目录
顺序表概念
顺序表是用一段 物理地址连续 的存储单元依次存储数据元素的线性结构,一般情况下采用数组存储。在数组上完成数据的增删查改。
顺序表一般可以分为:
- 静态顺序表:使用定长数组存储元素 。
- 动态顺序表:使用动态开辟的数组存储。
顺序表初始化
cpp
void SLInit(SL* psl)
{
assert(psl);
psl->a = NULL;
psl->size = 0;
psl->capacity = 0;
}
顺序表销毁
cpp
void SLDestory(SL* psl)
{
if (psl->a != NULL)
{
free(psl->a);
psl->a = NULL;
psl->size = 0;
psl->capacity = 0;
}
}
顺序表尾插
- 尾插不需要扩容的直接插入即可
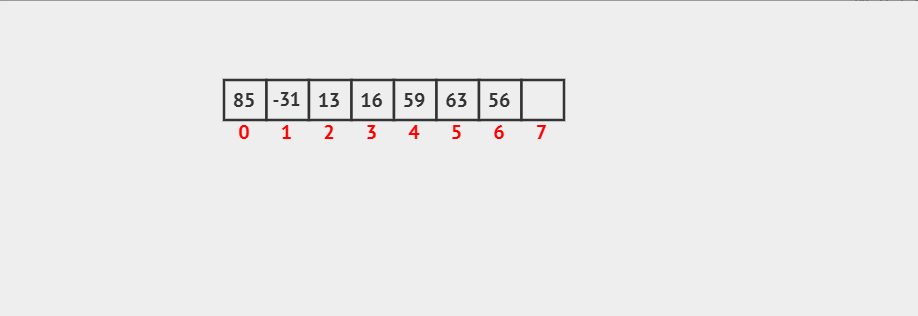
- 尾插需要扩容的
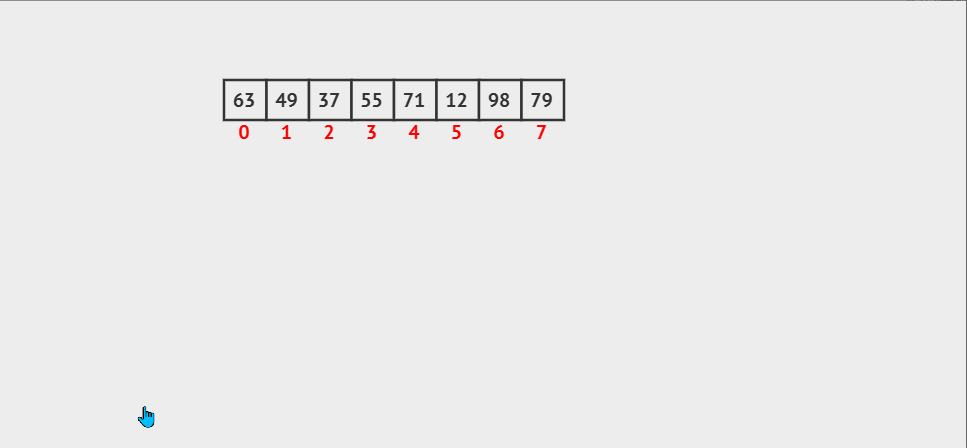
尾插的代码如下:
cpp
void SLPushBack(SL* psl, SLDateType x)
{
SLCheackCapacity(psl);
psl->a[psl->size++] = x;
}
顺序表尾删
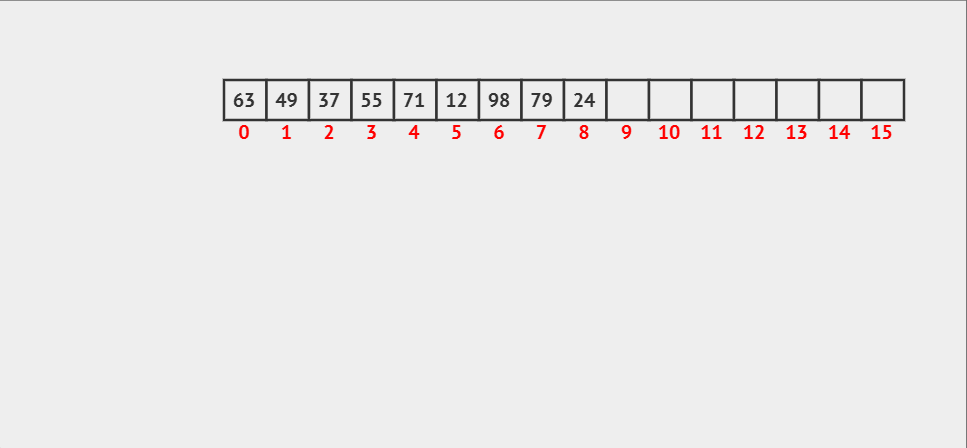
尾删代码如下:
cpp
void SLPopBack(SL* psl)
{
assert(psl->size > 0);
psl->size--;
}
顺序表头删
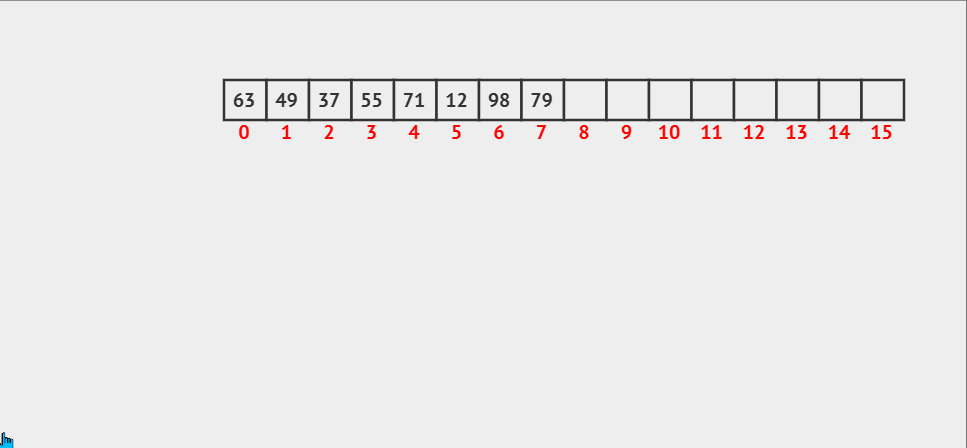
顺序表头删代码如下
cpp
void SLPopFront(SL* psl)
{
assert(psl->size > 0);
int begin = 0;
while (begin <psl->size-1 )
{
psl->a[begin] = psl->a[begin + 1];
begin++;
}
psl->size--;
/*int start = psl->a[0];
while (1)
{
psl->a[] = psl > a[psl->];
}*/
}
顺序表头插
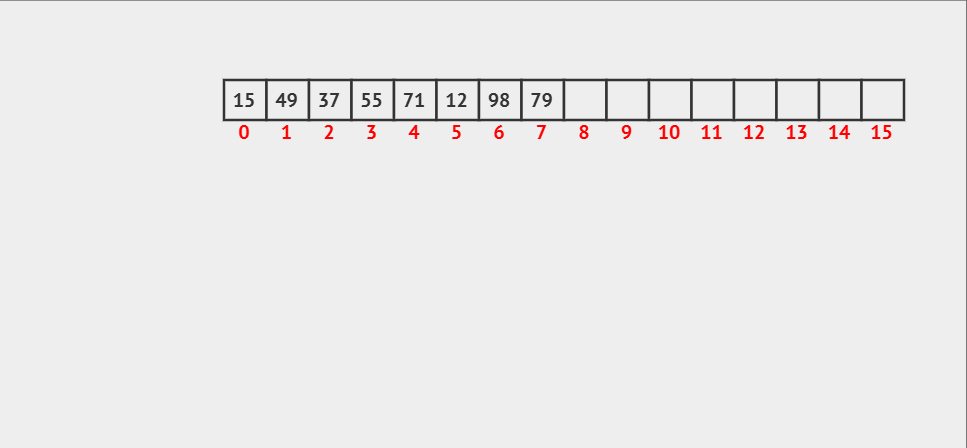
顺序表头插代码如下:
cpp
void SLPushFront(SL* psl, SLDateType x)
{
//检查空间够不够
SLCheackCapacity(psl);
int end = psl->size - 1;
while (end >= 0)
{
psl->a[end + 1] = psl->a[end];
--end;
}
psl->a[0] = x;
psl->size++;
}
总结:顺序表的头删头插的复杂度极高,如果想进行头删头插需要将数据一个一个的挪动,建议:不要使用顺序表进行头删头插,效率太低了
顺序表pos位置插入
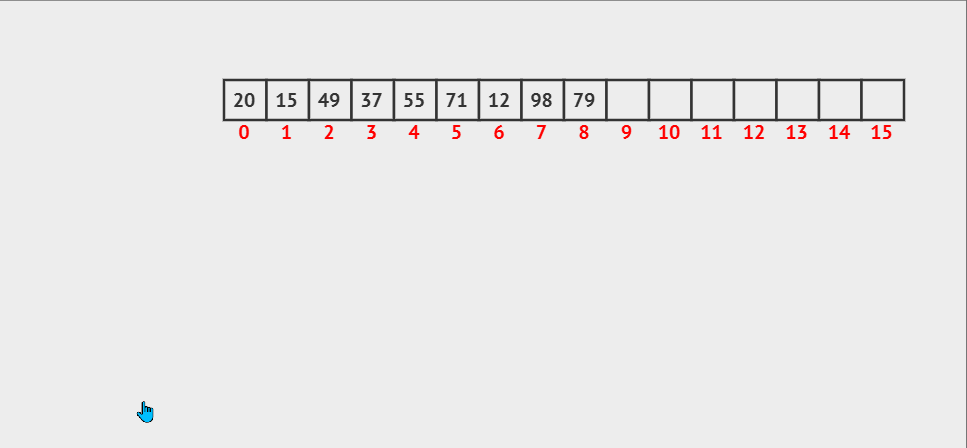
顺序表pos位置插入代码如下
cpp
void SLInert(SL* psl, int pos, SLDateType x)
{
assert(psl);
assert(pos >= 0 && pos <= psl->size);
SLCheackCapacity(psl);
int end = psl->size - 1;
while (end >= pos)
{
psl->a[end + 1] = psl->a[end];
--end;
}
psl->a[pos] = x;
psl->size++;
}
顺序表pos位置删除
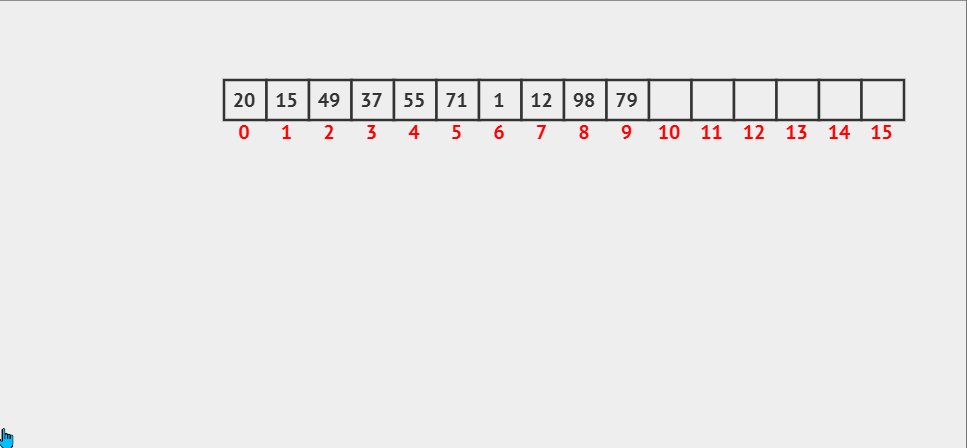
顺序表pos位置删除代码如下:
cpp
void SLErace(SL* psl, int pos, SLDateType x)
{
assert(psl);
assert(pos >= 0 && pos < psl->size);
int begin = pos + 1;
//往前覆盖
while (begin < psl->size)
{
psl->a[begin - 1] = psl->a[begin];
begin++;
}
psl->size--;
}
有没有发现我们如果想快速找到pos位置的值我可以可以通过下标进行找到。
顺序表全部代码如下:
cpp
void SLInit(SL* psl)
{
assert(psl);
psl->a = NULL;
psl->size = 0;
psl->capacity = 0;
}
void SLDestory(SL* psl)
{
if (psl->a != NULL)
{
free(psl->a);
psl->a = NULL;
psl->size = 0;
psl->capacity = 0;
}
}
void SLPushBack(SL* psl, SLDateType x)
{
SLCheackCapacity(psl);
psl->a[psl->size++] = x;
}
void SLPrint(SL* psl)
{
for (int i = 0; i < psl->size; i++)
{
printf("%d ", psl->a[i]);
}
printf("\n");
}
void SLCheackCapacity(SL* psl)
{
if (psl->size == psl->capacity)
{
int newCapacity = psl->capacity == 0 ? 4 : psl->capacity * 2;
SLDateType* temp = realloc(psl->a, sizeof(SLDateType) * newCapacity); //扩容
if (temp == NULL)
{
perror("realloc fail:");
return;
}
psl->a = temp;
psl->capacity = newCapacity;
}
}
void SLPushFront(SL* psl, SLDateType x)
{
//检查空间够不够
SLCheackCapacity(psl);
int end = psl->size - 1;
while (end >= 0)
{
psl->a[end + 1] = psl->a[end];
--end;
}
psl->a[0] = x;
psl->size++;
}
void SLPopBack(SL* psl)
{
//if (psl->size == 0)
//{
// printf("顺序表现在是空的状态!");
// return;
//}
assert(psl->size > 0);
psl->size--;
//不能free 不支持分期还款
//还有问题,若为空,在pop 就有问题了
/*assert(psl->size == 0);*/
}
void SLPopFront(SL* psl)
{
assert(psl->size > 0);
int begin = 0;
while (begin <psl->size-1 )
{
psl->a[begin] = psl->a[begin + 1];
begin++;
}
psl->size--;
/*int start = psl->a[0];
while (1)
{
psl->a[] = psl > a[psl->];
}*/
}
void SLInert(SL* psl, int pos, SLDateType x)
{
assert(psl);
assert(pos >= 0 && pos <= psl->size);
SLCheackCapacity(psl);
int end = psl->size - 1;
while (end >= pos)
{
psl->a[end + 1] = psl->a[end];
--end;
}
psl->a[pos] = x;
psl->size++;
}
void SLErace(SL* psl, int pos, SLDateType x)
{
assert(psl);
assert(pos >= 0 && pos < psl->size);
int begin = pos + 1;
//往前覆盖
while (begin < psl->size)
{
psl->a[begin - 1] = psl->a[begin];
begin++;
}
psl->size--;
}
int SlFind(SL* psl, int pos, SLDateType x)
{
assert(psl);
for (int i = 0; i < psl->size; i++)
{
if (psl->a[i] == x)
{
return i;
}
}
return -1;
}