很多时候我们需要在项目里面嵌入其他项目或者被其他项目嵌入,如我们开发一个开源项目b,用户需要在自己的项目a嵌入b项目,使用b项目的功能,而且要实现a项目工作最小化,最好实现引入即用。
1.定义b项目的自定义配置
(1).将你的application.properties或yml配置文件改个名,如我改成了application-project.properties,这样做是为了b项目被a项目嵌入引用的时候,配置不会被覆盖和冲突。
(2).既然改了名,就要让springboot知道你有这份配置,可以用@Configuration和@PropertySource注解。
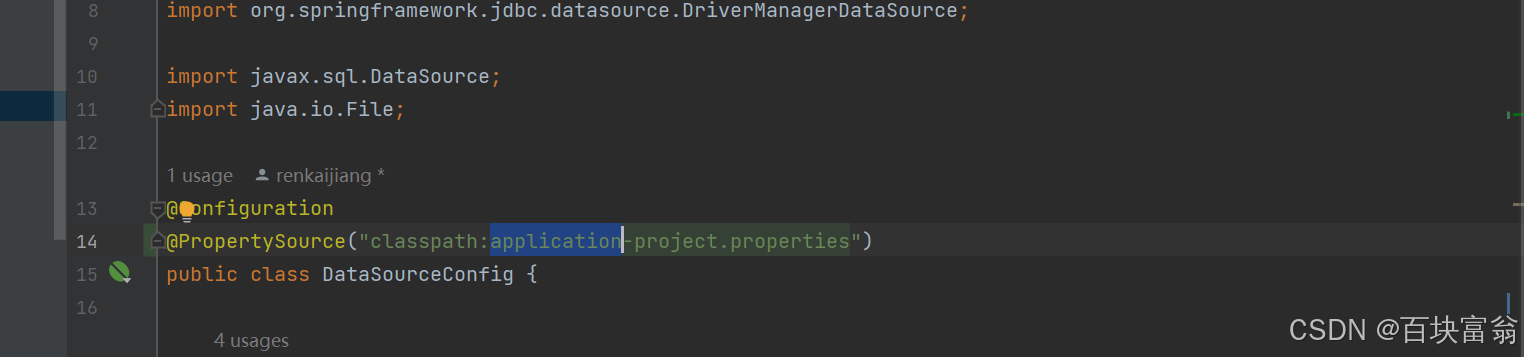
(3).将一些常用配置改成自定义名称配置,避免a项目引用时冲突覆盖,并且在配置文件中加载到springboot中,如我这里的数据库配置把前缀改成了自定义。
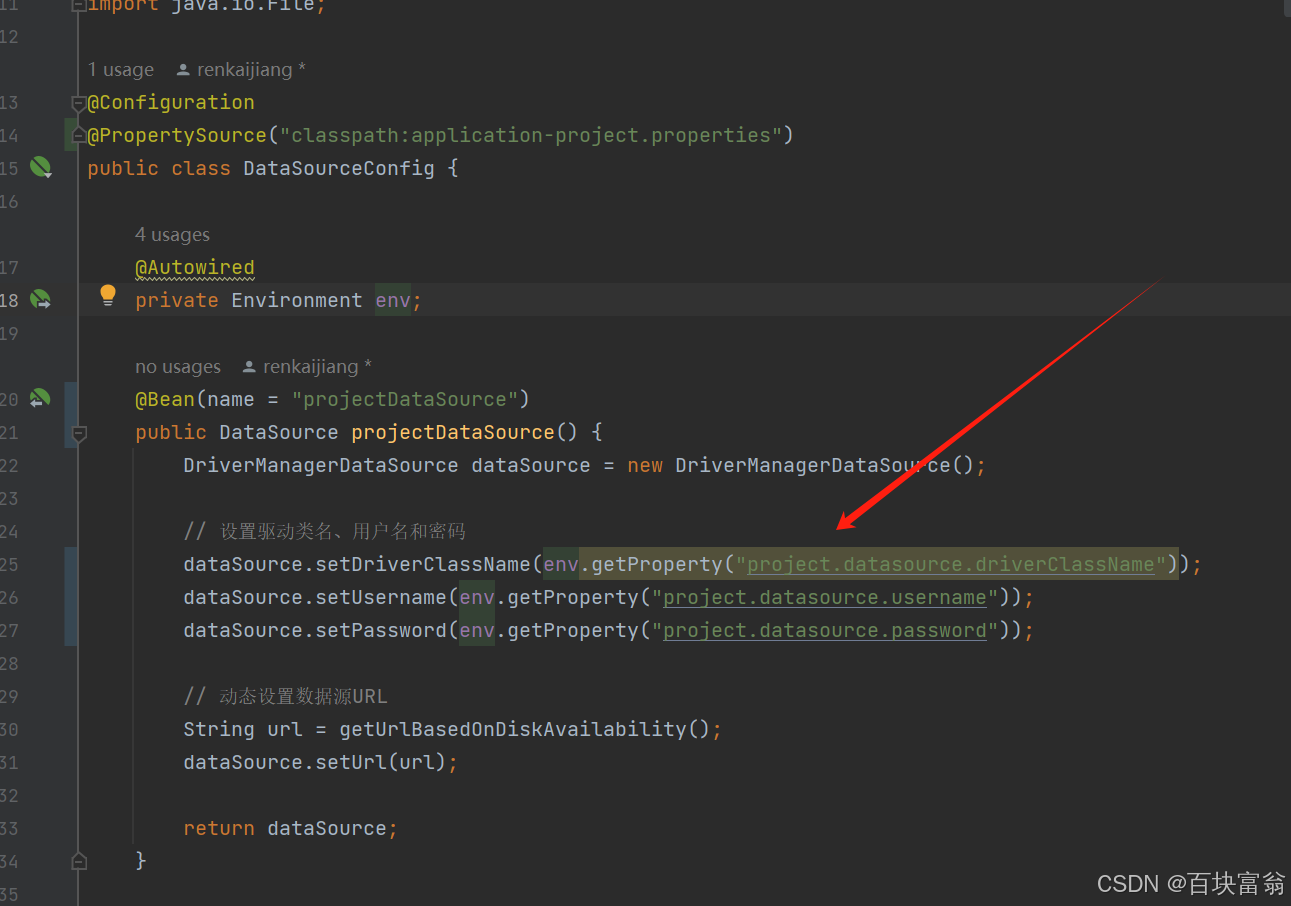
2.配置自动注册类,确保你的b项目的配置能在a项目中自动配置
(1).引入spring boot自动配置包
java
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure</artifactId>
</dependency>
(2). 定义自动配置类,创建自动配置类:编写一个或多个配置类来定义项目 A 提供的服务、控制器以及 FreeMarker 配置等,让a项目在引用b项目的时候会自动加载这些配置,如我这里自定义了一些需要自动配置的组件。
java
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.web.servlet.ViewResolver;
import org.springframework.web.servlet.view.ContentNegotiatingViewResolver;
import org.springframework.web.servlet.view.freemarker.FreeMarkerConfigurer;
import org.springframework.web.servlet.view.freemarker.FreeMarkerViewResolver;
import javax.sql.DataSource;
import java.util.ArrayList;
import java.util.List;
@Configuration
@Import(DataSourceConfig.class)
@ConditionalOnProperty(prefix = "project.datasource", name = "enabled", havingValue = "true", matchIfMissing = true)
@EnableConfigurationProperties(ProjectProperties.class)
@MapperScan(basePackages = "com.missyoubug.easyjtest.dao")
public class ProjectAutoConfiguration {
@Bean(name = "bprojectSqlSessionFactory")
public SqlSessionFactoryBean bprojectSqlSessionFactory(@Qualifier("projectDataSource") DataSource dataSource) throws Exception {
SqlSessionFactoryBean sessionFactory = new SqlSessionFactoryBean();
sessionFactory.setDataSource(dataSource);
sessionFactory.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/*.xml"));
return sessionFactory;
}
@Bean
public ContentNegotiatingViewResolver contentNegotiatingViewResolver(FreeMarkerViewResolver freeMarkerViewResolver) {
ContentNegotiatingViewResolver resolver = new ContentNegotiatingViewResolver();
List<ViewResolver> viewResolvers = new ArrayList<>();
viewResolvers.add(freeMarkerViewResolver);
resolver.setViewResolvers(viewResolvers);
return resolver;
}
@Bean
public FreeMarkerViewResolver freeMarkerViewResolver() {
FreeMarkerViewResolver resolver = new FreeMarkerViewResolver();
resolver.setPrefix("");
resolver.setSuffix(".ftl");
resolver.setContentType("text/html;charset=UTF-8");
resolver.setOrder(0); // 设置最高优先级
return resolver;
}
@Bean
public FreeMarkerConfigurer freemarkerConfig() {
FreeMarkerConfigurer configurer = new FreeMarkerConfigurer();
configurer.setTemplateLoaderPath("classpath:/templates/");
return configurer;
}
}
java
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "project.datasource")
public class ProjectProperties {
private String url;
private String driverClassName;
private String username;
private String password;
// Getters and Setters
}
(3).让Spring boot加载我们的自定义自动配置,创建 spring.factories 文件:在 src/main/resources/META-INF/ 目录下创建 spring.factories 文件,并在其中声明自动配置类。

java
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.missyoubug.easyjtest.config.ProjectAutoConfiguration
3.配置maven打包
(1).使用maven-compiler-plugin和maven-resources-plugin插件处理java源代码和静态资源打包,注意不要用spring-boot-maven-plugin打包。
(2).在<resource>详细列明你需要打进去哪些资源(这里踩坑很久,开始以为只要有<directory>src/main/resources</directory>,就会把resources下的资源全打进去,然而并不是这样,需要详细列明)。
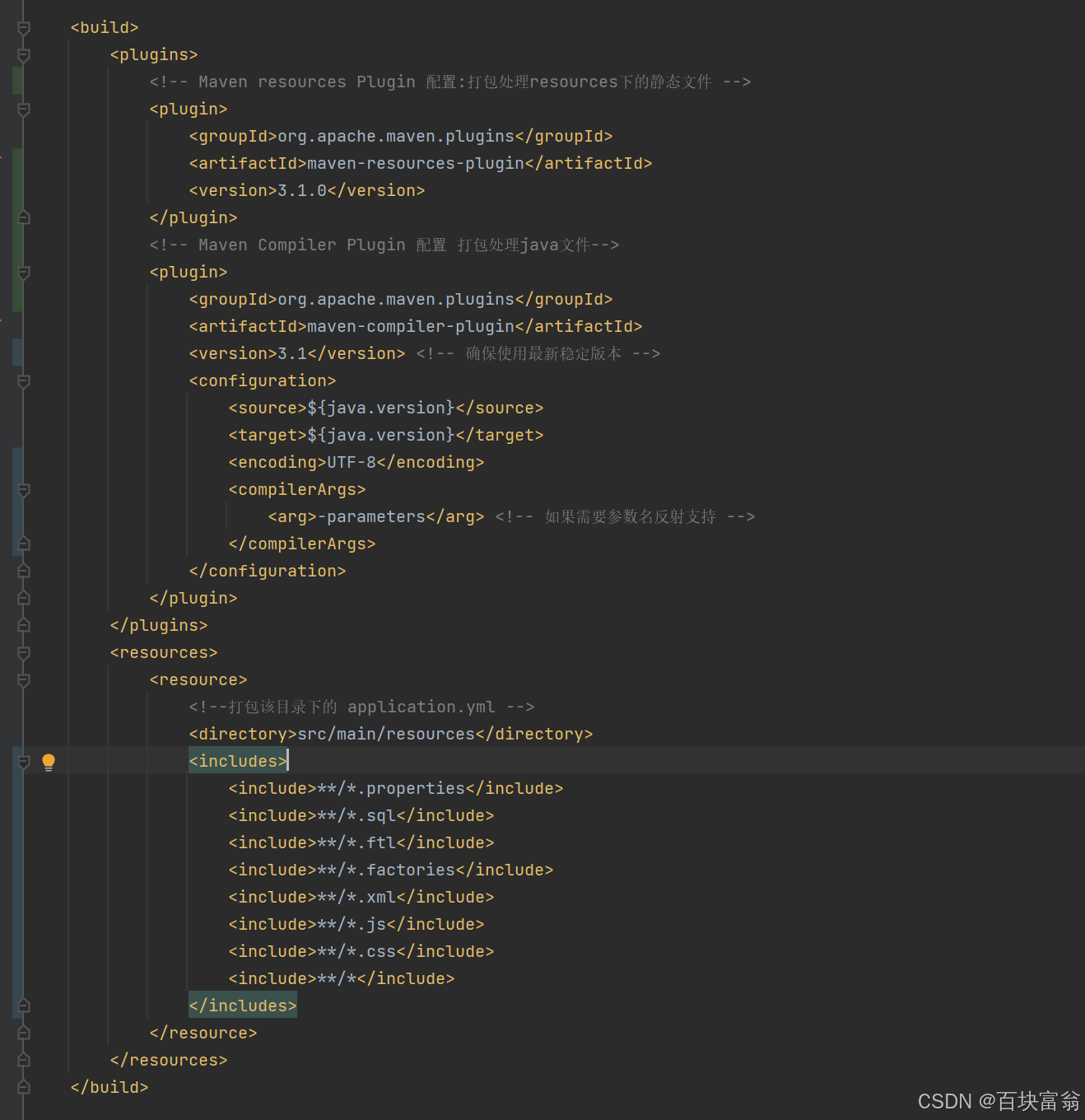
(3).运行mvn clean install命令将b项目打成jar到本地maven仓库,或者是远程仓库。
4.a项目引用及使用被嵌入的b项目
(1).a项目maven引入b项目的maven坐标,如我这里:
java
<dependency>
<groupId>com.missyouBUG</groupId>
<artifactId>Easy-JTest</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
(2).a项目让springboot扫描到b项目的资源文件,一般不用做这一步,但我这里a项目需要使用b项目的页面资源。
(3)如此上,a项目就能完全嵌入b项目使用b项目的资源及功能,如还有问题可以打出a项目详细spring加载过程日志,看看有什么没有加载的在解决。
java
logging.level.org.springframework.boot.autoconfigure=DEBUG
logging.level.org.springframework.jdbc=DEBUG
logging.level.org.springframework.web=DEBUG
logging.level.org.springframework.boot.autoconfigure.web.servlet=DEBUG
#com替换成自己的路径
logging.level.com.missyoubug.easyjtest.config=DEBUG
这两天在搞一个帮助自测的开源项目,踩了这里的坑所以记录下,也希望各位开发者多发扬开源及写原创博客的优良传统,这样自己遇到问题,也最大可能的有前人写下的经验,大家都才能更快的解决,不要抄过去抄过来的,保持严谨的开发态度。