文章目录
1.长度最小的子数组
1.题目
2.解题思路
3.代码编写
cpp
class Solution {
public:
int minSubArrayLen(int target, vector<int>& nums) {
int sum = 0, ret = INT_MAX;
for(int left = 0, right = 0; right < nums.size(); right++)
{
sum += nums[right];
while(sum >= target)
{
ret = min(ret, right - left + 1);
sum -= nums[left++];
}
}
return ret == INT_MAX ? 0 : ret;
}
};
2.无重复字符的最长字串
1.题目
2.解题思路
3.解题代码
cpp
class Solution {
public:
int lengthOfLongestSubstring(string s) {
unordered_map<char, int> ci;
int ret = 0;
for(int left = 0, right = 0; right < s.size(); right++)
{
ci[s[right]]++;
while(ci[s[right]] > 1)
ci[s[left++]]--;
ret = max(ret, right - left + 1);
}
return ret;
}
};
3.最大连续1的个数Ⅲ
1.题目
2.解题思路
3.解题代码
cpp
class Solution {
public:
int longestOnes(vector<int>& nums, int k) {
int ret = 0,ret0 = 0;
for(int left = 0, right = 0; right < nums.size();)
{
while(right < nums.size())
{
ret0 += 1 - nums[right++];
if(ret0 > k)
break;
else ret = max(ret, right - left);
}
while(ret0 > k)
{
ret0 -= 1 - nums[left++];
}
}
return ret;
}
};
4.将x减到0的最小操作数
1.题目
2.解题思路
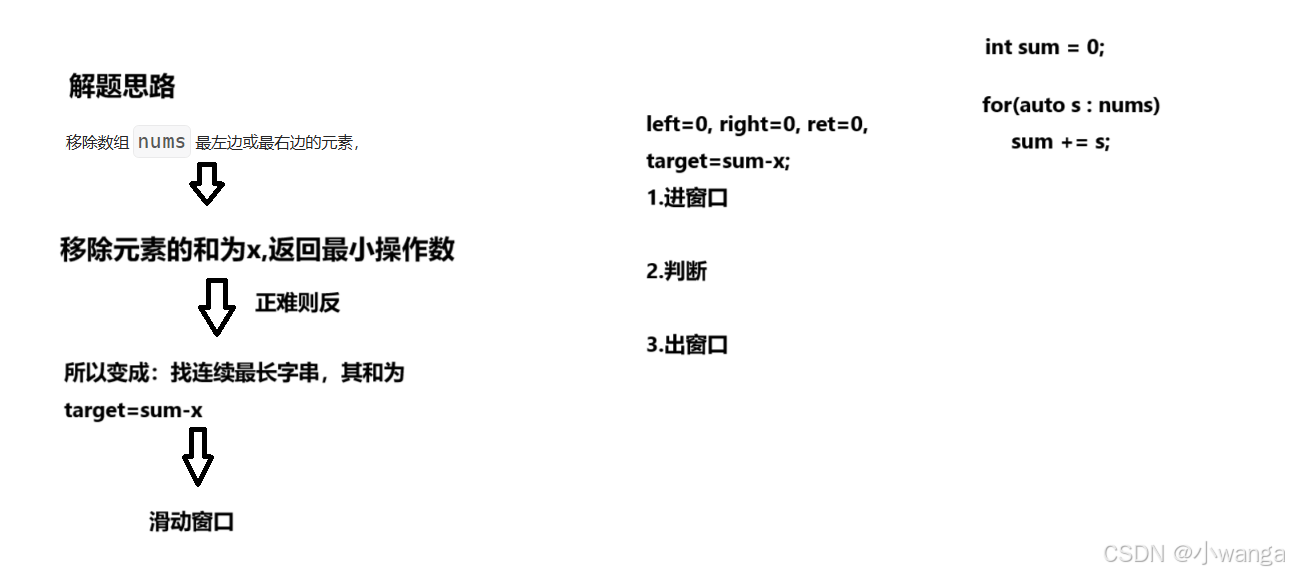
3.解题代码
cpp
class Solution {
public:
int minOperations(vector<int>& nums, int x) {
int sum = 0, ret = -1, n = nums.size();
for(int s : nums)
sum += s;
int target = sum - x;
int sum1 = 0;
//小细节,要注意
if(target < 0) return -1;
for(int left = 0, right = 0; right < n; right++)
{
//1.进窗口
sum1 += nums[right];
//2.判断 出窗口
while(sum1 > target)
sum1 -= nums[left++];
//3.判断 更新ret
if(sum1 == target) ret = max(ret, right - left + 1);
}
//输出时也要注意
if(ret == -1) return ret;
else return n - ret;
}
};
5.水果成篮
1.题目
2.解题思路
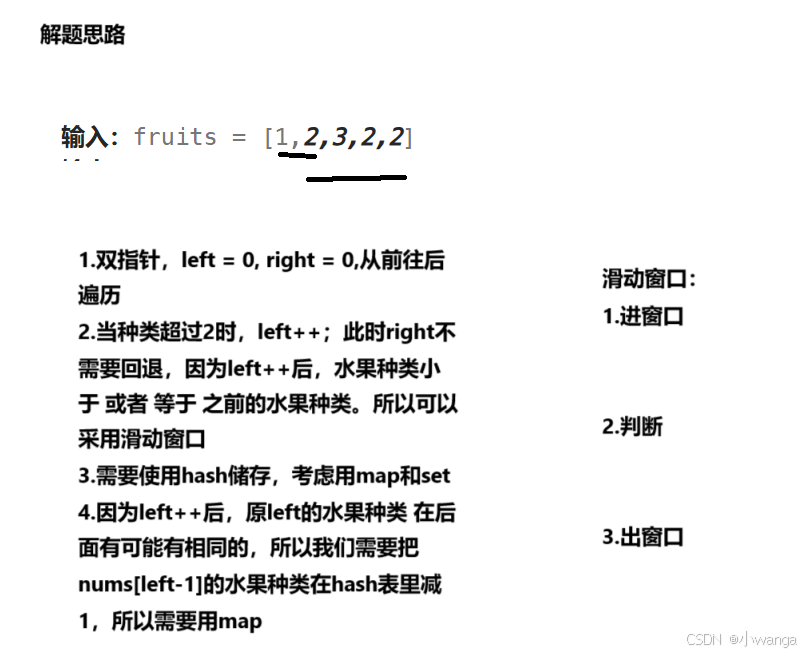
3.解题代码
cpp
class Solution {
public:
int totalFruit(vector<int>& nums) {
unordered_map<int, int> hash;
int n = nums.size(), ret = 0;
if(n == 0) return 0;
for(int left = 0, right = 0; right < n; right++)
{
hash[nums[right]]++;
while(hash.size() > 2)
{
hash[nums[left]]--;
if(hash[nums[left]] == 0)
hash.erase(nums[left]);
left++;
}
ret = max(ret, right - left + 1);
}
return ret;
}
};
优化时间复杂度
cpp
class Solution {
public:
int totalFruit(vector<int>& nums) {
//unordered_map<int, int> hash;
int hash[100001] = {0};
int n = nums.size(), ret = 0;
if(n == 0) return 0;
for(int left = 0, right = 0,kinds = 0; right < n; right++)
{
if(hash[nums[right]] == 0) kinds++;
hash[nums[right]]++;
while(kinds > 2)
{
hash[nums[left]]--;
if(hash[nums[left]] == 0)
kinds--;
left++;
}
ret = max(ret, right - left + 1);
}
return ret;
}
};
6.找到字符串中所有字母异位词
1.题目
2.解题思路
3.解题代码
cpp
//完全理解题意和思路后 写出来的代码
class Solution {
public:
vector<int> findAnagrams(string s, string p) {
int m = p.size(), n = s.size(), hash1[26] = {0}, hash2[26] = {0};
vector<int> ret;
for(auto c : p) hash2[c - 'a']++;
for(int left = 0, right = 0, count = 0; right < n; right++)
{
hash1[s[right] - 'a']++;
if(hash1[s[right] - 'a'] <= hash2[s[right] - 'a']) count++;
if(right - left + 1 > m)
{
if(hash1[s[left] - 'a'] <= hash2[s[left] - 'a']) count--;
hash1[s[left] - 'a']--;
left++;
}
if(count == m) ret.push_back(left);
}
return ret;
}
};
cpp
//第一次看题解写出来的代码,当时不是很懂
class Solution {
public:
vector<int> findAnagrams(string s, string p) {
vector<int> ans;
array<int, 26> cnt_s{};
array<int, 26> cnt_p{};
for (auto s : p)
{
cnt_p[s - 'a']++;
}
int n = s.size();
int left = 0;
for(int right = 0; right < n; right++)
{
cnt_s[s[right] - 'a']++;
if (right - left + 1 < p.length())
continue;
if (cnt_s == cnt_p)
ans.push_back(left);
cnt_s[s[left] - 'a']--;
left++;
}
return ans;
}
};
7.串联所有单词的字串
1.题目
2.解题思路
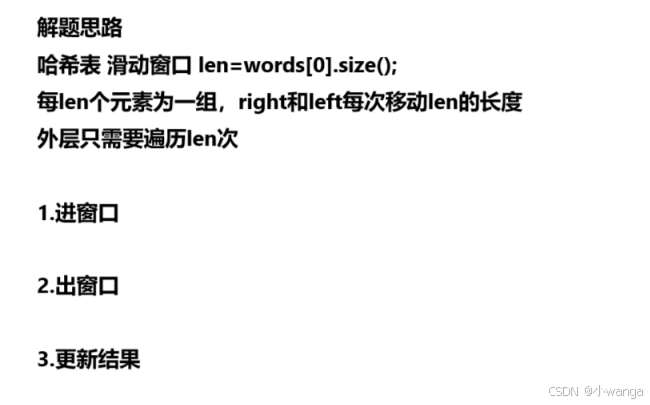
3.解题代码
cpp
class Solution {
public:
vector<int> findSubstring(string s, vector<string>& words) {
vector<int> ret;
unordered_map<string, int> hash1;
int n = s.size(), m = words.size(), len = words[0].size();
for(auto& ss : words)
{
hash1[ss]++;
}
for(int i = 0; i < len; i++)
{
unordered_map<string, int> hash2;
for(int left = i, right = i, count = 0; right + len <= n; right += len)
{
string in = s.substr(right, len);
hash2[in]++;
if(hash1.count(in) && hash2[in] <= hash1[in]) count++;
if(right - left + 1 > len * m)
{
string out = s.substr(left, len);
if(hash1.count(out) && hash2[out] <= hash1[out]) count--;
left += len;
hash2[out]--;
}
if(count == m) ret.push_back(left);
}
}
return ret;
}
};
8.最小覆盖字串
1.题目
2.解题思路
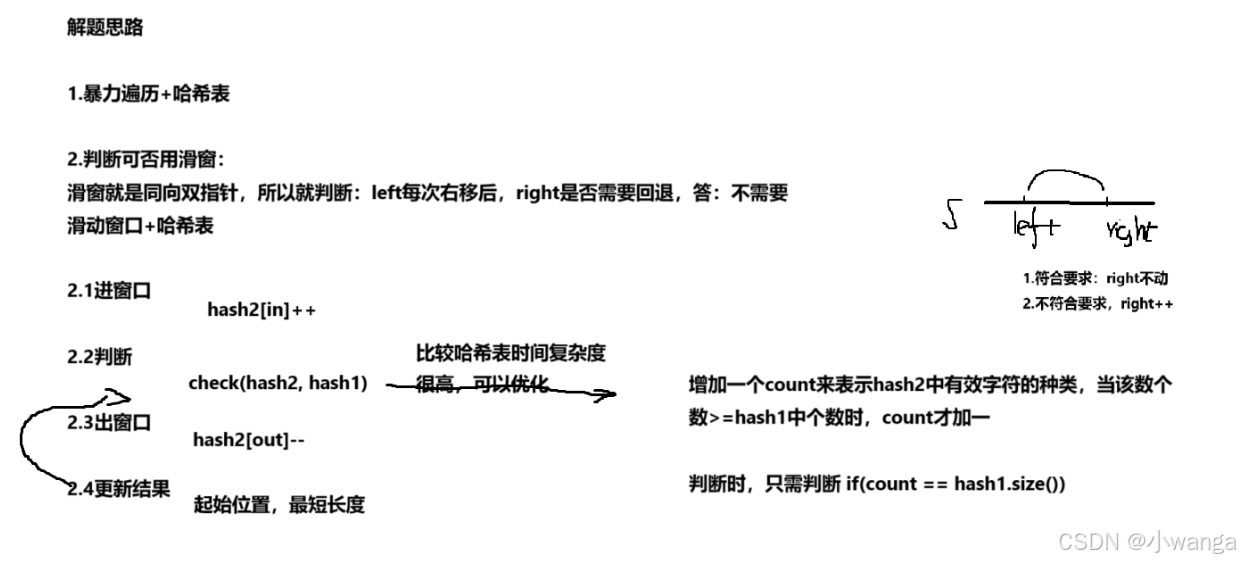
3.解题代码
cpp
class Solution {
public:
string minWindow(string s, string t) {
unordered_map<char, int> hash1, hash2;
int n = s.size();
for(auto ss : t) hash1[ss]++;
int m = hash1.size(), pos = 0, length = 100001;
for(int left = 0, right = 0, count = 0; right < n; right++)
{
char in = s[right];
hash2[in]++;
if(hash1.count(in) && hash2[in] == hash1[in]) count++;
while(count == m)
{
if(length > right - left + 1)
{
length = right - left + 1;
pos = left;
}
char out = s[left];
if(hash1.count(out) && hash2[out] == hash1[out]) count--;
hash2[out]--;
left++;
}
}
string ret = s.substr(pos, length);
if(length == 100001) ret = "";
return ret;
}
};