A. Array Divisibility
Given n𝑛, find an array of positive nonzero integers, with each element less than or equal to 105 that is beautiful subject to all 1≤𝑘≤𝑛.
It can be shown that an answer always exists.

#include<bits/stdc++.h>
using namespace std;
vector<int> findBeautifulArray(int n) {
vector<int> array(n);
for (int i = 0; i < n; ++i) {
array[i] = i + 1; // 选取每个数为其下标的平方
}
return array;
}
void solve(){
int n;
cin >> n;
vector<int> beautifulArray = findBeautifulArray(n);
for (int num : beautifulArray) {
cout << num << " ";
}
cout << endl;
}
int main(){
int T;
ios::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
cin >> T;
for(int i = 0; i < T; i ++)
solve();
return 0;
}
B. Corner Twist
You are given two grids of numbers a𝑎 and b𝑏, with n𝑛 rows and m𝑚 columns. All the values in the grid are 00, 11 or 22.
You can perform the following operation on a𝑎 any number of times:
-
Pick any subrectangle in the grid with length and width ≥2≥2. You are allowed to choose the entire grid as a subrectangle.
-
The subrectangle has four corners. Take any pair of diagonally opposite corners of the chosen subrectangle and add 11 to their values modulo 33.
-
For the pair of corners not picked, add 22 to their values modulo 33.
#include<bits/stdc++.h>
using namespace std;
void solve(){
int n, m;
cin >> n >> m;vector<vector<int>> a(n, vector<int>(m)), b(n, vector<int>(m)); for (int i = 0; i < n; ++i) { string s; cin >> s; for (int j = 0; j < m; ++j) a[i][j] = s[j] - '0'; } for (int i = 0; i < n; ++i) { string s; cin >> s; for (int j = 0; j < m; ++j) b[i][j] = s[j] - '0'; } for(int i = 0; i < n; i ++){ int sum1 = 0, sum2 = 0; for(int j = 0; j < m; j ++){ sum1 += a[i][j]; sum2 += b[i][j]; } if(sum1 % 3 != sum2 % 3){ cout << "NO" << endl; return ; } } for(int i = 0; i < m; i ++){ int sum1 = 0, sum2 = 0; for(int j = 0; j < n; j ++){ sum1 += a[j][i]; sum2 += b[j][i]; } if(sum1 % 3 != sum2 % 3){ cout << "NO" << endl; return ; } } cout << "Yes" << endl;
}
int main(){
int T;
ios::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
cin >> T;
for(int i = 0; i < T; i ++)
solve();
return 0;
}
C. Have Your Cake and Eat It Too
Given the values of each piece of the cake for each person, you need to give each person a contiguous slice of cake.In other words, the indices at the left and right ends of these subarrays (the slices given to each person) can be represented as (𝑙𝑎,𝑟𝑎), (𝑙𝑏,𝑟𝑏) and(𝑙𝑐,𝑟𝑐) respectively for Alice, Bob and Charlie. The division needs to satisfy the following constraints:

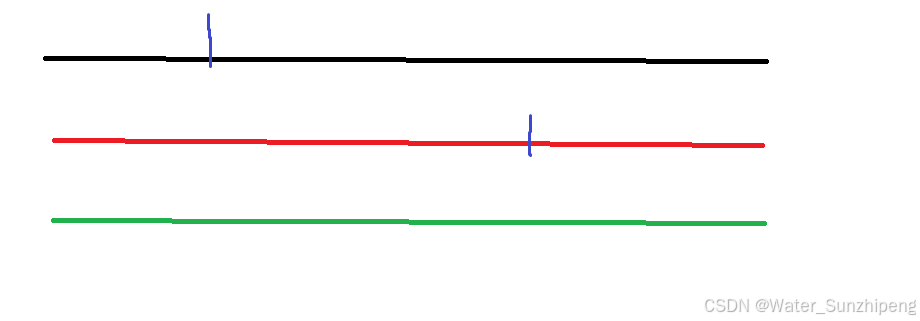
#include<bits/stdc++.h>
using namespace std;
void solve(){
int n;
cin >> n;
array<vector<int>, 3> a;
for(int i = 0; i < 3; i ++)
a[i].resize(n);
long long tot = 0;
for(int i = 0; i < 3; i ++){
for(int j = 0; j < n; j ++){
cin >> a[i][j];
if(i == 0)
tot += a[i][j];
}
}
array<int, 3> perm{0, 1, 2};
do{
array<int, 3> l{}, r{};
int cur = 0;
bool ok = true;
for(int i = 0; i < 3; i ++){
l[perm[i]] = cur + 1;
long long sum = 0;
while(sum < (tot + 2)/ 3 && cur < n)
sum += a[perm[i]][cur ++];
if(sum < (tot + 2) / 3){
ok = false;
break;
}
r[perm[i]] = cur;
}
if(ok){
for(int i = 0; i < 3; i ++)
cout << l[i] << " " << r[i] << " \n"[i == 2];
return ;
}
}while(next_permutation(perm.begin(), perm.end()));
cout << "-1\n";
return ;
}
int main(){
int T;
ios::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
cin >> T;
for(int i = 0; i < T; i ++)
solve();
return 0;
}
D. Swap Dilemma
Given two arrays of distinct positive integers a𝑎 and b𝑏 of length n𝑛, we would like to make both the arrays the same. Two arrays x𝑥 and y𝑦 of length k𝑘 are said to be the same when for all 1≤𝑖≤𝑘, 𝑥𝑖=𝑦𝑖.Now in one move, you can choose some index 𝑙 and 𝑟 in 𝑎 (𝑙≤𝑟) and swap 𝑎𝑙 and 𝑎𝑟, then choose some 𝑝 and 𝑞 (𝑝≤𝑞) in 𝑏 such that 𝑟−𝑙=𝑞−𝑝 and swap 𝑏𝑝 and 𝑏𝑞.
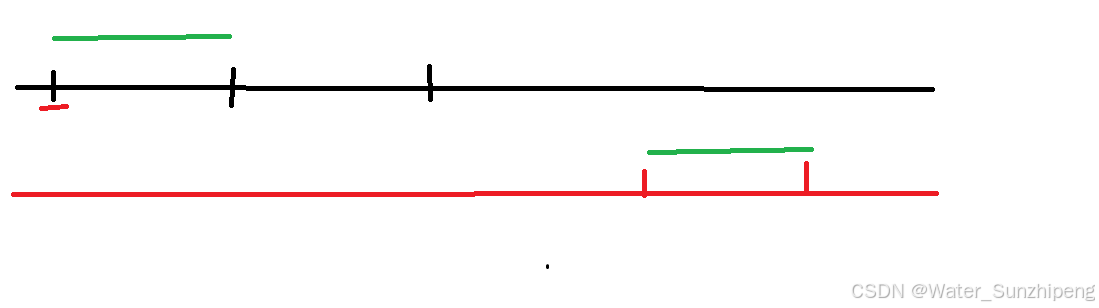
处理索引,在进行奇偶判断
#include<bits/stdc++.h>
using namespace std;
bool parity(const vector<int> a){
const int n = a.size();
vector<bool> vis(n);
int p = n % 2;
for(int i = 0; i < n; i ++){
if(vis[i])
continue;
for(int j = i; !vis[j] ; j = a[j])
vis[j] = true;
p ^= 1;
}
return p;
}
void solve(){
int n;
cin >> n;
vector<int> a(n), b(n);
for(int i = 0; i < n; i ++)
cin >> a[i];
for(int i = 0; i < n; i ++)
cin >> b[i];
auto va = a, vb = b;
sort(va.begin(), va.end());
sort(vb.begin(), vb.end());
if(va != vb){
cout << "NO" << endl;
return ;
}
for(int i = 0; i < n; i ++){
a[i] = lower_bound(va.begin(), va.end(), a[i]) - va.begin();
}
for(int i = 0; i < n; i ++){
b[i] = lower_bound(vb.begin(), vb.end(), b[i]) - vb.begin();
}
if(parity(a) != parity(b))
cout << "NO" << "\n";
else
cout << "YES" << "\n";
return ;
}
int main(){
int T;
ios::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
cin >> T;
for(int i = 0; i < T; i ++)
solve();
return 0;
}