在 Go 语言中实现随机森林(Random Forest)算法通常涉及以下几个步骤:
-
数据准备:将数据集分为训练集和测试集,确保数据格式适合算法使用。
-
决策树的构建:随机森林是由多个决策树构成的,首先需要实现一个单独的决策树。
-
随机抽样:从训练数据中随机抽样生成多个子集,分别用来训练每棵树。
-
投票机制:对于分类问题,通过各树的投票决定最终的预测结果;对于回归问题,计算各树预测值的平均值。
以下是一个简化的随机森林实现示例,主要关注结构和逻辑:
package main
import (
"fmt"
"math/rand"
)
type DecisionTree struct {
// 决策树相关参数
// ...
}
type RandomForest struct {
trees []*DecisionTree
n int // 树的数量
}
func (rf *RandomForest) Train(data [][]float64, labels []int) {
for i := 0; i < rf.n; i++ {
// 随机抽样
sampleData, sampleLabels := bootstrapSample(data, labels)
tree := &DecisionTree{}
tree.Train(sampleData, sampleLabels)
rf.trees = append(rf.trees, tree)
}
}
func (rf *RandomForest) Predict(input []float64) int {
votes := make(map[int]int)
for _, tree := range rf.trees {
prediction := tree.Predict(input)
votes[prediction]++
}
// 投票机制
var maxVote int
var result int
for label, vote := range votes {
if vote > maxVote {
maxVote = vote
result = label
}
}
return result
}
func bootstrapSample(data [][]float64, labels []int) ([][]float64, []int) {
n := len(data)
sampleData := make([][]float64, n)
sampleLabels := make([]int, n)
for i := 0; i < n; i++ {
index := rand.Intn(n)
sampleData[i] = data[index]
sampleLabels[i] = labels[index]
}
return sampleData, sampleLabels
}
func (tree *DecisionTree) Train(data [][]float64, labels []int) {
// 实现决策树训练逻辑
}
func (tree *DecisionTree) Predict(input []float64) int {
// 实现决策树预测逻辑
return 0 // 返回分类结果
}
func main() {
rand.Seed(42) // 设置随机种子
data := [][]float64{
{1.0, 2.0},
{2.0, 3.0},
{3.0, 4.0},
// 添加更多数据
}
labels := []int{0, 1, 0} // 示例标签
rf := &RandomForest{n: 10} // 10棵树
rf.Train(data, labels)
input := []float64{2.5, 3.5}
prediction := rf.Predict(input)
fmt.Println("预测结果:", prediction)
}
注意事项
- 上述示例代码是一个简化版,实际的决策树实现需要更复杂的逻辑,比如选择最佳分裂点、处理连续和离散特征等。
- 需要引入更多的错误处理和性能优化。
- 使用随机森林的库(如 GoML 等)可以提高效率和可靠性。
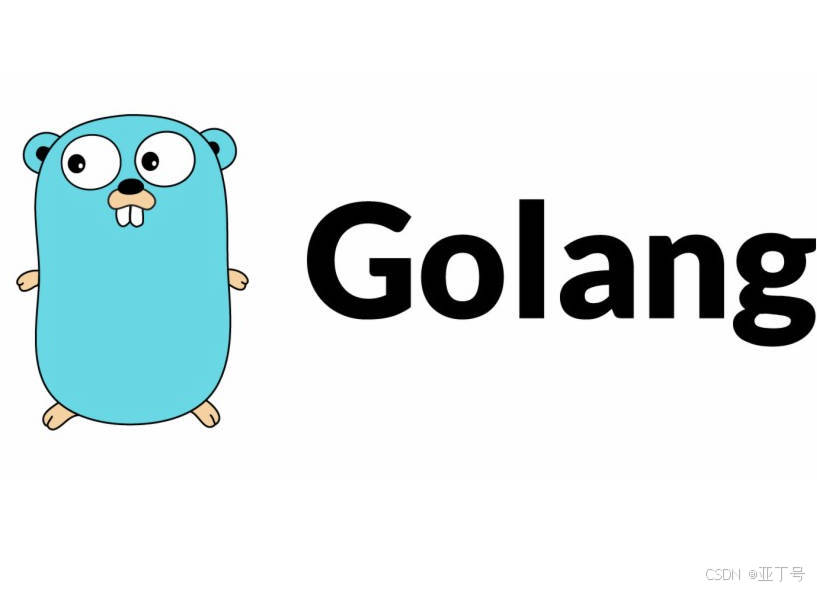