目录
[1. 空字符串及null](#1. 空字符串及null)
[2. value值的双引号与单引号问题](#2. value值的双引号与单引号问题)
[3. 配置对象](#3. 配置对象)
[4. 配置集合](#4. 配置集合)
在上文中,已经介绍了SpringBoot项目中最常用的properties和yml配置文件的基本语法形式:
[【Spring】_SpringBoot配置文件-CSDN博客若需在项目中主动读取配置文件,可以使用@Value注解实现,使以"{ }"格式读取,示例如下:# 自定义配置启动程序,通过Chrome进行访问:可见配置文件成功读取;若需在项目中主动读取配置文件,可以使用@Value注解实现,使以"{ }"格式读取,示例如下:demo:启动程序,使用Chrome进行访问:可见配置文件成功读取;在yml配置的文件使用{ }进行读取时,需将配置文件中的冒号与空格更换为.;https://blog.csdn.net/m0_63299495/article/details/145479386https://blog.csdn.net/m0_63299495/article/details/145479386https://blog.csdn.net/m0_63299495/article/details/145479386](https://blog.csdn.net/m0_63299495/article/details/145479386 "【Spring】_SpringBoot配置文件-CSDN博客若需在项目中主动读取配置文件,可以使用@Value注解实现,使以\"{ }"格式读取,示例如下:# 自定义配置启动程序,通过Chrome进行访问:可见配置文件成功读取;若需在项目中主动读取配置文件,可以使用@Value注解实现,使以"{ }\"格式读取,示例如下:demo:启动程序,使用Chrome进行访问:可见配置文件成功读取;在yml配置的文件使用{ }进行读取时,需将配置文件中的冒号与空格更换为.;https://blog.csdn.net/m0_63299495/article/details/145479386https://blog.csdn.net/m0_63299495/article/details/145479386")由于properties配置仍有代码的冗余,yml配置文件略显优势。且由于yml文件的语法要求较多,本篇再行更详细地介绍yml文件的配置及读取。
1. 空字符串及null
在yml配置文件中,有两个较为特殊的内容配置:空字符串和null;
null使用~ ,空字符串可直接用空格表示,但并不直观,建议空字符用单引号或双引号引起;
使用示例:
application.yml内容如下:
demo:
key1: hello,yml
key2: hello
key3: ~
key4: ~
key5: ''
key6: ''
ymlController内容如下:
java
package com.example.iocdemo1.Controller;
import com.example.iocdemo1.Model.DBType;
import com.example.iocdemo1.Model.Student;
import jakarta.annotation.PostConstruct;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ymlController {
@Value("${demo.key3}")
private String key3;
@Value("${demo.key4}")
private Integer key4;
@Value("${demo.key5}")
private String key5;
@Value("${demo.key6}")
private Integer key6;
@PostConstruct
public void init(){
// 以String类型读取null
System.out.println("key3: "+key3);
System.out.println(key3==null);
// 以Integer类型读取null
System.out.println("key4: "+key4);
System.out.println(key4==null);
// 以String类型读取''
System.out.println("key5: "+key5);
System.out.println(StringUtils.hasLength(key5));
// 以Integer类型读取''
System.out.println("key6: "+key6);
System.out.println(key6==null);
}
}
启动程序,查看日志:
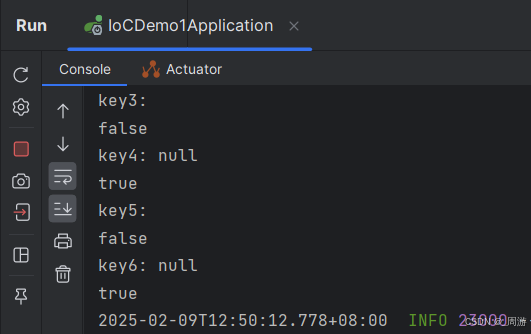
~ 和 '' 采用String读取时,都被解析为空字符串;
~ 和 '' 采用Integer(包括其他包装类)读取时,都被解析为null;
2. value值的双引号与单引号问题
采用\n(含义为换行)进行测试,若实现了换行,说明未被转义;
application.yml内容如下:
string:
str1: Hello \n Spring Boot
str2: 'Hello \n Spring Boot'
str3: "Hello \n Spring Boot"
ymlController内容如下:
java
package com.example.iocdemo1.Controller;
import jakarta.annotation.PostConstruct;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ymlController {
@Value("${string.str1}")
private String str1;
@Value("${string.str2}")
private String str2;
@Value("${string.str3}")
private String str3;
@PostConstruct
public void init(){
System.out.println("str1: "+str1);
System.out.println("str2: "+str2);
System.out.println("str3: "+str3);
}
}
启动程序,日志如下:
可见,无引号和使用单引号的字符串中的\n并未被解析为换行,即发生了转义;
使用双引号的字符串中的\n被解析为换行,即未发生转义;
单引号会对特殊字符进行了转义,双引号不会对特殊字符进行转义;
3. 配置对象
在yml中新增student对象相关配置项:
html
student:
id: 1
name: zhangsan
age: 25
创建Model包,编写student类并使用@Component将其交给Spring容器管理:
java
package com.example.iocdemo1.Model;
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "student")
@Data
public class Student {
private Integer id;
private String name;
private Integer age;
}
在ymlController中对配置文件中的对象进行读取:
java
package com.example.iocdemo1.Controller;
import com.example.iocdemo1.Student;
import jakarta.annotation.PostConstruct;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ymlController {
@Autowired
private Student student;
@PostConstruct
public void init(){
System.out.println("student: "+student);
}
}
启动项目,日志如下:
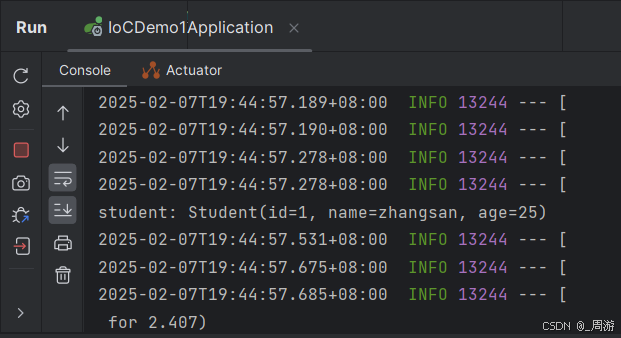
可见配置的对象的配置项获取成功;
@Configurationproperties注解用于从配置文件中读取对象,
@Configurationproperties(prefix = "xxx")表示从配置文件中读取前缀为xxx的对象;
4. 配置集合
yml文件如下:
java
dbtype:
name:
- mysql
- sqlserver
- db2
在Model包下新建DBType类,并使用@Component注解将其交给Spring容器管理:
java
package com.example.iocdemo1.Model;
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
@ConfigurationProperties(prefix = "dbtype")
@Data
public class DBType {
// private List<String> name;
private String[] name;
}
在ymlController中对配置文件中的该集合进行读取:
java
package com.example.iocdemo1.Controller;
import com.example.iocdemo1.Model.DBType;
import com.example.iocdemo1.Model.Student;
import jakarta.annotation.PostConstruct;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ymlController {
@Autowired
private DBType dbType;
@PostConstruct
public void init(){
System.out.println("dbtype: "+dbType);
System.out.println("length: "+dbType.getName().length);
}
}
启动程序,日志如下:
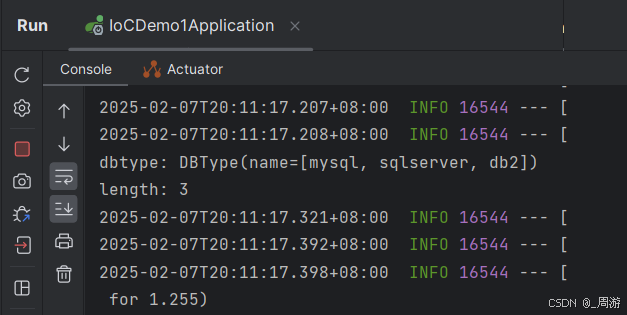
注:1、DBType类中的成员变量采用List<String> 或 String [ ] 接收均可,但其名称必须与yml配置文件中的name保持一致,否则将无法成功读取yml配置文件;
2、在yml配置文件中,若-后不加空格,这部分内容会被解析为一个整体,统一赋值给对应属性:
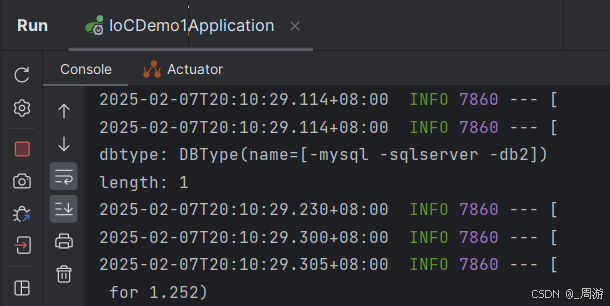