//
// Created by 徐昌真 on 2024/10/5.
//
#include <iostream>
using namespace std;
//定义一个结构体
struct Circle{
int x, y, r;
};
//定义一个可以修改结构体内容的函数
void movePoint(Circle c, int x, int y){
c.x += x;
c.y += y;
}
//定义一个可以输出结构体内容的函数
void printCircle(Circle c){
cout << '(' << c.x << ',' << c.y << ") " << c.r << endl;
}
int main() {
//创建一个结构体
Circle c = {1,2,3};
//调用修改结构体的函数
movePoint(c,1,2);
//调用输出结构体的函数
printCircle(c);
return 0;
}
我们这样子写完之后 会发现修改的结果并没有生效 这是因为值的改变只在函数的内部 并没有在main函数内部改变 我们需要用指针 在地址里面修改值 才可以
这是输出结果
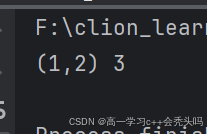
这是修改后的代码
//
// Created by 徐昌真 on 2024/10/5.
//
#include <iostream>
using namespace std;
//定义一个结构体
struct Circle{
int x, y, r;
};
//定义一个可以修改结构体内容的函数
void movePoint(Circle* c, int x, int y){
c->x += x;
c->y += y;
}
//定义一个可以输出结构体内容的函数
void printCircle(Circle c){
cout << '(' << c.x << ',' << c.y << ") " << c.r << endl;
}
int main() {
//创建一个结构体
Circle d = {1,2,3};
//调用修改结构体的函数
movePoint(&d,1,2);
//调用输出结构体的函数
printCircle(d);
return 0;
}
我们只需传入指针类型的数据即可
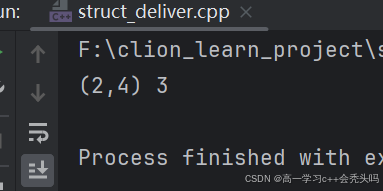