在 JSON 中,anyOf
语句通常用于 JSON Schema(JSON 模式)中,来定义多个可能的模式,表示数据可以匹配多个子模式中的任意一个。这种功能常用于验证 JSON 数据是否符合某一组可能的条件之一。
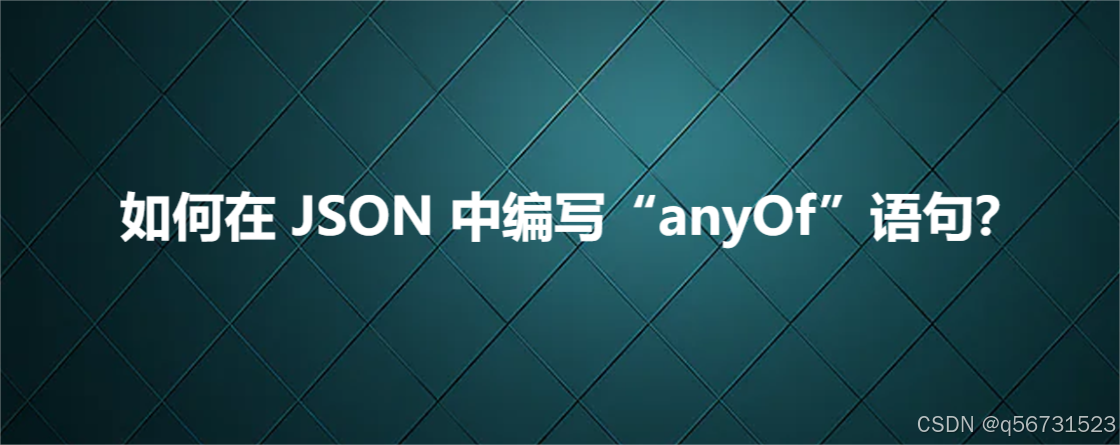
1、问题背景
问题:如何编写 JSON 使其符合给定的 JSON Schema 结构?在 JSON Schema 中,存在一个"anyOf"关键字,要求至少满足一个条件。
2、解决方案
为了符合给定的 JSON Schema 结构,需要对 JSON 进行以下修改:
- 使用
anyOf
关键字可以确保至少满足一个条件。
python
special_needs = [
{
"main": ["learning"],
"sub": ["ADD/ADHD"]
},
{
"main": ["behavioral"]
}
]
main
和sub
属性需要放在同一个对象中。
python
special_needs = [
{
"category_0": {
"main": ["learning"],
"sub": ["ADD/ADHD", "dyslexia", "general learning disability", "language disorder", "intellectual giftedness", "other"]
}
},
{
"category_1": {
"main": ["mental"],
"sub": ["down's syndrome", "asperger's syndrome", "autism", "other"]
}
},
{
"category_2": {
"main": ["behavioral"]
}
},
{
"category_3": {
"main": ["medical"],
"sub": ["diabetes", "allergies", "eating disorders", "chronic illness", "other"]
}
},
{
"category_4": {
"main": ["physical"],
"sub": ["blind", "deaf", "cerebral palsy", "other"]
}
}
]
- 将JSON中的
dict
改为[]
,这样才符合anyOf
中的要求。
python
special_needs = [
{
"category_0": {
"main": ["learning"],
"sub": ["ADD/ADHD", "dyslexia", "general learning disability", "language disorder", "intellectual giftedness", "other"]
}
},
{
"category_1": {
"main": ["mental"],
"sub": ["down's syndrome", "asperger's syndrome", "autism", "other"]
}
},
{
"category_2": {
"main": ["behavioral"]
}
},
{
"category_3": {
"main": ["medical"],
"sub": ["diabetes", "allergies", "eating disorders", "chronic illness", "other"]
}
},
{
"category_4": {
"main": ["physical"],
"sub": ["blind", "deaf", "cerebral palsy", "other"]
}
}
]
anyOf
仅适用于数组类型,因此special_needs
应该是一个数组。
python
special_needs = [
{
"category_0": {
"main": ["learning"],
"sub": ["ADD/ADHD", "dyslexia", "general learning disability", "language disorder", "intellectual giftedness", "other"]
}
},
{
"category_1": {
"main": ["mental"],
"sub": ["down's syndrome", "asperger's syndrome", "autism", "other"]
}
},
{
"category_2": {
"main": ["behavioral"]
}
},
{
"category_3": {
"main": ["medical"],
"sub": ["diabetes", "allergies", "eating disorders", "chronic illness", "other"]
}
},
{
"category_4": {
"main": ["physical"],
"sub": ["blind", "deaf", "cerebral palsy", "other"]
}
}
]
代码示例:
python
import json
# 创建一个包含"anyOf"关键字的 JSON Schema
schema = {
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"special_needs": {
"type": "array",
"items": {
"anyOf": [
{
"properties": {
"category_0": {
"type": "object",
"properties": {
"main": {
"type": "array",
"items": {
"type": "string",
"enum": ["learning"]
}
},
"sub": {
"type": "array",
"items": {
"type": "string",
"enum": ["ADD/ADHD", "dyslexia", "general learning disability", "language disorder", "intellectual giftedness", "other"]
}
}
}
}
}
},
{
"properties": {
"category_1": {
"type": "object",
"properties": {
"main": {
"type": "array",
"items": {
"type": "string",
"enum": ["mental"]
}
},
"sub": {
"type": "array",
"items": {
"type": "string",
"enum": ["down's syndrome", "asperger's syndrome", "autism", "other"]
}
}
}
}
}
},
{
"properties": {
"category_2": {
"type": "object",
"properties": {
"main": {
"type": "array",
"items": {
"type": "string",
"enum": ["behavioral"]
}
}
}
}
}
},
{
"properties": {
"category_3": {
"type": "object",
"properties": {
"main": {
"type": "array",
"items": {
"type": "string",
"enum": ["medical"]
}
},
"sub": {
"type": "array",
"items": {
"type": "string",
"enum": ["diabetes", "allergies", "eating disorders", "chronic illness", "other"]
}
}
}
}
}
},
{
"properties": {
"category_4": {
"type": "object",
"properties": {
"main": {
"type": "array",
"items": {
"type": "string",
"enum": ["physical"]
}
},
"sub": {
"type": "array",
"items": {
"type": "string",
"enum": ["blind", "deaf", "cerebral palsy", "other"]
}
}
}
}
}
}
]
}
}
}
}
# 创建一个符合 JSON Schema 的 JSON 数据
data = {
"special_needs": [
{
"category_0": {
"main": ["learning"],
"sub": ["ADD/ADHD"]
}
},
{
"category_1": {
"main": ["mental"],
"sub": ["down's syndrome"]
}
}
]
}
# 验证 JSON 数据是否符合 JSON Schema
validator = jsonschema.Draft4Validator(schema)
if validator.is_valid(data):
print("JSON data is valid")
else:
print("JSON data is invalid")
# 输出 JSON 数据
print(json.dumps(data, indent=4))
运行代码输出如下:
python
JSON data is valid
{
"special_needs": [
{
"category_0": {
"main": [
"learning"
],
"sub": [
"ADD/ADHD"
]
}
},
{
"category_1": {
"main": [
"mental"
],
"sub": [
"down's syndrome"
]
}
}
]
}
总结
anyOf
允许定义多个可能的模式,数据只需符合其中之一即可。- 它在 JSON Schema 中用于灵活的验证场景,尤其当字段可以有多种可能的结构时。
这种模式非常适合需要灵活数据验证的场景,比如 API 请求的验证、表单数据的校验等。