在现代软件开发中,API(应用程序编程接口)扮演着至关重要的角色,尤其是在数据交互和网络通信方面。本文将通过一个具体的示例,展示如何使用Python解析API返回的数据结构,并提供代码示例。
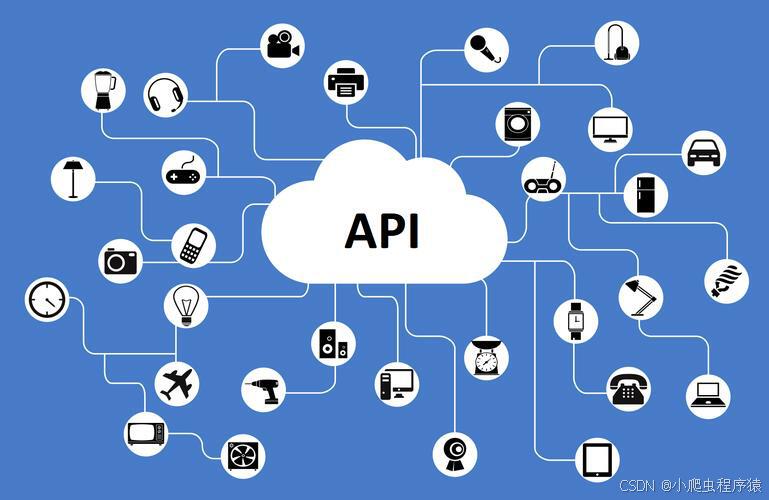
一、理解API返回的数据结构
API返回的数据通常以JSON(JavaScript Object Notation)格式存在,它是一种轻量级的数据交换格式,易于人阅读和编写,同时也易于机器解析和生成。一个典型的JSON对象可能包含多个字段,每个字段都有对应的值。
二、淘宝商品详情API返回值结构
以淘宝商品详情API为例,该API返回的数据结构可能包含以下字段:
num_iid
:商品ID。title
:商品标题。price
:商品价格。pic_url
:商品图片URL。skus
:商品SKU信息。sold_quantity
:商品销量。total_sold
:总销量。comment_count
:评价数量。
三、Python代码示例
以下是一个Python代码示例,展示如何调用淘宝商品详情API并解析返回的数据结构:
python
import requests
import hashlib
import time
import urllib.parse
# 淘宝开放平台API相关配置
APP_KEY = 'your_app_key'
APP_SECRET = 'your_app_secret'
SESSION = 'your_session' # 如果使用Session方式认证,需要传入
def get_taobao_sign(params):
"""生成淘宝API签名"""
params['app_key'] = APP_KEY
params['timestamp'] = str(int(time.time()))
params = sorted(params.items())
sign_content = ''.join(f'{k}{v}' for k, v in params if k not in ['sign'])
sign = hashlib.md5(sign_content.encode('utf-8')).hexdigest().upper()
params['sign'] = sign
return params
def taobao_item_get(item_ids):
"""调用淘宝商品详情API"""
url = 'https://eco.taobao.com/router/rest'
params = {
'method': 'taobao.item.get',
'v': '2.0',
'fields': 'num_iid,title,price,pic_url,skus,sold_quantity,total_sold,comment_count',
'item_ids': ','.join(map(str, item_ids)),
# 如果是Session方式认证,需要传入session参数
# 'session': SESSION,
}
params = get_taobao_sign(params)
response = requests.get(url, params=params)
return response.json()
# 示例调用
item_ids = [1234567890] # 替换为实际的商品ID
result = taobao_item_get(item_ids)
# 解析返回值
if result['taobao_response']['code'] == 200:
items = result['taobao_response']['item_get_response']['items']['item']
for item in items:
print(f"商品ID: {item['num_iid']}")
print(f"商品标题: {item['title']}")
print(f"商品价格: {item['price']}")
print(f"商品图片: {item['pic_url']}")
print(f"商品销量: {item['sold_quantity']}")
print(f"总销量: {item['total_sold']}")
print(f"评价数量: {item['comment_count']}")
print("SKU信息:")
for sku in item['skus']['sku']:
print(f" SKU ID: {sku['properties_name']}, 价格: {sku['price']}, 库存: {sku['quantity']}")
else:
print(f"调用失败: {result['taobao_response']['msg']}")
四、注意事项
- 遵守使用规范:在使用淘宝API时,必须遵守淘宝开放平台的使用规范和限制,不得进行违规操作。
- 调用频率限制:注意API的调用频率限制,避免频繁调用导致被封禁。
- 敏感信息处理:对敏感信息进行妥善处理,确保用户数据的安全。