目录
信号量
信号量可以理解为计数器;
信号量的理解
信号量有时候叫信号灯--用来保护共享资源的--临界资源
POSIX信号量和SystemV信号量作用相同,都是用于同步操作,达到无冲突的访问共享资源目的。 但POSIX可以用于线程间同步。
快速认识接口
初始化信号量:
int sem_init(sem_t *sem, int pshared, unsigned int value);
销毁信号量:int sem_destroy(sem_t *sem);
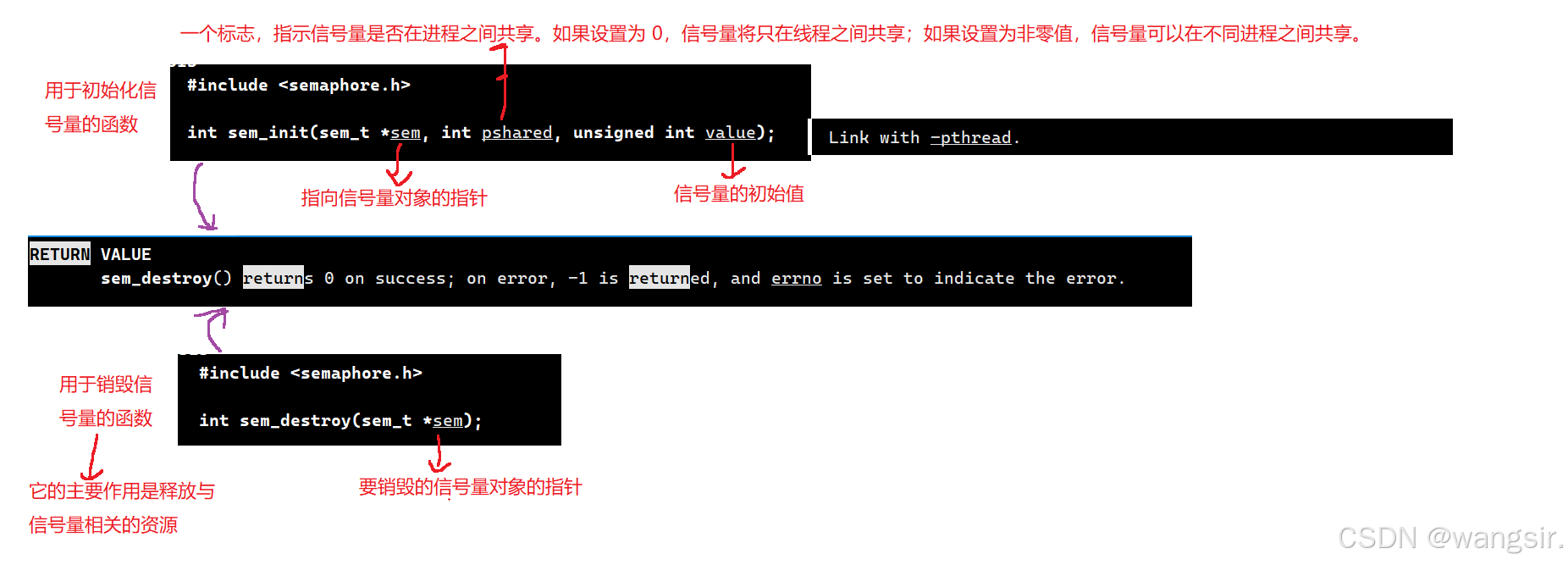
等待信号量:
int sem_wait(sem_t *sem); //P()
功能:等待信号量,会将信号量的值减1
发布信号量:int sem_post(sem_t *sem);//V()
功能:发布信号量,表示资源使用完毕,可以归还资源了。将信号量值加1
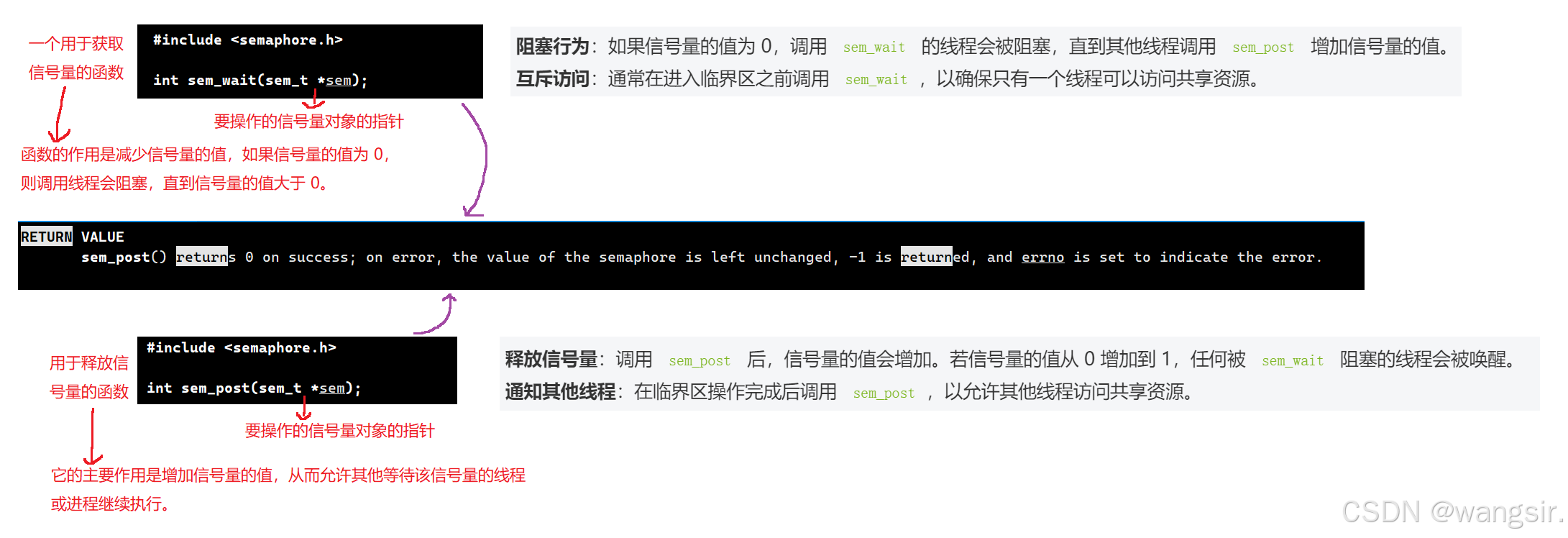
基于环形队列的生产消费模型
上一个生产者-消费者的例子是基于queue的,其空间可以动态分配,现在基于固定大小的环形队列重写这个程序(POSIX信号量)
在物理层面上是线性结构但是在逻辑层面上描述为环形结构;
理论
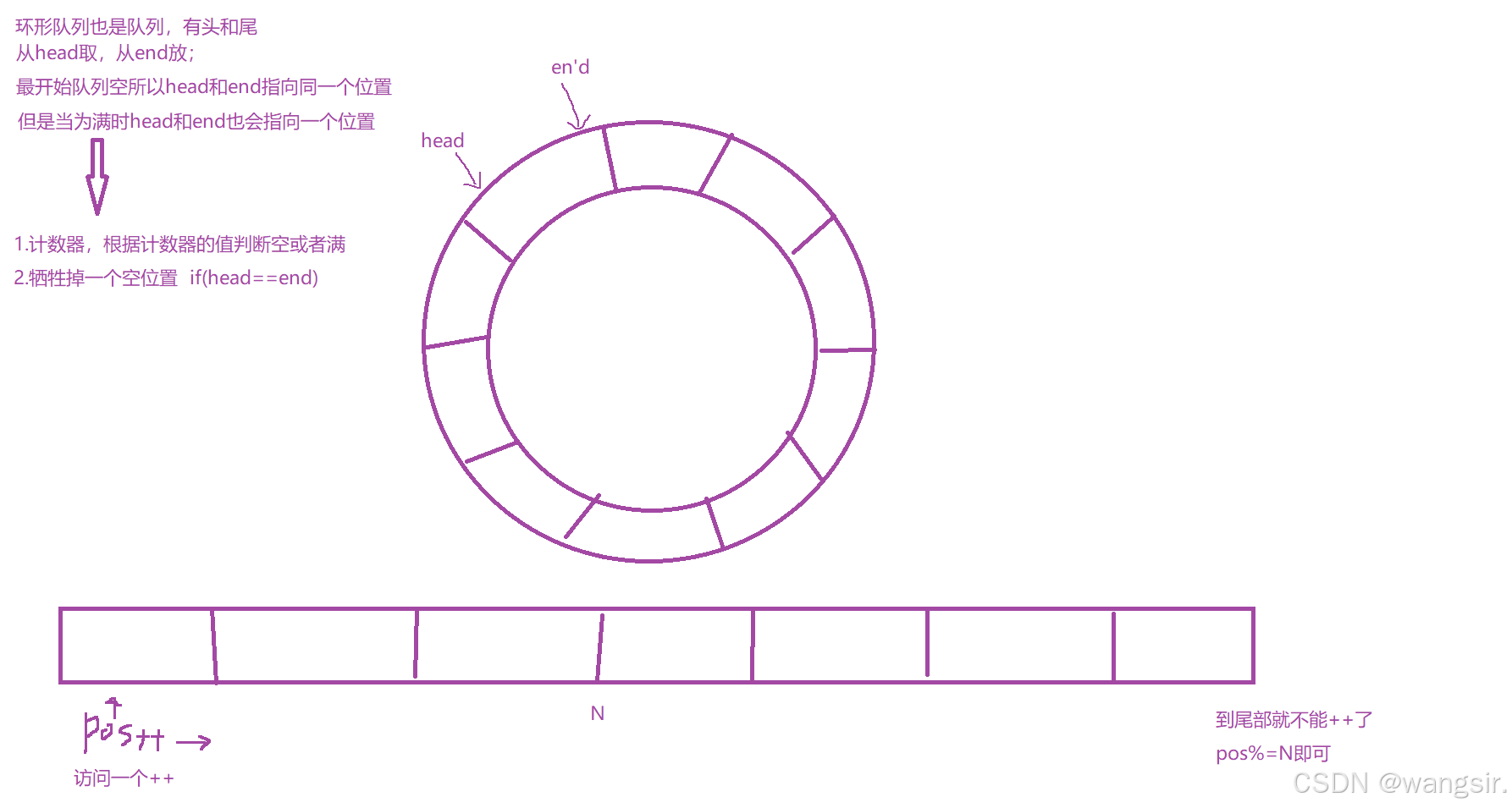
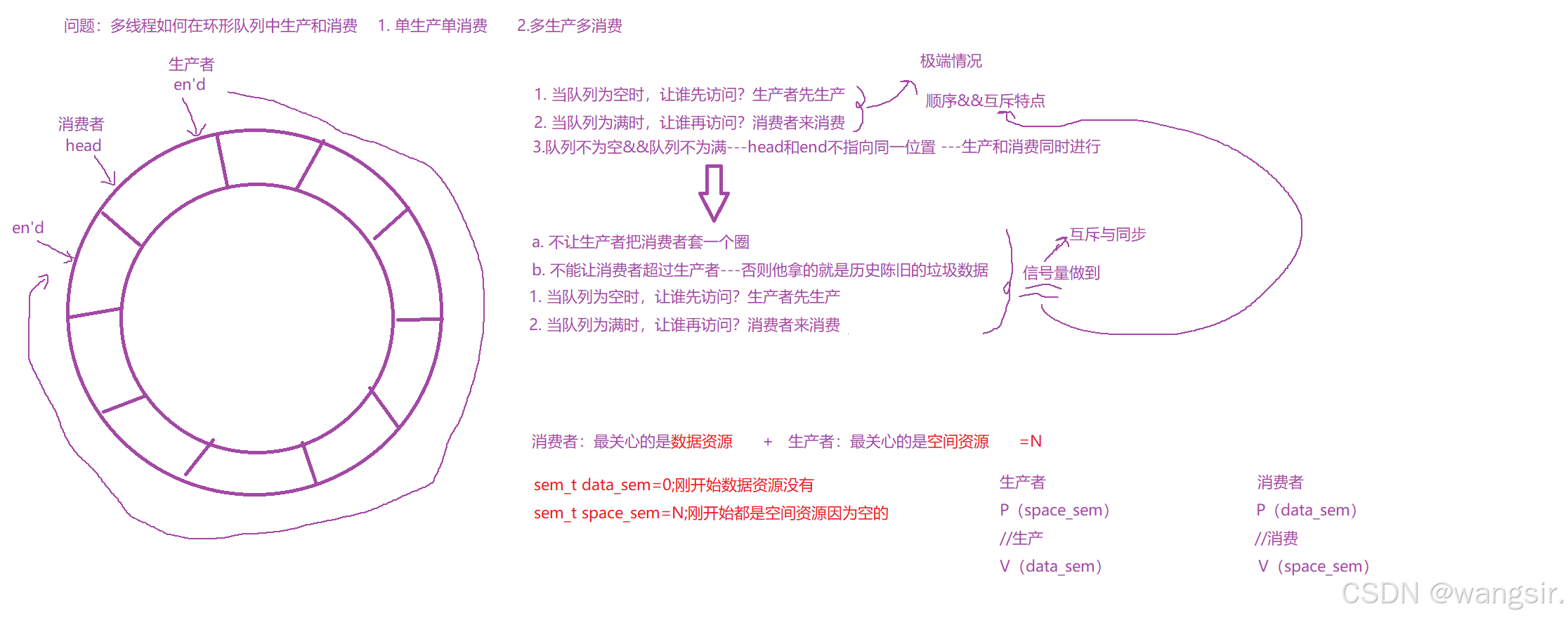
具体实现
单-单
大概方案:单生产单消费
ringqueue.hpp:
#pragma once
#include <iostream>
#include <vector>
#include <string>
#include <semaphore.h>
using namespace std;
template <typename T> // 表示这是一个模板,T 是模板参数,可以是任意类型。
class ringqueue
{
private:
void p(sem_t &s)
{
sem_wait(&s); // 进行p操作,将信号量--
}
void v(sem_t &s)
{
sem_post(&s); // 进行v操作,将信号量++
}
public:
ringqueue(int max_cap) : _max_cap(max_cap), _ringqueue(max_cap), _c_step(0), _p_step(0) // 初始位置都为0
{
sem_init(&_data_sem, 0, 0); // 刚开始没有为0
sem_init(&_space_sem, 0, max_cap); // 初始化信号量,刚开始全是
}
void Push(const T &in)
{ // 使用引用来传入数据,这样可以高效且安全地传递参数。
// 生产者
p(_space_sem); // 预定资源,申请空间信号量才能生产
_ringqueue[_p_step] = in; // 往环形队列里的p位置生产
_p_step++;
_p_step %= _max_cap;
v(_data_sem); // 释放资源
}
void Pop(T *out)
{ // 使用指针以允许函数直接修改外部变量,返回弹出的数据
p(_data_sem); // 预定资源,申请数据信号量才能消费;没有的话就阻塞在这里
// 走到这里说明一定有资源了
*out = _ringqueue[_c_step]; // 在环形队列里的c位置拿
_c_step++;
_c_step %= _max_cap;
v(_space_sem); // 释放资源
}
~ringqueue()
{
sem_destroy(&_data_sem);
sem_destroy(&_space_sem);
}
private:
vector<T> _ringqueue;
int _max_cap; // 最大容量
int _c_step; // 生产者位置
int _p_step; // 消费者位置
sem_t _data_sem; // 数据信号量
sem_t _space_sem; // 空间信号量
};
task.hpp:
#pragma once
#include<iostream>
#include<functional>
using namespace std;
// using task_t=function<void()>;//等价于typedef function<void()> task_t;
// //定义了一个新的类型别名 task_t,它表示一个接受无参数并返回 void 的函数类型
// void download(){
// cout<<"i am a download task"<<endl;
// }
class task
{
public:
task() {}
task(int x, int y) : _x(x), _y(y)
{
}
void excute()
{
_result = _x + _y;
}
void operator()(){
excute();
}
string debug()
{
string msg = to_string(_x) + "+" + to_string(_y) + "=?";
return msg;
}
string result()
{
string msg = to_string(_x) + "+" + to_string(_y) +"="+ to_string(_result);
return msg;
}
~task()
{
}
private:
int _x;
int _y;
int _result;
};
main.cc :
#include "ringqueue.hpp"
#include"task.hpp"
#include <iostream>
#include <ctime>
#include <unistd.h>
void *consumer(void *agv)
{
ringqueue<task> *rq = static_cast<ringqueue<task> *>(agv); // 强转
while (true)
{
sleep(1);
// 1.消费数据
task t;//消费者拿走任务,得有个空任务
rq->Pop(&t);
// 2.处理数据
t();// 这将调用 t.operator(),从而执行 excute() 方法
cout << "consumer-> " << t.result() << endl;//任务结果
}
}
void *producter(void *agv)
{
ringqueue<task> *rq = static_cast<ringqueue<task> *>(agv);
while (true)
{
// 1.构造数据
int x = rand() % 10 + 1;
usleep(x*1000);
int y=rand() % 10 + 1;
task t(x,y);//构造出来一个任务
// 2.生产
rq->Push(t);
cout << "producter-> " <<t.debug() << endl;//任务名字
}
}
int main()
{
srand(time(nullptr) ^ getpid());
ringqueue<task> *rq = new ringqueue<task>(5); // 定义一个环形队列
// 这里使用int类型
pthread_t c, p; // 单生产单消费
pthread_create(&c, nullptr, consumer, rq);
pthread_create(&p, nullptr, producter, rq); // 传过来就看到同一个环形队列
pthread_join(c, nullptr);
pthread_join(p, nullptr);
}
int:
class task:
多-多
**多生产多消费:**得加锁,因为_data_step和_space_step只有一个,多个进来的话会乱导致数据竞争和不一致问题;
多个生生产者得先竞争,竞争到的才能申请信号量生产--同理消费者也如此,所以加两把锁;
那么是先加锁还是先申请信号量?如果是先加锁,那么势必申请信号量的线程只能是一个生产者--过程没问题,那么其他线程只能在锁那里等待 ;
如果先申请信号量(信号量足够)再加锁,那么所有线程都申请到了再竞争锁看谁先生产,然后解锁,其他线程再竞争锁就不用再申请信号量了因为已经申请过了;
那么我们选择先申请信号量,因为申请信号量是原子的-不会出错,让线程先把可用的资源瓜分了然后竞争锁或等待锁看谁进去--效率高;
ringqueue.hpp:
#pragma once
#include <iostream>
#include <vector>
#include <string>
#include <semaphore.h>
using namespace std;
template <typename T> // 表示这是一个模板,T 是模板参数,可以是任意类型。
class ringqueue
{
private:
void p(sem_t &s)
{
sem_wait(&s); // 进行p操作,将信号量--
}
void v(sem_t &s)
{
sem_post(&s); // 进行v操作,将信号量++
}
public:
ringqueue(int max_cap) : _max_cap(max_cap), _ringqueue(max_cap), _c_step(0), _p_step(0) // 初始位置都为0
{
sem_init(&_data_sem, 0, 0); // 刚开始没有为0
sem_init(&_space_sem, 0, max_cap); // 初始化信号量,刚开始全是
pthread_mutex_init(&_c_mutex, nullptr); // 对锁初始化
pthread_mutex_init(&_p_mutex, nullptr);
}
void Push(const T &in)
{ // 使用引用来传入数据,这样可以高效且安全地传递参数。
// 生产者
p(_space_sem); // 预定资源,申请空间信号量才能生产
pthread_mutex_lock(&_p_mutex); // 竞争成功的才能申请信号量
_ringqueue[_p_step] = in; // 往环形队列里的p位置生产
_p_step++;
_p_step %= _max_cap;
v(_data_sem); // 释放资源
pthread_mutex_unlock(&_p_mutex);
}
void Pop(T *out)
{ // 使用指针以允许函数直接修改外部变量,返回弹出的数据
p(_data_sem); // 预定资源,申请数据信号量才能消费;没有的话就阻塞在这里
pthread_mutex_lock(&_c_mutex); // 竞争成功的才能申请信号量
// 走到这里说明一定有资源了
*out = _ringqueue[_c_step]; // 在环形队列里的c位置拿
_c_step++;
_c_step %= _max_cap;
v(_space_sem); // 释放资源
pthread_mutex_unlock(&_c_mutex);
}
~ringqueue()
{
sem_destroy(&_data_sem);
sem_destroy(&_space_sem);
pthread_mutex_destroy(&_c_mutex);
pthread_mutex_destroy(&_p_mutex);
}
private:
vector<T> _ringqueue;
int _max_cap; // 最大容量
int _c_step; // 生产者位置
int _p_step; // 消费者位置
sem_t _data_sem; // 数据信号量
sem_t _space_sem; // 空间信号量
pthread_mutex_t _c_mutex; // 消费者锁
pthread_mutex_t _p_mutex; // 生产者锁
};
task.hpp:
#pragma once
#include<iostream>
#include<functional>
using namespace std;
// using task_t=function<void()>;//等价于typedef function<void()> task_t;
// //定义了一个新的类型别名 task_t,它表示一个接受无参数并返回 void 的函数类型
// void download(){
// cout<<"i am a download task"<<endl;
// }
class task
{
public:
task() {}
task(int x, int y) : _x(x), _y(y)
{
}
void excute()
{
_result = _x + _y;
}
void operator()(){
excute();
}
string debug()
{
string msg = to_string(_x) + "+" + to_string(_y) + "=?";
return msg;
}
string result()
{
string msg = to_string(_x) + "+" + to_string(_y) +"="+ to_string(_result);
return msg;
}
~task()
{
}
private:
int _x;
int _y;
int _result;
};
#include "ringqueue.hpp"
#include"task.hpp"
#include <iostream>
#include <ctime>
#include <unistd.h>
void *consumer(void *agv)
{
ringqueue<task> *rq = static_cast<ringqueue<task> *>(agv); // 强转
while (true)
{
sleep(1);
// 1.消费数据
task t;//消费者拿走任务,得有个空任务
rq->Pop(&t);
// 2.处理数据
t();// 这将调用 t.operator(),从而执行 excute() 方法
cout << "consumer-> " << t.result() << endl;//任务结果
}
}
void *producter(void *agv)
{
ringqueue<task> *rq = static_cast<ringqueue<task> *>(agv);
while (true)
{
// 1.构造数据
int x = rand() % 10 + 1;
usleep(x*1000);
int y=rand() % 10 + 1;
task t(x,y);//构造出来一个任务
// 2.生产
rq->Push(t);
cout << "producter-> " <<t.debug() << endl;//任务名字
}
}
int main()
{
srand(time(nullptr) ^ getpid());
ringqueue<task> *rq = new ringqueue<task>(5); // 定义一个环形队列
pthread_t c1, c2, p1, p2;
pthread_create(&c1, nullptr, consumer, rq);// 传过来就看到同一个环形队列
pthread_create(&c2, nullptr, consumer, rq);
pthread_create(&p1, nullptr, producter, rq);
pthread_create(&p2, nullptr, producter, rq);
pthread_join(c1, nullptr);
pthread_join(c2, nullptr);
pthread_join(p1, nullptr);
pthread_join(p2, nullptr);
return 0;
}
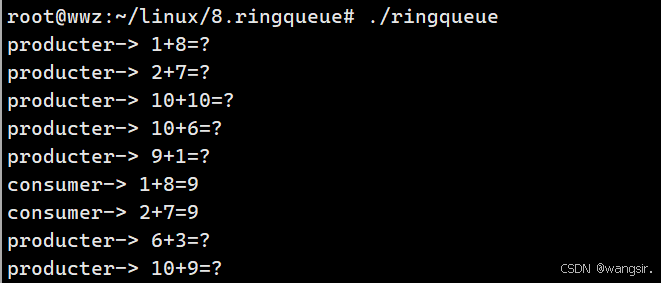