收藏关注不迷路!!
🌟文末获取源码+数据库🌟
感兴趣的可以先收藏起来,还有大家在毕设选题(免费咨询指导选题),项目以及论文编写等相关问题都可以给我留言咨询,希望帮助更多的人
文章目录
摘要
随着社会的发展,系统的管理形势越来越严峻。越来越多的用户利用互联网获得信息,但各种信息鱼龙混杂,信息真假难以辨别。为了方便用户更好的获得在线考试信息,因此,设计一种安全高效的在线考试系统极为重要。
为设计一个安全便捷,并且使用户更好获取在线考试信息,本文主要有安全、简洁为理念,实现用户快捷寻找在线考试信息,从而解决在线考试信息管理复杂难辨的问题。该系统采用java语言、Spring Boot框架和mysql数据库进行开发设计,通过对在线考试管理业务流程的分析,分析了其功能性需求,设计了在线考试系统,该系统包括管理员和教师、学生三部分。同时还能为用户提供一个方便实用的在线考试系统,使得用户能够及时地找到合适自己的在线考试系统。管理员界面管理教师、学生的信息,也可以对系统上的所有信息进行修删查等操作,用户通过本系统可以及时了解在线考试信息。
关键词:在线考试系统;java语言;mysql数据库;
一、开发技术介绍
- Java
- SpringBoot
- MySQL
- MyBatis
二、功能介绍
本次系统采用springboot框架进行开发,springboot框架是一款企业界主流的软件开发技术,其简化了开发流程,大大缩减了软件开发所需的时间提高了软件的响应速度。系统总体结构图如图4-1所示。
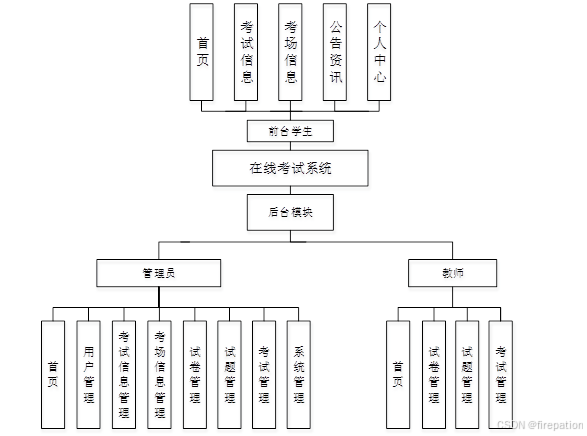
三、代码展示
java
package com.cl.controller;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.cl.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.cl.annotation.IgnoreAuth;
import com.cl.entity.JiaoshiEntity;
import com.cl.entity.view.JiaoshiView;
import com.cl.service.JiaoshiService;
import com.cl.service.TokenService;
import com.cl.utils.PageUtils;
import com.cl.utils.R;
import com.cl.utils.MPUtil;
import com.cl.utils.CommonUtil;
import java.io.IOException;
/**
* 教师
* 后端接口
* @author
* @email
*/
@RestController
@RequestMapping("/jiaoshi")
public class JiaoshiController {
@Autowired
private JiaoshiService jiaoshiService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
JiaoshiEntity u = jiaoshiService.selectOne(new EntityWrapper<JiaoshiEntity>().eq("jiaoshigonghao", username));
if(u==null || !u.getJiaoshimima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(u.getId(), username,"jiaoshi", "教师" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody JiaoshiEntity jiaoshi){
//ValidatorUtils.validateEntity(jiaoshi);
JiaoshiEntity u = jiaoshiService.selectOne(new EntityWrapper<JiaoshiEntity>().eq("jiaoshigonghao", jiaoshi.getJiaoshigonghao()));
if(u!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
jiaoshi.setId(uId);
jiaoshiService.insert(jiaoshi);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
JiaoshiEntity u = jiaoshiService.selectById(id);
return R.ok().put("data", u);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
JiaoshiEntity u = jiaoshiService.selectOne(new EntityWrapper<JiaoshiEntity>().eq("jiaoshigonghao", username));
if(u==null) {
return R.error("账号不存在");
}
u.setJiaoshimima("123456");
jiaoshiService.updateById(u);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,JiaoshiEntity jiaoshi,
HttpServletRequest request){
EntityWrapper<JiaoshiEntity> ew = new EntityWrapper<JiaoshiEntity>();
PageUtils page = jiaoshiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, jiaoshi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,JiaoshiEntity jiaoshi,
HttpServletRequest request){
EntityWrapper<JiaoshiEntity> ew = new EntityWrapper<JiaoshiEntity>();
PageUtils page = jiaoshiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, jiaoshi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( JiaoshiEntity jiaoshi){
EntityWrapper<JiaoshiEntity> ew = new EntityWrapper<JiaoshiEntity>();
ew.allEq(MPUtil.allEQMapPre( jiaoshi, "jiaoshi"));
return R.ok().put("data", jiaoshiService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(JiaoshiEntity jiaoshi){
EntityWrapper< JiaoshiEntity> ew = new EntityWrapper< JiaoshiEntity>();
ew.allEq(MPUtil.allEQMapPre( jiaoshi, "jiaoshi"));
JiaoshiView jiaoshiView = jiaoshiService.selectView(ew);
return R.ok("查询教师成功").put("data", jiaoshiView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
JiaoshiEntity jiaoshi = jiaoshiService.selectById(id);
jiaoshi = jiaoshiService.selectView(new EntityWrapper<JiaoshiEntity>().eq("id", id));
return R.ok().put("data", jiaoshi);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
JiaoshiEntity jiaoshi = jiaoshiService.selectById(id);
jiaoshi = jiaoshiService.selectView(new EntityWrapper<JiaoshiEntity>().eq("id", id));
return R.ok().put("data", jiaoshi);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody JiaoshiEntity jiaoshi, HttpServletRequest request){
jiaoshi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(jiaoshi);
JiaoshiEntity u = jiaoshiService.selectOne(new EntityWrapper<JiaoshiEntity>().eq("jiaoshigonghao", jiaoshi.getJiaoshigonghao()));
if(u!=null) {
return R.error("用户已存在");
}
jiaoshi.setId(new Date().getTime());
jiaoshiService.insert(jiaoshi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody JiaoshiEntity jiaoshi, HttpServletRequest request){
jiaoshi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(jiaoshi);
JiaoshiEntity u = jiaoshiService.selectOne(new EntityWrapper<JiaoshiEntity>().eq("jiaoshigonghao", jiaoshi.getJiaoshigonghao()));
if(u!=null) {
return R.error("用户已存在");
}
jiaoshi.setId(new Date().getTime());
jiaoshiService.insert(jiaoshi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
public R update(@RequestBody JiaoshiEntity jiaoshi, HttpServletRequest request){
//ValidatorUtils.validateEntity(jiaoshi);
jiaoshiService.updateById(jiaoshi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
jiaoshiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
}
四、效果图
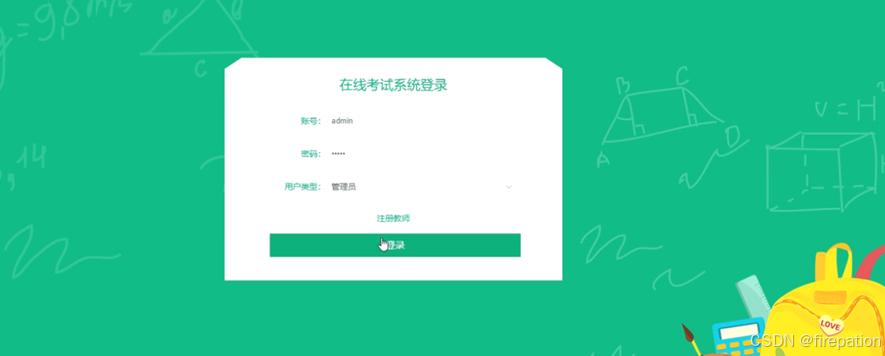
五 、源码获取
下方名片联系我即可!!
大家点赞、收藏、关注、评论啦 、查看👇🏻获取联系方式👇🏻