server端代码:
python
#encoding=utf-8
# 服务端代码
import socket
def server():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
host = socket.gethostname()
port = 12345
server_socket.bind((host, port))
server_socket.listen(5)
print('等待客户端连接...')
while True:
client_socket, addr = server_socket.accept()
print('连接地址:', addr)
while True:
message = client_socket.recv(1024).decode('utf-8')
if not message:
break
print(f"客户端消息:{message}")
# 服务端回复消息
reply = input('回复客户端:')
client_socket.send(reply.encode('utf-8'))
client_socket.close()
if __name__ == '__main__':
server()
Client端代码:
python
# 客户端代码
import socket
def client():
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
host='xxxxx'
port = 12345
client_socket.connect((host, port))
while True:
message = input('发送消息:')
client_socket.send(message.encode('utf-8'))
# 接收服务端消息
server_message = client_socket.recv(1024).decode('utf-8')
print(f"服务端消息:{server_message}")
client_socket.close()
if __name__ == '__main__':
client()
运行后的结果:
Client:
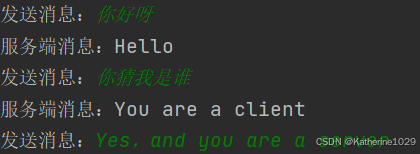
Server端:

host是主机的Device 名字
服务器与客户端建立 TCP 通信连接的交互过程:
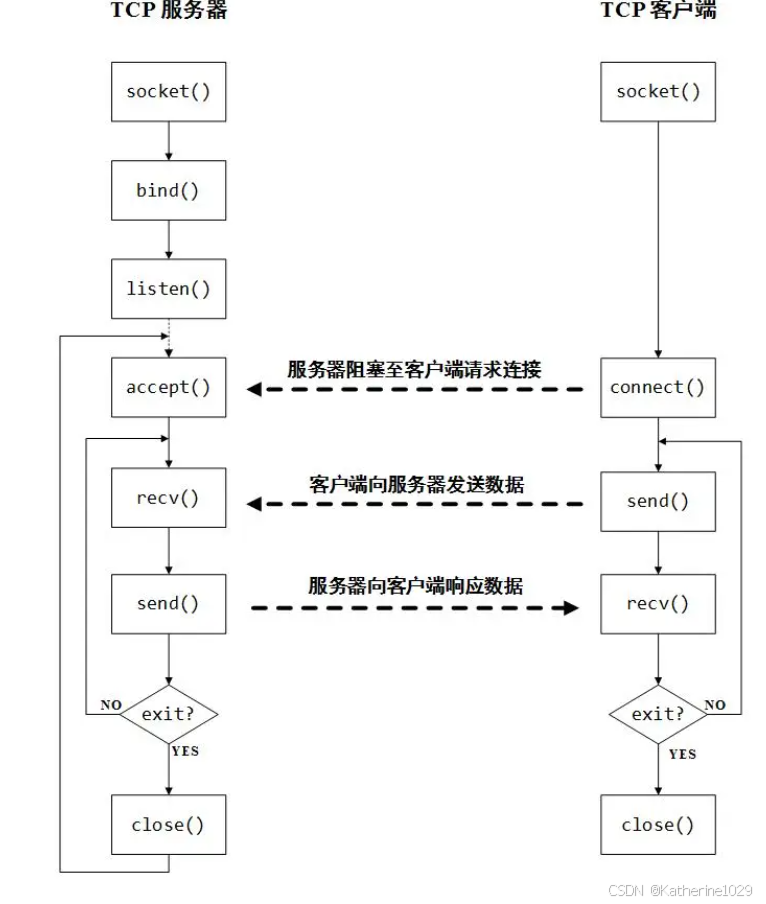
UDP Server:
python
# UDP服务器端代码
import socket
# 创建套接字
server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 绑定地址和端口
server_address = ('localhost', 12345)
server_socket.bind(server_address)
# 接收数据
data, client_address = server_socket.recvfrom(1024)
print(f"Received: {data.decode()} from {client_address}")
# 关闭套接字
server_socket.close()
UDP Client:
python
# UDP客户端代码
import socket
# 创建套接字
client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 发送数据
message = "Hello, server!"
server_address = ('localhost', 12345)
client_socket.sendto(message.encode(), server_address)
# 关闭套接字
client_socket.close()
运行结果:
