<h1>计算器</h1>
数字1:<input name="num1" type="text"><br>
数字2:<input name="num2" type="text"><br>
<input type="submit" value=" 点击相加 ">
</form>
</body>
</html>
(5)服务器代码
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RequestMapping("/calc")
@RestController
public class CalcController {
@RequestMapping("/sum")
public String sum(Integer num1, Integer num2){
Integer sum = num1+num2;
return "计算器计算结果:"+ sum;
}
}
(6)运⾏测试
启动服务, 运⾏并测试

2.⽤户登录
(1)需求分析
需求: ⽤户输⼊账号和密码, 后端进⾏校验密码是否正确。
- 如果不正确, 前端进⾏⽤户告知
2.如果正确, 跳转到⾸⻚. ⾸⻚显示当前登录⽤户
3.后续再访问⾸⻚, 可以获取到登录⽤户信息
(2)接⼝定义
校验接⼝
1.请求路径: /user/login
2.请求⽅式: POST
3.接⼝描述:校验账号密码是否正确
查询登录⽤户接⼝
1.请求路径:/user/index
2.请求⽅式:GET
3.接⼝描述:查询当前登录的⽤⼾
(3)请求参数
校验接⼝
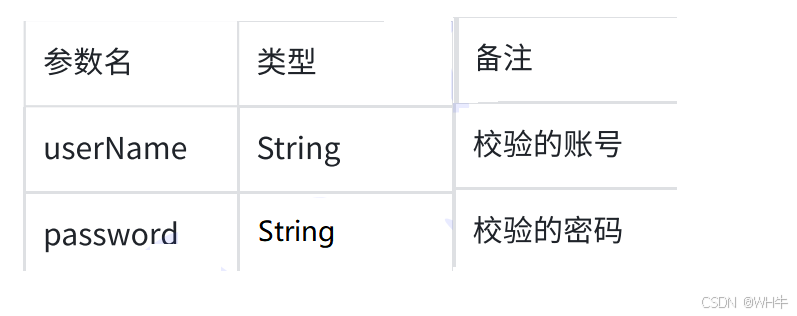
查询登录⽤户接⼝
请求参数: ⽆
(4)前端⻚⾯代码
校验接⼝
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>登录页面</title>
</head>
<body>
<h1>用户登录</h1>
用户名:<input name="userName" type="text" id="userName"><br>
密码:<input name="password" type="password" id="password"><br>
<input type="button" value="登录" onclick="login()">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script>
function login() {
$.ajax({
url: "/user/login",
type: "post",
data: {
userName: $("#userName").val(),
password: $("#password").val()
},
success: function (result) {
if (result == true) {
location.href = "index.html";
} else {
alert("输入错误");
}
}
});
}
</script>
</body>
</html>
查询登录⽤户接⼝
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>用户登录首页</title>
</head>
<body>
登录人: <span id="loginUser"></span>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script>
$.ajax({
url:"/user/index",
type:"post",
success:function(loginName){
$("#loginUser").text(loginName);
}
});
</script>
</body>
</html>
(5)服务器代码
import jakarta.servlet.http.HttpSession;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.SessionAttribute;
@RequestMapping("/user")
@RestController
public class UserController {
@RequestMapping("/login")
public Boolean login(String userName, String password, HttpSession session){
//参数校验
// if (userName==null || userName.length()==0
// || password ==null || password.length()==0){
// return false;
// }
if (!StringUtils.hasLength(userName) || !StringUtils.hasLength(password)){
return false;
}
//判断密码是否正确
//上面已经做了判空的处理, userName不会为null, 这是一种习惯
if ("admin".equals(userName) && "admin".equals(password)){
//设置session
session.setAttribute("userName", userName);
return true;
}
return false;
}
@RequestMapping("/index")
public String getUserName(@SessionAttribute("userName") String userName){
return userName;
}
}
(6)运⾏测试
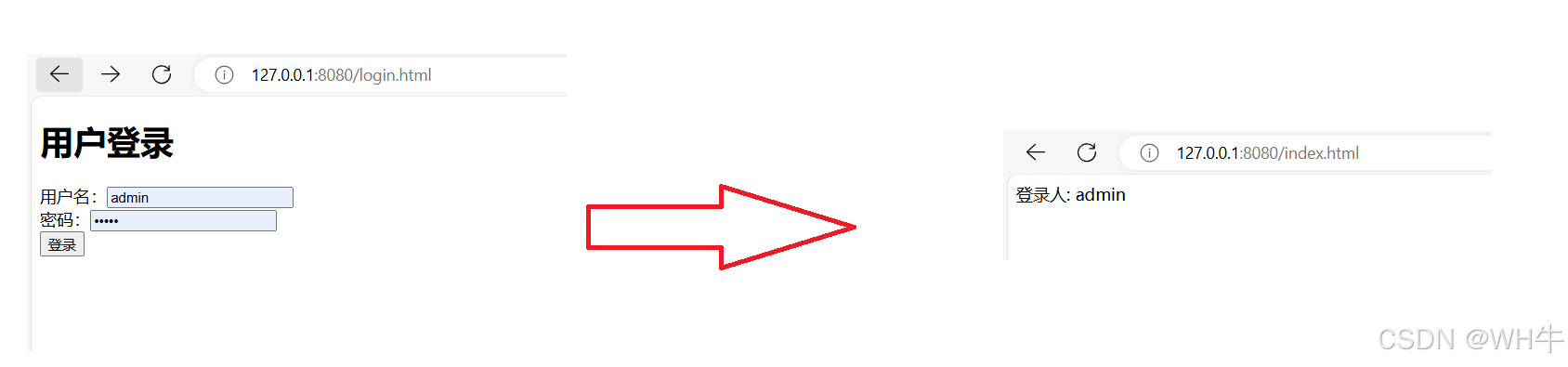
3.留⾔板
(1)需求分析
后端需要提供两个服务
- 提交留⾔: ⽤户输⼊留⾔信息之后, 后端需要把留⾔信息保存起来
2.展示 留⾔: ⻚⾯展示时, 需要从后端获取到所有的留⾔信息
(2)接⼝定义
提交留⾔
1.请求路径:/message/publish
2.请求⽅式:post
展示留⾔
1.请求路径:/message/getList
2.请求⽅式:get
(3)请求参数
提交留⾔
请求参数为MessageInfo对象
import lombok.Data;
@Data
public class MessageInfo {
private String from;
private String to;
private String say;
}
展示留⾔
请求参数: ⽆
(4)前端⻚⾯代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>留言板</title>
<style>
.container {
width: 350px;
height: 300px;
margin: 0 auto;
/* border: 1px black solid; */
text-align: center;
}
.grey {
color: grey;
}
.container .row {
width: 350px;
height: 40px;
display: flex;
justify-content: space-between;
align-items: center;
}
.container .row input {
width: 260px;
height: 30px;
}
#submit {
width: 350px;
height: 40px;
background-color: orange;
color: white;
border: none;
margin: 10px;
border-radius: 5px;
font-size: 20px;
}
</style>
</head>
<body>
<div class="container">
<h1>留言板</h1>
<p class="grey">输入后点击提交, 会将信息显示下方空白处</p>
<div class="row">
<span>谁:</span> <input type="text" name="" id="from">
</div>
<div class="row">
<span>对谁:</span> <input type="text" name="" id="to">
</div>
<div class="row">
<span>说什么:</span> <input type="text" name="" id="say">
</div>
<input type="button" value="提交" id="submit" onclick="submit()">
<!-- <div>A 对 B 说: hello</div> -->
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script>
$.ajax({
url: "/message/getList",
type: "get",
success: function (messages) {
var finalHtml = '';
for (var message of messages) {
finalHtml += '<div>' + message.from + '对' + message.to + '说:' + message.say + '</div>';
}
$(".container").append(finalHtml);
}
});
function submit() {
//1. 获取留言的内容
var from = $('#from').val();
var to = $('#to').val();
var say = $('#say').val();
if (from == '' || to == '' || say == '') {
return;
}
$.ajax({
url: "/message/publish",
type: "post",
data: {
from: $('#from').val(),
to: $('#to').val(),
say: $('#say').val()
},
success: function (result) {
if (result) {
//2. 构造节点
var divE = "<div>" + from + "对" + to + "说:" + say + "</div>";
//3. 把节点添加到页面上
$(".container").append(divE);
//4. 清空输入框的值
$('#from').val("");
$('#to').val("");
$('#say').val("");
} else {
alert("输入不合法");
}
}
});
}
</script>
</body>
</html>
(5)服务器代码
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
@RequestMapping("message")
@RestController
public class MessageController {
ArrayList<MessageInfo> arrayList = new ArrayList<>();
@RequestMapping("publish")
public boolean publish(MessageInfo message) {
if (!StringUtils.hasLength(message.getFrom()) ||
!StringUtils.hasLength(message.getSay()) ||
!StringUtils.hasLength(message.getFrom())) {
return false;
}
arrayList.add(message);
return true;
}
@RequestMapping("/getList")
public ArrayList getList() {
return arrayList;
}
}
(6)运⾏测试
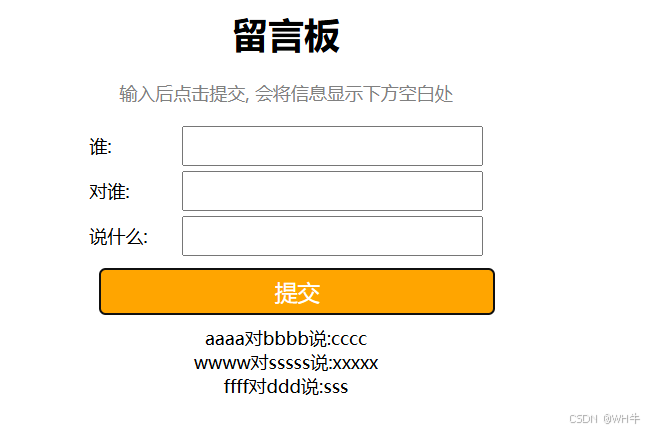
以上为我个人的小分享,如有问题,欢迎讨论!!!
都看到这了,不如关注一下,给个免费的赞
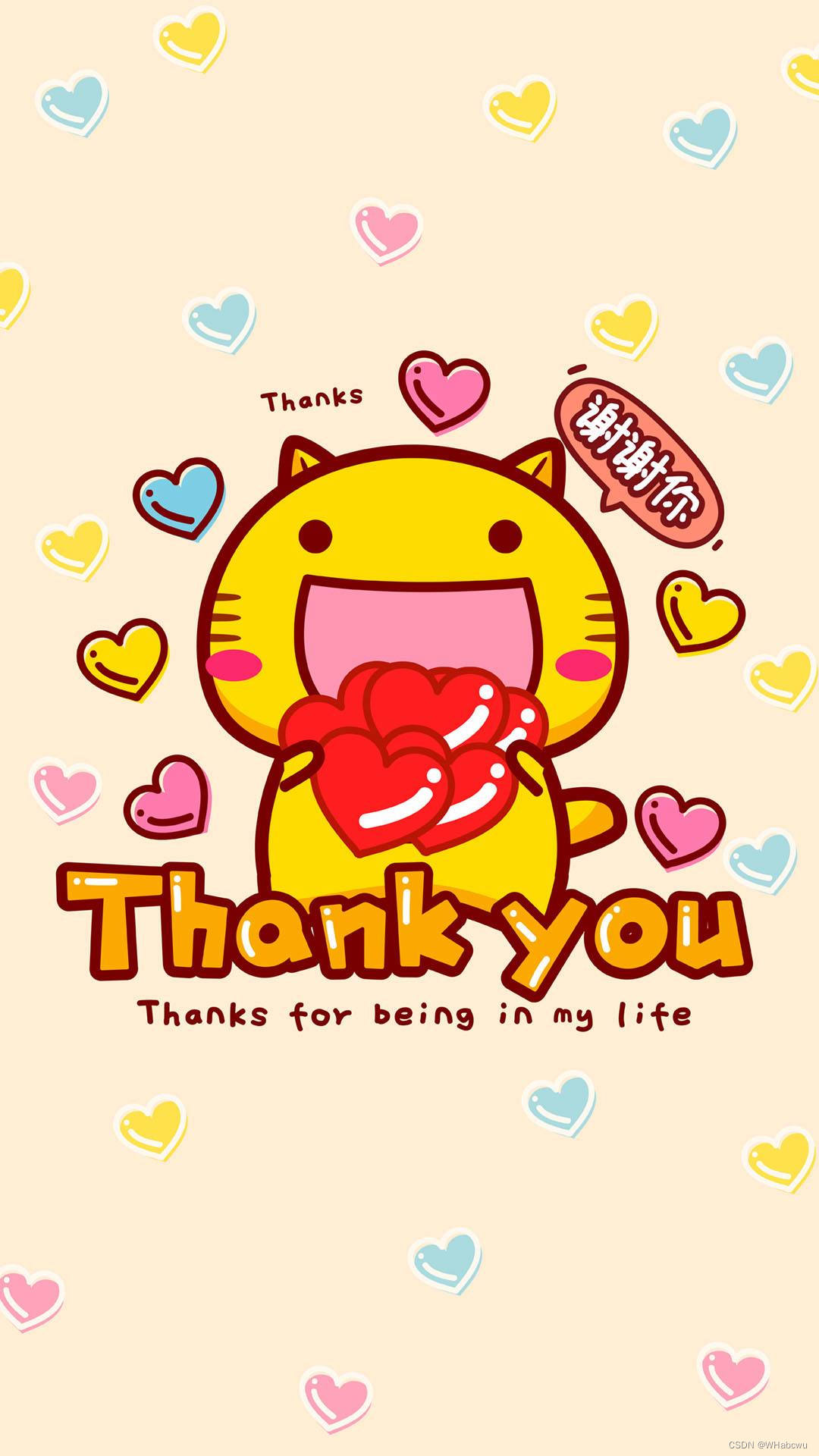