- 平衡二叉树
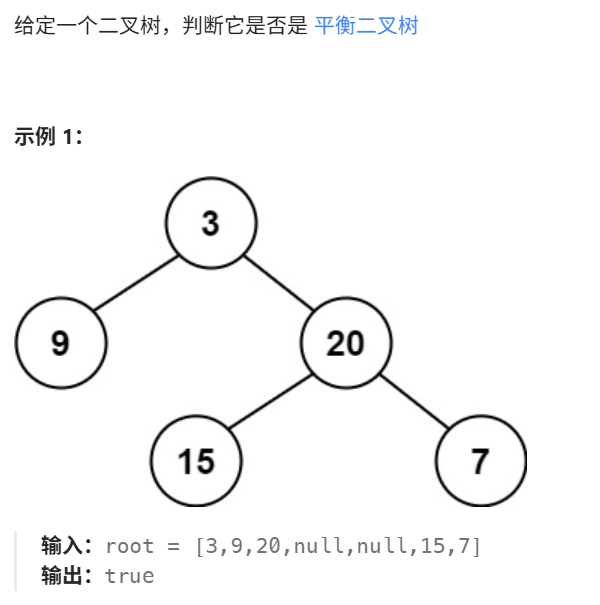
cpp
int postorder(TreeNode* root) {
if (root == NULL) return 0;
int l = postorder(root->left);
if (l == -1) return -1; // 只要出现-1 左子树中某个子树不是平衡二叉树,直接返回-1
int r = postorder(root->right);
if (r == -1) return -1;// 道理同上
if (abs(l - r) > 1) return -1; // 尽管左右子树都是完全二叉树,两颗子树高度差>1 说明整颗树不是平衡二叉树
int height = 1 + max(l,r);
return height; // 否则返回最大高度
}
bool isBalanced(TreeNode* root) {
if (postorder(root) == -1) return false;
return true;
}
257.二叉树的所有路径
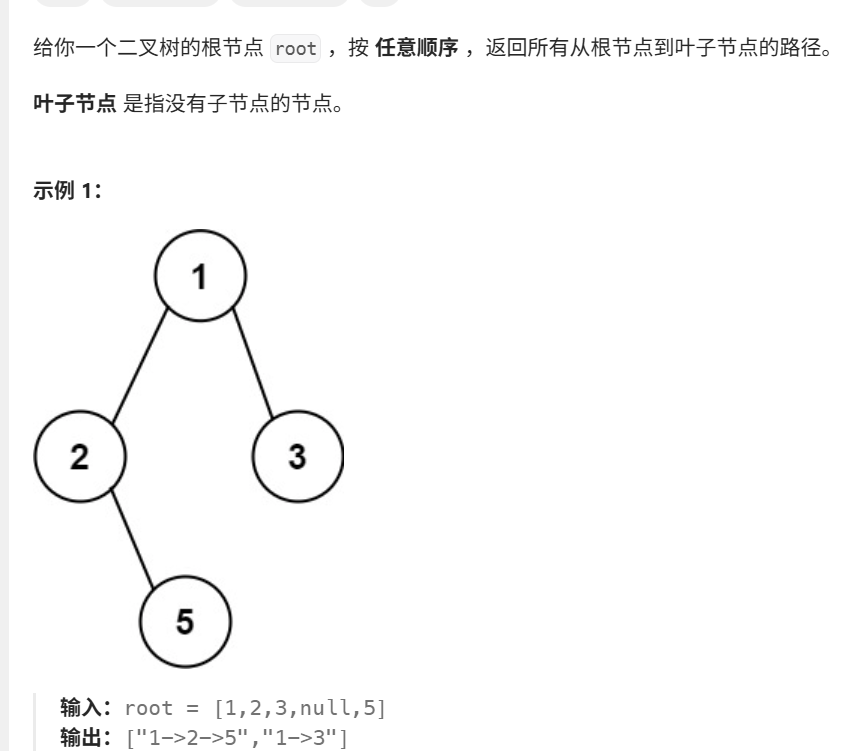
cpp
vector<string> res;
// 从根节点寻找所有路径,肯定是前序遍历,注意要保持字符串的输出格式
void dfs(TreeNode* root, string s) {
s += to_string(root->val);//
if (root->left == NULL && root->right == NULL) {
res.push_back(s);
return;
}
if (root -> left) dfs(root->left, s + "->");
if (root -> right) dfs(root->right, s + "->");
return;
}
vector<string> binaryTreePaths(TreeNode* root) {
res.clear();
if (root == NULL) return res;
string s;
dfs(root, s);
return res;
}
- 二叉树的左叶子之和
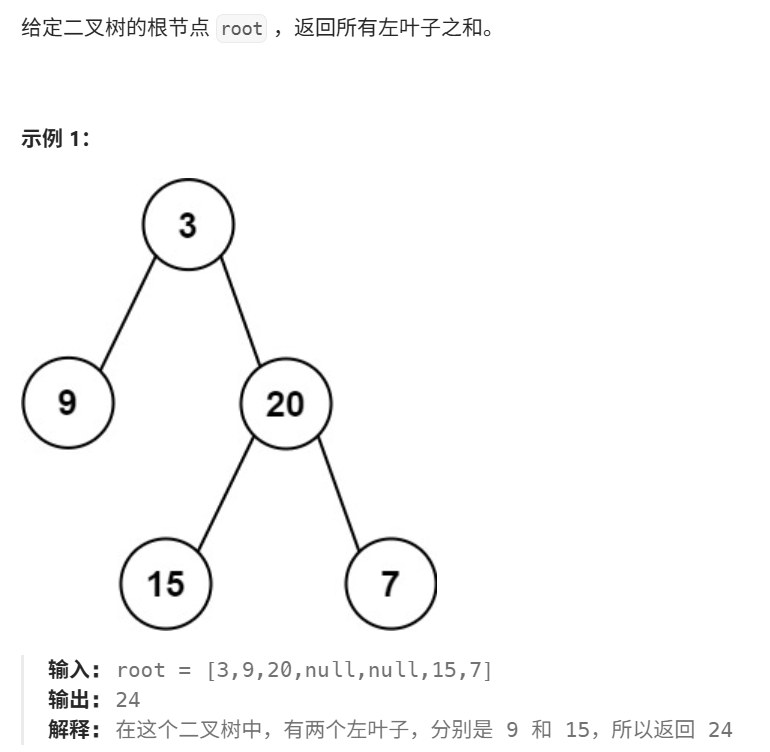
看到本题可能一下想到层序遍历,其实没有那么简单,因为找的是左叶子不是左侧节点, 使用递归的话后序最好
cpp
// 后序方便一些
int res = 0;
void dfs(TreeNode* root) {
// 看有无左儿子
if (root->left) {
// 判断左儿子是不是叶子
if (root->left->left == NULL && root->left->right == NULL) {
res += root->left->val;
// 不是叶子就向下找
}else dfs(root->left);
}
// 右儿子直接向下找
if (root->right) {
dfs (root->right);
}
return;
}
int sumOfLeftLeaves(TreeNode* root) {
// 节点数大于等于1
if (root -> left == NULL && root->right == NULL) return res; // 节点数等于1和为0
dfs(root);
return res;
}