1、目标
当从道具栏中拖出一个道具到地面的时候,光标区域会显示是否可放置物体的可视化显示。绿色表示可以放置物体,红色表示不可以放置物体。
2、优化InventoryManager脚本
添加2个方法:
cs
/// <summary>
/// Returns the itemDetails(from the SO_ItemList)for the currently selected item in the inventoryLocation,
/// or null if an item isn't selected
/// </summary>
/// <param name="inventoryLocation"></param>
/// <returns></returns>
public ItemDetails GetSelectedInventoryItemDetails(InventoryLocation inventoryLocation)
{
int itemCode = GetSelectedInventoryItem(inventoryLocation);
if(itemCode == -1)
{
return null;
}
else
{
return GetItemDetails(itemCode);
}
}
/// <summary>
/// Set the selected inventory item for inventoryLocation to itemCode
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="itemCode"></param>
public void SetSelectedInventoryItem(InventoryLocation inventoryLocation, int itemCode)
{
selectedInventoryItem[(int)inventoryLocation] = itemCode;
}
完整的代码如下:
cs
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class InventoryManager : SingletonMonobehaviour<InventoryManager>
{
private Dictionary<int, ItemDetails> itemDetailsDictionary;
private int[] selectedInventoryItem; // the index of the array is the inventory list, and the value is the item code,表明每个位置对应被选中物品的code
public List<InventoryItem>[] inventoryLists; // 每个位置的库存清单
// 每个位置的库存数。 The index of the array is the inventory list(from the
// InventoryLocation enum), and the value is the capacity of that inventory list
[HideInInspector] public int[] inventoryListCapacityIntArray;
[SerializeField] private SO_ItemList itemList = null;
protected override void Awake()
{
base.Awake();
// Create Inventory lists
CreateInventoryLists();
// Create item details dictionary
CreateItemDetailsDictionary();
// Initialize selected inventory item array
selectedInventoryItem = new int[(int)InventoryLocation.count];
for(int i = 0; i < selectedInventoryItem.Length; i++)
{
selectedInventoryItem[i] = -1;
}
}
private void CreateInventoryLists()
{
inventoryLists = new List<InventoryItem>[(int)InventoryLocation.count];
for (int i = 0; i < (int)InventoryLocation.count; i++)
{
inventoryLists[i] = new List<InventoryItem>();
}
// initialize inventory list capacity array
inventoryListCapacityIntArray = new int[(int)InventoryLocation.count];
// initialize player inventory list capacity
inventoryListCapacityIntArray[(int)InventoryLocation.player] = Settings.playerInitialInventoryCapacity;
}
/// <summary>
/// Populates the itemDetailsDictionary from the scriptable object items list
/// </summary>
private void CreateItemDetailsDictionary()
{
itemDetailsDictionary = new Dictionary<int, ItemDetails>();
foreach (ItemDetails itemDetails in itemList.itemDetails)
{
itemDetailsDictionary.Add(itemDetails.itemCode, itemDetails);
}
}
/// <summary>
/// Add an item to the inventory list for the inventoryLocation and then destroy the gameObjectToDelete
/// 角色拾取到物品后,物品需要消失掉
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="item"></param>
/// <param name="gameObjectToDelete"></param>
public void AddItem(InventoryLocation inventoryLocation, Item item, GameObject gameObjectToDelete)
{
AddItem(inventoryLocation, item);
Destroy(gameObjectToDelete);
}
/// <summary>
/// Add an item to the inventory list for the inventoryLocation
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="item"></param>
public void AddItem(InventoryLocation inventoryLocation, Item item)
{
int itemCode = item.ItemCode;
List<InventoryItem> inventoryList = inventoryLists[(int)inventoryLocation];
// Check if inventory already contains the item
int itemPosition = FindItemInInventory(inventoryLocation, itemCode);
if(itemPosition != -1)
{
AddItemPosition(inventoryList, itemCode, itemPosition);
}
else
{
AddItemPosition(inventoryList, itemCode);
}
// Send event that inventory has been updated
EventHandler.CallInventoryUpdatedEvent(inventoryLocation, inventoryLists[(int)inventoryLocation]);
}
/// <summary>
/// Add item to position in the inventory
/// </summary>
/// <param name="inventoryList"></param>
/// <param name="itemCode"></param>
/// <param name="position"></param>
/// <exception cref="NotImplementedException"></exception>
private void AddItemPosition(List<InventoryItem> inventoryList, int itemCode, int position)
{
InventoryItem inventoryItem = new InventoryItem();
int quantity = inventoryList[position].itemQuantity + 1;
inventoryItem.itemQuantity = quantity;
inventoryItem.itemCode = itemCode;
inventoryList[position] = inventoryItem;
Debug.ClearDeveloperConsole();
//DebugPrintInventoryList(inventoryList);
}
/// <summary>
/// Get the item type description for an item type - returns the item type description as a string for a given ItemType
/// </summary>
/// <param name="itemType"></param>
/// <returns></returns>
public string GetItemTypeDescription(ItemType itemType)
{
string itemTypeDescription;
switch (itemType)
{
case ItemType.Breaking_tool:
itemTypeDescription = Settings.BreakingTool;
break;
case ItemType.Chopping_tool:
itemTypeDescription = Settings.ChoppingTool;
break;
case ItemType.Hoeing_tool:
itemTypeDescription = Settings.HoeingTool;
break;
case ItemType.Reaping_tool:
itemTypeDescription = Settings.ReapingTool;
break;
case ItemType.Watering_tool:
itemTypeDescription = Settings.WateringTool;
break;
case ItemType.Collecting_tool:
itemTypeDescription = Settings.CollectingTool;
break;
default:
itemTypeDescription = itemType.ToString();
break;
}
return itemTypeDescription;
}
/// <summary>
/// Remove an item from the inventory, and create a game object at the position it was dropped
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="itemCode"></param>
public void RemoveItem(InventoryLocation inventoryLocation, int itemCode)
{
List<InventoryItem> inventoryList = inventoryLists[(int)inventoryLocation];
// Check if inventory already contains the item
int itemPosition = FindItemInInventory(inventoryLocation, itemCode);
if(itemPosition != -1)
{
RemoveItemAtPosition(inventoryList, itemCode, itemPosition);
}
// Send event that inventory has been updated
EventHandler.CallInventoryUpdatedEvent(inventoryLocation, inventoryLists[(int)inventoryLocation]);
}
private void RemoveItemAtPosition(List<InventoryItem> inventoryList, int itemCode, int position)
{
InventoryItem inventoryItem = new InventoryItem();
int quantity = inventoryList[position].itemQuantity - 1;
if(quantity > 0)
{
inventoryItem.itemQuantity = quantity;
inventoryItem.itemCode = itemCode;
inventoryList[position] = inventoryItem;
}
else
{
inventoryList.RemoveAt(position);
}
}
/// <summary>
/// Swap item at fromItem index with item at toItem index in inventoryLocation inventory list
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="fromItem"></param>
/// <param name="toItem"></param>
public void SwapInventoryItems(InventoryLocation inventoryLocation, int fromItem, int toItem)
{
// if fromItem index and toItemIndex are within the bounds of the list, not the same, and greater than or equal to zero
if(fromItem < inventoryLists[(int)inventoryLocation].Count && toItem < inventoryLists[(int)inventoryLocation].Count
&& fromItem != toItem && fromItem >= 0 && toItem >= 0)
{
InventoryItem fromInventoryItem = inventoryLists[(int)inventoryLocation][fromItem];
InventoryItem toInventoryItem = inventoryLists[(int)inventoryLocation][toItem];
inventoryLists[(int)inventoryLocation][toItem] = fromInventoryItem;
inventoryLists[(int)inventoryLocation][fromItem] = toInventoryItem;
// Send event that inventory has been updated
EventHandler.CallInventoryUpdatedEvent(inventoryLocation, inventoryLists[(int)inventoryLocation]);
}
}
private void DebugPrintInventoryList(List<InventoryItem> inventoryList)
{
foreach(InventoryItem inventoryItem in inventoryList)
{
Debug.Log("Item Description:" + InventoryManager.Instance.GetItemDetails(inventoryItem.itemCode).itemDescription + " Item Quantity:" + inventoryItem.itemQuantity);
}
Debug.Log("*******************************************************************************");
}
/// <summary>
/// Add item to the end of the inventory
/// </summary>
/// <param name="inventoryList"></param>
/// <param name="itemCode"></param>
/// <exception cref="NotImplementedException"></exception>
private void AddItemPosition(List<InventoryItem> inventoryList, int itemCode)
{
InventoryItem inventoryItem = new InventoryItem();
inventoryItem.itemCode = itemCode;
inventoryItem.itemQuantity = 1;
inventoryList.Add(inventoryItem);
//DebugPrintInventoryList(inventoryList);
}
/// <summary>
/// Find if an itemCode is already in the inventory. Returns the item position
/// in the inventory list, or -1 if the item is not in the inventory
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="itemCode"></param>
/// <returns></returns>
/// <exception cref="NotImplementedException"></exception>
public int FindItemInInventory(InventoryLocation inventoryLocation, int itemCode)
{
List<InventoryItem> inventoryList = inventoryLists[(int)inventoryLocation];
for (int i = 0; i < inventoryList.Count; i++)
{
if(inventoryList[i].itemCode == itemCode)
{
return i;
}
}
return -1;
}
/// <summary>
/// Returns the itemDetails (from the SO_ItemList) for the itemCode, or null if the item doesn't exist
/// </summary>
/// <param name="itemCode"></param>
/// <returns></returns>
public ItemDetails GetItemDetails(int itemCode)
{
ItemDetails itemDetails;
if(itemDetailsDictionary.TryGetValue(itemCode, out itemDetails))
{
return itemDetails;
}
else
{
return null;
}
}
/// <summary>
/// Get the selected item for inventoryLocation -- returns itemCode for -1 if nothing is selected
/// </summary>
/// <param name="inventoryLocation"></param>
/// <returns></returns>
private int GetSelectedInventoryItem(InventoryLocation inventoryLocation)
{
return selectedInventoryItem[(int)inventoryLocation];
}
/// <summary>
/// Returns the itemDetails(from the SO_ItemList)for the currently selected item in the inventoryLocation,
/// or null if an item isn't selected
/// </summary>
/// <param name="inventoryLocation"></param>
/// <returns></returns>
public ItemDetails GetSelectedInventoryItemDetails(InventoryLocation inventoryLocation)
{
int itemCode = GetSelectedInventoryItem(inventoryLocation);
if(itemCode == -1)
{
return null;
}
else
{
return GetItemDetails(itemCode);
}
}
/// <summary>
/// Set the selected inventory item for inventoryLocation to itemCode
/// </summary>
/// <param name="inventoryLocation"></param>
/// <param name="itemCode"></param>
public void SetSelectedInventoryItem(InventoryLocation inventoryLocation, int itemCode)
{
selectedInventoryItem[(int)inventoryLocation] = itemCode;
}
/// <summary>
/// Clear the selected inventory item for inventoryLocation
/// </summary>
/// <param name="inventoryLocation"></param>
public void ClearSelectedInventoryItem(InventoryLocation inventoryLocation)
{
selectedInventoryItem[(int)inventoryLocation] = -1;
}
}
3、创建GridCursor脚本
在Assets -> Scripts -> UI 下创建脚本命名为GridCursor。
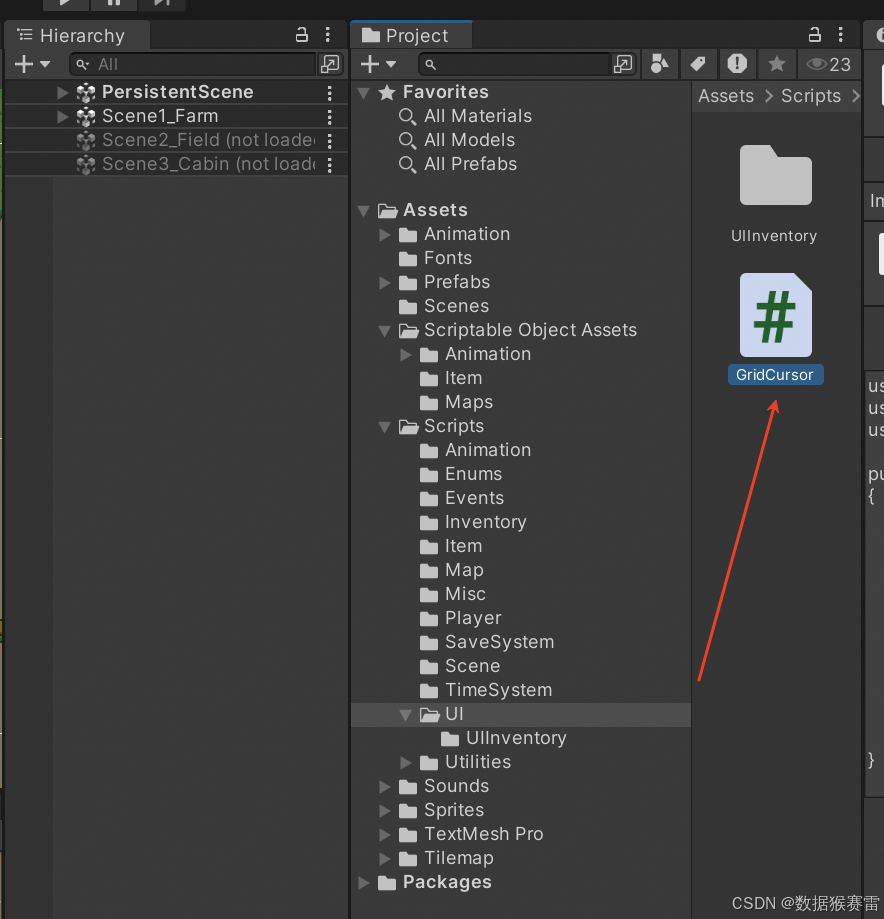
完整代码如下:
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GridCursor : MonoBehaviour
{
private Canvas canvas; // 存储白色画布,UI光标位于其中
private Grid grid; // tilemap地图
private Camera mainCamera; // 对主相机的引用
[SerializeField] private Image cursorImage = null;
[SerializeField] private RectTransform cursorRectTransform = null; // 光标对象的引用
[SerializeField] private Sprite greenCursorSprite = null;
[SerializeField] private Sprite redCursorSprite = null;
private bool _cursorPositionIsValid = false;
public bool CursorPositionIsValid { get => _cursorPositionIsValid; set => _cursorPositionIsValid = value; }
private int _itemUseGridRadius = 0;
public int ItemUseGridRadius { get => _itemUseGridRadius; set => _itemUseGridRadius = value; }
private ItemType _selectedItemType;
public ItemType SelectedItemType { get => _selectedItemType; set => _selectedItemType = value; }
private bool _cursorIsEnabled = false;
public bool CursorIsEnabled { get => _cursorIsEnabled; set => _cursorIsEnabled = value; }
private void OnDisable()
{
EventHandler.AfterSceneLoadEvent -= SceneLoaded;
}
private void OnEnable()
{
EventHandler.AfterSceneLoadEvent += SceneLoaded;
}
// Start is called before the first frame update
void Start()
{
mainCamera = Camera.main;
canvas = GetComponentInParent<Canvas>();
}
// Update is called once per frame
void Update()
{
if (CursorIsEnabled)
{
DisplayCursor();
}
}
private Vector3Int DisplayCursor()
{
if (grid != null)
{
// 之所以需要Grid是因为某些定位是基于grid的
// Get grid position for cursor
Vector3Int gridPosition = GetGridPositionForCursor();
// Get grid position for player
Vector3Int playerGridPosition = GetGridPositionForPlayer();
// Set cursor sprite,基于gridPosition和playerGridPosition设置光标的有效性
SetCursorValidity(gridPosition, playerGridPosition);
// Get rect transform position for cursor
cursorRectTransform.position = GetRectTransformPositionForCursor(gridPosition);
return gridPosition;
}
else
{
return Vector3Int.zero;
}
}
public Vector3Int GetGridPositionForCursor()
{
// z is how far the objects are in front of the camera - camera is at -10 so objects are(-)-10 in front = 10
Vector3 worldPosition = mainCamera.ScreenToWorldPoint(new Vector3(Input.mousePosition.x, Input.mousePosition.y, -mainCamera.transform.position.z));
return grid.WorldToCell(worldPosition);
}
public Vector3Int GetGridPositionForPlayer()
{
return grid.WorldToCell(Player.Instance.transform.position);
}
public Vector2 GetRectTransformPositionForCursor(Vector3Int gridPosition)
{
Vector3 gridWorldPosition = grid.CellToWorld(gridPosition);
Vector2 gridScreenPosition = mainCamera.WorldToScreenPoint(gridWorldPosition);
return RectTransformUtility.PixelAdjustPoint(gridScreenPosition, cursorRectTransform, canvas);
}
private void SceneLoaded()
{
grid = GameObject.FindObjectOfType<Grid>();
}
private void SetCursorValidity(Vector3Int cursorGridPosition, Vector3Int playerGridPosition)
{
SetCursorToValid();
// Check item use radius is valid
if(Mathf.Abs(cursorGridPosition.x - playerGridPosition.x) > ItemUseGridRadius
|| Mathf.Abs(cursorGridPosition.y - playerGridPosition.y) > ItemUseGridRadius)
{
SetCursorToInvalid();
return;
}
// Get selected item details
ItemDetails itemDetails = InventoryManager.Instance.GetSelectedInventoryItemDetails(InventoryLocation.player);
if(itemDetails == null)
{
SetCursorToInvalid();
return;
}
// Get grid property details at cursor position
GridPropertyDetails gridPropertyDetails = GridPropertiesManager.Instance.GetGridPropertyDetails(cursorGridPosition.x, cursorGridPosition.y);
if(gridPropertyDetails != null)
{
// Determine cursor validity based on inventory item selected and grid property details
switch (itemDetails.itemType)
{
case ItemType.Seed:
if (!IsCursorValidForSeed(gridPropertyDetails))
{
SetCursorToInvalid();
return;
}
break;
case ItemType.Commodity:
if (!IsCursorValidForCommodity(gridPropertyDetails))
{
SetCursorToInvalid();
return;
}
break;
case ItemType.none:
break;
case ItemType.count:
break;
default:
break;
}
}
else
{
SetCursorToInvalid();
return;
}
}
private bool IsCursorValidForSeed(GridPropertyDetails gridPropertyDetails)
{
return gridPropertyDetails.canDropItem;
}
private bool IsCursorValidForCommodity(GridPropertyDetails gridPropertyDetails)
{
return gridPropertyDetails.canDropItem;
}
private void SetCursorToValid()
{
cursorImage.sprite = greenCursorSprite;
CursorPositionIsValid = true;
}
private void SetCursorToInvalid()
{
cursorImage.sprite = redCursorSprite;
CursorPositionIsValid = false;
}
/// <summary>
/// DisableCursor is called in the UIInventorySlot.ClearCursors() method when an inventory slot item is no longer selected
/// </summary>
public void DisableCursor()
{
cursorImage.color = Color.clear;
CursorIsEnabled = false;
}
/// <summary>
/// EnableCursor is called in the UIInventorySlot.SetSelectedItem() method when an inventory slot item is selected and its itemUseGrid radius>0
/// </summary>
public void EnableCursor()
{
cursorImage.color = new Color(1f, 1f, 1f, 1f);
CursorIsEnabled = true;
}
}
4、优化UIInventorySlot脚本
增加如下变量:
cs
private GridCursor gridCursor;
在Start()中添加:
cs
gridCursor = FindObjectOfType<GridCursor>();
添加如下方法:
cs
private void ClearCursors()
{
// Disable cursor
gridCursor.DisableCursor();
// Set item type to none
gridCursor.SelectedItemType = ItemType.none;
}
在SetSelectedItem方法中添加如下方法:
cs
// Set use radius for cursors
gridCursor.ItemUseGridRadius = itemDetails.itemUseGridRadius;
// If item requires a grid cursor then enable cursor
if(itemDetails.itemUseGridRadius > 0)
{
gridCursor.EnableCursor();
}
else
{
gridCursor.DisableCursor();
}
// Set item type
gridCursor.SelectedItemType = itemDetails.itemType;
在ClearSelectedItem方法中添加:
cs
ClearCursors();
在DropSelectedItemAtMousePosition方法中,删除如下3行代码:
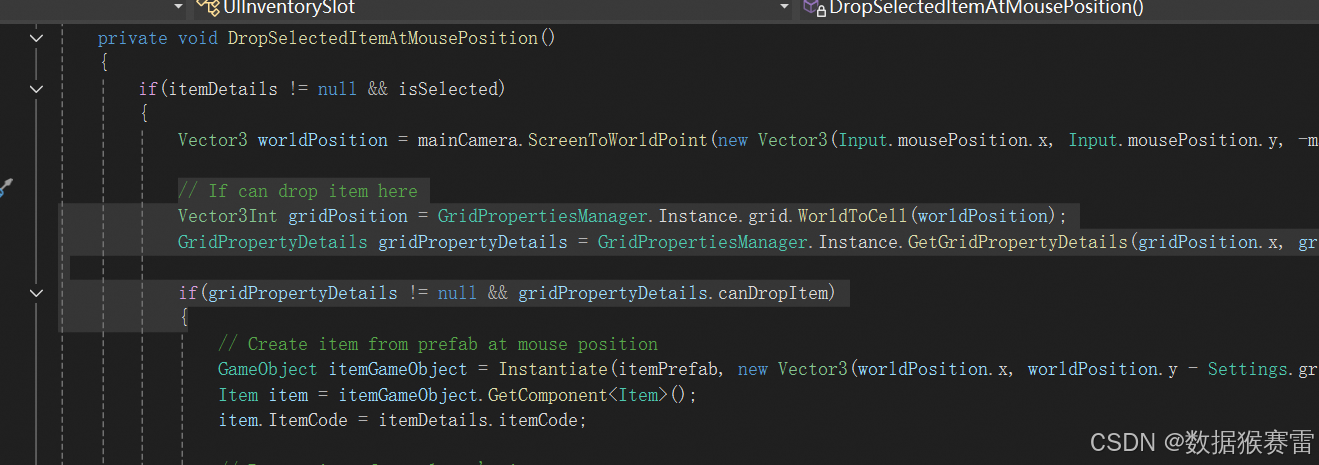
替换为:
cs
if (gridCursor.CursorPositionIsValid) {
Vector3 worldPosition = mainCamera.ScreenToWorldPoint(new Vector3(Input.mousePosition.x, Input.mousePosition.y, -mainCamera.transform.position.z));
5、创建Panel
在Hierarchy -> PersistentScene -> UI -> MainGameUICanves -> UICanvasGroup下创建新的Panel命令为UIPanel。
并且移到第一个位置。
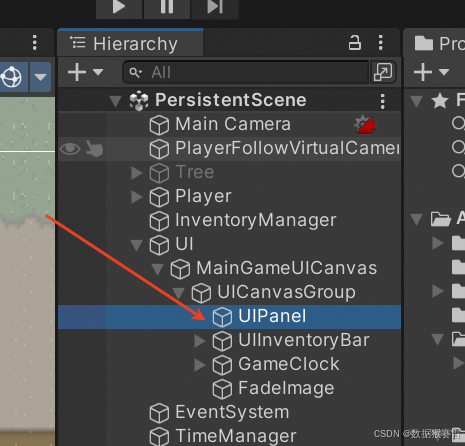
设置UIPanel的Image组件,其Source Image为None,Color的值全为0。
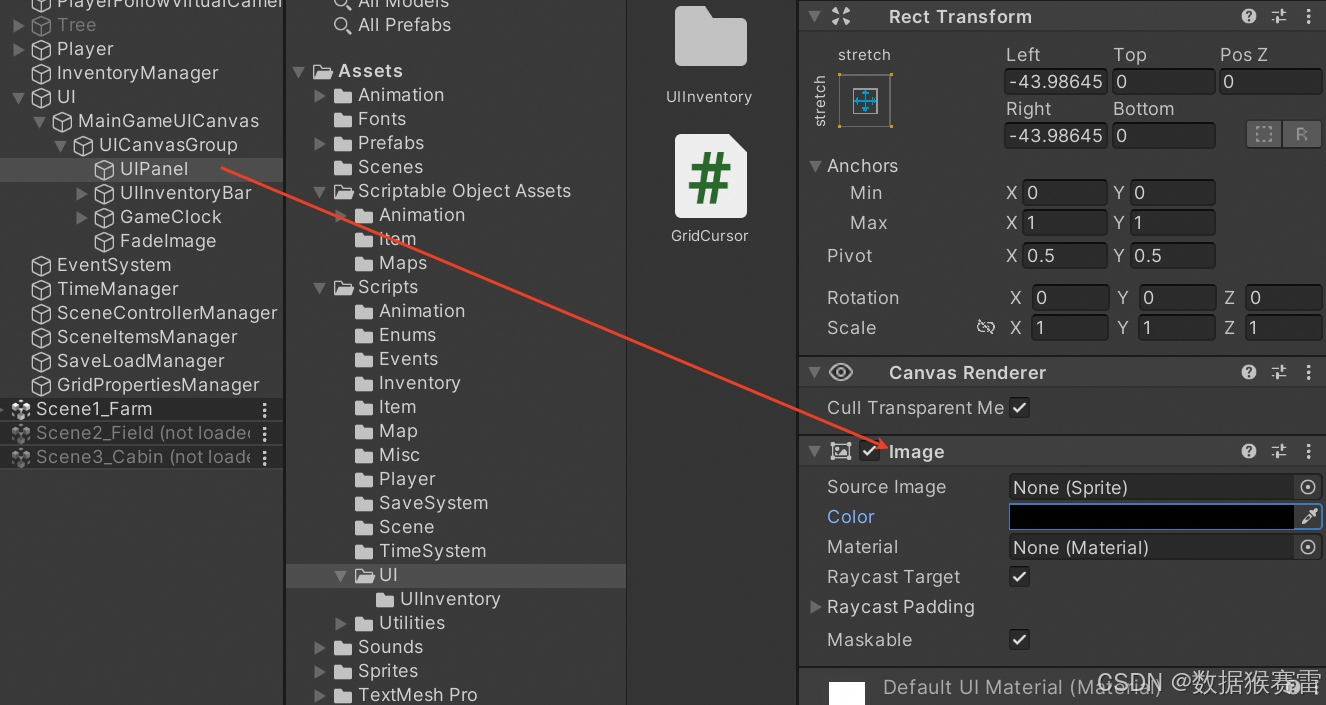
添加Canvas Group组件,反勾选Blocks Raycasts。
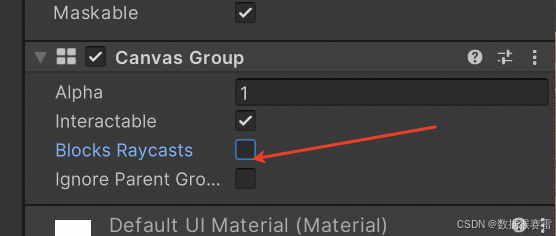
添加Grid Cursor组件:
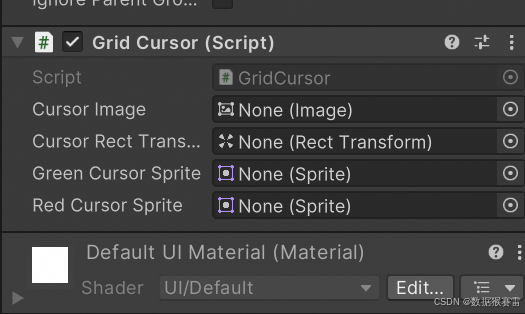
在UIPanel下创建空物体命名为GridCursor。
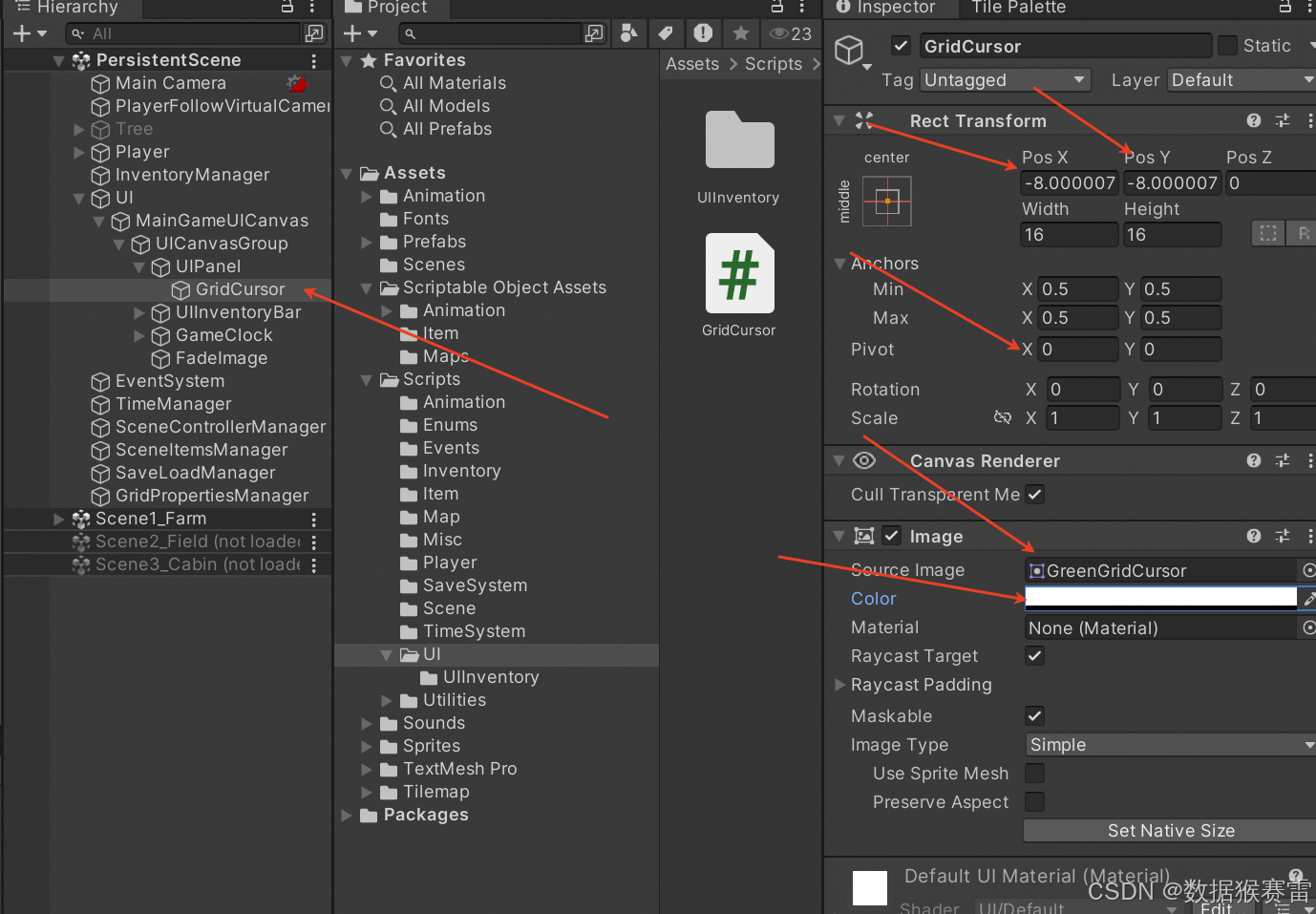
给GridCursor添加Image组件,Source Image选择"GreenGridCursor"图形,并且设置Color的透明度为0。
设置GridCursor的其他属性如上。
回到UIPanel物体,设置属性如下:
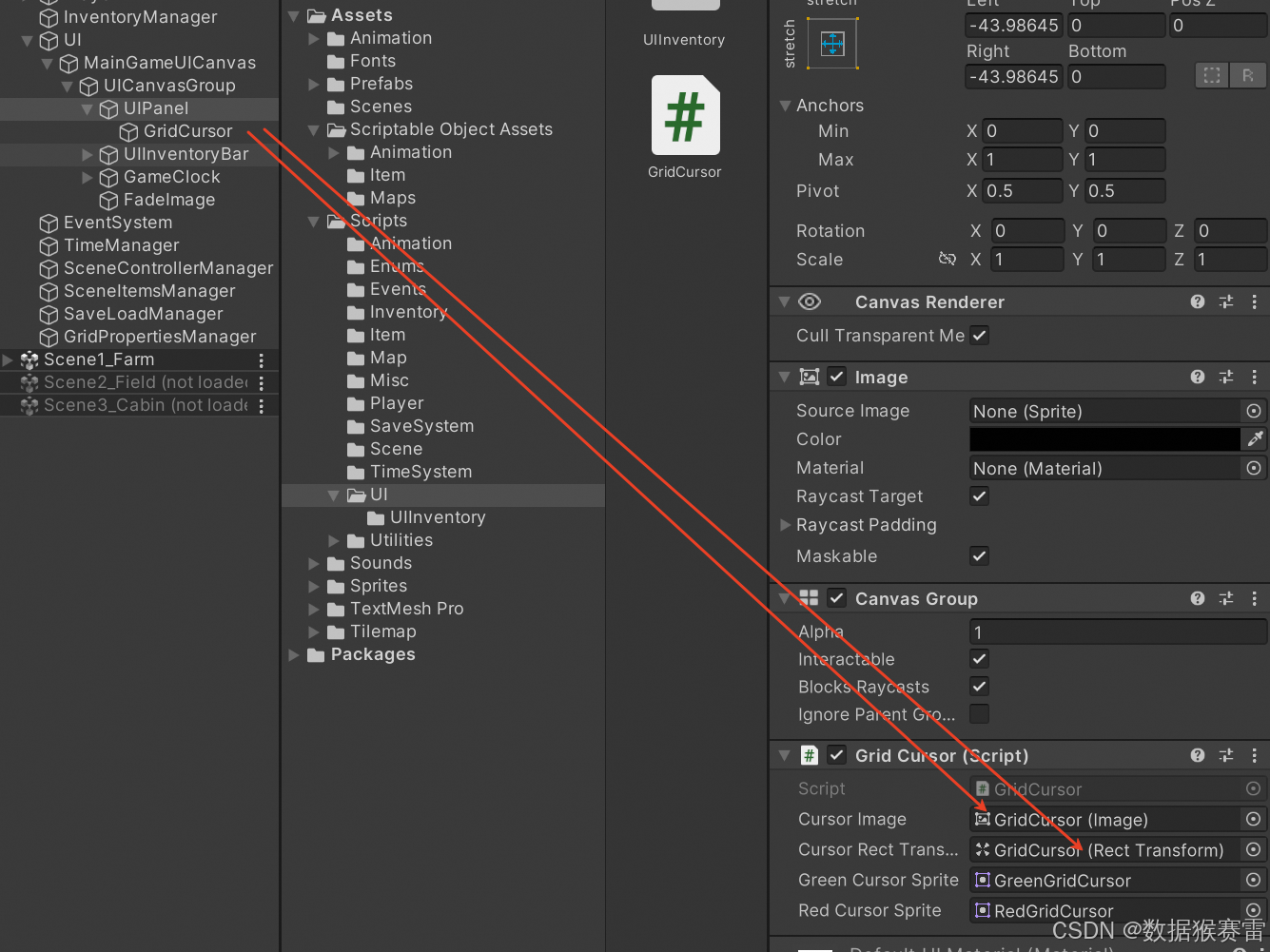
6、运行程序
不能放置的情况:
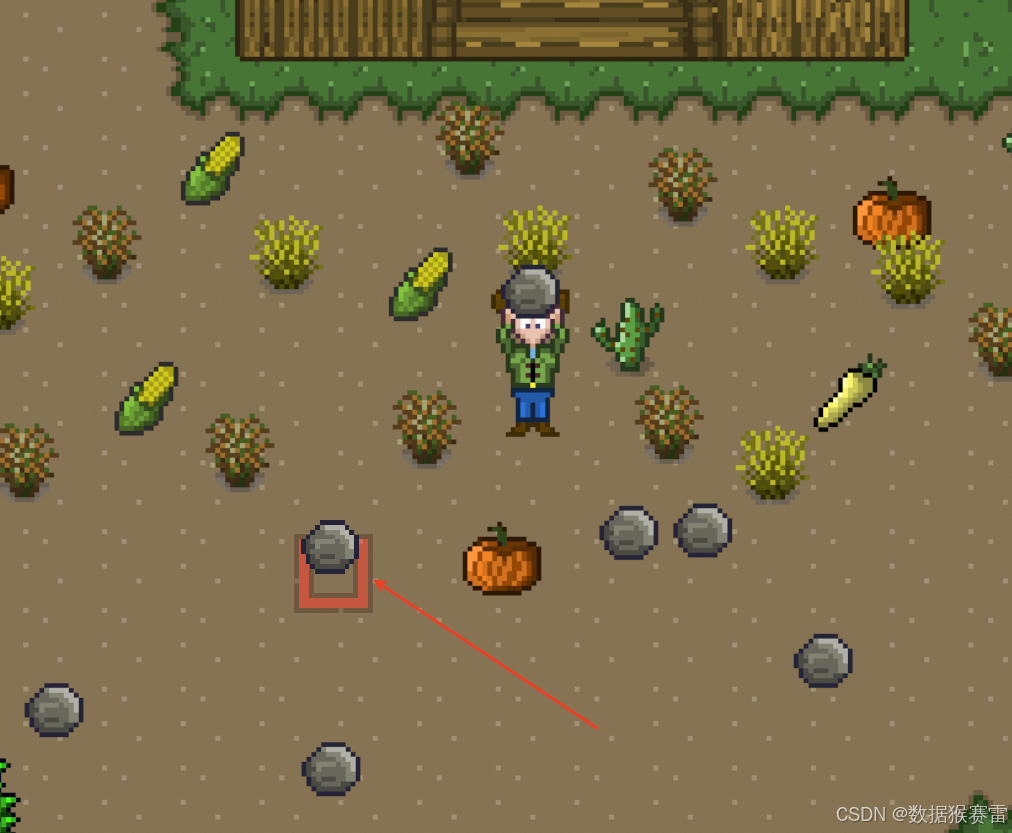
可以放置的情况:
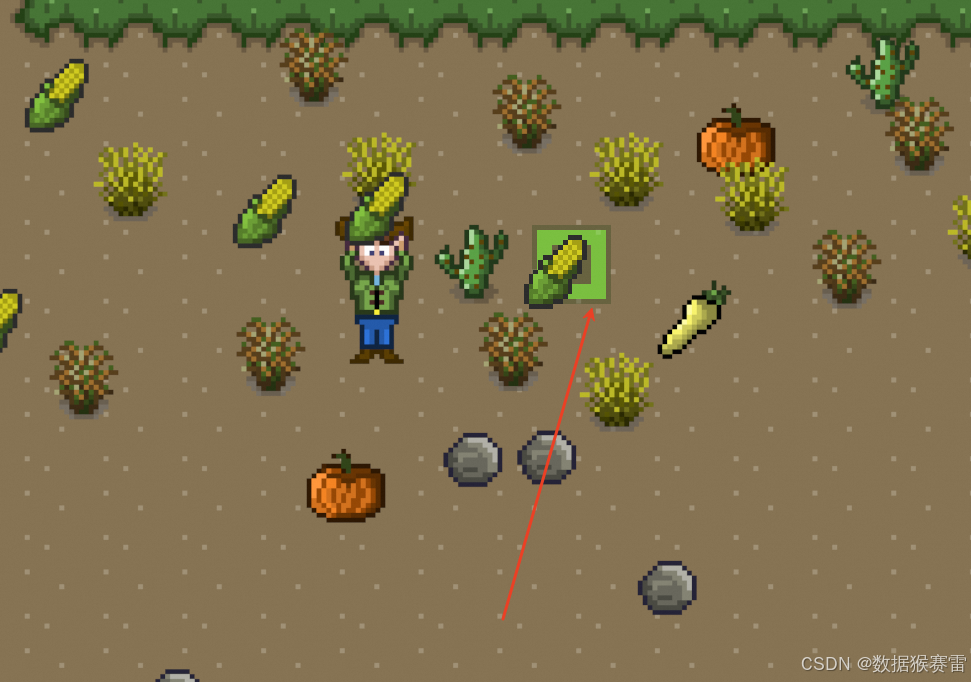