文章目录
- 前言
- 一、代码部分
-
- [1. User](#1. User)
- 2.UserMapper1
- [3. UserSerivce](#3. UserSerivce)
- [4. UserController1](#4. UserController1)
- [5. Result](#5. Result)
- 二、测试
- 总结
前言
下面介绍用户注册接口。
一、代码部分
1. User
java
package org.example.springboot3.bigevent.entity;
import com.baomidou.mybatisplus.annotation.TableId;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import java.time.LocalDateTime;
@Getter
@Setter
@ToString
public class User {
@TableId
private Integer id;//主键ID
private String username;//用户名
private String password;//密码
private String nickname;//昵称
private String email;//邮箱
private String userPic;//用户头像地址
private LocalDateTime createTime;//创建时间
private LocalDateTime updateTime;//更新时间
}
2.UserMapper1
java
package org.example.springboot3.bigevent.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
import org.example.springboot3.bigevent.entity.User;
/**
* Create by zjg on 2024/5/22
*/
@Mapper
public interface UserMapper1 extends BaseMapper<User> {
}
3. UserSerivce
java
package org.example.springboot3.bigevent.service;
import org.example.springboot3.bigevent.entity.User;
/**
* Create by zjg on 2024/5/22
*/
public interface UserSerivce {
User findUserByName(String username);
int addUser(String username, String password);
}
java
package org.example.springboot3.bigevent.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import org.example.springboot3.bigevent.mapper.UserMapper1;
import org.example.springboot3.bigevent.entity.User;
import org.example.springboot3.bigevent.service.UserSerivce;
import org.example.springboot3.bigevent.utils.Md5Util;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.time.LocalDateTime;
/**
* Create by zjg on 2024/5/22
*/
@Service
public class UserSerivceImpl implements UserSerivce {
@Autowired
UserMapper1 userMapper1;
@Override
public User findUserByName(String username) {
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.ne("username", username);
return userMapper1.selectOne(queryWrapper);
}
@Override
public int addUser(String username, String password) {
User user = new User();
user.setUsername(username);
user.setPassword(Md5Util.getMD5String(password));
user.setCreateTime(LocalDateTime.now());
user.setUpdateTime(LocalDateTime.now());
return userMapper1.insert(user);
}
}
4. UserController1
java
package org.example.springboot3.bigevent.controller;
import org.example.springboot3.bigevent.entity.Result;
import org.example.springboot3.bigevent.entity.User;
import org.example.springboot3.bigevent.service.UserSerivce;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* Create by zjg on 2024/5/22
*/
@RequestMapping("/user/")
@RestController
public class UserController1 {
@Autowired
UserSerivce userSerivce;
@RequestMapping("register")
public Result register(String username, String password){
User user=userSerivce.findUserByName(username);
if(user==null){//用户不存在,可以注册
int i=userSerivce.addUser(username,password);
if(i!=1){
return Result.error("失败注册,请稍后重新注册!");
}
}else{
return Result.error("该用户已存在,请重新注册!");
}
return Result.success();
}
}
5. Result
java
package org.example.springboot3.bigevent.entity;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
//统一响应结果
@Data
@NoArgsConstructor
@AllArgsConstructor
public class Result<T> {
private Integer code;//业务状态码 0-成功 1-失败
private String message;//提示信息
private T data;//响应数据
//快速返回操作成功响应结果(带响应数据)
public static <E> Result<E> success(E data) {
return new Result<>(0, "操作成功", data);
}
//快速返回操作成功响应结果
public static Result success() {
return new Result(0, "操作成功", null);
}
public static Result error(String message) {
return new Result(1, message, null);
}
}
二、测试
1.注册
2.再次注册
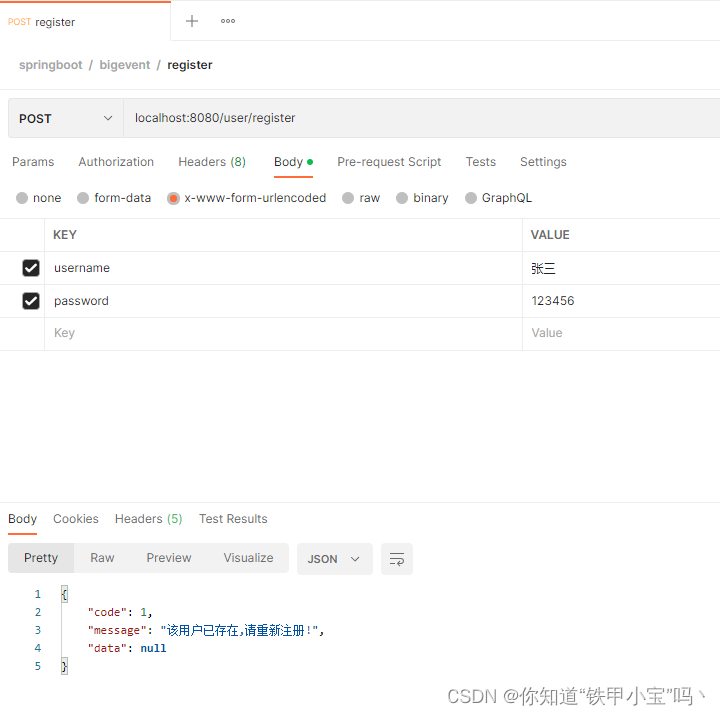