一,基本介绍
本文中主要介绍ESP32、ESP32S3系列单片机,基于Vscode Platform Arduino和Arduino框架下如何使用外部PSAM,以及必要的API调用函数进行内存分配和管理。
使用前提是开发板有外部PSRAM。
二,平台配置
2.1 Arduino平台
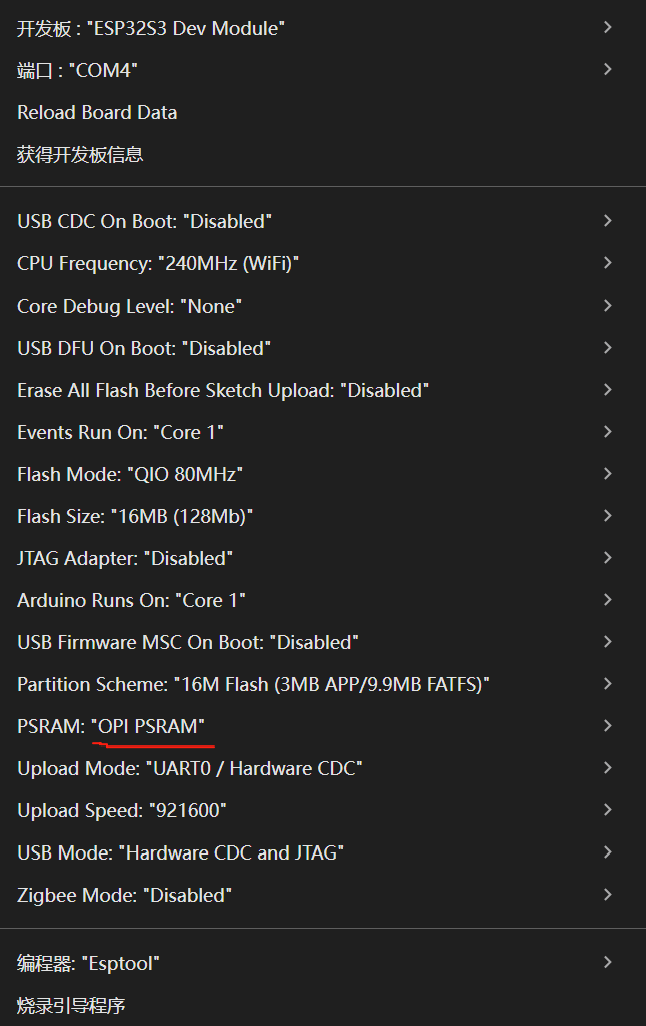
2.2 Platform IO
cpp
; PlatformIO Project Configuration File
;
; Build options: build flags, source filter
; Upload options: custom upload port, speed and extra flags
; Library options: dependencies, extra library storages
; Advanced options: extra scripting
;
; Please visit documentation for the other options and examples
; https://docs.platformio.org/page/projectconf.html
[env:esp32-s3-devkitc-1]
platform = espressif32
board = esp32-s3-devkitc-1
framework = arduino
;串口波特率
monitor_speed = 115200
;串口下载速度
upload_speed = 921600
;CPU的运行速度240Mhz
board_build.f_cpu = 240000000
;根据自己的开发板,选择分区表
board_build.partitions = default_16MB.csv
;外部PSRAM使用qio或者opi
board_build.arduino.memory_type = qio_opi
;通过该宏定义,启动外部的PSRAM
build_flags = -DBOARD_HAS_PSRAM
;外部Flash的大小
board_upload.flash_size = 16MB
三,内存测试代码
测试单片机为ESP32S3N16R8,具有16MB Flash 和8MB PSAM
cpp
#include <Arduino.h>
#include <esp_heap_caps.h>
void setup() {
Serial.begin(115200);
//查看ESP32堆的总共大小
Serial.printf("ESP32 total Heap size :%d bytes\n",ESP.getHeapSize());
//查看ESP32堆的可用大小
Serial.printf("ESP32 free Heap size :%d bytes\n",ESP.getFreeHeap());
//查看ESP32的Flash的大小
Serial.printf("Flash size: %d bytes\n", ESP.getFlashChipSize());
//查看ESP32的内部和外部RAM的总共大小
Serial.printf("Deafult total size: %d bytes\n", heap_caps_get_total_size(MALLOC_CAP_DEFAULT));
//查看ESP32的内部和外部RAM的可用大小
Serial.printf("Deafult free size: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_DEFAULT));
//查看ESP32的内部RAM总共大小
Serial.printf("Internal total size: %d bytes\n", heap_caps_get_total_size(MALLOC_CAP_INTERNAL));
//查看ESP32的内部RAM可用大小
Serial.printf("Internal free size: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_INTERNAL));
//查看ESP32的外部RAM的可用大小
Serial.printf("PSRAM total size: %d bytes\n", heap_caps_get_total_size(MALLOC_CAP_SPIRAM));
//查看ESP32的外部RAM的可用大小
Serial.printf("PSRAM free size: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_SPIRAM));
}
void loop() {
delay(10);
}
串口输出结果
四,内存申请与释放代码
测试单片机为ESP32S3N16R8
cpp
//从芯片内部申请PSARAM,大小为1000字节,数据类型为char型
char* str1=(char *)heap_caps_malloc(1000,MALLOC_CAP_INTERNAL);
//从芯片外部申请PSARAM,大小为1000字节,数据类型为char型
char* str1=(char *)heap_caps_malloc(1000,MALLOC_CAP_SPIRAM);
//内存释放
heap_caps_free(str);
cpp
#include <Arduino.h>
#include <esp_heap_caps.h>
void setup() {
Serial.begin(115200);
Serial.printf("内部RAM可使用大小: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_INTERNAL));
char* str=(char *)heap_caps_malloc(1000,MALLOC_CAP_INTERNAL);
sprintf(str,"hello world! I am a handsome boy!");
Serial.println(str);
Serial.printf("内部RAM可使用大小: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_INTERNAL));
Serial.printf("外部RAM可使用大小: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_SPIRAM));
char* str1=(char *)heap_caps_malloc(1000,MALLOC_CAP_SPIRAM);
sprintf(str1,"hello world! I am a pretty girl!");
Serial.println(str1);
Serial.printf("外部RAM可使用大小: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_SPIRAM));
Serial.println("释放内存!");
heap_caps_free(str);
heap_caps_free(str1);
Serial.printf("内部RAM可使用大小: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_INTERNAL));
Serial.printf("外部RAM可使用大小: %d bytes\n", heap_caps_get_free_size(MALLOC_CAP_SPIRAM));
}
void loop() {
delay(10);
}
串口输出结果
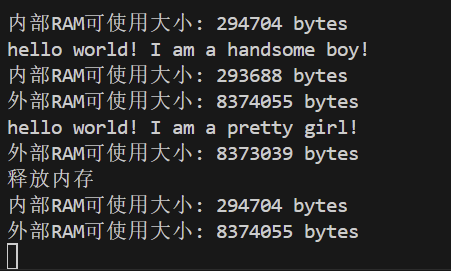