1、把课上类的三个练习题的构造函数写出来
cpp
#include <iostream>
#include <cstring>
using namespace std;
class Car
{
string color;
string brond;
double speed;
public:
Car(string c,string b,double s):color("black"),brond("Benz"),speed(180.9)
{
color = c;
brond = b;
speed = s;
cout << "Car的有参构造" << endl;
}
//无参时使用默认参数
Car():color("black"),brond("Benz"),speed(180.9){}
void display();
void acc(int a);
};
void Car::display()
{
cout << "汽车品牌:" << brond << endl;
cout << "汽车颜色:" << color << endl;
cout << "速度:" << speed << "km/h" << endl;
}
void Car::acc(int a)
{
speed += a;
}
int main()
{
//有参构造函数
Car c1("white","buick",50.5);
c1.display();
c1.acc(5);
c1.display();
Car c3;
c3.display();
return 0;
}
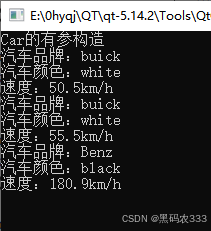
cpp
#include <iostream>
using namespace std;
class Curcle
{
int radius;
public:
Curcle(int r):radius(5)
{
radius = r;
}
Curcle():radius(5){}
void show(double PI=3.14);
};
void Curcle::show(double PI)
{
double perimeter = 2 * PI * radius;
double area = PI * radius * radius;
cout << "圆的周长 = " << perimeter << endl;
cout << "圆的面积 = " << area << endl;
}
int main()
{
Curcle s1(3);
s1.show();
Curcle s2;
s2.show();
return 0;
}
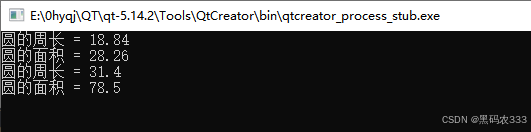
cpp
#include <iostream>
using namespace std;
class Rec
{
int length;
int width;
public:
// void set_length(int l);
// void set_width(int w);
Rec(int l,int w)
{
length = l;
width = w;
}
int get_length();
int get_width();
void show();
};
//void Rec::set_length(int l)
//{
// length = l;
//}
//void Rec::set_width(int w)
//{
// width = w;
//}
int Rec::get_length()
{
cout << "length = " << length << endl;
return length;
}
int Rec::get_width()
{
cout << "width = " << width << endl;
return width;
}
void Rec::show()
{
cout << "s = " << length * width << endl;
}
int main()
{
Rec s1(5,4);
// s1.set_length(5);
// s1.set_width(4);
int l = s1.get_length();
int w = s1.get_width();
s1.show();
return 0;
}
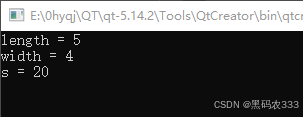
Xmind
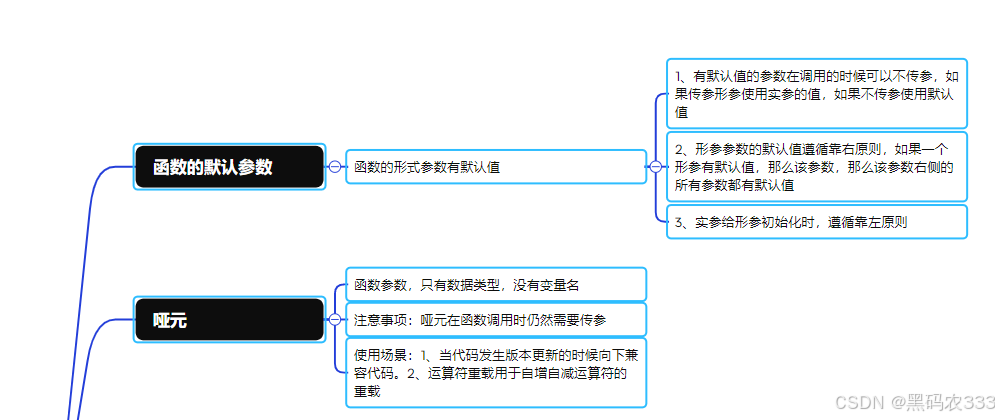
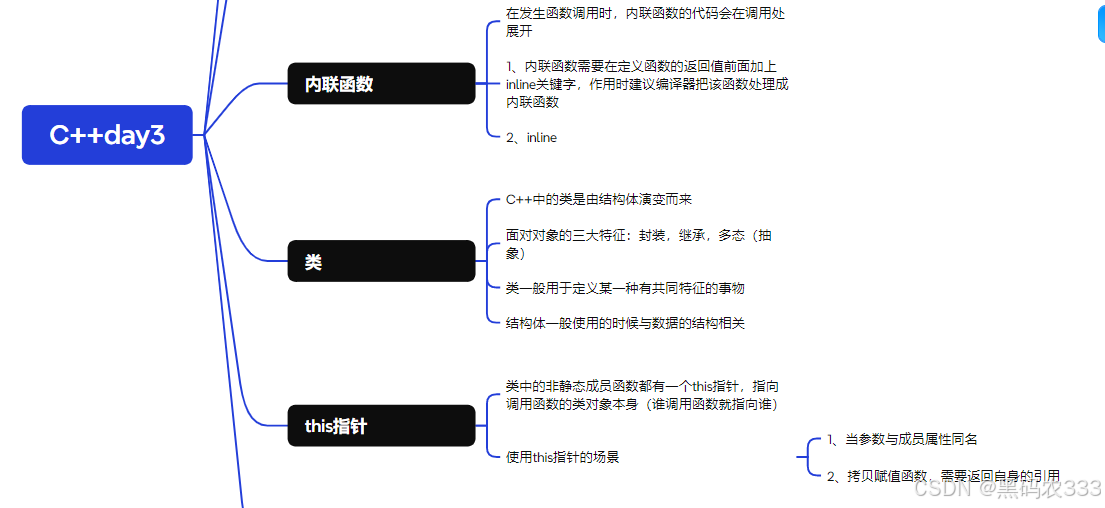