栈的概念
栈是一种特殊的线性表,其只允许在固定的一端进行插入和删除元素操作。进行数据插入和删除操作的一端称为栈顶,另一端称为栈底。栈中的数据元素遵守后进先出LIFO(Last In First Out)的原则。
画个图理解一下
咱们可以观察到,进出数据只在栈顶进行
实现栈用数组最方便
栈的实现
cpp
#pragma once
#include<stdio.h>
#include<stdlib.h>
#include<stdbool.h>
#include<assert.h>
typedef int STDataType;
typedef struct Stack {
STDataType* a;
int top;
int capacity;
}ST;
void STInit(ST* pst);//初始化
void STDestroy(ST* pst);//销毁
void STPush(ST* pst, STDataType x);//入栈
void STPop(ST* pst);//出栈
STDataType STTop(ST* pst);//获取栈顶元素
int STSize(ST* pst);//获取数据个数
bool STEmpty(ST* pst);//判空
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"Stack.h"
void STInit(ST* pst)
{
assert(pst);
pst->a = NULL;
pst->capacity = 0;
pst->top = 0;
}
void STDestroy(ST* pst)
{
assert(pst);
free(pst->a);
pst->a = NULL;
pst->capacity = 0;
pst->top = 0;
}
void STPush(ST* pst, STDataType x)
{
assert(pst);
if (pst->capacity == pst->top) {
int newcapacity = pst->capacity == 0 ? 4 : pst->capacity * 2;
STDataType* temp = (STDataType*)realloc(pst->a, newcapacity * sizeof(STDataType));//扩容扩的是数组,不是结构体
if (temp == NULL) {
perror("realloc fail");
return;
}
pst->a = temp;
pst->capacity = newcapacity;
}
pst->a[pst->top] = x;
pst->top++;
}
void STPop(ST* pst)
{
assert(pst);
assert(pst->top > 0);
pst->top--;
}
STDataType STTop(ST* pst) {
assert(pst);
return pst->a[pst->top - 1];
}
int STSize(ST* pst)
{
assert(pst);
return pst->top - 1;
}
bool STEmpty(ST* pst)
{
assert(pst);
return pst->top == 0;
}
栈的应用
let's看题目
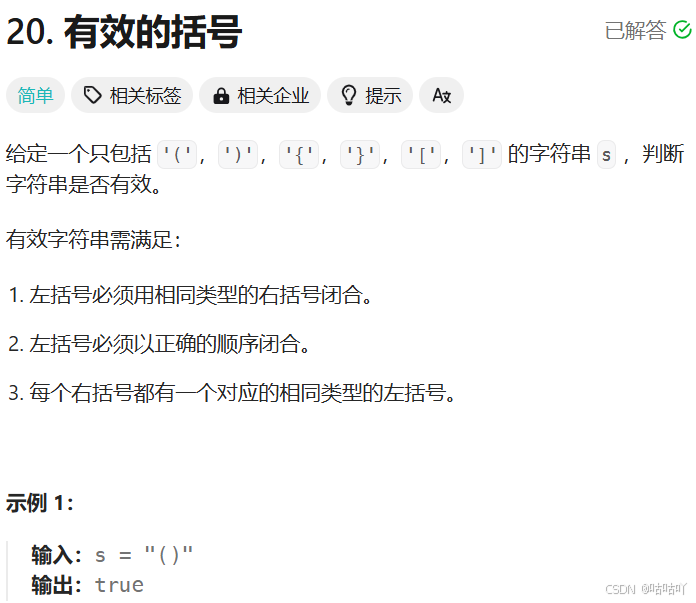
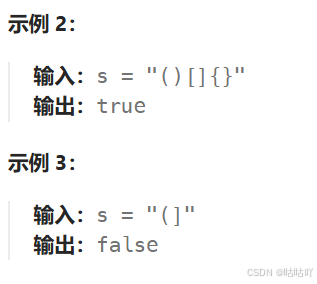
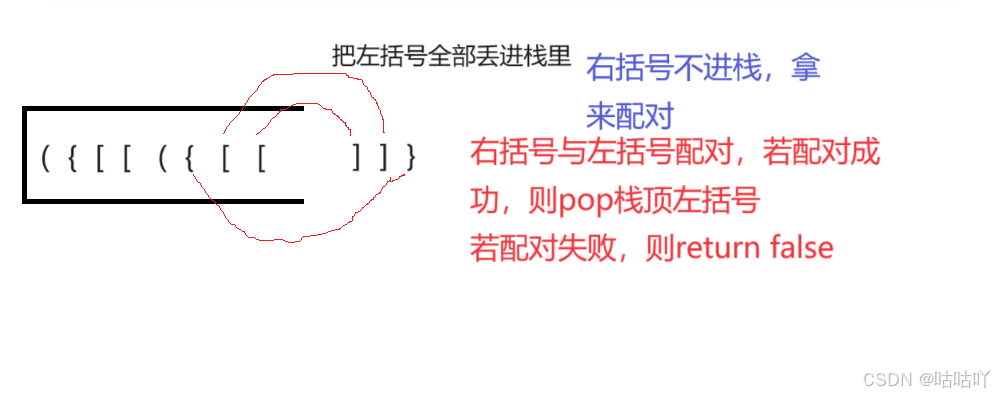
然后是代码实现
cpp
#include<stdio.h>
#include<stdlib.h>
#include<stdbool.h>
#include<assert.h>
typedef char STDataType;
typedef struct Stack {
STDataType* a;
int top;
int capacity;
}ST;
void STInit(ST* pst)
{
assert(pst);
pst->a = NULL;
pst->capacity = 0;
pst->top = 0;
}
void STDestroy(ST* pst)
{
assert(pst);
free(pst->a);
pst->a = NULL;
pst->capacity = 0;
pst->top = 0;
}
void STPush(ST* pst, STDataType x)
{
assert(pst);
if (pst->capacity == pst->top) {
int newcapacity = pst->capacity == 0 ? 4 : pst->capacity * 2;
ST* temp = (ST*)realloc(pst->a, newcapacity * sizeof(STDataType));//扩容扩的是数组,不是结构体
if (temp == NULL) {
perror("realloc fail");
return;
}
pst->a = temp;
pst->capacity = newcapacity;
}
pst->a[pst->top] = x;
pst->top++;
}
void STPop(ST* pst)
{
assert(pst);
assert(pst->top > 0);
pst->top--;
}
STDataType STTop(ST* pst) {
assert(pst);
return pst->a[pst->top - 1];
}
int STSize(ST* pst)
{
assert(pst);
return pst->top - 1;
}
bool STEmpty(ST* pst)
{
assert(pst);
return pst->top == 0;
}
bool isValid(char* s) {
ST st;
STInit(&st);
while(*s){
if(*s=='('||*s=='{'||*s=='['){
STPush(&st,*s);
}
else{
if(STEmpty(&st)){
return false;
}
char top=STTop(&st);
STPop(&st);
if((top=='('&&*s!=')')||(top=='{'&&*s!='}')||(top=='['&&*s!=']')){
STDestroy(&st);
return false;
}
}
++s;
}
bool ret=STEmpty(&st);
STDestroy(&st);
return ret;
}