Django的设计模式及模板层
传统的MVC(例如java)
Django的MTV
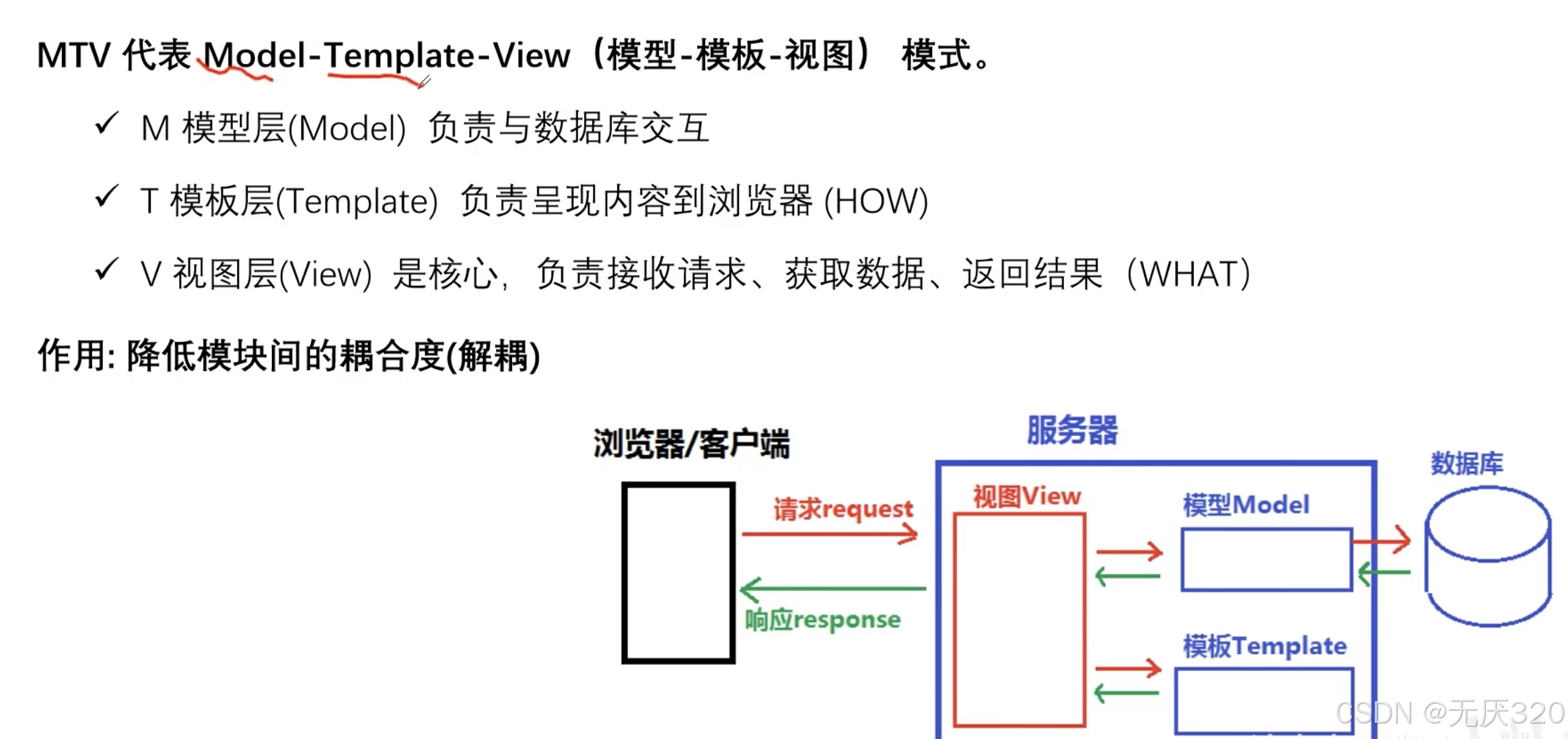
模板层:
模板加载:
代码:
python
def test_html(request):
#方案一
# from django.template import loader
# 1. 使用loader加载模板
# t = loader.get_template('test_html.html')
# # 将t转换成html字符串
# html = t.render()
# return HttpResponse(html)
# 方案二
from django.shortcuts import render
return render(request, 'test_html.html')
python
path('test_html', views.test_html)
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>Hello World</h1>
</body>
</html>
视图层与模板层之间的交互:
代码:
python
def test_html(request):
#方案一
# from django.template import loader
# 1. 使用loader加载模板
# t = loader.get_template('test_html.html')
# # 将t转换成html字符串
# html = t.render()
# return HttpResponse(html)
# 方案二
from django.shortcuts import render
dic = {
'name': 'zhangsan',
'age': 18,
'sex': '男',
'hobby': ['吃饭', '睡觉', '打豆豆'],
'person': {'name': 'lisi', 'age': 20},
}
return render(request, 'test_html.html', dic)
templates
python
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>{{name}}今年{{ age }}性别{{ sex }}爱好{{ hobby }}</h1>
</body>
</html>
python
path('test_html', views.test_html)
结果:
模板的变量和标签:
代码:
python
path('test_html_param', views.test_html_param)
python
def test_html_param(request):
dic = {}
dic['int'] = 88
dic['str'] = 'xiaobai'
dic['lst'] = ['Tom', 'Jack', 'Lily']
dic['dict'] = {'a': 9, 'b': 8}
dic['func'] = say_hi
dic['classobj'] = Dog()
return render(request, 'test_html_param.html', dic)
def say_hi():
return 'hahah'
class Dog(object):
def say(self):
return 'wangwang'
templates
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h3>int是{{int }}</h3>
<h3>str是{{str }}</h3>
<h3>lst 是{{lst}}</h3>
<h3>lst是{{lst.0 }}</h3>
<h3>dict是{{dict}}</h3>
<h3>dict['a']是{{dict.a}}</h3>
<h3>function是{{func}}</h3>
<h3>class obj是{{classobj.say}}</h3>
</body>
</html>
结果:
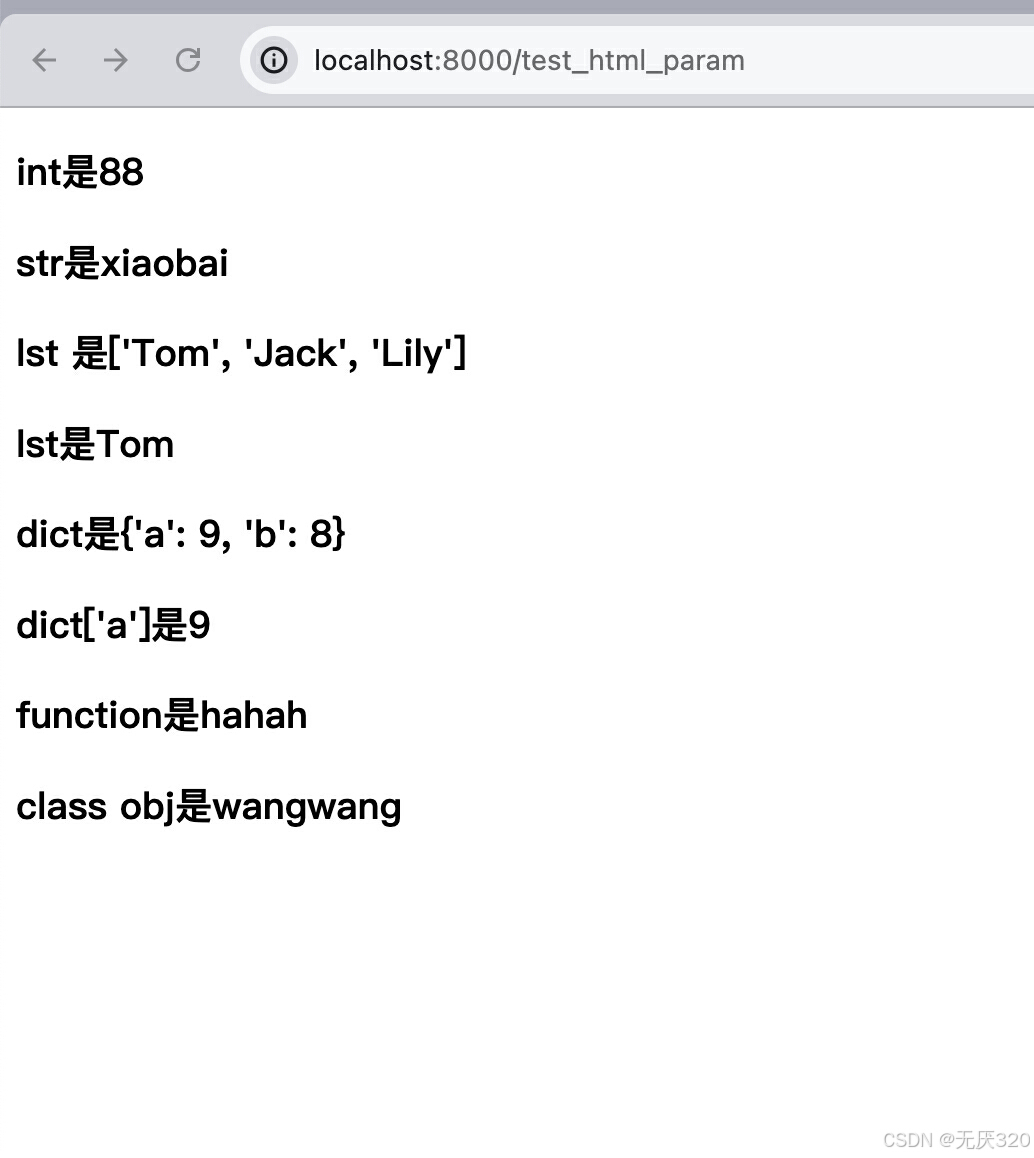
模板标签:
代码:
python
path('test_if_for', views.test_if_for)
python
def test_if_for(request):
dic = {}
dic['x'] = 5
return render(request, 'test_if_for.html', dic)
templates
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>测试if和for</title>
</head>
<body>
{% if x >= 4 %}
输入的值大于等于4
{% else %}
输入的值为小于4
{% endif %}
</body>
</html>
注意:在标签中使用变量时,直接使用变量名即可,不需要加双大括号
for标签:
urls:
python
path('test_if_for', views.test_if_for)
python
def test_if_for(request):
dic = {}
dic['x'] = 5
dic['ls'] = ['a', 'b', 'c']
return render(request, 'test_if_for.html', dic)
templates:
python
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>测试if和for</title>
</head>
<body>
<p>
{% if x >= 4 %}
输入的值大于等于4
{% else %}
输入的值为小于4
{% endif %}
<br>
{% for name in ls %}
{% if forloop.first %}########{% endif %}
{{ forloop.counter0 }}{{ name }}</p>
{% if forloop.last %}########{% endif %}
{% empty %}
没有值
{% endfor %}
</body>
</html>