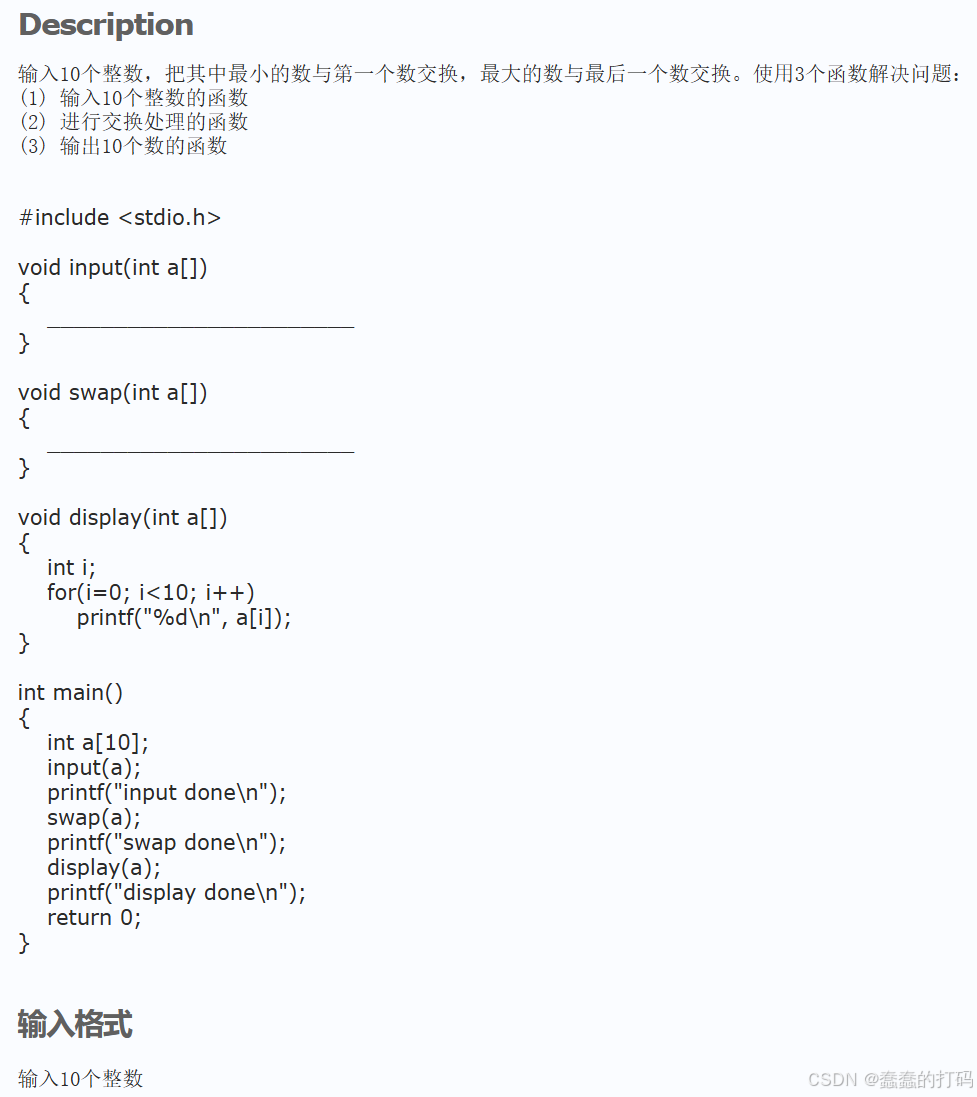
**思路**:
-
**输入函数**: 从用户输入中读取10个整数并存储在数组中。
-
**交换函数**: 找到数组中的最小值和最大值,分别与第一个和最后一个元素交换。
-
**输出函数**: 输出数组中的所有元素。
**伪代码**:
- **输入函数**:
- 使用循环读取10个整数并存储在数组中。
- **交换函数**:
-
初始化最小值和最大值的索引为0。
-
遍历数组,找到最小值和最大值的索引。
-
交换最小值与第一个元素,最大值与最后一个元素。
- **输出函数**:
- 使用循环输出数组中的所有元素。
**C代码**:
cpp
#include <stdio.h>
void input(int a[])
{
for(int i = 0; i < 10; i++)
{
scanf("%d", &a[i]);
}
}
void swap(int a[])
{
int minIndex = 0, maxIndex = 0;
for(int i = 1; i < 10; i++)
{
if(a[i] < a[minIndex])
minIndex = i;
if(a[i] > a[maxIndex])
maxIndex = i;
}
// Swap the minimum value with the first element
int temp = a[0];
a[0] = a[minIndex];
a[minIndex] = temp;
// If the maximum value was at the first position, update its index
if(maxIndex == 0)
maxIndex = minIndex;
// Swap the maximum value with the last element
temp = a[9];
a[9] = a[maxIndex];
a[maxIndex] = temp;
}
void display(int a[])
{
for(int i = 0; i < 10; i++)
{
printf("%d\n", a[i]);
}
}
int main()
{
int a[10];
input(a);
printf("input done\n");
swap(a);
printf("swap done\n");
display(a);
printf("display done\n");
return 0;
}