【rCore OS 开源操作系统】Rust 枚举与模式匹配
前言
目前虽然人在大阪,但是还是把笔记本是从来都带身上的。
每天玩了之后,晚上还可以学一学rust
写写代码。
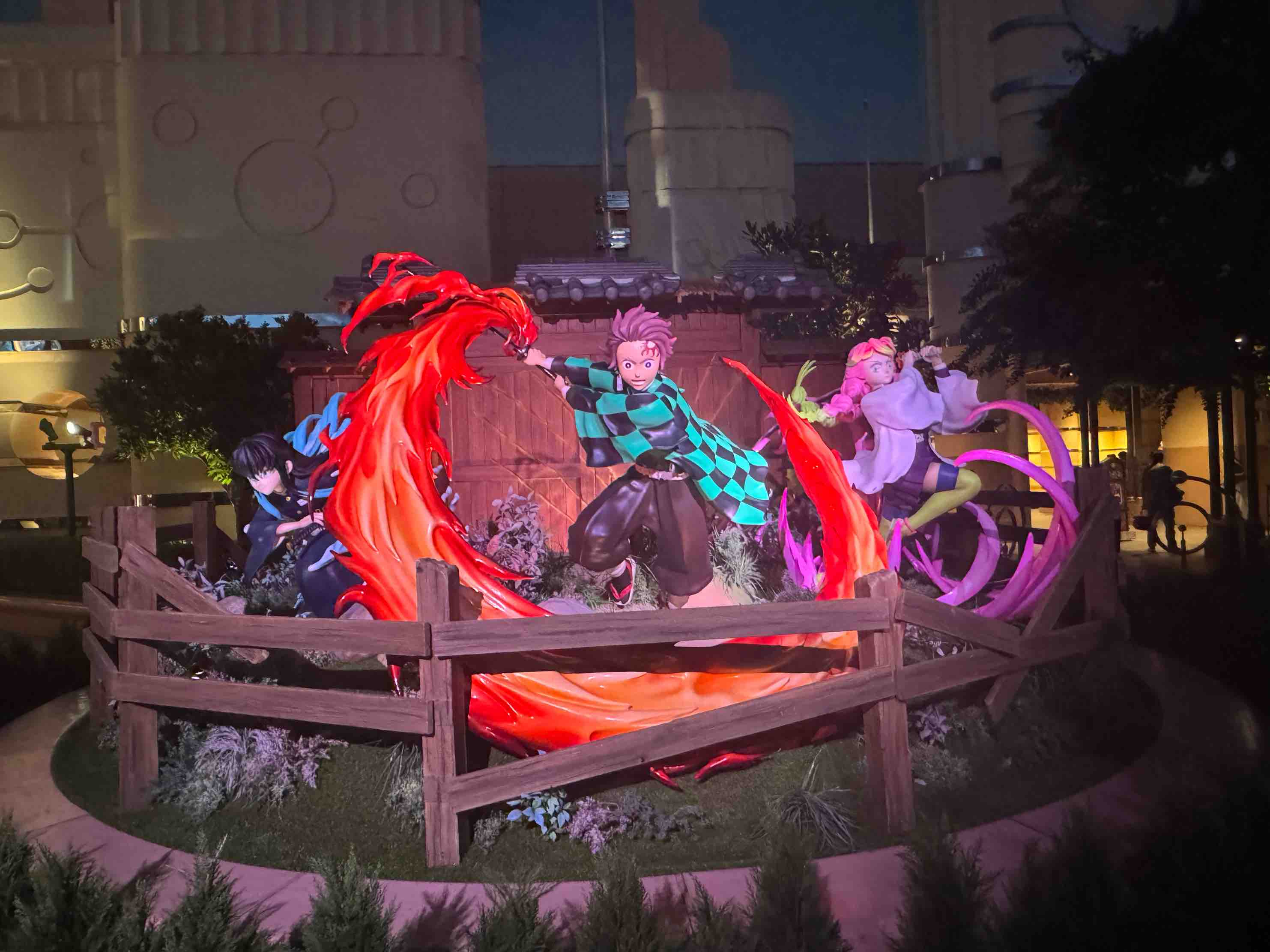
言归正传,本章节涉及到的知识点有:
- 枚举的应用:这里不再是基本语法,而是要学会用。
- 模式匹配实战:使用
match
来处理问题。 - 语法糖:使用
where let
和if let
来处理简化代码。 - 引用与借用:复习一下。
知识点
练习题
option1
题目
rust
// options1.rs
//
// Execute `rustlings hint options1` or use the `hint` watch subcommand for a
// hint.
// I AM NOT DONE
// This function returns how much icecream there is left in the fridge.
// If it's before 10PM, there's 5 pieces left. At 10PM, someone eats them
// all, so there'll be no more left :(
fn maybe_icecream(time_of_day: u16) -> Option<u16> {
// We use the 24-hour system here, so 10PM is a value of 22 and 12AM is a
// value of 0 The Option output should gracefully handle cases where
// time_of_day > 23.
// TODO: Complete the function body - remember to return an Option!
???
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn check_icecream() {
assert_eq!(maybe_icecream(9), Some(5));
assert_eq!(maybe_icecream(10), Some(5));
assert_eq!(maybe_icecream(23), Some(0));
assert_eq!(maybe_icecream(22), Some(0));
assert_eq!(maybe_icecream(25), None);
}
#[test]
fn raw_value() {
// TODO: Fix this test. How do you get at the value contained in the
// Option?
let icecreams = maybe_icecream(12);
assert_eq!(icecreams, 5);
}
}
题解
没有特别难的点,主要是搞懂题目的意思:
22 点之前,冰箱里都有 5 个冰淇淋🍦;
22 点及之后,冰箱里的冰淇淋都被吃掉🍽️;
0~24点之外的情况不存在,需要处理🙅;
rust
fn maybe_icecream(time_of_day: u16) -> Option<u16> {
if time_of_day > 24 || time_of_day < 0 {
None
} else if time_of_day < 22 {
Some(5)
} else {
Some(0)
}
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn check_icecream() {
assert_eq!(maybe_icecream(9), Some(5));
assert_eq!(maybe_icecream(10), Some(5));
assert_eq!(maybe_icecream(23), Some(0));
assert_eq!(maybe_icecream(22), Some(0));
assert_eq!(maybe_icecream(25), None);
}
#[test]
fn raw_value() {
let icecreams = maybe_icecream(12);
assert_eq!(icecreams.unwrap_or(0), 5);
}
}
option2
题目
这里主要是要求把题目中涉及的模式匹配代码改成if let
表达式。
rust
// options2.rs
//
// Execute `rustlings hint options2` or use the `hint` watch subcommand for a
// hint.
// I AM NOT DONE
#[cfg(test)]
mod tests {
#[test]
fn simple_option() {
let target = "rustlings";
let optional_target = Some(target);
// TODO: Make this an if let statement whose value is "Some" type
word = optional_target {
assert_eq!(word, target);
}
}
#[test]
fn layered_option() {
let range = 10;
let mut optional_integers: Vec<Option<i8>> = vec![None];
for i in 1..(range + 1) {
optional_integers.push(Some(i));
}
let mut cursor = range;
// TODO: make this a while let statement - remember that vector.pop also
// adds another layer of Option<T>. You can stack `Option<T>`s into
// while let and if let.
integer = optional_integers.pop() {
assert_eq!(integer, cursor);
cursor -= 1;
}
assert_eq!(cursor, 0);
}
}
题解
rust
#[cfg(test)]
mod tests {
#[test]
fn simple_option() {
let target = "rustlings";
let optional_target = Some(target);
if let optional_target = target {
let word = optional_target;
assert_eq!(word, target)
}
}
#[test]
fn layered_option() {
let range = 10;
let mut optional_integers: Vec<Option<i8>> = vec![None];
for i in 1..(range + 1) {
optional_integers.push(Some(i));
}
let mut cursor = range;
// where let 能依次匹配每一个 vec item
while let Some(maybe_integer) = optional_integers.pop() {
// 由于 optional_integers 的 item 每个都是 Option
// 所以这还得用 if let 再比较一次
if let Some(integer) = maybe_integer {
assert_eq!(integer, cursor);
cursor -= 1;
} else {
// 如果 maybe_integer 是 None,跳过这一轮循环
continue;
}
}
assert_eq!(cursor, 0);
}
}
option3
题目
rust
// options3.rs
//
// Execute `rustlings hint options3` or use the `hint` watch subcommand for a
// hint.
// I AM NOT DONE
struct Point {
x: i32,
y: i32,
}
fn main() {
let y: Option<Point> = Some(Point { x: 100, y: 200 });
match y {
Some(p) => println!("Co-ordinates are {},{} ", p.x, p.y),
_ => panic!("no match!"),
}
y; // Fix without deleting this line.
}
题解
题目代码的报错信息中已经说得很明白了:
shell
⚠️ Compiling of exercises/options/options3.rs failed! Please try again. Here's the output:
error[E0382]: use of partially moved value: `y`
--> exercises/options/options3.rs:20:5
|
17 | Some(p) => println!("Co-ordinates are {},{} ", p.x, p.y),
| - value partially moved here
...
20 | y; // Fix without deleting this line.
| ^ value used here after partial move
|
= note: partial move occurs because value has type `Point`, which does not implement the `Copy` trait
help: borrow this binding in the pattern to avoid moving the value
|
17 | Some(ref p) => println!("Co-ordinates are {},{} ", p.x, p.y),
| +++
error: aborting due to 1 previous error
For more information about this error, try `rustc --explain E0382`.
注意其中这一段:
所以问题就应迎刃而解了,使用引用实现借用即可。
rust
// options3.rs
//
// Execute `rustlings hint options3` or use the `hint` watch subcommand for a
// hint.
struct Point {
x: i32,
y: i32,
}
fn main() {
let y: Option<Point> = Some(Point { x: 100, y: 200 });
// 这里改成引用,这样所有权就不会转移而是被借用
match &y {
Some(p) => println!("Co-ordinates are {},{} ", p.x, p.y),
_ => panic!("no match!"),
}
y; // Fix without deleting this line.
}