目录
牛客_最长公共子序列_DP
描述:
给定两个字符串 s1 和 s2,长度为 n 和 m 。求两个字符串最长公共子序列的长度。
所谓子序列,指一个字符串删掉部分字符(也可以不删)形成的字符串。例如:字符串 "arcaea" 的子序列有 "ara" 、 "rcaa" 等。但 "car" 、 "aaae" 则不是它的子序列。
所谓 s1 和 s2 的最长公共子序列,即一个最长的字符串,它既是 s1 的子序列,也是 s2 的子序列。
数据范围 : 1≤m,n≤1000。保证字符串中的字符只有小写字母。
要求:空间复杂度 O(mn),时间复杂度 O(mn)
进阶:空间复杂度 O(min(m,n)),时间复杂度 O(mn)
输入描述:
第一行输入一个整数 n 和 m ,表示字符串 s1 和 s2 的长度。
接下来第二行和第三行分别输入一个字符串 s1 和 s2。
输出描述:
输出两个字符串的最长公共子序列的长度
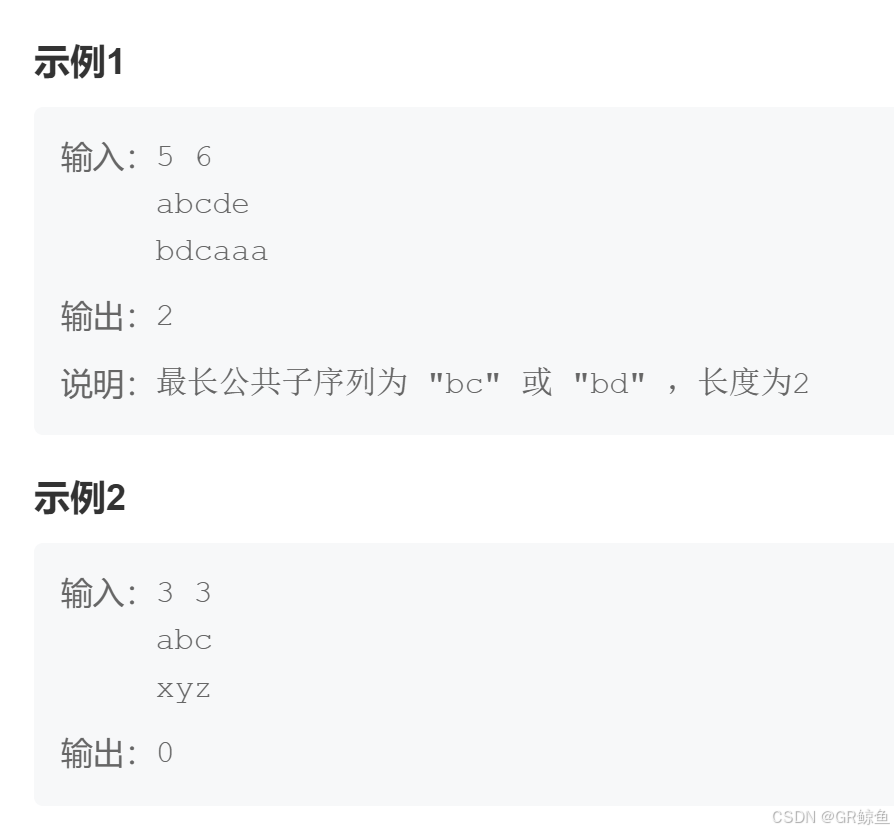
题目解析
子序列即两个字符串中公共的字符,但不一定连续。
从题干中可以提取出问题:求字符串s和t的最长公共子序列 假设LCS(m,n)为长度为m的字符串s与长度为n的字符串t的最长公共子序列,直接进行求解时不太 好求解,那么可以将问题简化为其子问题,假设s和t的长度为任意值i和j,即求LCS(i,j):
C++代码
cpp
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int n = 0, m = 0;
string str1, str2;
cin >> n >> m >> str1 >> str2;;
vector<vector<int>> dp(n + 1, vector<int>(m + 1));
str1 = " " + str1, str2 = " " + str2;
for (int i = 1; i <= n; ++i)
{
for (int j = 1; j <= m; ++j)
{
if (str1[i] == str2[j])
{
// dp[i][j] = max(dp[i][j], dp[i - 1][j - 1] + 1);
dp[i][j] = dp[i - 1][j - 1] + 1;
}
else
{
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
cout << dp[n][m];
return 0;
}
Java代码
cpp
import java.util.Scanner;
// 注意类名必须为 Main, 不要有任何 package xxx 信息
public class Main
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
int n = in.nextInt(), m = in.nextInt();
char[] s1 = in.next().toCharArray();
char[] s2 = in.next().toCharArray();
int[][] dp = new int[n + 1][m + 1];
for(int i = 1; i <= n; i++)
{
for(int j = 1; j <= m; j++)
{
if(s1[i - 1] == s2[j - 1])
dp[i][j] = dp[i - 1][j - 1] + 1;
else
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
System.out.println(dp[n][m]);
}
}