目录
- [(一)练习常用的HBase Shell命令](#(一)练习常用的HBase Shell命令)
- [(二)HBase 常用的Java API 及应用实例](#(二)HBase 常用的Java API 及应用实例)
-
- 1、启动hbase服务
- 2、启动eclipse
- [3、新建java project](#3、新建java project)
- [4、关闭 hbase](#4、关闭 hbase)
(一)练习常用的HBase Shell命令
1、启动HBase
先启动HDFS 再启动HBase
进入shell交互式执行环境
2、练习shell命令
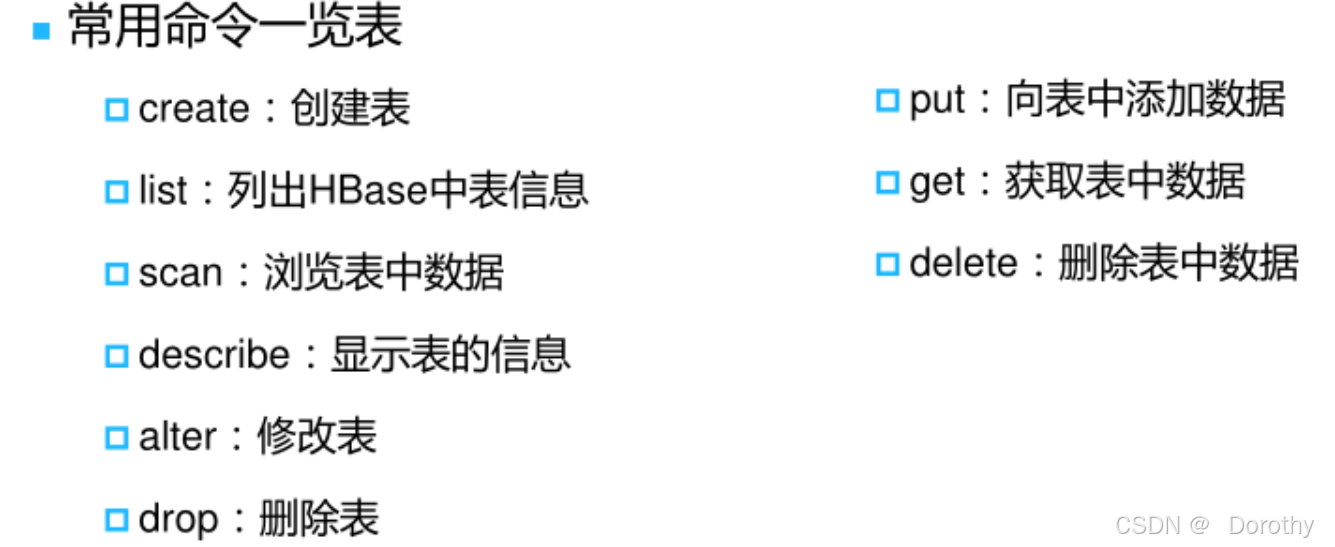
create scan list describe alter
put
get
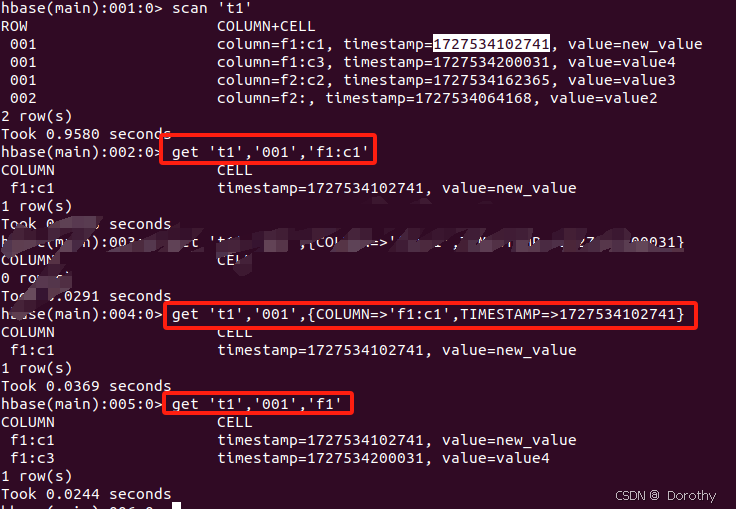
delete
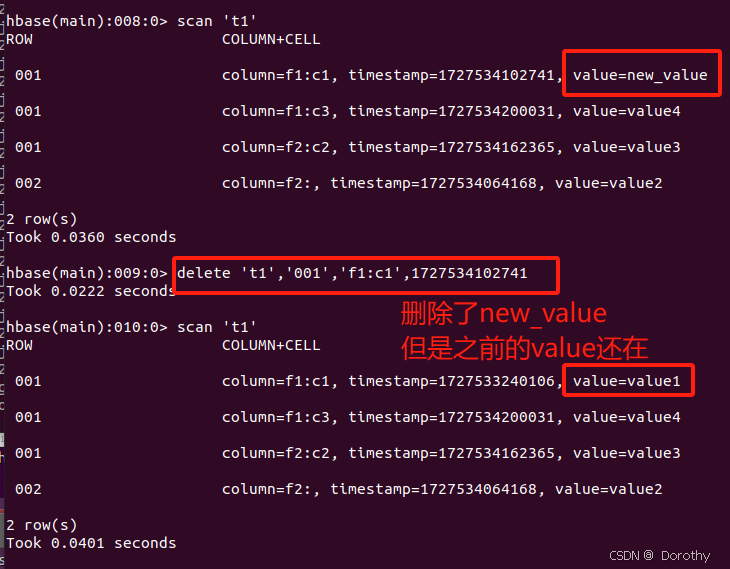
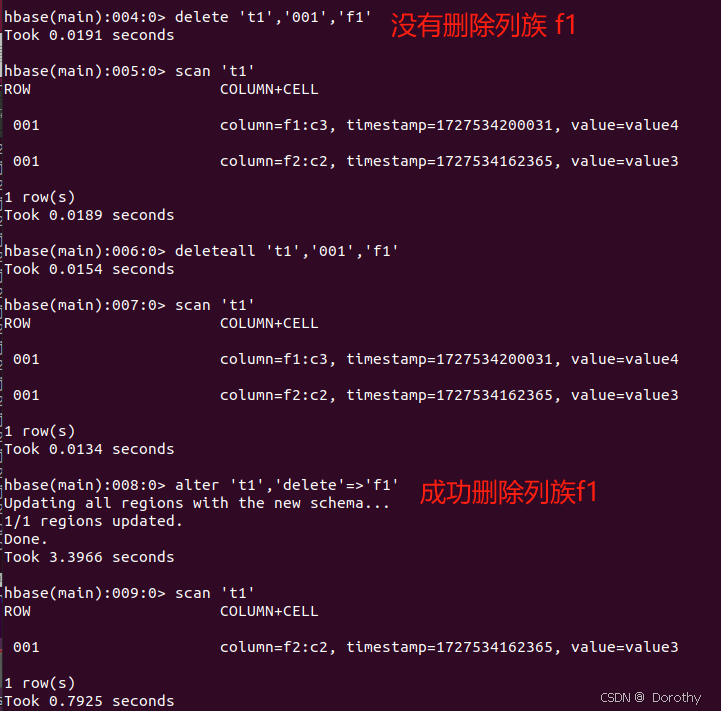
drop
删除表前要disable它
关于NoSQL数据库中的列族和列
这些数据库允许你以非常灵活的方式存储和检索数据
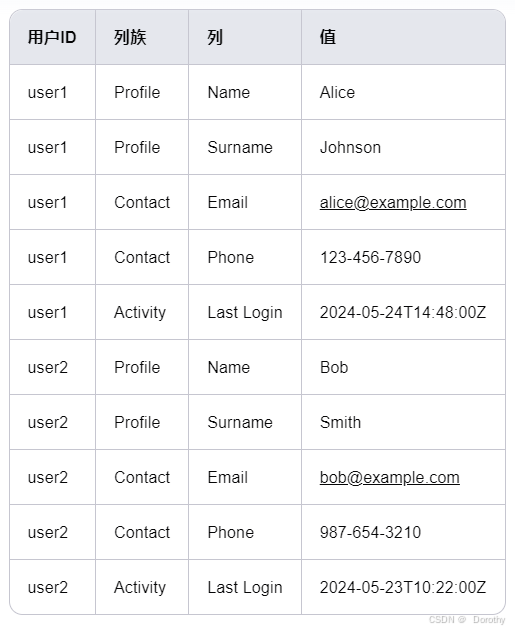
3、关闭hbase服务
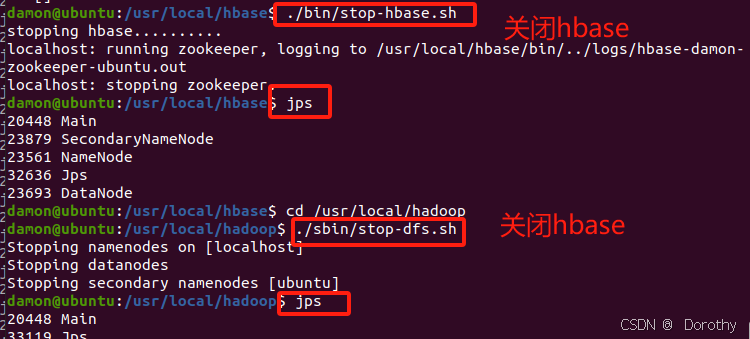
(二)HBase 常用的Java API 及应用实例
1、启动hbase服务
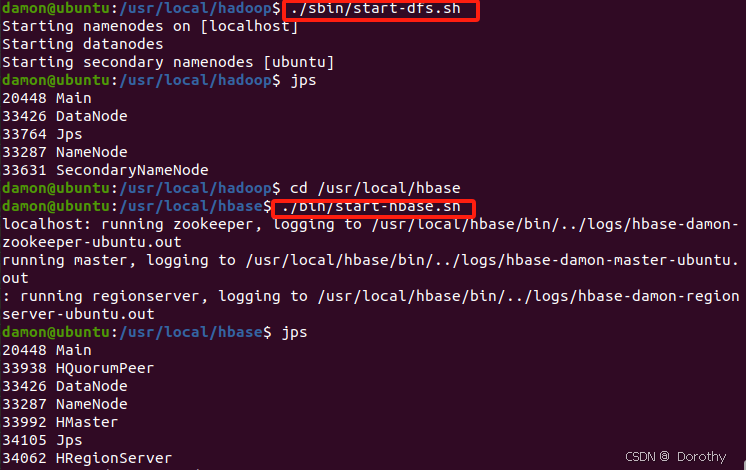
2、启动eclipse

3、新建java project
导入jar包
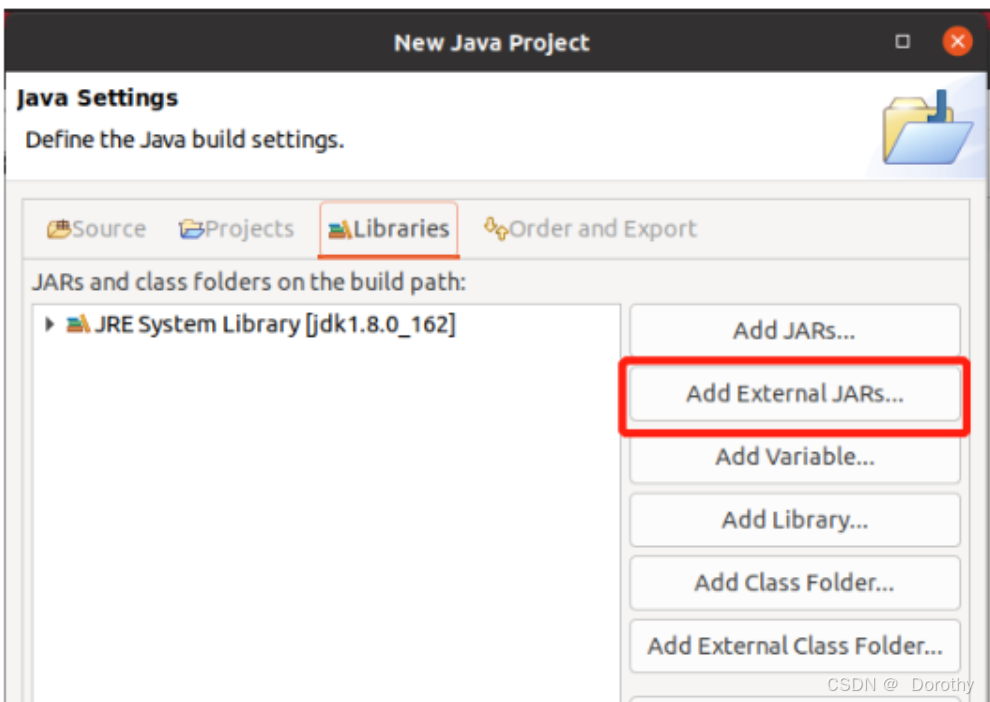
导入/usr/local/hbase/lib中的所有jar包
再导入/usr/local/hbase/lib/client-facing-thirdparty中的所有jar 包
创建类文件
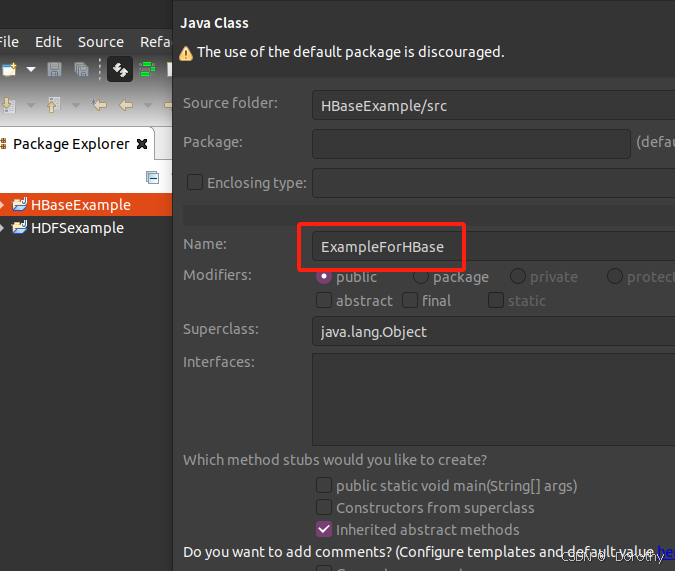
常用Java API:
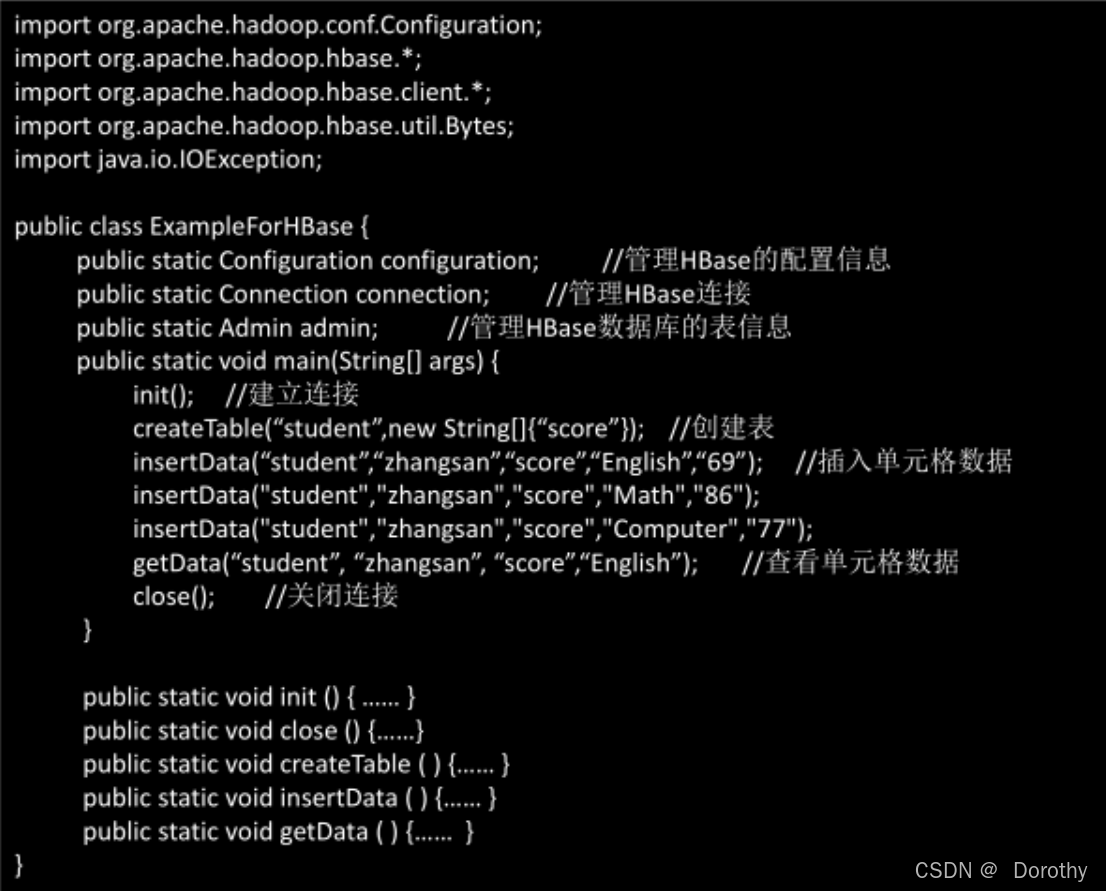
java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.*;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
import java.io.IOException;
public class ExampleForHBase {
public static Configuration configuration;
public static Connection connection;
public static Admin admin;
public static void main(String[] args) throws IOException {
init();
createTable("student", new String[]{"score"});
insertData("student", "zhangsan", "score", "English", "69");
insertData("student", "zhangsan", "score", "Math", "86");
insertData("student", "zhangsan", "score", "Computer", "77");
getData("student", "zhangsan", "score", "English");
close();
}
public static void init() {
configuration = HBaseConfiguration.create();
configuration.set("hbase.rootdir", "hdfs://localhost:9000/hbase");
try {
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void close() {
try {
if (admin != null) {
admin.close();
}
if (connection != null) {
connection.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static void createTable(String myTableName, String[] colFamily) throws IOException {
TableName tableName = TableName.valueOf(myTableName);
if (admin.tableExists(tableName)) {
System.out.println("table is exists!");
} else {
TableDescriptorBuilder tableDescriptor = TableDescriptorBuilder.newBuilder(tableName);
for (String str : colFamily) {
ColumnFamilyDescriptor family = ColumnFamilyDescriptorBuilder.newBuilder(Bytes.toBytes(str)).build();
tableDescriptor.setColumnFamily(family);
}
admin.createTable(tableDescriptor.build());
}
}
public static void insertData(String tableName, String rowKey, String colFamily, String col, String val) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
Put put = new Put(rowKey.getBytes());
put.addColumn(Bytes.toBytes(colFamily), Bytes.toBytes(col), Bytes.toBytes(val));
table.put(put);
table.close();
}
public static void getData(String tableName, String rowKey, String colFamily, String col) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
Get get = new Get(rowKey.getBytes());
get.addColumn(Bytes.toBytes(colFamily), Bytes.toBytes(col));
Result result = table.get(get);
System.out.println(new String(result.getValue(Bytes.toBytes(colFamily), Bytes.toBytes(col))));
table.close();
}
}
run------>run as application
打开hbase shell
list scan