题目来自洛谷网站:
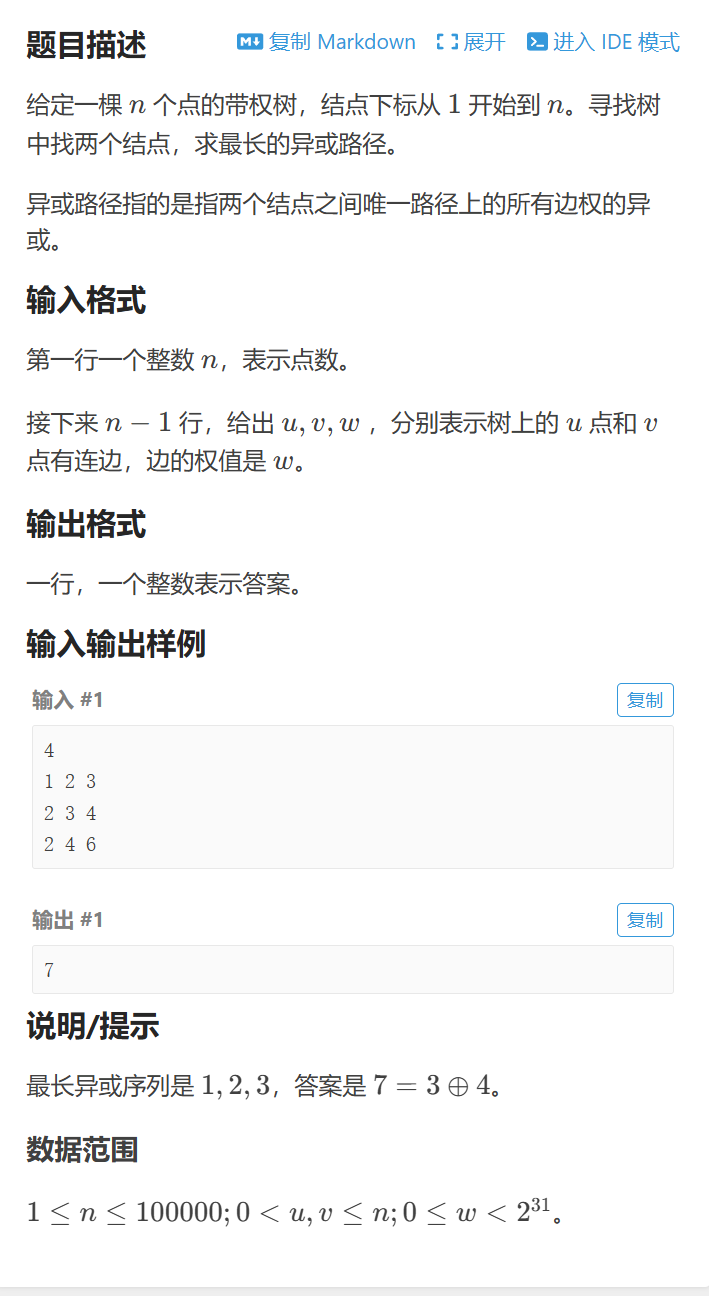
思路:
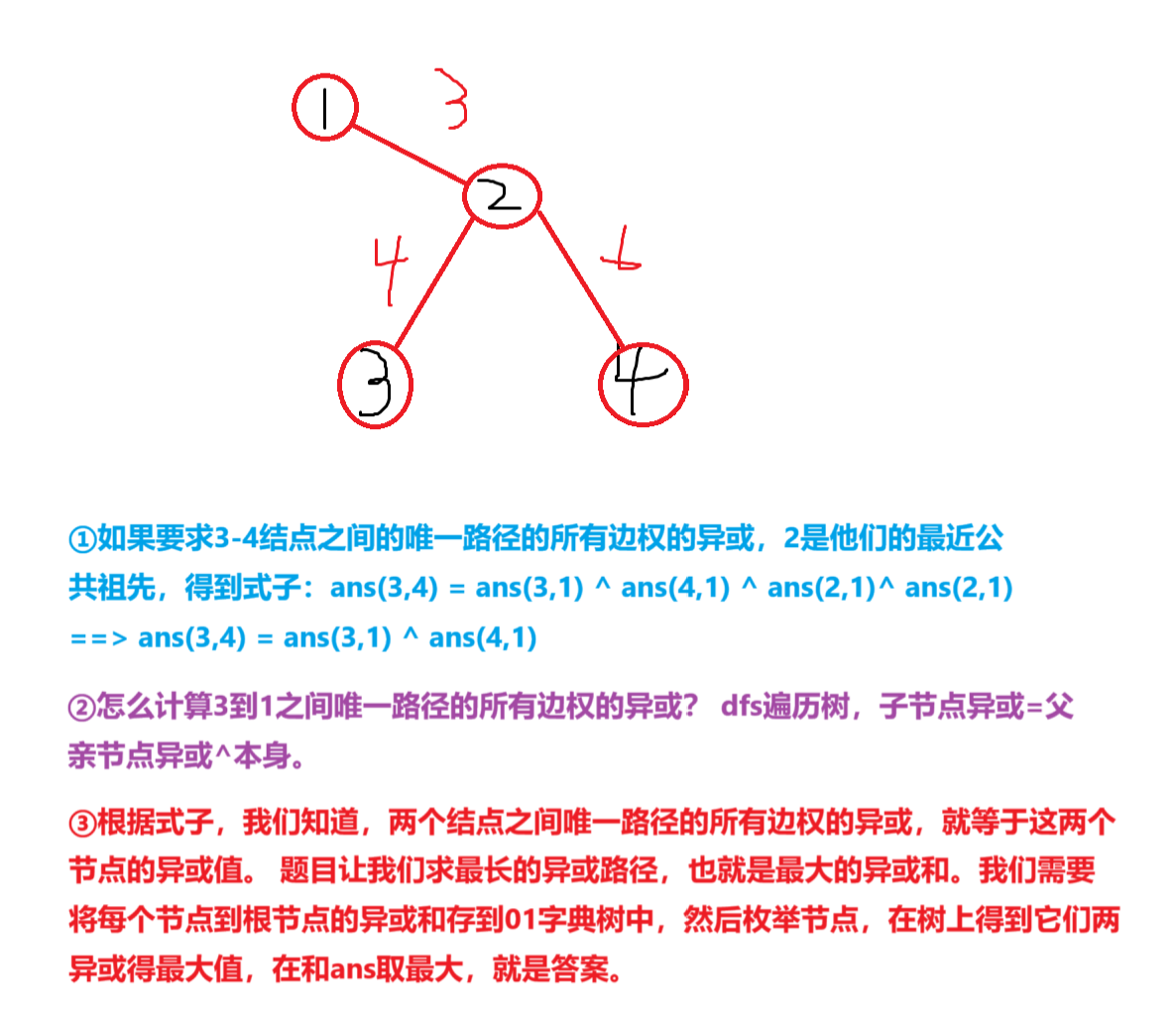
代码:
cpp
#include<bits/stdc++.h>
#define int long long
using namespace std;
const int N = 100010;
int n;
//存树
typedef pair<int, int> PII;
vector<PII> t[N];
//记录这个点到根节点的异或和
int ls[N];
//01字典树
int ch[N*31][2], idx;
//找到每个点到起点这一段的异或和
void dfs(int u, int father){
for(auto [v, w]: t[u]){
//判断子节点是不是父亲节点
if(v == father) continue;
//该点到起点 异或和
ls[v] = ls[u] ^ w;
dfs(v, u);
}
}
void insert(int x){
int p = 0;
for(int i = 30; i >= 0; i--){
int j = x >> i & 1;
if(!ch[p][j]) ch[p][j] = ++idx;
p = ch[p][j];
}
}
//在树中查找1个节点和另1个节点的异或最大值
//返回的结果
int query(int x){
int res = 0, p =0;
for(int i = 30; i >= 0; i--){
int j = x >> i & 1;
if(ch[p][!j]){
res += 1 << i;
p = ch[p][!j];
}
else p = ch[p][j];
}
return res;
}
signed main(){
cin >> n;
for(int i = 1; i < n; i++){
int x, y, z; cin >> x >> y >> z;
//无向边
t[x].push_back({y,z});
t[y].push_back({x,z});
}
//找到每个点到起点这一段的异或和
//节点从1开始的
dfs(1,0);
//将各点到起点的异或和 存到01字典树中
for(int i = 1;i <= n; i++) insert(ls[i]);
//枚举一个节点,在找到这个节点和树中一个节点异或的最大值
int ans = 0;
for(int i = 1; i <= n; i++){
ans = max(ans, query(ls[i]));
}
cout << ans << endl;
return 0;
}