发送简单邮件+模版邮件
1.pom引入依赖
XML
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
<version>2.5.13</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
2.配置yaml文件
java
mail:
host: smtp.qq.com
port: 465 # 必须改用SSL专用端口
username: 配置发件人的邮箱
password: 发件人的秘钥不是登录密码
properties:
mail:
smtp:
auth: true
ssl:
enable: true # 显式启用SSL
socketFactory:
class: javax.net.ssl.SSLSocketFactory # 指定SSL工厂
starttls:
enable: false # 禁用STARTTLS(与SSL互斥)
3.代码
java
@GetMapping("/sendSimpleMail")
public Result sendMail() {
String recipientStr ="收件人邮箱";
SendMailParam sendMailParam = new SendMailParam();
sendMailParam.setContent("你好,现在是 "+System.currentTimeMillis()+",邮件发送成功!");
mailUtil.sendSimpleMessage(recipientStr, "测试邮件主题", sendMailParam, "");
return Result.ok();
}
@GetMapping("/sendTemplateMail")
public Result sendTemplateMail() {
String recipientStr ="收件人邮箱";
mailUtil.sendThemplateMessage(recipientStr,"测试邮件主题");
return Result.ok();
}
java
import com.hmdp.config.MailConfig;
import com.hmdp.entity.SendMailParam;
import com.hmdp.service.ISendMailService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.javamail.JavaMailSenderImpl;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import org.springframework.util.StringUtils;
import org.thymeleaf.TemplateEngine;
import org.thymeleaf.context.Context;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import java.io.*;
/**
* @author don
* @version 1.0.0
* @ClassName SendMailServiceImpl.java
* @Description TODO
* @createTime 2024年03月06日 17:38:00
*/
@Service
@Slf4j
public class SendMailServiceImpl implements ISendMailService {
@Autowired
private JavaMailSenderImpl mailSender;
@Autowired
private TemplateEngine templateEngine;
@Value("${spring.mail.username}")
private String from;
@Override
public void sendSimpleMessage(String to, String subject, SendMailParam sendMailParam, String fileList) {
try {
MimeMessage mailMessage=mailSender.createMimeMessage();
MimeMessageHelper helper=new MimeMessageHelper(mailMessage,true);
//设置寄件人
helper.setFrom(from);
//设置邮件标题
helper.setSubject(subject);
//设置邮件内容
helper.setText(sendMailParam.getContent());
//设置收件人
helper.setTo(to);
// 发送邮件
mailSender.send(mailMessage);
} catch (MessagingException e) {
e.printStackTrace();
}
}
@Override
public void sendThemplateMessage(String to, String subject) {
try {
MimeMessage mailMessage = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mailMessage, true);
//设置寄件人
helper.setFrom(from);
//设置邮件标题
helper.setSubject(subject);
//设置收件人
helper.setTo(to);
//设置模板
//thymeleaf模版解析成String
Context ctx = new Context();
ctx.setVariable("mailSubject", "测试主题");
ctx.setVariable("noticeTime", "2025-03-18");
ctx.setVariable("noticeNumber", "ASDF456");
ctx.setVariable("name", "张三");
ctx.setVariable("billDate", "2025-03-18");
ctx.setVariable("groupCode", "45");
ctx.setVariable("creator", "admin");
ctx.setVariable("content", "hello world !");
//模板填充数据
String htmlContent = templateEngine.process("data", ctx);
helper.setText(htmlContent, true);
// 发送邮件
mailSender.send(mailMessage);
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
4.模板位置及内容
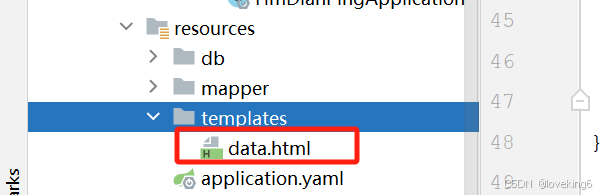
html
<!DOCTYPE html>
<html xmlns:th="http://www.w3.org/1999/xhtml">
<head>
<meta charset="utf-8">
<title>表格</title>
</head>
<body>
<table border="1">
<tr>
<td><h4>邮件主题</h4></td>
<td colspan="5">
<a th:href="@{http://ip/}" th:text="${mailSubject}"> </a>
</td>
</tr>
<tr>
<td><h4>通知时间</h4></td>
<td colspan="5" th:text="${noticeTime}"></td>
</tr>
<tr>
<td><h4>通知类型</h4></td>
<td colspan="3">
<span th:text="${noticeText}" th:style="${noticeText == '正常通知' ? 'color: green;' : 'color: red;' }"></span>
</td>
<td><h4>任务编号</h4></td>
<td colspan="3">
<span style="color: red;"><span th:text="${noticeNumber}"></span></span>
</td>
</tr>
<tr>
<td><h4>姓名</h4></td>
<td colspan="3" th:text="${name}">
</td>
<td><h4>日期</h4></td>
<td colspan="3" th:text="${billDate}">
</td>
</tr>
<tr>代码</h4></td>
<td colspan="3" th:text="${groupCode}">
</td>
<td><h4>创建者</h4></td>
<td colspan="3" th:text="${creator}">
</td>
</tr>
<tr>
<td><h4>通知内容</h4></td>
<td colspan="5">
<div>
<span th:utext="${content}"></span>
</div>
</td>
</tr>
</table>
</body>
</html>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
div {
margin-top:10px;
}
.icon {
text-align: center;
display: inline-block;
width: 20px;
height: 20px;
background-color: yellowgreen;
border-radius: 50%;
color: #fff;
margin-right: 4px;
font-weight: bold;
}
.icon.success {
background-color: yellowgreen;
line-height: 22px;
}
.icon.error {
background-color: red;
line-height: 18px;
}
td {
padding: 0px 10px;
}
</style>
5.效果示例
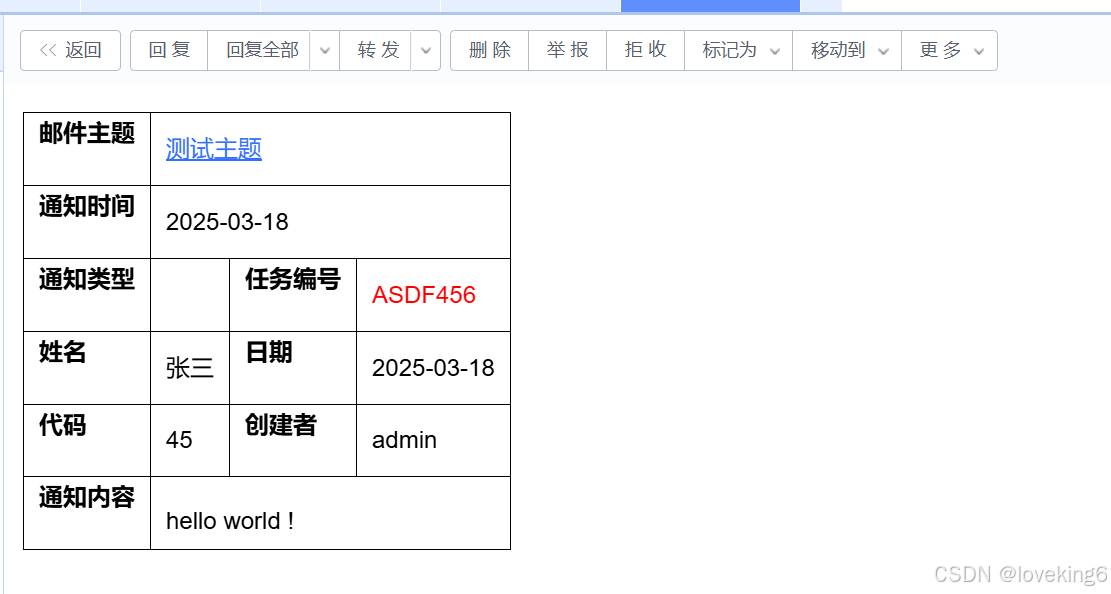
6.模版邮件注意事项
代码里的字段要和模板里的字段对应上