31-40
https://leetcode.cn/studyplan/top-100-liked/
cpp
class Solution {
public:
pair<ListNode*, ListNode*> myreverse(ListNode* head, ListNode* tail){
ListNode* prev = head, *curr = head;
while(prev != tail){
ListNode* nex = curr->next;
curr->next = prev;
prev = curr;
curr = nex;
}
return {tail, head};
}
ListNode* reverseKGroup(ListNode* head, int k) {
ListNode* hair = new ListNode(0);
hair->next = head;
ListNode* pre = hair;
while(head){
ListNode* tail = pre;
for(int i = 0; i< k; i++){
tail = tail->next;
if(!tail) return hair->next;
}
ListNode* nex = tail->next;
tie(head, tail) = myreverse(head, tail);
pre->next = head;
tail->next = nex;
pre = tail;
head = pre->next;
}
return hair->next;
}
};
//31
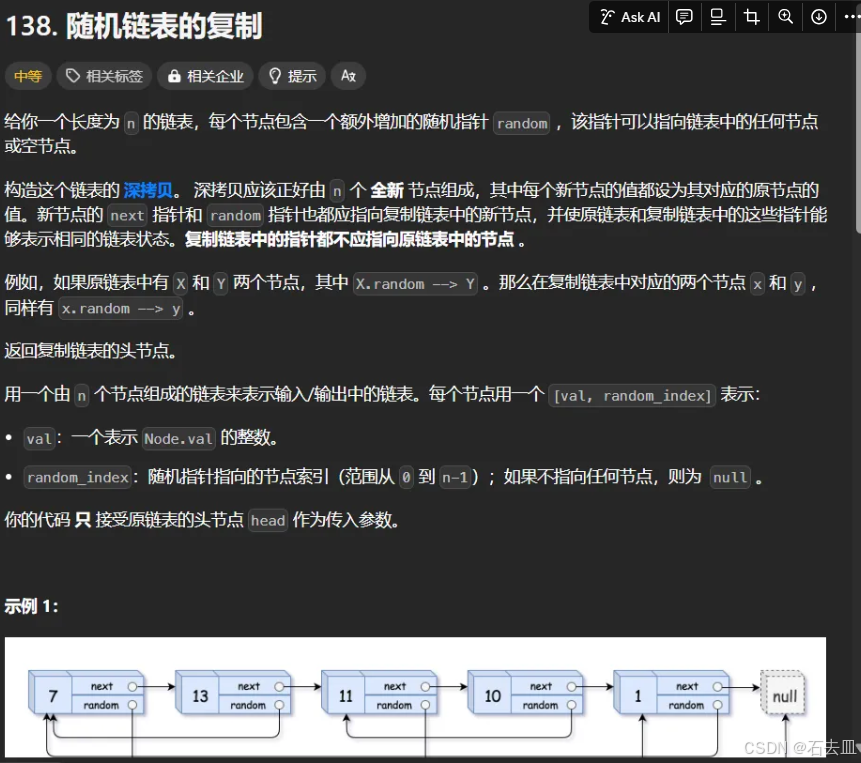
cpp
class Solution {
public:
unordered_map<Node*, Node*> cachednode;
Node* copyRandomList(Node* head) {
if(head == nullptr) return nullptr;
if(!cachednode.count(head)){
Node* headnew = new Node(head->val);
cachednode[head] = headnew;
headnew->next = copyRandomList(head->next);
headnew->random = copyRandomList(head->random);
}
return cachednode[head];
}
};
//32
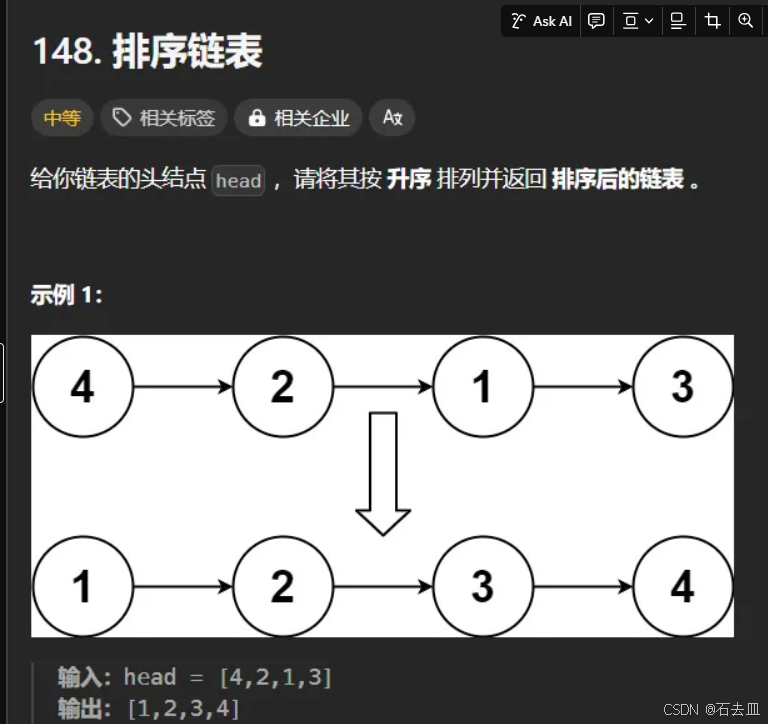
cpp
class Solution {
public:
ListNode* sortList(ListNode* head) {
auto cur = head;
int n=0;
while(cur){cur=cur->next;n++;}
ListNode* dummyHead = new ListNode(0);
dummyHead->next=head;
for(int k=1;k<n;k<<=1){
cur=dummyHead->next;
auto ans = dummyHead;
while(cur){
auto l1=cur;
auto l2=split(cur, k);
cur = split(l2, k);
ans->next=merge(l1, l2);
while(ans->next) ans=ans->next;
}
}
return dummyHead->next;
}
ListNode* split(ListNode* head, int n){
ListNode* cur = head;
while(--n&&cur){
cur=cur->next;
}
if(!cur) return nullptr;
ListNode* tmp=cur->next;
cur->next=nullptr;
return tmp;
}
ListNode* merge(ListNode* l1, ListNode* l2){
ListNode* dummyHead = new ListNode(0);
auto cur=dummyHead;
while(l1&&l2){
if(l1->val<=l2->val){
cur->next=l1;
l1=l1->next;
// cur->next->next=nullptr;
cur=cur->next;
}else{
cur->next=l2;
l2=l2->next;
// cur->next->next=nullptr;
cur=cur->next;
}
}
if(l1) cur->next=l1;
else cur->next=l2;
return dummyHead->next;
}
};
//33
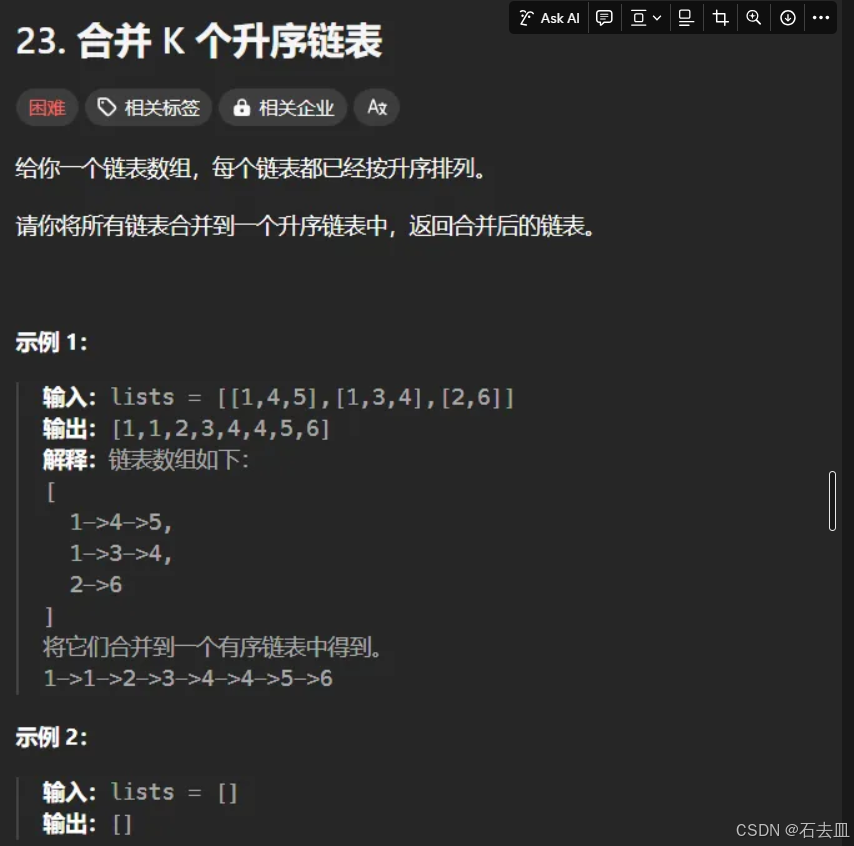
cpp
class Solution {
public:
ListNode* mergetwolsit(ListNode* list1, ListNode* list2){
ListNode* dummy = new ListNode(0);
ListNode* cur = dummy;
while(list1 && list2){
if(list1->val > list2->val){
cur->next = list2;
list2 = list2->next;
}else{
cur->next = list1;
list1 = list1->next;
}
cur = cur->next;
}
cur->next = list1 ? list1:list2;
return dummy->next;
}
ListNode* mergeKLists(vector<ListNode*>& lists) {
ListNode * ans = nullptr;
for(int i = 0; i<lists.size(); i++){
ans = mergetwolsit(ans, lists[i]);
}
return ans;
}
};
//34
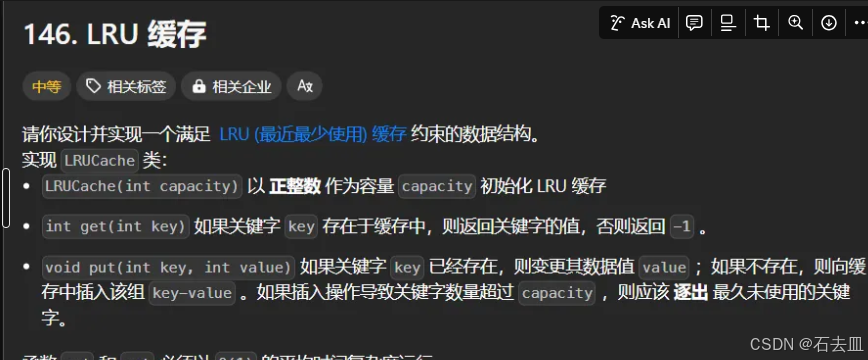
cpp
class LRUCache {
public:
int cap;
list<pair<int, int>> datalists;
unordered_map<int, list<pair<int,int>>::iterator> mp;
LRUCache(int capacity) {
cap = capacity;
}
int get(int key) {
if(mp.find(key)!=mp.end()){
int value = mp[key]->second;
datalists.erase(mp[key]);
datalists.push_front(make_pair(key, value));
// 更新哈希表中的映射
mp[key] = datalists.begin();
return value;
}else{
return -1;
}
}
void put(int key, int value) {
if(mp.find(key)!=mp.end()){
datalists.erase(mp[key]);
}else if(datalists.size() == cap){
mp.erase(datalists.back().first);
datalists.pop_back();
}
datalists.push_front(make_pair(key, value));
mp[key] = datalists.begin();
}
};
//35
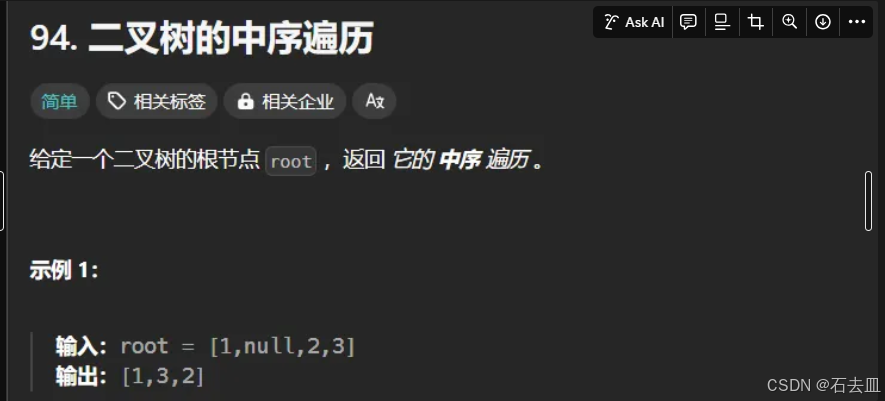
cpp
class Solution {
public:
void inorder(TreeNode* root,vector<int>& res){
if(!root) return;
inorder(root->left, res);
res.push_back(root->val);
inorder(root->right, res);
}
vector<int> inorderTraversal(TreeNode* root) {
vector<int> res;
inorder(root, res);
return res;
}
};//36
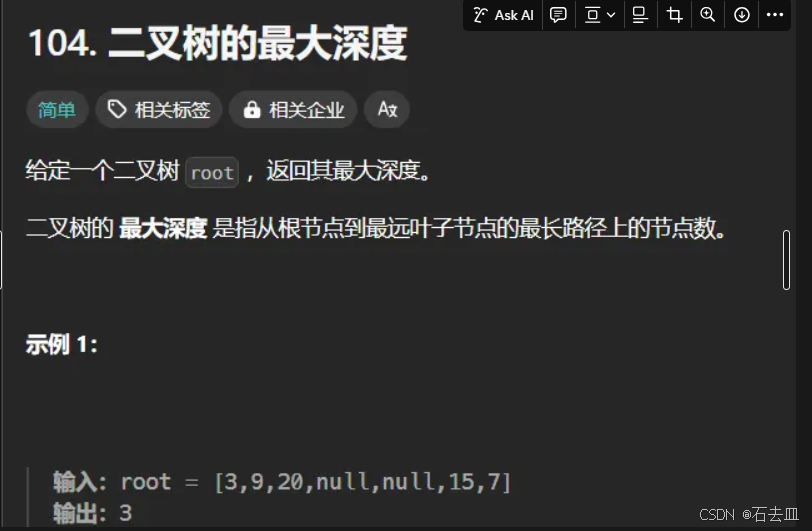
cpp
class Solution {
public:
int maxDepth(TreeNode* root) {
if(!root) return 0;
return max(maxDepth(root->left), maxDepth(root->right)) + 1;
}
};//37
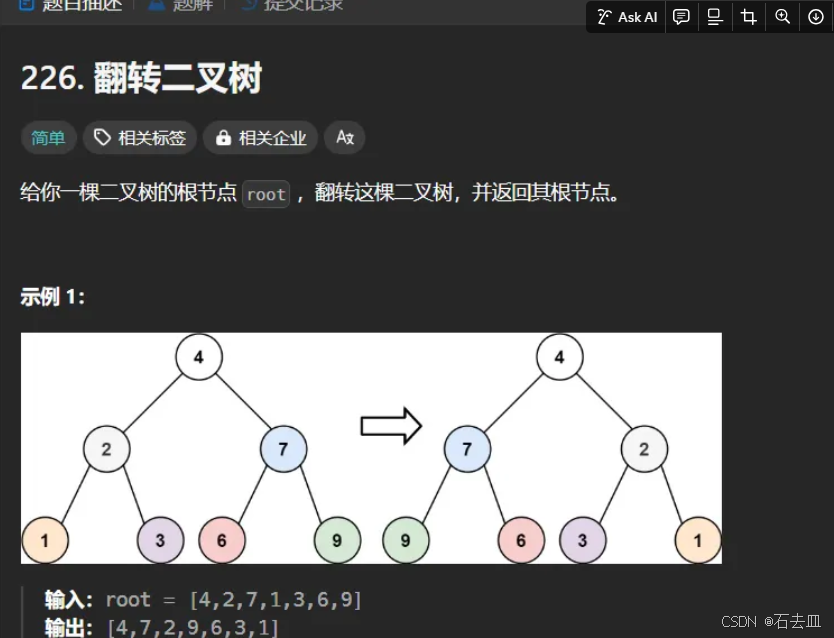
cpp
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if(!root) return root;
TreeNode* left = invertTree(root->left);
TreeNode* right = invertTree(root->right);
root->left = right;
root->right = left;
return root;
}
};//38
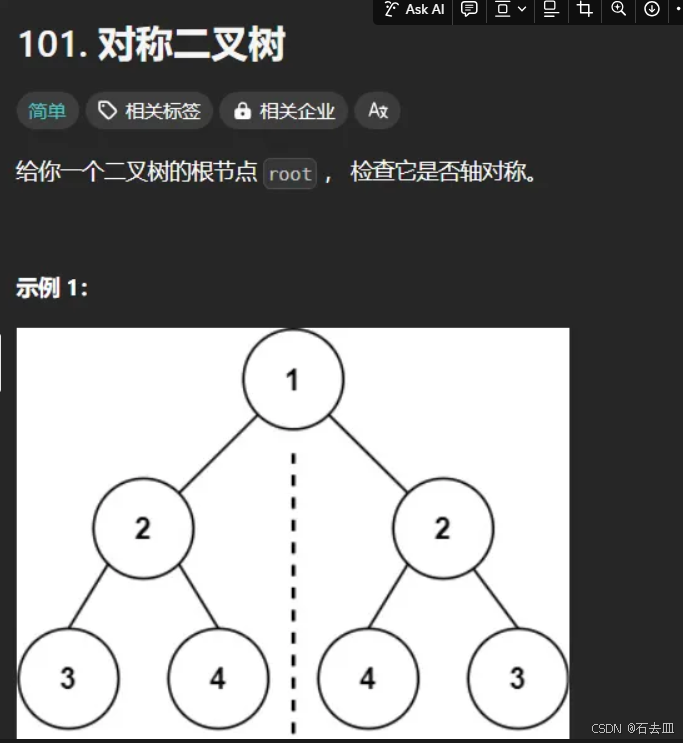
cpp
class Solution {
public:
bool check(TreeNode *p, TreeNode *q){
if(!p && !q) return true;
if(!p || !q || p->val != q->val) return false;
return check(p->left, q->right) && check(p->right, q->left);
}
bool isSymmetric(TreeNode* root) {
if(!root) return true;
return check(root->left,root->right);
}
};//39
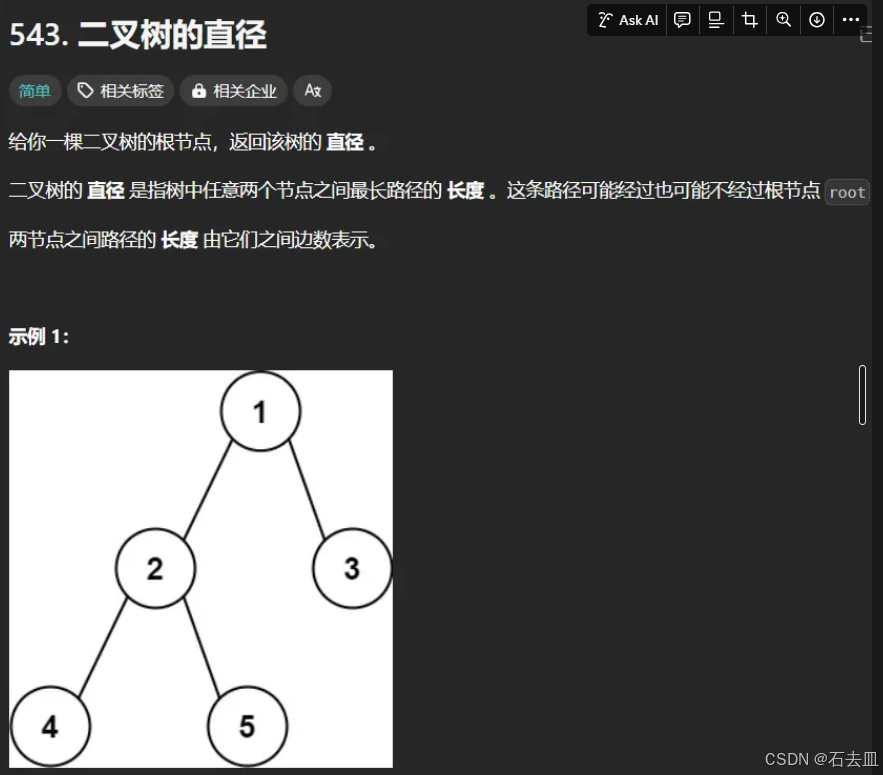
cpp
class Solution {
int ans;
int depth(TreeNode *rt){
if(!rt) return 0;
int ld = depth(rt->left);
int rd = depth(rt->right);
ans = max(ans, ld+rd+1);
return max(rd, ld)+1;
}
public:
int diameterOfBinaryTree(TreeNode* root) {
ans=1;
depth(root);
return ans-1;
}
};//40