目录
- [1- 思路](#1- 思路)
- [2- 实现](#2- 实现)
-
- [⭐234. 回文链表------题解思路](#⭐234. 回文链表——题解思路)
- [3- ACM 实现](#3- ACM 实现)
- 原题连接:234. 回文链表
1- 思路
快慢指针+链表拆分+反转链表
思路
①将链表拆分前后两个部分------>找拆分点 、②反转后面部分 、③根据反转结果,同时利用两个指针遍历
- ① 找拆分点:快慢指针
- 利用快慢指针,满指针的 next 就是 后半部分的头指针
- ② 反转链表
- 递归反转后半部分
- ③ 遍历判断
- 依次同时移动两个指针判断
2- 实现
⭐234. 回文链表------题解思路
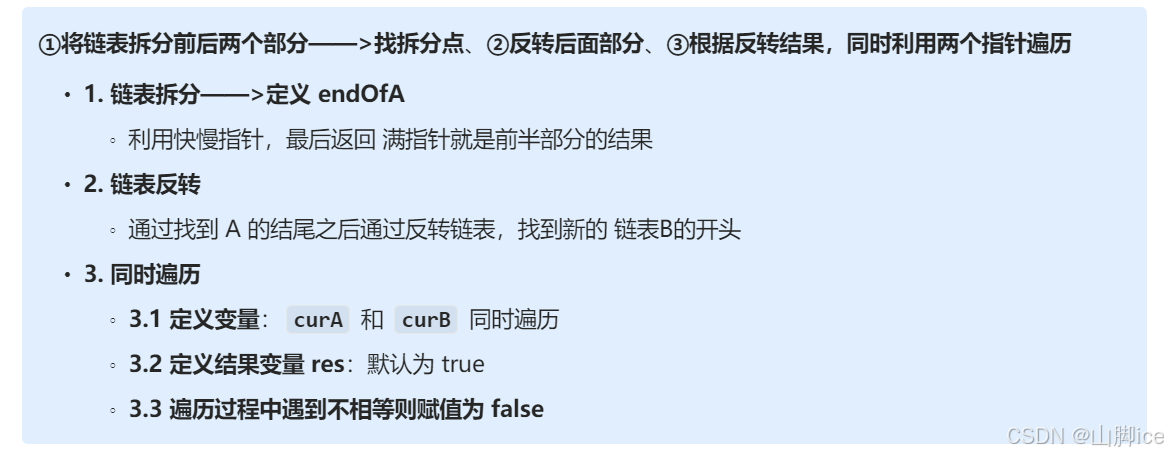
java
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public boolean isPalindrome(ListNode head) {
ListNode endA = endOfA(head);
boolean res = true;
ListNode headB = reverseL(endA.next);
ListNode curA = head;
ListNode curB = headB;
while(res && curB!=null){
if(curA.val != curB.val){
res = false;
}
curA = curA.next;
curB = curB.next;
}
// 恢复 B
reverseL(headB);
return res;
}
public ListNode endOfA(ListNode head){
ListNode slow = head;
ListNode fast = head;
while(fast.next!=null && fast.next.next!=null){
slow = slow.next;
fast = fast.next.next;
}
return slow;
}
public ListNode reverseL(ListNode head){
if(head==null || head.next==null){
return head;
}
ListNode cur = reverseL(head.next);
head.next.next = head;
head.next = null;
return cur;
}
}
3- ACM 实现
java
public class isPalindrome {
public static class ListNode {
int val;
ListNode next;
ListNode(int x) {
val = x;
next = null;
}
}
public static boolean isP(ListNode head){
ListNode endA = endOfA(head);
// 反转
ListNode headB = reverseL(endA.next);
ListNode curA = head;
ListNode curB = headB;
boolean res = true;
while(curB!=null){
if(curA.val != curB.val){
res = false;
}
curA = curA.next;
curB = curB.next;
}
return res;
}
private static ListNode endOfA(ListNode head){
ListNode slow = head;
ListNode fast = head;
while(fast.next!=null && fast.next.next!=null){
slow = slow.next;
fast = fast.next;
}
return slow;
}
private static ListNode reverseL(ListNode head){
if(head == null || head.next==null){
return head;
}
ListNode cur = reverseL(head.next);
head.next.next = head;
head.next = null;
return cur;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("输入链表长度");
int n = sc.nextInt();
ListNode head = null,tail=null;
for(int i = 0 ; i < n;i++){
ListNode nowNode = new ListNode(sc.nextInt());
if(head==null){
head = nowNode;
tail = nowNode;
}else{
tail.next = nowNode;
tail = nowNode;
}
}
System.out.println("结果是"+isP(head));
}
}